C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
Display Class Reference
Display class Functions for use with the Midas 16x2 I2C LCD Display (MCCOG21605x6W) LCD Farnell part 2218942 or 2063206. More...
#include <display.h>
Public Member Functions | |
Display () | |
Create the LCD Display object connected to the default pins (sda = p28, scl = p27, reset = p29, backlight = p30) | |
Display (PinName sda, PinName scl, PinName reset, PinName backlight) | |
Create the LCD Display object connected to specific pins. | |
void | clear_display (void) |
Clear the display. | |
void | home (void) |
Set cursor to home position. | |
void | write_string (char *message) |
Print string message. | |
void | write_string (char *message, char length) |
Print string message of given length. | |
void | set_position (char row, char column) |
Set the row and column of cursor position. | |
void | set_cursor (char enable) |
Enable or disable cursor. | |
void | set_blink (char enable) |
Enable or disable cursor blink. | |
void | set_display (char enable) |
Enable or disable display. | |
void | set_backlight_brightness (float brightness) |
Set the brightness of the backlight. |
Detailed Description
Display class Functions for use with the Midas 16x2 I2C LCD Display (MCCOG21605x6W) LCD Farnell part 2218942 or 2063206.
Example:
#include "psiswarm.h" int main() { init(); display.clear_display; //Clears display display.set_position(0,2); //Set cursor to row 0 column 2 display.write_string("YORK ROBOTICS"); display.set_position(1,3); //Set cursor to row 1 column 3 display.write_string("LABORATORY"); }
Definition at line 53 of file display.h.
Constructor & Destructor Documentation
Display | ( | ) |
Create the LCD Display object connected to the default pins (sda = p28, scl = p27, reset = p29, backlight = p30)
Definition at line 47 of file display.cpp.
Display | ( | PinName | sda, |
PinName | scl, | ||
PinName | reset, | ||
PinName | backlight | ||
) |
Create the LCD Display object connected to specific pins.
- Parameters:
-
sda pin - default is p28 scl pin - default is p27 reset pin - default is p29 backlight pin - default is p30
Definition at line 44 of file display.cpp.
Member Function Documentation
void clear_display | ( | void | ) |
Clear the display.
Definition at line 230 of file display.cpp.
void home | ( | void | ) |
Set cursor to home position.
Definition at line 238 of file display.cpp.
void set_backlight_brightness | ( | float | brightness ) |
Set the brightness of the backlight.
- Parameters:
-
brightness - Sets the brightness of the display (range 0.0 to 1.0)
Definition at line 198 of file display.cpp.
void set_blink | ( | char | enable ) |
Enable or disable cursor blink.
- Parameters:
-
enable - Set to 1 to enable the cursor blinking mode
Definition at line 188 of file display.cpp.
void set_cursor | ( | char | enable ) |
Enable or disable cursor.
- Parameters:
-
enable - Set to 1 to enable the cursor visibility
Definition at line 183 of file display.cpp.
void set_display | ( | char | enable ) |
Enable or disable display.
- Parameters:
-
enable - Set to 1 to enable the display output
Definition at line 193 of file display.cpp.
void set_position | ( | char | row, |
char | column | ||
) |
Set the row and column of cursor position.
- Parameters:
-
row - The row of the display to set the cursor to (either 0 or 1) column - The column of the display to set the cursor to (range 0 to 15)
Definition at line 174 of file display.cpp.
void write_string | ( | char * | message, |
char | length | ||
) |
Print string message of given length.
- Parameters:
-
message - The message to print length - The number of characters to display
Definition at line 154 of file display.cpp.
void write_string | ( | char * | message ) |
Print string message.
- Parameters:
-
message - The null-terminated message to print
Definition at line 131 of file display.cpp.
Generated on Tue Jul 12 2022 21:11:24 by
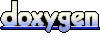