C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
animations.cpp
00001 /* University of York Robotics Laboratory PsiSwarm Library: Animations Source File 00002 * 00003 * Copyright 2016 University of York 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 00007 * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS 00008 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00009 * See the License for the specific language governing permissions and limitations under the License. 00010 * 00011 * Library of simple predetermined movements and LED animations 00012 * 00013 * File: animations.cpp 00014 * [Was dances.cpp in version 0.7] 00015 * 00016 * (C) Dept. Electronics & Computer Science, University of York 00017 * James Hilder, Alan Millard, Alexander Horsfield, Homero Elizondo, Jon Timmis 00018 * 00019 * PsiSwarm Library Version: 0.8 00020 * 00021 * October 2016 00022 * 00023 * 00024 */ 00025 00026 00027 #include "psiswarm.h" 00028 00029 char hold_colour = 1; 00030 // 00031 void Animations::set_colour(char colour) 00032 { 00033 hold_colour = colour; 00034 } 00035 00036 void Animations::led_run1() 00037 { 00038 if(animation_counter == 0)led.save_led_states(); 00039 char led_pattern = 0x0; 00040 if(animation_counter < 16){ 00041 switch(animation_counter % 4){ 00042 case 0: 00043 led_pattern = 0x10; 00044 break; 00045 case 1: 00046 led_pattern = 0x28; 00047 break; 00048 case 2: 00049 led_pattern = 0x44; 00050 break; 00051 case 3: 00052 led_pattern = 0x83; 00053 break; 00054 } 00055 } 00056 if(animation_counter == 16 || animation_counter == 18 || animation_counter == 20) led_pattern = 0x01; 00057 char green_state = 0; 00058 char red_state = 0; 00059 if(hold_colour % 2 == 1) red_state = led_pattern; 00060 if(hold_colour > 1) green_state = led_pattern; 00061 led.set_leds(green_state,red_state); 00062 animation_counter++; 00063 if(animation_counter < 21) { 00064 animation_timeout.attach(this, &Animations::led_run1, 0.05f); 00065 } else { 00066 animation_counter = 0; 00067 led.restore_led_states(); 00068 } 00069 } 00070 00071 //Do a simple wiggle 00072 void Animations::vibrate(void) 00073 { 00074 if(animation_counter == 0)led.save_led_states(); 00075 if(animation_counter % 2 == 0) { 00076 led.set_leds(0xC7,0x00); 00077 motors.turn(1.0); 00078 } else { 00079 led.set_leds(0x00,0xC7); 00080 motors.turn(-1.0); 00081 } 00082 animation_counter++; 00083 00084 if(animation_counter < 14) { 00085 float wiggle_timeout_period = 0.06; 00086 //Move less on first 'wiggle' so that we stay in roughly the same place! 00087 if(animation_counter == 0) wiggle_timeout_period = 0.03; 00088 animation_timeout.attach(this, &Animations::vibrate, wiggle_timeout_period); 00089 } else { 00090 animation_counter = 0; 00091 motors.brake(); 00092 led.restore_led_states(); 00093 } 00094 }
Generated on Tue Jul 12 2022 21:11:24 by
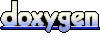