Library for the MAX7219 LED display driver
Dependents: MAXREFDES99_demo MAXREFDES99_RTC_Display nucleo_spi_max7219_led8x8 max7219 ... more
max7219.h
00001 /******************************************************************//** 00002 * @file max7219.h 00003 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a 00006 * copy of this software and associated documentation files (the "Software"), 00007 * to deal in the Software without restriction, including without limitation 00008 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00009 * and/or sell copies of the Software, and to permit persons to whom the 00010 * Software is furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included 00013 * in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00016 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00018 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00019 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00020 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00021 * OTHER DEALINGS IN THE SOFTWARE. 00022 * 00023 * Except as contained in this notice, the name of Maxim Integrated 00024 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00025 * Products, Inc. Branding Policy. 00026 * 00027 * The mere transfer of this software does not imply any licenses 00028 * of trade secrets, proprietary technology, copyrights, patents, 00029 * trademarks, maskwork rights, or any other form of intellectual 00030 * property whatsoever. Maxim Integrated Products, Inc. retains all 00031 * ownership rights. 00032 **********************************************************************/ 00033 00034 00035 #ifndef MAX7219_H 00036 #define MAX7219_H 00037 00038 #include "mbed.h" 00039 00040 00041 /** 00042 * @brief Structure for device configuration 00043 * @code 00044 * #include "max7219.h" 00045 * 00046 * max7219_configuration_t cfg = { 00047 * .device_number = 1, 00048 * .decode_mode = 0, 00049 * .intensity = Max7219::MAX7219_INTENSITY_8, 00050 * .scan_limit = Max7219::MAX7219_SCAN_8 00051 * }; 00052 * @endcode 00053 */ 00054 typedef struct 00055 { 00056 uint8_t device_number; 00057 uint8_t decode_mode; 00058 uint8_t intensity; 00059 uint8_t scan_limit; 00060 }max7219_configuration_t; 00061 00062 00063 /** 00064 * @brief Serially Interfaced, 8-Digit, LED Display Driver 00065 * 00066 * @details The MAX7219/MAX7221 are compact, serial input/output common-cathode 00067 * display drivers that interface microprocessors (µPs) to 7-segment numeric 00068 * LED displays of up to 8 digits, bar-graph displays, or 64 individual LEDs. 00069 * Included on-chip are a BCD code-B decoder, multiplex scan circuitry, segment 00070 * and digit drivers, and an 8x8 static RAM that stores each digit. Only one 00071 * external resistor is required to set the segment current for all LEDs. 00072 * The MAX7221 is compatible with SPI™, QSPI™, and MICROWIRE™, and has 00073 * slew-rate-limited segment drivers to reduce EMI. 00074 * 00075 * @code 00076 * #include "mbed.h" 00077 * #include "max7219.h" 00078 * 00079 * Max7219 max7219(SPI0_MOSI, SPI0_MISO, SPI0_SCK, SPI0_SS); 00080 * 00081 * int main() 00082 * { 00083 * max7219_configuration_t cfg = { 00084 * .device_number = 1, 00085 * .decode_mode = 0, 00086 * .intensity = Max7219::MAX7219_INTENSITY_8, 00087 * .scan_limit = Max7219::MAX7219_SCAN_8 00088 * }; 00089 * 00090 * max7219.init_device(cfg); 00091 * max7219.enable_device(1); 00092 * max7219.set_display_test(); 00093 * wait(1); 00094 * max7219.clear_display_test(); 00095 * 00096 * while (1) { 00097 * max7219.write_digit(1, Max7219::MAX7219_DIGIT_0, ... 00098 * } 00099 * } 00100 * @endcode 00101 */ 00102 class Max7219 00103 { 00104 public: 00105 00106 /** 00107 * @brief Digit and Control Registers 00108 * @details The 14 addressable digit and control registers. 00109 */ 00110 typedef enum 00111 { 00112 MAX7219_NO_OP = 0, 00113 MAX7219_DIGIT_0, 00114 MAX7219_DIGIT_1, 00115 MAX7219_DIGIT_2, 00116 MAX7219_DIGIT_3, 00117 MAX7219_DIGIT_4, 00118 MAX7219_DIGIT_5, 00119 MAX7219_DIGIT_6, 00120 MAX7219_DIGIT_7, 00121 MAX7219_DECODE_MODE, 00122 MAX7219_INTENSITY, 00123 MAX7219_SCAN_LIMIT, 00124 MAX7219_SHUTDOWN, 00125 MAX7219_DISPLAY_TEST = 15 00126 }max7219_register_e; 00127 00128 00129 /** 00130 * @brief Intensity values 00131 * @details Digital control of display brightness is provided by an 00132 * internal pulse-width modulator, which is controlled by 00133 * the lower nibble of the intensity register. 