
Animation demo with MIP8F_SPI_Ver60
Dependencies: mbed MIP8F_SPI_Ver60 MIP8f_FRDM_LineBuffer_sample MIP8f_FRDM_TransferMode_sample
main.cpp
00001 /** 00002 * @file main.cpp 00003 * @brief Ver6.0 Sample source code for MIP8 diplay. 00004 * @details 00005 * Ver6.0 Addtional function is Animation sample code. goldfish swin form left to right, from bottom to top. 00006 * Ver5.0 Addtional function is checking the refresh display speed between 4bit,3bit and 1bit transfer mode 00007 * Ver4.0 Addtional function is Line buffer version.the Sample bitmap display color and monochrome by line buffer. 00008 * Ver3.0 Addtional function is font display. this demo code is the nunber is counted up like a meter panel 00009 * ver2.0 Addtional function is Monochome display by 1bit mode of SPI transfer. 00010 * 00011 * spi-transfer to Display has 3 mode. 00012 * 4bit mode is color display, this bit arrange is R,G,B,x. R,G,B = R,G,B subpixel bit. x bit is Dummy. 00013 * 3bit mode is color display, this bit arrange is R,G,B. R,G,B = R,G,B subpixel bit. No bit is Dummy. 00014 * 1bit mode is monocrome display,high speed refresh mode. a only Green subpixel of bitmap data is transfered. 00015 * <License> 00016 * Copyright 2018 Japan Display Inc. 00017 * Licensed under the Apache License, Version 2.0 (the "License"); 00018 * you may not use this file except in compliance with the License. 00019 * You may obtain a copy of the License at 00020 * 00021 * http://www.apache.org/licenses/LICENSE-2.0 00022 * 00023 * Unless required by applicable law or agreed to in writing, software 00024 * distributed under the License is distributed on an "AS IS" BASIS, 00025 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00026 * See the License for the specific language governing permissions and 00027 * limitations under the License. 00028 */ 00029 #include "stdio.h" 00030 #include "string" 00031 #include "mbed.h" 00032 #include "MIP8F_SPI.h" 00033 #include "SDFileSystem.h" 00034 #include "StateSW.h" 00035 #include "TglSW.h" 00036 00037 #include "Arial12x12.h" 00038 #include "Arial24x23.h" 00039 #include "Arial28x28.h" 00040 #include "Arial37x36.h" 00041 #include "Arial42x42.h" 00042 #include "font_big.h" 00043 #include "Arial94x94.h" 00044 00045 #define ON 1 00046 #define OFF 0 00047 00048 //K64F 00049 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); // mosi,miso,sck,cs 00050 memLCD8 WD(PTD2,PTD3,PTD1,PTD0,PTC4,PTD3); //PTC12); // mosi,miso,clk,cs,disp,power 00051 PwmOut BK(PTC3); 00052 PwmOut COM60HZ(PTC10); //add 20161108 00053 DigitalOut COMSEL(PTA2,0); //add 20161108 00054 DigitalOut BLCTRL(PTB10,0); //add 20171115 00055 // SW2 00056 StateSW swPWMorOFF(SW2); //PTC6); 00057 InterruptIn PWMorOFF(SW2); 00058 // SW3 00059 TglSW HaltSW(SW3); //PTA4); 00060 DigitalIn TexSW(SW3); 00061 00062 //for debug 00063 Serial pc(USBTX, USBRX); // tx, rx 00064 00065 void SDtex2BUF(char *filepath); 00066 00067 //function prototype 