
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX30101.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "mbed.h" 00035 #include "MAX30101.h" 00036 00037 MAX30101 *MAX30101::instance = NULL; 00038 00039 //****************************************************************************** 00040 MAX30101::MAX30101(PinName sda, PinName scl, int slaveAddress): 00041 slaveAddress(slaveAddress) { 00042 i2c = new I2C(sda, scl); 00043 i2c_owner = true; 00044 i2c->frequency(400000); 00045 onInterruptCallback = NULL; 00046 onDataAvailableCallback = NULL; 00047 instance = this; 00048 } 00049 00050 //****************************************************************************** 00051 MAX30101::MAX30101(I2C *_i2c, int slaveAddress) : 00052 slaveAddress(slaveAddress) { 00053 i2c = _i2c; 00054 i2c_owner = false; 00055 i2c->frequency(400000); 00056 onInterruptCallback = NULL; 00057 onDataAvailableCallback = NULL; 00058 instance = this; 00059 } 00060 00061 //****************************************************************************** 00062 MAX30101::~MAX30101(void) { 00063 if (i2c_owner) { 00064 delete i2c; 00065 } 00066 } 00067 00068 //****************************************************************************** 00069 int MAX30101::int_handler(void) { 00070 uint16_t index, i; 00071 uint16_t rx_bytes, second_rx_bytes; 00072 char temp_int; 00073 char temp_frac; 00074 uint16_t num_active_led; 00075 uint32_t sample; 00076 int loop = 1; 00077 static uint8_t cntr_int = 0; 00078 00079 max30101_Interrupt_Status_1_t Interrupt_Status_1; 00080 max30101_Interrupt_Status_2_t Interrupt_Status_2; 00081 max30101_mode_configuration_t mode_configuration; 00082 max30101_multiLED_mode_ctrl_1_t multiLED_mode_ctrl_1; 00083 max30101_multiLED_mode_ctrl_2_t multiLED_mode_ctrl_2; 00084 max30101_spo2_configuration_t spo2_configuration; 00085 max30101_fifo_configuration_t fifo_configuration; 00086 00087 cntr_int++; 00088 00089 while (loop) { 00090 if (i2c_reg_read(REG_INT_STAT_1, &Interrupt_Status_1.all) != 0) { ///< Read Interrupt flag bits 00091 return -1; 00092 } 00093 00094 if (i2c_reg_read(REG_INT_STAT_2, &Interrupt_Status_2.all) != 0) { ///< Read Interrupt flag bits 00095 return -1; 00096 } 00097 00098 /* Read all the relevant register bits */ 00099 if (i2c_reg_read(REG_MODE_CFG, &mode_configuration.all) != 0) { 00100 return -1; 00101 } 00102 00103 00104 if (i2c_reg_read(REG_SLT2_SLT1, &multiLED_mode_ctrl_1.all) != 0) { 00105 return -1; 00106 } 00107 00108 00109 if (i2c_reg_read(REG_SLT4_SLT3, &multiLED_mode_ctrl_2.all) != 0) { 00110 return -1; 00111 } 00112 00113 00114 if (i2c_reg_read(REG_SPO2_CFG, &spo2_configuration.all) != 0) { 00115 return -1; 00116 } 00117 00118 00119 if (i2c_reg_read(REG_FIFO_CFG, &fifo_configuration.all) != 0) { 00120 return -1; 00121 } 00122 00123 00124 00125 if (Interrupt_Status_1.bit.a_full) { 00126 ///< Read the sample(s) 00127 char reg = REG_FIFO_DATA; 00128 00129 num_active_led = 0; 00130 00131 if (mode_configuration.bit.mode == 0x02) {///< Heart Rate mode, i.e. 1 led 00132 num_active_led = 1; 00133 } else if (mode_configuration.bit.mode == 0x03) { ///< SpO2 mode, i.e. 2 led 00134 num_active_led = 2; 00135 } else if (mode_configuration.bit.mode == 0x07) { ///< Multi-LED mode, i.e. 1-4 led 00136 if (multiLED_mode_ctrl_1.bit.slot1 != 0) { 00137 num_active_led++; 00138 } 00139 00140 if (multiLED_mode_ctrl_1.bit.slot2 != 0) { 00141 num_active_led++; 00142 } 00143 00144 if (multiLED_mode_ctrl_2.bit.slot3 != 0) { 00145 num_active_led++; 00146 } 00147 00148 if (multiLED_mode_ctrl_2.bit.slot4 != 0) { 00149 num_active_led++; 00150 } 00151 } 00152 ///< 3bytes/LED x Number of Active LED x FIFO level selected 00153 rx_bytes = 3 * num_active_led * (32-fifo_configuration.bit.fifo_a_full); 00154 00155 second_rx_bytes = rx_bytes; 00156 00157 /** 00158 * @brief: 00159 * The FIFO Size is determined by the Sample size. The number of bytes 00160 * in a Sample is dictated by number of LED's 00161 * 00162 * #LED Selected Bytes in "1" sample 00163 * 1 3 00164 * 2 6 00165 * 3 9 00166 * 4 12 00167 * 00168 * The I2C API function limits the number of bytes to read, to 256 (i.e. 00169 * char). Therefore, when set for Multiple LED's and the FIFO 00170 * size is set to 32. It would mean there is more than 256 bytes. 