Library for the MAX7219 LED display driver
Dependents: MAXREFDES99_demo MAXREFDES99_RTC_Display nucleo_spi_max7219_led8x8 max7219 ... more
Max7219 Class Reference
Serially Interfaced, 8-Digit, LED Display Driver. More...
#include <max7219.h>
Public Types | |
enum | max7219_register_e |
Digit and Control Registers. More... | |
enum | max7219_intensity_e |
Intensity values. More... | |
enum | max7219_scan_limit_e |
Scan limit for mutiplexing digits. More... | |
Public Member Functions | |
Max7219 (SPI *spi_bus, PinName cs) | |
Constructor for Max7219 Class. | |
Max7219 (PinName mosi, PinName miso, PinName sclk, PinName cs) | |
Constructor for Max7219 Class. | |
~Max7219 () | |
Default destructor for Max7219 Class. | |
int32_t | set_num_devices (uint8_t num_devices) |
Sets the number of MAX7219 devices being used. | |
void | set_display_test (void) |
Tests all devices being used. | |
void | clear_display_test (void) |
Stops test. | |
int32_t | init_device (max7219_configuration_t config) |
Initializes specific device in display with given config data. | |
void | init_display (max7219_configuration_t config) |
Initializes all devices with given config data. | |
int32_t | enable_device (uint8_t device_number) |
Enables specific device in display. | |
void | enable_display (void) |
Enables all device in display. | |
int32_t | disable_device (uint8_t device_number) |
Disables specific device in display. | |
void | disable_display (void) |
Disables all devices in display. | |
int32_t | write_digit (uint8_t device_number, uint8_t digit, uint8_t data) |
Writes digit of given device with given data, user must enter correct data for decode_mode chosen. | |
int32_t | clear_digit (uint8_t device_number, uint8_t digit) |
Clears digit of given device. | |
int32_t | device_all_on (uint8_t device_number) |
Turns on all segments/digits of given device. | |
int32_t | device_all_off (uint8_t device_number) |
Turns off all segments/digits of given device. | |
void | display_all_on (void) |
Turns on all segments/digits of display. | |
void | display_all_off (void) |
Turns off all segments/digits of display. |
Detailed Description
Serially Interfaced, 8-Digit, LED Display Driver.
The MAX7219/MAX7221 are compact, serial input/output common-cathode display drivers that interface microprocessors (µPs) to 7-segment numeric LED displays of up to 8 digits, bar-graph displays, or 64 individual LEDs. Included on-chip are a BCD code-B decoder, multiplex scan circuitry, segment and digit drivers, and an 8x8 static RAM that stores each digit. Only one external resistor is required to set the segment current for all LEDs. The MAX7221 is compatible with SPI™, QSPI™, and MICROWIRE™, and has slew-rate-limited segment drivers to reduce EMI.
#include "mbed.h" #include "max7219.h" Max7219 max7219(SPI0_MOSI, SPI0_MISO, SPI0_SCK, SPI0_SS); int main() { max7219_configuration_t cfg = { .device_number = 1, .decode_mode = 0, .intensity = Max7219::MAX7219_INTENSITY_8, .scan_limit = Max7219::MAX7219_SCAN_8 }; max7219.init_device(cfg); max7219.enable_device(1); max7219.set_display_test(); wait(1); max7219.clear_display_test(); while (1) { max7219.write_digit(1, Max7219::MAX7219_DIGIT_0, ... } }
Definition at line 102 of file max7219.h.
Member Enumeration Documentation
enum max7219_intensity_e |
enum max7219_register_e |
enum max7219_scan_limit_e |
Constructor & Destructor Documentation
Max7219 | ( | SPI * | spi_bus, |
PinName | cs | ||
) |
Constructor for Max7219 Class.
Allows user to pass pointer to existing SPI bus
On Entry:
- Parameters:
-
[in] spi_bus - pointer to existing SPI object [in] cs - pin to use for cs
On Exit:
- Returns:
- None
Definition at line 39 of file max7219.cpp.
Max7219 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs | ||
) |
Constructor for Max7219 Class.
