
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
BMP280.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 /** 00033 * Bosch BMP280 Digital Pressure Sensor 00034 * 00035 */ 00036 00037 #ifndef BMP280_H_ 00038 #define BMP280_H_ 00039 00040 #include "mbed.h" 00041 00042 /** 00043 * @brief Bosch BMP280 Digital Pressure Sensor 00044 */ 00045 class BMP280 { 00046 00047 public: 00048 00049 typedef enum { ///< BMP280 Register addresses 00050 00051 BMP280_READID = 0x58, 00052 BMP280_TEMP_XLSB = 0xFC, 00053 BMP280_TEMP_LSB = 0xFB, 00054 BMP280_TEMP_MSB = 0xFA, 00055 BMP280_PRESS_XLSB = 0xF9, 00056 BMP280_PRESS_LSB = 0xF8, 00057 BMP280_PRESS_MSB = 0xF7, 00058 BMP280_CONFIG = 0xF5, 00059 BMP280_CTRL_MEAS = 0xF4, 00060 BMP280_STATUS = 0xF3, 00061 BMP280_RESET = 0xE0, 00062 BMP280_ID = 0xD0, 00063 ///< calib25-calib00: 0xA1-0x88 00064 BMP280_CALIB25 = 0xA1, ///< Beginning address 00065 BMP280_CALIB00 = 0x88, ///< Ending address 00066 00067 BMP280_REGISTER_CHIPID = 0xD0, 00068 BMP280_REGISTER_VERSION = 0xD1, 00069 BMP280_REGISTER_SOFTRESET = 0xE0, 00070 BMP280_REGISTER_CAL26 = 0xE1, // R calibration stored in 0xE1-0xF0 00071 BMP280_REGISTER_CONTROL = 0xF4, 00072 BMP280_REGISTER_CONFIG = 0xF5, 00073 BMP280_REGISTER_PRESSUREDATA = 0xF7, 00074 BMP280_REGISTER_TEMPDATA = 0xFA, 00075 00076 } BMP280_REG_map_t ; 00077 00078 00079 /** 00080 * @brief BMP280 constructor. 00081 * @param sda mbed pin to use for SDA line of I2C interface. 00082 * @param scl mbed pin to use for SCL line of I2C interface. 00083 * @param slaveAddress Slave Address of the device. 00084 */ 00085 BMP280(PinName sda, PinName scl, int slaveAddress); 00086 00087 /** 00088 * @brief BMP280 constructor. 00089 * @param i2c I2C object to use. 00090 * @param slaveAddress Slave Address of the device. 00091 */ 00092 BMP280(I2C *i2c, int slaveAddress); 00093 00094 char loggingEnabled; 00095 char loggingSampleRate; 00096 00097 /** 00098 * @brief Write a device register 00099 */ 00100 int writeReg(char reg, char value); 00101 /** 00102 * @brief Read a device register 00103 */ 00104 int readReg(char reg, char *value); 00105 00106 ///< @brief CONFIG_REG (0xF5) 00107 typedef union bmp280_config_reg { 00108 char all; 00109 struct { 00110 char spi3w_en : 1; 00111 char reserved : 1; 00112 char filter : 3; 00113 char t_sb : 3; 00114 } bit; 00115 } bmp280_config_t; 00116 00117 ///< @brief CTRL_MEAS (0xF4) 00118 typedef union bmp280_ctrl_meas { 00119 char all; 00120 struct { 00121 char mode : 2; 00122 char osrs_p : 3; 00123 char osrs_t : 3; 00124 } bit; 00125 } bmp280_ctrl_meas_t; 00126 00127 ///< @brief STATUS (0xF3) 00128 typedef union bmp280_status { 00129 char all; 00130 struct { 00131 char im_update : 1; 00132 char reserved1 : 2; 00133 char measuring : 1; 00134 char reserved2 : 4; 00135 } bit; 00136 } bmp280_status_t; 00137 00138 00139 ///< @brief RESET (0xE0) 00140 char bmp280_reset; 00141 00142 ///< @brief ID (0xD0) 00143 char bmp280_id; 00144 00145 typedef enum { 00146 SKIPPED_P = 0, 00147 OVERSAMPLING_X1_P = 1, 00148 OVERSAMPLING_X2_P = 2, 00149 OVERSAMPLING_X4_P = 3, 00150 OVERSAMPLING_X8_P = 4, 00151 OVERSAMPLING_X16_P = 5 00152 } bmp280_osrs_P_t; 00153 00154 typedef enum { 00155 SKIPPED_T = 0, 00156 OVERSAMPLING_X1_T = 1, 00157 OVERSAMPLING_X2_T = 2, 00158 OVERSAMPLING_X4_T = 3, 00159 OVERSAMPLING_X8_T = 4, 00160 OVERSAMPLING_X16_T = 5 00161 } bmp280_osrs_T_t; 00162 00163 typedef enum { 00164 FILT_OFF = 1, 00165 FILT_2 = 2, 00166 FILT_3 = 4, 00167 FILT_4 = 8, 00168 FILT_5 = 16 00169 } bmp280_FILT_t; 