00171 * In that case two I2C reads have to be made. However It is important 00172 * to note that each "Sample" must be read completely and reading only 00173 * partial number of bytes from a sample will result in erroneous data. 00174 * 00175 * 00176 * For example: 00177 * Num of LED selected = 3 and FIFO size is set to 32 (i.e. 0 value in 00178 * register), then the number of bytes will be 00179 * 3bytes/Led * 3led's * 32 = 288 bytes in all. Since there are 00180 * 3 LED's each sample will contain (3 * 3) 9bytes. 00181 * Therefore Sample 1 = 9bytes, Sample 2 = 18,... Sample 28 = 252. 00182 * Therefore the first I2C read should be 252 bytes and the second 00183 * read should be 288-252 = 36. 00184 * 00185 * It turns out that this size issue comes up only when number of LED 00186 * selected is 3 or 4 and choosing 252bytes 00187 * for the first I2C read would work for both Number of LED selection. 00188 */ 00189 00190 if (rx_bytes <= CHUNK_SIZE) { 00191 I2CM_Read(slaveAddress, ®, 1, &max30101_rawData[0], 00192 (char)rx_bytes /*total_databytes_1*/); 00193 } else { 00194 I2CM_Read(slaveAddress, ®, 1, &max30101_rawData[0], CHUNK_SIZE); 00195 00196 second_rx_bytes = second_rx_bytes - CHUNK_SIZE; 00197 I2CM_Read(slaveAddress, ®, 1, &max30101_rawData[CHUNK_SIZE], 00198 (char)second_rx_bytes); 00199 } 00200 00201 index = 0; 00202 00203 for (i = 0; i < rx_bytes; i += 3) { 00204 sample = ((uint32_t)(max30101_rawData[i] & 0x03) << 16) | (max30101_rawData[i + 1] << 8) | max30101_rawData[i + 2]; 00205 00206 ///< Right shift the data based on the LED_PW setting 00207 sample = sample >> (3 - spo2_configuration.bit.led_pw); // 0=shift 3, 1=shift 2, 2=shift 1, 3=no shift 00208 00209 max30101_buffer[index++] = sample; 00210 } 00211 00212 onDataAvailableCallback(MAX30101_OXIMETER_DATA + num_active_led, max30101_buffer, index); 00213 } 00214 00215 00216 ///< This interrupt handles the temperature interrupt 00217 if (Interrupt_Status_2.bit.die_temp_rdy) { 00218 char reg; 00219 00220 reg = REG_TINT; 00221 if (I2CM_Read(slaveAddress, ®, 1, &temp_int, 1) != 0) { 00222 return -1; 00223 } 00224 00225 reg = REG_TFRAC; 00226 if (I2CM_Read(slaveAddress, ®, 1, &temp_frac, 1) != 0) { 00227 return -1; 00228 } 00229 00230 max30101_final_temp = (int8_t)temp_int + 0.0625f * temp_frac; 00231 00232 if (i2c_reg_write(REG_TEMP_EN, 0x00) != 0) { ///< Die Temperature Config, Temp disable... after one read... 00233 return -1; 00234 } 00235 } 00236 00237 if (i2c_reg_read(REG_INT_STAT_1, &Interrupt_Status_1.all) != 0) { ///< Read Interrupt flag bits 00238 00239 return -1; 00240 } 00241 if (Interrupt_Status_1.bit.a_full != 1) { 00242 loop = 0; 00243 } 00244 } 00245 00246 interruptPostCallback(); 00247 00248 00249 return 0; 00250 } 00251 00252 //****************************************************************************** 00253 int MAX30101::SpO2mode_init(uint8_t fifo_waterlevel_mark, uint8_t sample_avg, 00254 uint8_t sample_rate, uint8_t pulse_width, 00255 uint8_t red_led_current, uint8_t ir_led_current) { 00256 00257 char status; 00258 00259 max30101_mode_configuration_t mode_configuration; 00260 max30101_fifo_configuration_t fifo_configuration; 