Library for LoRa communication using MultiTech MDOT.
Dependents: mDot_test_rx adc_sensor_lora mDotEVBM2X mDot_AT_firmware ... more
MTSCircularBuffer Class Reference
This class provides a circular byte buffer meant for temporary storage during IO transactions. More...
#include <MTSCircularBuffer.h>
Public Member Functions | |
MTSCircularBuffer (int bufferSize) | |
Creates an MTSCircularBuffer object with the specified static size. | |
~MTSCircularBuffer () | |
Destructs an MTSCircularBuffer object and frees all related resources. | |
int | read (char *data, int length) |
This method enables bulk reads from the buffer. | |
int | read (char &data) |
This method reads a single byte from the buffer. | |
int | write (const char *data, int length) |
This method enables bulk writes to the buffer. | |
int | write (char data) |
This method writes a signle byte as a char to the buffer. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void), int threshold, RelationalOperator op) |
This method is used to setup a callback funtion when the buffer reaches a certain threshold. | |
void | attach (void(*fptr)(void), int threshold, RelationalOperator op) |
This method is used to setup a callback funtion when the buffer reaches a certain threshold. | |
int | capacity () |
This method returns the size of the storage space currently allocated for the buffer. | |
int | remaining () |
This method returns the amount of space left for writing. | |
int | size () |
This method returns the number of bytes available for reading. | |
bool | isFull () |
This method returns whether the buffer is full. | |
bool | isEmpty () |
This method returns whether the buffer is empty. | |
void | clear () |
This method clears the buffer. |
Detailed Description
This class provides a circular byte buffer meant for temporary storage during IO transactions.
It contains many of the common methods you would expect from a circular buffer like read, write, and various methods for checking the size or status. It should be noted that this class does not include any special code for thread safety like a lock. In most cases this is not problematic, but is something to be aware of.
Definition at line 17 of file MTSCircularBuffer.h.
Constructor & Destructor Documentation
MTSCircularBuffer | ( | int | bufferSize ) |
Creates an MTSCircularBuffer object with the specified static size.
bufferSize size of the buffer in bytes.
~MTSCircularBuffer | ( | ) |
Destructs an MTSCircularBuffer object and frees all related resources.
Member Function Documentation
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
int | threshold, | ||
RelationalOperator | op | ||
) |
This method is used to setup a callback funtion when the buffer reaches a certain threshold.
The threshold condition is checked after every read and write call is completed. The condition is made up of both a threshold value and operator. An example that would trigger a callback is if the threshold was 10, the operator GREATER, and there were 12 bytes added to an empty buffer.
- Parameters:
-
tptr a pointer to the object to be called when the condition is met. mptr a pointer to the function within the object to be called when the condition is met. threshold the value in bytes to be used as part of the condition. op the operator to be used in conjunction with the threshold as part of the condition.
Definition at line 80 of file MTSCircularBuffer.h.
void attach | ( | void(*)(void) | fptr, |
int | threshold, | ||
RelationalOperator | op | ||
) |
This method is used to setup a callback funtion when the buffer reaches a certain threshold.
The threshold condition is checked after every read and write call is completed. The condition is made up of both a threshold value and operator. An example that would trigger a callback is if the threshold was 10, the operator GREATER, and there were 12 bytes added to an empty buffer.
- Parameters:
-
fptr a pointer to the static function to be called when the condition is met. threshold the value in bytes to be used as part of the condition. op the operator to be used in conjunction with the threshold as part of the condition.
Definition at line 99 of file MTSCircularBuffer.h.
int capacity | ( | ) |
This method returns the size of the storage space currently allocated for the buffer.
This value is equivalent to the one passed into the constructor. This value is equal or greater than the size() of the buffer.
- Returns:
- the allocated size of the buffer in bytes.
void clear | ( | ) |
This method clears the buffer.
This is done through setting the internal read and write indexes to the same value and is therefore not an expensive operation.
bool isEmpty | ( | ) |
This method returns whether the buffer is empty.
- Returns:
- true if empty, otherwise false.
bool isFull | ( | ) |
This method returns whether the buffer is full.
- Returns:
- true if full, otherwise false.
int read | ( | char & | data ) |
This method reads a single byte from the buffer.
- Parameters:
-
data char where the read byte will be stored.
- Returns:
- 1 if byte is read or 0 if no bytes available.
int read | ( | char * | data, |
int | length | ||
) |
This method enables bulk reads from the buffer.
If more data is requested then available it simply returns all remaining data within the buffer.
- Parameters:
-
data the buffer where data read will be added to. length the amount of data in bytes to be read into the buffer.
- Returns:
- the total number of bytes that were read.
int remaining | ( | ) |
This method returns the amount of space left for writing.
- Returns:
- numbers of unused bytes in buffer.
int size | ( | ) |
This method returns the number of bytes available for reading.
- Returns:
- number of bytes currently in buffer.
int write | ( | const char * | data, |
int | length | ||
) |
This method enables bulk writes to the buffer.
If more data is requested to be written then space available the method writes as much data as possible and returns the actual amount written.
- Parameters:
-
data the byte array to be written. length the length of data to be written from the data paramter.
- Returns:
- the number of bytes written to the buffer, which is 0 if the buffer is full.
int write | ( | char | data ) |
This method writes a signle byte as a char to the buffer.
- Parameters:
-
data the byte to be written as a char.
- Returns:
- 1 if the byte was written or 0 if the buffer was full.
Generated on Wed Jul 13 2022 11:18:29 by
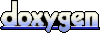