00134 */ 00135 typedef enum 00136 { 00137 MAX7219_INTENSITY_0 = 0, 00138 MAX7219_INTENSITY_1, 00139 MAX7219_INTENSITY_2, 00140 MAX7219_INTENSITY_3, 00141 MAX7219_INTENSITY_4, 00142 MAX7219_INTENSITY_5, 00143 MAX7219_INTENSITY_6, 00144 MAX7219_INTENSITY_7, 00145 MAX7219_INTENSITY_8, 00146 MAX7219_INTENSITY_9, 00147 MAX7219_INTENSITY_A, 00148 MAX7219_INTENSITY_B, 00149 MAX7219_INTENSITY_C, 00150 MAX7219_INTENSITY_D, 00151 MAX7219_INTENSITY_E, 00152 MAX7219_INTENSITY_F 00153 }max7219_intensity_e; 00154 00155 00156 /** 00157 * @brief Scan limit for mutiplexing digits 00158 * @details The scan-limit register sets how many digits are 00159 * displayed, from 1 to 8. They are displayed in a multiplexed 00160 * manner with a typical display scan rate of 800Hz with 8 00161 * digits displayed. 00162 */ 00163 typedef enum 00164 { 00165 MAX7219_SCAN_1 = 0, 00166 MAX7219_SCAN_2, 00167 MAX7219_SCAN_3, 00168 MAX7219_SCAN_4, 00169 MAX7219_SCAN_5, 00170 MAX7219_SCAN_6, 00171 MAX7219_SCAN_7, 00172 MAX7219_SCAN_8 00173 }max7219_scan_limit_e; 00174 00175 00176 /**********************************************************//** 00177 * @brief Constructor for Max7219 Class. 00178 * 00179 * @details Allows user to pass pointer to existing SPI bus 00180 * 00181 * On Entry: 00182 * @param[in] spi_bus - pointer to existing SPI object 00183 * @param[in] cs - pin to use for cs 00184 * 00185 * On Exit: 00186 * 00187 * @return None 00188 **************************************************************/ 00189 Max7219(SPI *spi_bus, PinName cs); 00190 00191 00192 /**********************************************************//** 00193 * @brief Constructor for Max7219 Class. 00194 * 00195 * @details Allows user to specify SPI peripheral to use 00196 * 00197 * On Entry: 00198 * @param[in] mosi - pin to use for mosi 00199 * @param[in] miso - pin to use for miso 00200 * @param[in] sclk - pin to use for sclk 00201 * @param[in] cs - pin to use for cs 00202 * 00203 * On Exit: 00204 * 00205 * @return None 00206 **************************************************************/ 00207 Max7219(PinName mosi, PinName miso, PinName sclk, PinName cs); 00208 00209 00210 /**********************************************************//** 00211 * @brief Default destructor for Max7219 Class. 00212 * 00213 * @details Destroys SPI object if owner 00214 * 00215 * On Entry: 00216 * 00217 * On Exit: 00218 * 00219 * @return None 00220 **************************************************************/ 00221 ~Max7219(); 00222 00223 00224 /**********************************************************//** 00225 * @brief Sets the number of MAX7219 devices being used. 00226 * Defaults to one 00227 * 00228 * @details 00229 * 00230 * On Entry: 00231 * @param[in] num_devices - number of MAX7219 devices being 00232 * used, max of 255 00233 * 00234 * On Exit: 00235 * 00236 * @return Returns number of devices 00237 **************************************************************/ 00238 int32_t set_num_devices(uint8_t num_devices); 00239 00240 00241 /**********************************************************//** 00242 * @brief Tests all devices being used 00243 * 00244 * @details Sets bit0 of DISPLAY_TEST regiser in all devices 00245 * 00246 * On Entry: 00247 * 00248 * On Exit: 00249 * 00250 * @return None 00251 **************************************************************/ 00252 void set_display_test(void); 00253 00254 00255 /**********************************************************//** 00256 * @brief Stops test 00257 * 00258 * @details Clear bit0 of DISPLAY_TEST regiser in all devices 00259 * 00260 * On Entry: 00261 * 00262 * On Exit: 00263 * 00264 * @return None 00265 **************************************************************/ 00266 void clear_display_test(void); 00267 00268 00269 /**********************************************************//** 00270 * @brief Initializes specific device in display with given 00271 * config data 00272 * 00273 * @details 00274 * 00275 * On Entry: 00276 * @param[in] config - Structure containing configuration 00277 * data of device 00278 * 00279 * On Exit: 00280 * 00281 * @return Returns 0 on success\n 00282 * Returns -1 if device number is > _num_devices\n 00283 * Returns -2 if device number is 0\n 00284 **************************************************************/ 00285 int32_t init_device(max7219_configuration_t config); 00286 00287 00288 /**********************************************************//** 00289 * @brief Initializes all devices with given config data 00290 * 00291 * @details All devices are configured with given data 00292 * 00293 * On Entry: 00294 * @param[in] config - Structure containing configuration 00295 * data 00296 * On Exit: 00297 * 00298 * @return None 00299 **************************************************************/ 00300 void init_display(max7219_configuration_t config); 00301 00302 00303 /**********************************************************//** 00304 * @brief Enables specific device in display 00305 * 00306 * @details 00307 * 00308 * On Entry: 00309 * @param[in] device_number - device to enable 00310 * 00311 * On Exit: 00312 * 00313 * @return Returns 0 on success\n 00314 * Returns -1 if device number is > _num_devices\n 