00261 max30101_spo2_configuration_t spo2_configuration; 00262 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00263 00264 mode_configuration.all = 0; 00265 mode_configuration.bit.reset = 1; 00266 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) // Reset the device 00267 { 00268 return -1; 00269 } 00270 00271 ///< Give it some settle time (100ms) 00272 wait(1.0 / 10.0); ///< Let things settle down a bit 00273 00274 fifo_configuration.all = 0; 00275 fifo_configuration.bit.smp_ave = sample_avg; ///< Sample averaging; 00276 fifo_configuration.bit.fifo_roll_over_en = 1; ///< FIFO Roll over enabled 00277 fifo_configuration.bit.fifo_a_full = fifo_waterlevel_mark; ///< Interrupt when certain level is filled 00278 00279 if (i2c_reg_write(REG_FIFO_CFG, fifo_configuration.all) != 0) { 00280 return -1; 00281 } 00282 00283 spo2_configuration.bit.spo2_adc_rge = 0x2; ///< ADC Range 8192 fullscale 00284 spo2_configuration.bit.spo2_sr = sample_rate; ///< 100 Samp/sec. 00285 spo2_configuration.bit.led_pw = pulse_width; ///< Pulse Width=411us and ADC Resolution=18 00286 if (i2c_reg_write(REG_SPO2_CFG, spo2_configuration.all) != 0) { 00287 return -1; 00288 } 00289 00290 if (i2c_reg_write(REG_LED1_PA, red_led_current) != 0) { 00291 return -1; 00292 } 00293 00294 if (i2c_reg_write(REG_LED2_PA, ir_led_current) != 0) { 00295 return -1; 00296 } 00297 00298 /************/ 00299 00300 if (i2c_reg_read(REG_INT_STAT_1, &status) != 0) ///< Clear INT1 by reading the status 00301 { 00302 return -1; 00303 } 00304 00305 if (i2c_reg_read(REG_INT_STAT_2, &status) != 0) ///< Clear INT2 by reading the status 00306 { 00307 return -1; 00308 } 00309 00310 if (i2c_reg_write(REG_FIFO_W_PTR, 0x00) != 0) ///< Clear FIFO ptr 00311 { 00312 return -1; 00313 } 00314 00315 if (i2c_reg_write(REG_FIFO_OVF_CNT, 0x00) != 0) ///< Clear FIFO ptr 00316 { 00317 return -1; 00318 } 00319 00320 if (i2c_reg_write(REG_FIFO_R_PTR, 0x00) != 0) ///< Clear FIFO ptr 00321 { 00322 return -1; 00323 } 00324 00325 Interrupt_Enable_1.all = 0; 00326 Interrupt_Enable_1.bit.a_full_en = 1; ///< Enable FIFO almost full interrupt 00327 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00328 return -1; 00329 } 00330 00331 mode_configuration.all = 0; 00332 mode_configuration.bit.mode = 0x03; ///< SpO2 mode 00333 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00334 return -1; 00335 } 00336 00337 return 0; 00338 } 00339 00340 //****************************************************************************** 00341 int MAX30101::SpO2mode_stop(void) { 00342 00343 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00344 max30101_mode_configuration_t mode_configuration; 00345 uint8_t led1_pa; 00346 uint8_t led2_pa; 00347 00348 Interrupt_Enable_1.all = 0; 00349 Interrupt_Enable_1.bit.a_full_en = 0; ///< Disable FIFO almost full interrupt 00350 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00351 return -1; 00352 } 00353 00354 mode_configuration.all = 0; 00355 mode_configuration.bit.mode = 0x00; ///< SpO2 mode off 00356 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00357 return -1; 00358 } 00359 00360 led1_pa = 0; ///< RED LED current, 0.0 00361 if (i2c_reg_write(REG_LED1_PA, led1_pa) != 0) { 00362 return -1; 00363 } 00364 00365 led2_pa = 0; ///< IR LED current, 0.0 00366 if (i2c_reg_write(REG_LED2_PA, led2_pa) != 0) { 00367 return -1; 00368 } 00369 00370 return 0; 00371 } 00372 00373 //****************************************************************************** 00374 int MAX30101::HRmode_init(uint8_t fifo_waterlevel_mark, uint8_t sample_avg, 00375 uint8_t sample_rate, uint8_t pulse_width, 00376 uint8_t red_led_current) { 00377 00378 /*uint8_t*/ char status; 00379 00380 max30101_mode_configuration_t