C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
Motors Class Reference
Motors class Functions to control the Psi Swarm robot motors. More...
#include <motors.h>
Public Member Functions | |
void | set_left_motor_speed (float speed) |
Set the left motor to the specified speed. | |
void | set_right_motor_speed (float speed) |
Set the left motor to the specified speed. | |
void | brake_left_motor (void) |
Enable the active brake on the left motor. | |
void | brake_right_motor (void) |
Enable the active brake on the right motor. | |
void | brake (void) |
Enable the active brake on the both motors. | |
void | stop (void) |
Stop both motors This sets the speed of both motors to 0; it does not enable the active brake. | |
void | forward (float speed) |
Sets both motors to the specified speed. | |
void | backward (float speed) |
Sets both motors to the specified inverted speed. | |
void | turn (float speed) |
Turn the robot on the spot by setting motors to equal and opposite speeds. | |
void | init_motors (void) |
Initialise the PWM settings for the motors. | |
void | time_based_forward (float speed, int microseconds, char brake) |
Make the robot move forward for a predetermined amount of time. | |
void | time_based_turn (float speed, int microseconds, char brake) |
Make the robot turn for a predetermined amount of time. |
Detailed Description
Motors class Functions to control the Psi Swarm robot motors.
Example:
#include "psiswarm.h" int main() { init(); motors.forward(0.5); //Set the motors to forward at speed 0.5 wait(0.5); motors.brake(); //Enable the hardware brake wait(0.5); motors.turn(0.5); //Turn clockwise at 50% speed wait(0.5); motors.stop(); //Sets motor speed to zero (but not hardware brake) }
Definition at line 46 of file motors.h.
Member Function Documentation
void backward | ( | float | speed ) |
Sets both motors to the specified inverted speed.
- Parameters:
-
speed - Set the motors to the specified speed (range -1.0 for max. forward to 1.0 for max. reverse)
Definition at line 83 of file motors.cpp.
void brake | ( | void | ) |
Enable the active brake on the both motors.
Definition at line 56 of file motors.cpp.
void brake_left_motor | ( | void | ) |
Enable the active brake on the left motor.
Definition at line 42 of file motors.cpp.
void brake_right_motor | ( | void | ) |
Enable the active brake on the right motor.
Definition at line 49 of file motors.cpp.
void forward | ( | float | speed ) |
Sets both motors to the specified speed.
- Parameters:
-
speed - Set the motors to the specified speed (range -1.0 for max. reverse to 1.0 for max. forward)
Definition at line 74 of file motors.cpp.
void init_motors | ( | void | ) |
Initialise the PWM settings for the motors.
Definition at line 299 of file motors.cpp.
void set_left_motor_speed | ( | float | speed ) |
Set the left motor to the specified speed.
- Parameters:
-
speed - The set motor to the specified (range -1.0 for max. reverse to 1.0 for max. forward)
Definition at line 28 of file motors.cpp.
void set_right_motor_speed | ( | float | speed ) |
Set the left motor to the specified speed.
- Parameters:
-
speed - The set motor to the specified (range -1.0 for max. reverse to 1.0 for max. forward)
Definition at line 35 of file motors.cpp.
void stop | ( | void | ) |
Stop both motors This sets the speed of both motors to 0; it does not enable the active brake.
Definition at line 65 of file motors.cpp.
void time_based_forward | ( | float | speed, |
int | microseconds, | ||
char | brake | ||
) |
Make the robot move forward for a predetermined amount of time.
- Parameters:
-
speed - Sets the motors to the specified speed (range -1.0 for max. forward to 1.0 for max. reverse) microseconds - The duration to keep moving brake - If set to 1, the brake instruction will be applied at the end of the move, else motors are just set to stop
Definition at line 103 of file motors.cpp.
void time_based_turn | ( | float | speed, |
int | microseconds, | ||
char | brake | ||
) |
Make the robot turn for a predetermined amount of time.
- Parameters:
-
speed - Sets the turning speed (range -1.0 for max. counter-clockwise to 1.0 for max. clockwise) microseconds - The duration to keep moving brake - If set to 1, the brake instruction will be applied at the end of the move, else motors are just set to stop
Definition at line 115 of file motors.cpp.
void turn | ( | float | speed ) |
Turn the robot on the spot by setting motors to equal and opposite speeds.
- Parameters:
-
speed - Sets the turning speed (range -1.0 for max. counter-clockwise to 1.0 for max. clockwise)
Definition at line 92 of file motors.cpp.
Generated on Tue Jul 12 2022 21:11:24 by
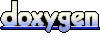