Allows user to specify SPI peripheral to use
On Entry:
- Parameters:
-
[in] mosi - pin to use for mosi [in] miso - pin to use for miso [in] sclk - pin to use for sclk [in] cs - pin to use for cs
On Exit:
- Returns:
- None
Definition at line 49 of file max7219.cpp.
~Max7219 | ( | ) |
Default destructor for Max7219 Class.
Destroys SPI object if owner
On Entry:
On Exit:
- Returns:
- None
Definition at line 61 of file max7219.cpp.
Member Function Documentation
int32_t clear_digit | ( | uint8_t | device_number, |
uint8_t | digit | ||
) |
Clears digit of given device.
On Entry:
- Parameters:
-
[in] device_number - device to write too [in] digit - digit to clear
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Returns -3 if digit > 8
Returns -4 if digit < 1
Definition at line 400 of file max7219.cpp.
void clear_display_test | ( | void | ) |
Stops test.
Clear bit0 of DISPLAY_TEST regiser in all devices
On Entry:
On Exit:
- Returns:
- None
Definition at line 103 of file max7219.cpp.
int32_t device_all_off | ( | uint8_t | device_number ) |
Turns off all segments/digits of given device.
On Entry:
- Parameters:
-
[in] device_number - device to write too
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Definition at line 482 of file max7219.cpp.
int32_t device_all_on | ( | uint8_t | device_number ) |
Turns on all segments/digits of given device.
On Entry:
- Parameters:
-
[in] device_number - device to write too
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Definition at line 450 of file max7219.cpp.
int32_t disable_device | ( | uint8_t | device_number ) |
Disables specific device in display.
On Entry:
- Parameters:
-
[in] device_number - device to disable
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Definition at line 294 of file max7219.cpp.
void disable_display | ( | void | ) |
Disables all devices in display.
On Entry:
On Exit:
- Returns:
- None
Definition at line 334 of file max7219.cpp.
void display_all_off | ( | void | ) |
Turns off all segments/digits of display.
On Entry:
On Exit:
- Returns:
- None
Definition at line 528 of file max7219.cpp.
void display_all_on | ( | void | ) |
Turns on all segments/digits of display.
On Entry:
On Exit:
- Returns:
- None
Definition at line 513 of file max7219.cpp.
int32_t enable_device | ( | uint8_t | device_number ) |
Enables specific device in display.
On Entry:
- Parameters:
-
[in] device_number - device to enable
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Definition at line 239 of file max7219.cpp.
void enable_display | ( | void | ) |
Enables all device in display.
On Entry:
On Exit:
- Returns:
- None
Definition at line 279 of file max7219.cpp.
int32_t init_device | ( | max7219_configuration_t | config ) |
Initializes specific device in display with given config data.
On Entry:
- Parameters:
-
[in] config - Structure containing configuration data of device
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Definition at line 118 of file max7219.cpp.
void init_display | ( | max7219_configuration_t | config ) |
Initializes all devices with given config data.
All devices are configured with given data
On Entry:
- Parameters:
-
[in] config - Structure containing configuration data On Exit:
- Returns:
- None
Definition at line 199 of file max7219.cpp.
void set_display_test | ( | void | ) |
Tests all devices being used.
Sets bit0 of DISPLAY_TEST regiser in all devices
On Entry:
On Exit:
- Returns:
- None
Definition at line 88 of file max7219.cpp.
int32_t set_num_devices | ( | uint8_t | num_devices ) |
Sets the number of MAX7219 devices being used.
Defaults to one
On Entry:
- Parameters:
-
[in] num_devices - number of MAX7219 devices being used, max of 255
On Exit:
- Returns:
- Returns number of devices
Definition at line 73 of file max7219.cpp.
int32_t write_digit | ( | uint8_t | device_number, |
uint8_t | digit, | ||
uint8_t | data | ||
) |
Writes digit of given device with given data, user must enter correct data for decode_mode chosen.
On Entry:
- Parameters:
-
[in] device_number - device to write too [in] digit - digit to write [in] data - data to write
On Exit:
- Returns:
- Returns 0 on success
Returns -1 if device number is > _num_devices
Returns -2 if device number is 0
Returns -3 if digit > 8
Returns -4 if digit < 1
Definition at line 350 of file max7219.cpp.
Generated on Tue Jul 12 2022 15:07:00 by
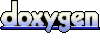