mode_configuration; 00381 max30101_fifo_configuration_t fifo_configuration; 00382 max30101_spo2_configuration_t spo2_configuration; 00383 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00384 00385 mode_configuration.all = 0; 00386 mode_configuration.bit.reset = 1; 00387 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) ///< Reset the device, Mode = don't use... 00388 { 00389 return -1; 00390 } 00391 00392 ///< Give it some settle time (100ms) 00393 wait(1.0 / 10.0); ///< Let things settle down a bit 00394 00395 fifo_configuration.all = 0; 00396 fifo_configuration.bit.smp_ave = sample_avg; ///< Sample averaging; 00397 fifo_configuration.bit.fifo_roll_over_en = 1; ///< FIFO Roll over enabled 00398 fifo_configuration.bit.fifo_a_full = fifo_waterlevel_mark; ///< Interrupt when certain level is filled 00399 if (i2c_reg_write(REG_FIFO_CFG, fifo_configuration.all) != 0) { 00400 return -1; 00401 } 00402 00403 spo2_configuration.bit.spo2_adc_rge = 0x2; ///< ADC Range 8192 fullscale 00404 spo2_configuration.bit.spo2_sr = sample_rate; ///< 100 Samp/sec. 00405 spo2_configuration.bit.led_pw = pulse_width; ///< Pulse Width=411us and ADC Resolution=18 00406 if (i2c_reg_write(REG_SPO2_CFG, spo2_configuration.all) != 0) { 00407 return -1; 00408 } 00409 00410 if (i2c_reg_write(REG_LED1_PA, red_led_current) != 0) { 00411 return -1; 00412 } 00413 00414 /************/ 00415 00416 if (i2c_reg_read(REG_INT_STAT_1, &status) != 0) ///< Clear INT1 by reading the status 00417 { 00418 return -1; 00419 } 00420 00421 if (i2c_reg_read(REG_INT_STAT_2, &status) != 0) ///< Clear INT2 by reading the status 00422 { 00423 return -1; 00424 } 00425 00426 if (i2c_reg_write(REG_FIFO_W_PTR, 0x00) != 0) ///< Clear FIFO ptr 00427 { 00428 return -1; 00429 } 00430 00431 if (i2c_reg_write(REG_FIFO_OVF_CNT, 0x00) != 0) ///< Clear FIFO ptr 00432 { 00433 return -1; 00434 } 00435 00436 if (i2c_reg_write(REG_FIFO_R_PTR, 0x00) != 0) ///< Clear FIFO ptr 00437 { 00438 return -1; 00439 } 00440 00441 Interrupt_Enable_1.all = 0; 00442 Interrupt_Enable_1.bit.a_full_en = 1; 00443 00444 // Interrupt 00445 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00446 return -1; 00447 } 00448 00449 mode_configuration.all = 0; 00450 mode_configuration.bit.mode = 0x02; ///< HR mode 00451 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00452 return -1; 00453 } 00454 00455 return 0; 00456 } 00457 00458 //****************************************************************************** 00459 int MAX30101::HRmode_stop(void) { 00460 00461 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00462 max30101_mode_configuration_t mode_configuration; 00463 00464 Interrupt_Enable_1.all = 0; 00465 Interrupt_Enable_1.bit.a_full_en = 0; ///< Disable FIFO almost full interrupt 00466 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00467 return -1; 00468 } 00469 00470 mode_configuration.all = 0; 00471 mode_configuration.bit.mode = 0x00; ///< HR mode off 00472 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00473 return -1; 00474 } 00475 00476 if (i2c_reg_write(REG_LED1_PA, 0) != 0) { 00477 return -1; 00478 } 00479 00480 return 0; 00481 } 00482 00483 //****************************************************************************** 00484 int MAX30101::Multimode_init(uint8_t fifo_waterlevel_mark, uint8_t sample_avg, 00485 uint8_t sample_rate, uint8_t pulse_width, 00486 uint8_t red_led_current, uint8_t ir_led_current, 00487 uint8_t green_led_current, uint8_t slot_1, 00488 uint8_t slot_2, uint8_t slot_3, uint8_t slot_4) { 00489 00490 char status; 00491 max30101_mode_configuration_t mode_configuration; 00492 max30101_fifo_configuration_t fifo_configuration; 00493 max30101_spo2_configuration_t spo2_configuration; 