00170 00171 typedef enum { 00172 SLEEP_MODE = 0, 00173 FORCED_MODE = 1, 00174 NORMAL_MODE = 3 00175 } bmp280_MODE_t; 00176 00177 typedef enum { 00178 T_0_5 = 0, 00179 T_62_5 = 1, 00180 T_125 = 2, 00181 T_250 = 3, 00182 T_500 = 4, 00183 T_1000 = 5, 00184 T_2000 = 6, 00185 T_4000 = 7 00186 } bmp280_TSB_t; 00187 00188 ///< @brief calib25... calib00 (0xA1...0x88) 00189 char bmp280_Calib[26]; 00190 00191 uint16_t dig_T1; ///< Unique temp coeffs. read from the chip 00192 int16_t dig_T2; 00193 int16_t dig_T3; 00194 00195 uint16_t dig_P1; ///< Unique Press. coeffs. read from the chip 00196 int16_t dig_P2; 00197 int16_t dig_P3; 00198 int16_t dig_P4; 00199 int16_t dig_P5; 00200 int16_t dig_P6; 00201 int16_t dig_P7; 00202 int16_t dig_P8; 00203 int16_t dig_P9; 00204 00205 int32_t t_fine; ///< This is calculated int the temperature to be used by the 00206 ///< pressure 00207 00208 int32_t bmp280_rawPress; 00209 int32_t bmp280_rawTemp; 00210 00211 float Temp_degC; 00212 float Press_Pa; 00213 00214 /** 00215 * @brief BMP280 constructor. 00216 * @param sda mbed pin to use for SDA line of I2C interface. 00217 * @param scl mbed pin to use for SCL line of I2C interface. 00218 */ 00219 BMP280(PinName sda, PinName scl); 00220 00221 /** 00222 * @brief BMP280 constructor. 00223 * @param i2c I2C object to use. 00224 */ 00225 BMP280(I2C *i2c); 00226 00227 /** 00228 * @brief BMP280 destructor. 00229 */ 00230 ~BMP280(void); 00231 00232 // Function Prototypes 00233 00234 /** 00235 * @brief This initializes the BMP280 00236 * @brief The BMP280 has 2 modes. FORCED mode and NORMAL mode. FORCED Mode gives more 00237 * @brief control to the processor as the processor sends out the Mode to initiate a conversion 00238 * @brief and a data is sent out then. NORMAL mode is initialized once and it just runs and sends 00239 * @brief out data at a programmed timed interval. (In this example the main() will set this to Normal 00240 * @brief function) 00241 * @param Osrs_p- Pressure oversampling 00242 * @param Osrs_t- Temperature oversampling 00243 * @param Filter- Filter Settings 00244 * @param Mode- Power Modes 00245 * @param T_sb- Standby time (used with Normal mode) 00246 * @param dig_T1, dig_T2, dig_T3- Coeffs used for temp conversion - GLOBAL variables (output) 00247 * @param dig_P1, .... , dig_P9- Coeffs used for press conversion - GLOBAL variables (output) 00248 * @returns 0-if no error. A non-zero value indicates an error. 00249 */ 00250 int init(bmp280_osrs_P_t Osrs_p, bmp280_osrs_T_t Osrs_t, bmp280_FILT_t Filter, 00251 bmp280_MODE_t Mode, bmp280_TSB_t T_sb); 00252 00253 /** 00254 * @brief The BMP280 has 2 modes. FORCED mode and NORMAL mode. FORCED Mode 00255 * gives more 00256 * @brief control to the processor as the processor sends out the Mode to 00257 * initiate a conversion 00258 * @brief and a data is sent out then. NORMAL mode is initialized once and it 00259 * just runs and sends 00260 * @brief out data at a programmed timed interval. (In this example the 00261 * main() will set this to Normal 00262 * @brief function) 00263 * @param *Temp_degC - Pointer to temperature (result in deg C) 00264 * @param *Press_Pa - Pointer to pressure (resul in Pascal) 00265 * @returns 0-if no error. A non-zero value indicates an error. 00266 */ 00267 int ReadCompData(float *Temp_degC, float *Press_Pa); 00268 00269 /** 00270 * @brief This function allows writing to a register. 00271 * @param reg- Address of the register to write to 00272 * @param value- Data written to the register 00273 * @returns 0-if no error. A non-zero value indicates an error. 