00068 void SD2BUF(char *filepath); 00069 void ifOFFseq(void); 00070 void ifswPWM(void); 00071 void OffSequence(void); 00072 void DispAllDir(const char *fsrc); 00073 void ReadBmp(const char *fsrc, uint32_t FileNum); 00074 uint32_t CntFile(const char *fsrc); 00075 00076 void fish_swim_RightToLeft(int y,int Speed,int No,int transfermode); 00077 void fish_swim_LeftToRight(int y,int Speed,int No,int transfermode); 00078 void fish_swim_BottomToTop(int x,int Speed,int No,int transfermode); 00079 void fish_swim_TopToBottom(int x,int Speed,int No,int transfermode); 00080 void fish_Surprise_LeftToRight(int y,int Speed,int no,int no2,int transfermode); 00081 //Grobal value 00082 int ifMargeTXT = 0; 00083 int width = 400; 00084 int height= 240; 00085 00086 float BKduty = 0; 00087 float BKjudge = 0; 00088 //double BKduty = 0; 00089 float bk_timer = 1.0; 00090 int blduty = 0; 00091 int bloff = 0; 00092 int blctrl_sel = 0; //0:40mA, 1:160mA //Y.S 00093 // Readed bitmap format, Color bit size = 1,4,8,(16),24,32 00094 uint16_t bmp_bitcount; 00095 00096 void ReadMovieBmp(const char *fsrc, uint32_t FileNum); 00097 00098 int main() { 00099 00100 uint32_t filenum; 00101 char filepath[60]; 00102 uint32_t filenum2; 00103 char filepath2[60]; 00104 //char countup[3+1]; 00105 //char KMPH[4+1]; 00106 //char Trans[13+1]; 00107 00108 sd.disk_initialize(); 00109 HaltSW.Enable(1); 00110 00111 00112 FILE *fp = fopen("/sd/settings2.txt", "rb"); //read binary 00113 if(fp != NULL) { 00114 fscanf(fp, "%d",&width); //width read 00115 fscanf(fp, "%d",&height); //height read 00116 fscanf(fp, "%d",&blctrl_sel);//blct_sel read //0:40mA, 1:160mA 00117 //sprintf(filepath,"/sd/%dx%d",width,height); //DispAllDir("/sd/180x180") //file name write to filepath 00118 sprintf(filepath,"/sd/backgrand_logo%dx%d",width,height); //DispAllDir("/sd/180x180") //file name write to filepath 00119 sprintf(filepath2,"/sd/fish%dx%d",width,height); //DispAllDir("/sd/180x180") //file name write to filepath 00120 fclose(fp); 00121 } else { 00122 FILE *fp = fopen("/sd/settings.txt", "rb"); //read binary 00123 if(fp != NULL) { 00124 fscanf(fp, "%d",&width); //width read 00125 fscanf(fp, "%d",&height); //height read 00126 sprintf(filepath,"/sd/%dx%d",width,height); //DispAllDir("/sd/180x180") //file name write to filepath 00127 fclose(fp); 00128 } else{ 00129 width =180; 00130 height=180; 00131 sprintf(filepath,"/sd/180x180"); //DispAllDir("/sd/180x180") 00132 } 00133 } 00134 00135 COMSEL.write(1); // 00136 00137 BLCTRL.write(blctrl_sel); // 00138 00139 WD.setWH(width,height); //input -> width, height 00140 WD.background(Black); //background = black 00141 WD.clsBUF(); //data initialize 00142 WD.writeDISP(); //picture write(black) 00143 WD.SwDisp(1); //disp on 00144 00145 PWMorOFF.fall(&ifswPWM); // Backlight //sw2 fall edge 00146 PWMorOFF.rise(&ifOFFseq); // OFF Sequence //sw2 rise edge 00147 00148 swPWMorOFF.Enable(8, 2, 1); //int ModeNum, int NoiseCancel, int OpenTime //???? 