00494 max30101_multiLED_mode_ctrl_1_t multiLED_mode_ctrl_1; 00495 max30101_multiLED_mode_ctrl_2_t multiLED_mode_ctrl_2; 00496 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00497 00498 mode_configuration.all = 0; 00499 mode_configuration.bit.reset = 1; 00500 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) ///< Reset the device, Mode = don't use... 00501 { 00502 return -1; 00503 } 00504 00505 /* Give it some settle time (100ms) */ ///< Let things settle down a bit 00506 wait(1.0 / 10.0); 00507 00508 fifo_configuration.all = 0; 00509 fifo_configuration.bit.smp_ave = sample_avg; ///< Sample averaging; 00510 fifo_configuration.bit.fifo_roll_over_en = 1; ///< FIFO Roll over enabled 00511 fifo_configuration.bit.fifo_a_full = 00512 fifo_waterlevel_mark; ///< Interrupt when certain level is filled 00513 if (i2c_reg_write(REG_FIFO_CFG, fifo_configuration.all) != 0) { 00514 return -1; 00515 } 00516 00517 spo2_configuration.bit.spo2_adc_rge = 0x2; ///< ADC Range 8192 fullscale 00518 spo2_configuration.bit.spo2_sr = sample_rate; ///< 100 Samp/sec. 00519 spo2_configuration.bit.led_pw = pulse_width; ///< Pulse Width=411us and ADC Resolution=18 00520 if (i2c_reg_write(REG_SPO2_CFG, spo2_configuration.all) != 0) { 00521 return -1; 00522 } 00523 00524 if (i2c_reg_write(REG_LED1_PA, red_led_current) != 0) { 00525 return -1; 00526 } 00527 00528 if (i2c_reg_write(REG_LED2_PA, ir_led_current) != 0) { 00529 return -1; 00530 } 00531 00532 if (i2c_reg_write(REG_LED3_PA, green_led_current) != 0) { 00533 return -1; 00534 } 00535 00536 ///< 0x01=Red(LED1), 0x02=IR(LED2), 0x03=Green(LED3) : Use LEDn_PA to adjust the intensity 00537 ///< 0x05=Red , 0x06=IR , 0x07=Green : Use PILOT_PA to adjust the intensity DO NOT USE THIS ROW... 00538 00539 multiLED_mode_ctrl_1.bit.slot1 = slot_1; 00540 multiLED_mode_ctrl_1.bit.slot2 = slot_2; 00541 if (i2c_reg_write(REG_SLT2_SLT1, multiLED_mode_ctrl_1.all)) { 00542 return -1; 00543 } 00544 00545 multiLED_mode_ctrl_2.all = 0; 00546 multiLED_mode_ctrl_2.bit.slot3 = slot_3; 00547 multiLED_mode_ctrl_2.bit.slot4 = slot_4; 00548 if (i2c_reg_write(REG_SLT4_SLT3, multiLED_mode_ctrl_2.all)) { 00549 return -1; 00550 } 00551 00552 /************/ 00553 00554 if (i2c_reg_read(REG_INT_STAT_1, &status) != 0) ///< Clear INT1 by reading the status 00555 { 00556 return -1; 00557 } 00558 00559 if (i2c_reg_read(REG_INT_STAT_2, &status) != 0) ///< Clear INT2 by reading the status 00560 { 00561 return -1; 00562 } 00563 00564 if (i2c_reg_write(REG_FIFO_W_PTR, 0x00) != 0) ///< Clear FIFO ptr 00565 { 00566 return -1; 00567 } 00568 00569 if (i2c_reg_write(REG_FIFO_OVF_CNT, 0x00) != 0) ///< Clear FIFO ptr 00570 { 00571 return -1; 00572 } 00573 00574 if (i2c_reg_write(REG_FIFO_R_PTR, 0x00) != 0) ///< Clear FIFO ptr 00575 { 00576 return -1; 00577 } 00578 00579 Interrupt_Enable_1.all = 0; 00580 Interrupt_Enable_1.bit.a_full_en = 1; ///< Enable FIFO almost full interrupt 00581 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00582 return -1; 00583 } 00584 00585 mode_configuration.all = 0; 00586 mode_configuration.bit.mode = 0x07; ///< Multi-LED mode 00587 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00588 return -1; 00589 } 00590 00591 return 0; 00592 } 00593 00594 //****************************************************************************** 00595 int MAX30101::Multimode_stop(void) { 00596 00597 max30101_Interrupt_Enable_1_t Interrupt_Enable_1; 00598 max30101_mode_configuration_t mode_configuration; 00599 00600 00601 Interrupt_Enable_1.all = 0; 00602 Interrupt_Enable_1.bit.a_full_en = 0; ///< Disable FIFO almost full interrupt 00603 if (i2c_reg_write(REG_INT_EN_1, Interrupt_Enable_1.all) != 0) { 00604 return -1; 00605 } 00606 00607 