C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
display.h
00001 /* University of York Robotics Laboratory PsiSwarm Library: Display Driver Header File 00002 * 00003 * Copyright 2016 University of York 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 00007 * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS 00008 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00009 * See the License for the specific language governing permissions and limitations under the License. 00010 * 00011 * File: display.h 00012 * 00013 * (C) Dept. Electronics & Computer Science, University of York 00014 * James Hilder, Alan Millard, Alexander Horsfield, Homero Elizondo, Jon Timmis 00015 * 00016 * PsiSwarm Library Version: 0.8 00017 * 00018 * October 2016 00019 * 00020 * 00021 * Driver for the Midas 16x2 I2C LCD Display (MCCOG21605x6W) LCD 00022 * 00023 * Farnell part 2218942 or 2063206 00024 * 00025 */ 00026 00027 00028 #ifndef DISPLAY_H 00029 #define DISPLAY_H 00030 00031 #define PAGE_TIME 0.4 00032 #define CLEAR_TIME 0.8 00033 00034 /** 00035 * Display class 00036 * Functions for use with the Midas 16x2 I2C LCD Display (MCCOG21605x6W) LCD 00037 * Farnell part 2218942 or 2063206 00038 * 00039 * Example: 00040 * @code 00041 * #include "psiswarm.h" 00042 * 00043 * int main() { 00044 * init(); 00045 * display.clear_display; //Clears display 00046 * display.set_position(0,2); //Set cursor to row 0 column 2 00047 * display.write_string("YORK ROBOTICS"); 00048 * display.set_position(1,3); //Set cursor to row 1 column 3 00049 * display.write_string("LABORATORY"); 00050 * } 00051 * @endcode 00052 */ 00053 class Display : public Stream 00054 { 00055 00056 // Public Functions 00057 00058 public: 00059 00060 /** Create the LCD Display object connected to the default pins 00061 * (sda = p28, scl = p27, reset = p29, backlight = p30) 00062 */ 00063 Display(); 00064 00065 /** Create the LCD Display object connected to specific pins 00066 * 00067 * @param sda pin - default is p28 00068 * @param scl pin - default is p27 00069 * @param reset pin - default is p29 00070 * @param backlight pin - default is p30 00071 */ 00072 Display(PinName sda, PinName scl, PinName reset, PinName backlight); 00073 00074 /** Clear the display 00075 */ 00076 void clear_display(void); 00077 00078 /** Set cursor to home position 00079 */ 00080 void home(void); 00081 00082 /** Print string message 00083 * @param message - The null-terminated message to print 00084 */ 00085 void write_string(char * message); 00086 00087 /** Print string message of given length 00088 * @param message - The message to print 00089 * @param length - The number of characters to display 00090 */ 00091 void write_string(char * message, char length); 00092 00093 /** Set the row and column of cursor position 00094 * @param row - The row of the display to set the cursor to (either 0 or 1) 00095 * @param column - The column of the display to set the cursor to (range 0 to 15) 00096 */ 00097 void set_position(char row, char column); 00098 00099 /** Enable or disable cursor 00100 * @param enable - Set to 1 to enable the cursor visibility 00101 */ 00102 void set_cursor(char enable); 00103 00104 /** Enable or disable cursor blink 00105 * @param enable - Set to 1 to enable the cursor blinking mode 00106 */ 00107 void set_blink(char enable); 00108 00109 /** Enable or disable display 00110 * @param enable - Set to 1 to enable the display output 00111 */ 00112 void set_display(char enable); 00113 00114 /** Set the brightness of the backlight 00115 * @param brightness - Sets the brightness of the display (range 0.0 to 1.0) 00116 */ 00117 void set_backlight_brightness(float brightness); 00118 00119 // Special function for when debug messages are sent to display 00120 void debug_page(char * message, char length); 00121 00122 // Internal function used to restore display after debug messages 00123 void IF_restore_page(void); 00124 00125 // Internal function used to show multi-page debug messages 00126 void IF_debug_multipage(void); 00127 00128 // Internal function used to toggle backlight 00129 void IF_backlight_toggle(void); 00130 00131 //Parts of initialisation routine 00132 void post_init(void); 00133 void post_post_init(void); 00134 00135 // Send a 1-byte control message to the display 00136 int i2c_message(char byte); 00137 00138 // Default initialisation sequence for the display 00139 void init_display(char mode); 00140 00141 int disp_putc(int c); 00142 00143 00144 private : 00145 00146 I2C _i2c; 00147 DigitalOut _reset; 00148 DigitalOut _backlight; 00149 00150 char display_on; 00151 char cursor_on; 00152 char blink_on; 00153 00154 void _set_display(); 00155 00156 virtual int _putc(int c); 00157 virtual int _getc(); 00158 00159 }; 00160 00161 #endif // DISPLAY_H
Generated on Tue Jul 12 2022 21:11:24 by
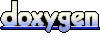