00274 */ 00275 int reg_write(BMP280_REG_map_t reg, char value); 00276 00277 /** 00278 * @brief This function allows writing to a register. 00279 * @params reg- Address of the register to read from (input) 00280 * @params *value- Pointer to the value read from the register (output) 00281 * @returns 0-if no error. A non-zero value indicates an error. 00282 */ 00283 int reg_read(BMP280_REG_map_t reg, char *value, char number); 00284 00285 /** 00286 * @brief Performs a soft reset on the BMP280 00287 * @param none 00288 * @returns none 00289 */ 00290 void Reset(void); 00291 00292 /** 00293 * @brief Detects if the BMP280 is present 00294 * @param none 00295 * @returns 1 for found, 0 for not found, -1 for comm error 00296 */ 00297 int Detect(void); 00298 00299 /** 00300 * @brief Performs calculations on the raw temperature data to convert to 00301 * @brief temperature in deg C, based on Bosch's algorithm 00302 * @param Raw Temp ADC value, Global dig_T1, dig_T2, dig_T3 00303 * @returns The Temperature in deg C 00304 */ 00305 float compensate_T_float(int32_t adc_T); // returned value Deg C. 00306 00307 /** 00308 * @brief Performs calculations on the raw pressure data to convert to 00309 * @brief pressure in Pascal, based on Bosch's algorithm 00310 * @param adc_P Raw Press ADC value, Global dig_P1, dig_P2,..., dig_P9 00311 * @returns The Pressure in Pascals 00312 */ 00313 float compensate_P_float(int32_t adc_P); // returned value Pascal. 00314 00315 /** 00316 * @brief Puts the BMP280 in low power Sleep mode 00317 * @param none 00318 * @returns 0 if no errors, -1 if error. 00319 */ 00320 int Sleep(void); 00321 00322 /** 00323 * @brief This reads the raw BMP280 data 00324 * @param *bmp280_rawData- array of raw output data 00325 * @returns 0-if no error. A non-zero value indicates an error. 00326 * 00327 */ 00328 int ReadCompDataRaw(char *bmp280_rawData); 00329 /** 00330 * @brief This reads the raw BMP280 data uses the Bosch algorithm to get the 00331 * data 00332 * @brief in float, then the float gets converted to an String 00333 * @param *bmp280_rawData- array of raw output data 00334 * @returns 0-if no error. A non-zero value indicates an error. 00335 * 00336 */ 00337 int ReadCompDataRaw2(char *bmp280_rawData); 00338 /** 00339 * @brief This converts the raw BMP280 data to couble based on Bosch's 00340 * algorithm 00341 * @param *bmp280_rawData- array of raw input data 00342 * @param *Temp_degC- pointer to output, Temp value in deg C 00343 * @param *Press_Pa- pointer to output, Press value in Pascals 00344 * @returns 0-if no error. A non-zero value indicates an error. 00345 * 00346 */ 00347 void ToFloat(char *bmp280_rawData, float *Temp_degC, float *Press_Pa); 00348 /** 00349 * @brief converts to Farenhite from Centigrade 00350 * @param temperature in Centigrade 00351 * @returns temperature value in Farenhite 00352 */ 00353 float ToFahrenheit(float temperature); 00354 00355 /** 00356 * @brief Reads a unique ID from the register 00357 * @param none 00358 * @returns The correct id value which is 0x58 00359 */ 00360 int ReadId(void); 00361 00362 private: 00363 /** 00364 * @brief I2C pointer 00365 */ 00366 I2C *i2c; 00367 /** 00368 * @brief Is this object the owner of the I2C object 00369 */ 00370 bool isOwner; 00371 /** 00372 * @brief Device slave address 00373 */ 00374 int slaveAddress; 00375 }; 00376 00377 #endif // BMP280_H_
Generated on Tue Jul 12 2022 17:59:19 by
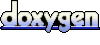