mode_configuration.all = 0; 00608 mode_configuration.bit.mode = 0x00; ///< Multi-LED mode off 00609 if (i2c_reg_write(REG_MODE_CFG, mode_configuration.all) != 0) { 00610 return -1; 00611 } 00612 00613 if (i2c_reg_write(REG_LED1_PA, 0) != 0) { 00614 return -1; 00615 } 00616 00617 if (i2c_reg_write(REG_LED2_PA, 0) != 0) { 00618 return -1; 00619 } 00620 00621 if (i2c_reg_write(REG_LED3_PA, 0) != 0) { 00622 return -1; 00623 } 00624 return 0; 00625 } 00626 00627 //****************************************************************************** 00628 int MAX30101::tempread(void) { 00629 00630 if (i2c_reg_write(REG_INT_EN_2, 0x02) != 0) {///< Interrupt Enable 2, Temperature Interrupt 00631 return -1; 00632 } 00633 00634 if (i2c_reg_write(REG_TEMP_EN, 0x01) != 0) {///< Die Temperature Config, Temp enable... 00635 00636 return -1; 00637 } 00638 return 0; 00639 } 00640 00641 //****************************************************************************** 00642 int MAX30101::i2c_reg_write(MAX30101_REG_map_t reg, char value) { 00643 00644 char cmdData[2] = {reg, value}; 00645 00646 if (I2CM_Write(slaveAddress, NULL, 0, cmdData, 2) != 0) { 00647 return -1; 00648 } 00649 00650 return 0; 00651 } 00652 00653 //****************************************************************************** 00654 int MAX30101::i2c_reg_read(MAX30101_REG_map_t reg, char *value) { 00655 if (I2CM_Read(slaveAddress, (char *)®, 1, value, 1) != 0 /*1*/) { 00656 return -1; 00657 } 00658 00659 return 0; 00660 } 00661 00662 //****************************************************************************** 00663 int MAX30101::I2CM_Read(int slaveAddress, char *writeData, char writeCount, 00664 char *readData, char readCount) { 00665 00666 if (writeData != NULL && writeCount != 0) { 00667 i2c->write(slaveAddress, writeData, writeCount, true); 00668 } 00669 if (readData != NULL && readCount != 0) { 00670 i2c->read(slaveAddress, readData, readCount); 00671 } 00672 return 0; 00673 } 00674 00675 //****************************************************************************** 00676 int MAX30101::I2CM_Write(int slaveAddress, char *writeData1, char writeCount1, 00677 char *writeData2, char writeCount2) { 00678 00679 if (writeData1 != NULL && writeCount1 != 0) { 00680 i2c->write(slaveAddress, writeData1, writeCount1); 00681 } 00682 if (writeData2 != NULL && writeCount2 != 0) { 00683 i2c->write(slaveAddress, writeData2, writeCount2); 00684 } 00685 return 0; 00686 } 00687 00688 //****************************************************************************** 00689 void MAX30101::onDataAvailable(DataCallbackFunction _onDataAvailable) { 00690 00691 onDataAvailableCallback = _onDataAvailable; 00692 } 00693 00694 //****************************************************************************** 00695 void MAX30101::dataAvailable(uint32_t id, uint32_t *buffer, uint32_t length) { 00696 00697 if (onDataAvailableCallback != NULL) { 00698 (*onDataAvailableCallback)(id, buffer, length); 00699 } 00700 } 00701 00702 //****************************************************************************** 00703 void MAX30101::onInterrupt(InterruptFunction _onInterrupt) { 00704 00705 onInterruptCallback = _onInterrupt; 00706 } 00707 00708 //****************************************************************************** 00709 void MAX30101::interruptPostCallback(void) { 00710 00711 if (onInterruptCallback != NULL) { 00712 00713 (*onInterruptCallback)(); 00714 } 00715 } 00716 00717 //****************************************************************************** 00718 void MAX30101::MidIntHandler(void) { 00719 00720 MAX30101::instance->int_handler(); 00721 }
Generated on Tue Jul 12 2022 17:59:19 by
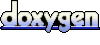