00315 * Returns -2 if device number is 0\n 00316 **************************************************************/ 00317 int32_t enable_device(uint8_t device_number); 00318 00319 00320 /**********************************************************//** 00321 * @brief Enables all device in display 00322 * 00323 * @details 00324 * 00325 * On Entry: 00326 * 00327 * On Exit: 00328 * 00329 * @return None 00330 **************************************************************/ 00331 void enable_display(void); 00332 00333 00334 /**********************************************************//** 00335 * @brief Disables specific device in display 00336 * 00337 * @details 00338 * 00339 * On Entry: 00340 * @param[in] device_number - device to disable 00341 * 00342 * On Exit: 00343 * @return Returns 0 on success\n 00344 * Returns -1 if device number is > _num_devices\n 00345 * Returns -2 if device number is 0\n 00346 **************************************************************/ 00347 int32_t disable_device(uint8_t device_number); 00348 00349 00350 /**********************************************************//** 00351 * @brief Disables all devices in display 00352 * 00353 * @details 00354 * 00355 * On Entry: 00356 * 00357 * On Exit: 00358 * 00359 * @return None 00360 **************************************************************/ 00361 void disable_display(void); 00362 00363 00364 /**********************************************************//** 00365 * @brief Writes digit of given device with given data, user 00366 * must enter correct data for decode_mode chosen 00367 * 00368 * @details 00369 * 00370 * On Entry: 00371 * @param[in] device_number - device to write too 00372 * @param[in] digit - digit to write 00373 * @param[in] data - data to write 00374 * 00375 * On Exit: 00376 * 00377 * @return Returns 0 on success\n 00378 * Returns -1 if device number is > _num_devices\n 00379 * Returns -2 if device number is 0\n 00380 * Returns -3 if digit > 8\n 00381 * Returns -4 if digit < 1\n 00382 **************************************************************/ 00383 int32_t write_digit(uint8_t device_number, uint8_t digit, uint8_t data); 00384 00385 00386 /**********************************************************//** 00387 * @brief Clears digit of given device 00388 * 00389 * @details 00390 * 00391 * On Entry: 00392 * @param[in] device_number - device to write too 00393 * @param[in] digit - digit to clear 00394 * 00395 * On Exit: 00396 * 00397 * @return Returns 0 on success\n 00398 * Returns -1 if device number is > _num_devices\n 00399 * Returns -2 if device number is 0\n 00400 * Returns -3 if digit > 8\n 00401 * Returns -4 if digit < 1\n 00402 **************************************************************/ 00403 int32_t clear_digit(uint8_t device_number, uint8_t digit); 00404 00405 00406 /**********************************************************//** 00407 * @brief Turns on all segments/digits of given device 00408 * 00409 * @details 00410 * 00411 * On Entry: 00412 * @param[in] device_number - device to write too 00413 * 00414 * On Exit: 00415 * 00416 * @return Returns 0 on success\n 00417 * Returns -1 if device number is > _num_devices\n 00418 * Returns -2 if device number is 0\n 00419 **************************************************************/ 00420 int32_t device_all_on(uint8_t device_number); 00421 00422 00423 /**********************************************************//** 00424 * @brief Turns off all segments/digits of given device 00425 * 00426 * @details 00427 * 00428 * On Entry: 00429 * @param[in] device_number - device to write too 00430 * 00431 * On Exit: 00432 * 00433 * @return Returns 0 on success\n 00434 * Returns -1 if device number is > _num_devices\n 00435 * Returns -2 if device number is 0\n 00436 **************************************************************/ 00437 int32_t device_all_off(uint8_t device_number); 00438 00439 00440 /**********************************************************//** 00441 * @brief Turns on all segments/digits of display 00442 * 00443 * @details 00444 * 00445 * On Entry: 00446 * 00447 * On Exit: 00448 * 00449 * @return None 00450 **************************************************************/ 00451 void display_all_on(void); 00452 00453 00454 /**********************************************************//** 00455 * @brief Turns off all segments/digits of display 00456 * 00457 * @details 00458 * 00459 * On Entry: 00460 * 00461 * On Exit: 00462 * 00463 * @return None 00464 **************************************************************/ 00465 void display_all_off(void); 00466 00467 00468 private: 00469 00470 SPI *_p_spi; 00471 DigitalOut *_p_cs; 00472 bool _spi_owner; 00473 00474 uint8_t _num_devices; 00475 00476 }; 00477 #endif /* MAX7219_H*/
Generated on Tue Jul 12 2022 15:07:00 by
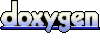