Library for LoRa communication using MultiTech MDOT.
Dependents: mDot_test_rx adc_sensor_lora mDotEVBM2X mDot_AT_firmware ... more
MTSCircularBuffer.h
00001 #ifndef MTSCIRCULARBUFFER_H 00002 #define MTSCIRCULARBUFFER_H 00003 00004 #include "Utils.h" 00005 00006 namespace mts 00007 { 00008 00009 /** This class provides a circular byte buffer meant for temporary storage 00010 * during IO transactions. It contains many of the common methods you 00011 * would expect from a circular buffer like read, write, and various 00012 * methods for checking the size or status. It should be noted that 00013 * this class does not include any special code for thread safety like 00014 * a lock. In most cases this is not problematic, but is something 00015 * to be aware of. 00016 */ 00017 class MTSCircularBuffer 00018 { 00019 public: 00020 /** Creates an MTSCircularBuffer object with the specified static size. 00021 * 00022 * @prarm bufferSize size of the buffer in bytes. 00023 */ 00024 MTSCircularBuffer(int bufferSize); 00025 00026 /** Destructs an MTSCircularBuffer object and frees all related resources. 00027 */ 00028 ~MTSCircularBuffer(); 00029 00030 /** This method enables bulk reads from the buffer. If more data is 00031 * requested then available it simply returns all remaining data within the 00032 * buffer. 00033 * 00034 * @param data the buffer where data read will be added to. 00035 * @param length the amount of data in bytes to be read into the buffer. 00036 * @returns the total number of bytes that were read. 00037 */ 00038 int read(char* data, int length); 00039 00040 /** This method reads a single byte from the buffer. 00041 * 00042 * @param data char where the read byte will be stored. 00043 * @returns 1 if byte is read or 0 if no bytes available. 00044 */ 00045 int read(char& data); 00046 00047 /** This method enables bulk writes to the buffer. If more data 00048 * is requested to be written then space available the method writes 00049 * as much data as possible and returns the actual amount written. 00050 * 00051 * @param data the byte array to be written. 00052 * @param length the length of data to be written from the data paramter. 00053 * @returns the number of bytes written to the buffer, which is 0 if 00054 * the buffer is full. 00055 */ 00056 int write(const char* data, int length); 00057 00058 /** This method writes a signle byte as a char to the buffer. 00059 * 00060 * @param data the byte to be written as a char. 00061 * @returns 1 if the byte was written or 0 if the buffer was full. 00062 */ 00063 int write(char data); 00064 00065 /** This method is used to setup a callback funtion when the buffer reaches 00066 * a certain threshold. The threshold condition is checked after every read 00067 * and write call is completed. The condition is made up of both a threshold 00068 * value and operator. An example that would trigger a callback is if the 00069 * threshold was 10, the operator GREATER, and there were 12 bytes added to an 00070 * empty buffer. 00071 * 00072 * @param tptr a pointer to the object to be called when the condition is met. 00073 * @param mptr a pointer to the function within the object to be called when 00074 * the condition is met. 00075 * @param threshold the value in bytes to be used as part of the condition. 00076 * @param op the operator to be used in conjunction with the threshold 00077 * as part of the condition. 00078 */ 00079 template<typename T> 00080 void attach(T *tptr, void( T::*mptr)(void), int threshold, RelationalOperator op) { 00081 _threshold = threshold; 00082 _op = op; 00083 notify.attach(tptr, mptr); 00084 } 00085 00086 /** This method is used to setup a callback funtion when the buffer reaches 00087 * a certain threshold. The threshold condition is checked after every read 00088 * and write call is completed. The condition is made up of both a threshold 00089 * value and operator. An example that would trigger a callback is if the 00090 * threshold was 10, the operator GREATER, and there were 12 bytes added to an 00091 * empty buffer. 00092 * 00093 * @param fptr a pointer to the static function to be called when the condition 00094 * is met. 00095 * @param threshold the value in bytes to be used as part of the condition. 00096 * @param op the operator to be used in conjunction with the threshold 00097 * as part of the condition. 00098 */ 00099 void attach(void(*fptr)(void), int threshold, RelationalOperator op) { 00100 _threshold = threshold; 00101 _op = op; 00102 notify.attach(fptr); 00103 } 00104 00105 /** This method returns the size of the storage space currently allocated for 00106 * the buffer. This value is equivalent to the one passed into the constructor. 00107 * This value is equal or greater than the size() of the buffer. 00108 * 00109 * @returns the allocated size of the buffer in bytes. 00110 */ 00111 int capacity(); 00112 00113 /** This method returns the amount of space left for writing. 00114 * 00115 * @returns numbers of unused bytes in buffer. 00116 */ 00117 int remaining(); 00118 00119 /** This method returns the number of bytes available for reading. 00120 * 00121 * @returns number of bytes currently in buffer. 00122 */ 00123 int size(); 00124 00125 /** This method returns whether the buffer is full. 00126 * 00127 * @returns true if full, otherwise false. 00128 */ 00129 bool isFull(); 00130 00131 /** This method returns whether the buffer is empty. 00132 * 00133 * @returns true if empty, otherwise false. 00134 */ 00135 bool isEmpty(); 00136 00137 /** This method clears the buffer. This is done through 00138 * setting the internal read and write indexes to the same 00139 * value and is therefore not an expensive operation. 00140 */ 00141 void clear(); 00142 00143 00144 private: 00145 int bufferSize; // total size of the buffer 00146 char* buffer; // internal byte buffer as a character buffer 00147 int readIndex; // read index for circular buffer 00148 int writeIndex; // write index for circular buffer 00149 int bytes; // available data 00150 FunctionPointer notify; // function pointer used for the internal callback notification 00151 int _threshold; // threshold for the notification 00152 RelationalOperator _op; // operator that determines the direction of the threshold 00153 void checkThreshold(); // private function that checks thresholds and processes notifications 00154 }; 00155 00156 } 00157 00158 #endif /* MTSCIRCULARBUFFER_H */
Generated on Wed Jul 13 2022 11:18:29 by
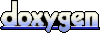