00149 swPWMorOFF.IfCntClose(ON); 00150 swPWMorOFF.IfCntState(ON); 00151 00152 COM60HZ.period_ms(8); //add 20161108 00153 COM60HZ.write(0.5); //add 20161108 00154 00155 BK.period_ms(1); //1ms cycle 20161012 00156 BK.write(1); //low output 20160725 <- high output 00157 00158 //DIR *d; 00159 filenum = CntFile(filepath); //file number read 00160 pc.printf("filenum=%d\n",filenum); 00161 00162 filenum2 = CntFile(filepath2); //file number read 00163 pc.printf("filenum2=%d\n",filenum2); 00164 for(int i = 0; i < filenum2; i++) 00165 { 00166 ReadMovieBmp(filepath2,i); 00167 } 00168 00169 00170 //int x,y; 00171 while(1) 00172 { //BITMAPS 00173 00174 for(int i = 0; i < filenum;i++) 00175 { 00176 //pc.printf("1\n"); 00177 while(HaltSW.State()); // VCOM invert when image is still 00178 HaltSW.Clear(); 00179 if( i%2 == 0 ) 00180 { 00181 //color anime 00182 ReadBmp(filepath,i); 00183 WD.writeDISP(TrBIT3); 00184 fish_swim_RightToLeft(60,10,2,TrBIT3); 00185 fish_swim_TopToBottom(200,15,0,TrBIT3); 00186 fish_swim_BottomToTop(100,10,4,TrBIT3); 00187 fish_Surprise_LeftToRight(80,30,2,6,TrBIT3); 00188 fish_swim_RightToLeft(80,10,8,TrBIT3); 00189 }else{ 00190 //monochrome anime 00191 ReadBmp(filepath,i); 00192 WD.writeDISP(TrBIT1); 00193 fish_swim_RightToLeft(60,10,2,TrBIT1); 00194 fish_swim_TopToBottom(200,15,4,TrBIT1); 00195 fish_swim_BottomToTop(100,10,4,TrBIT1); 00196 fish_Surprise_LeftToRight(80,30,2,6,TrBIT1); 00197 fish_swim_RightToLeft(80,5,8,TrBIT1); 00198 } 00199 } 00200 00201 } 00202 } 00203 /** 00204 * @brief Fish(bmp) swim from Right to Left 00205 */ 00206 void fish_swim_RightToLeft(int y,int Speed,int no,int transfermode) 00207 { 00208 int x; 00209 int i=no; 00210 for(x = width ; x >=-80 ; x-=Speed ) 00211 { 00212 //pc.printf("2\n"); 00213 WD.makeMovieFrame(x,y,i); 00214 //backgrand display 00215 WD.writeDISP(y,y+MOVIE_VERT_SIZE,transfermode); 00216 WD.RestoreMovieFrame(x,y,i); 00217 if( ++i > no+1 ) i = no; 00218 } 00219 } 00220 /** 00221 * @brief Fish(bmp) swim from Left to Right 00222 */ 00223 void fish_swim_LeftToRight(int y,int Speed,int no,int transfermode) 00224 { 00225 int x; 00226 int i=no; 00227 for(x = -80 ; x <=width+80 ; x+=Speed ) 00228 { 00229 //pc.printf("2\n"); 00230 WD.makeMovieFrame_Reverse(x,y,i); 00231 //backgrand display 00232 WD.writeDISP(y,y+MOVIE_VERT_SIZE,transfermode); 00233 WD.RestoreMovieFrame(x,y,i); 00234 if( ++i > no+1 ) i = no; 00235 } 00236 } 00237 /** 00238 * @brief Fish(bmp) swim from Left to Right,but he surprise!! and return 00239 */ 00240 void fish_Surprise_LeftToRight(int y,int Speed,int no,int no2,int transfermode) 00241 { 00242 int x; 00243 int i; 00244 i=no; 00245 for(x = -80 ; x <=width-120 ; x+=Speed ) 00246 { 00247 //pc.printf("2\n"); 00248 WD.makeMovieFrame_Reverse(x,y,i); 00249 //backgrand display 00250 WD.writeDISP(y,y+MOVIE_VERT_SIZE,transfermode); 00251 WD.RestoreMovieFrame(x,y,i); 00252 if( ++i > no+1 ) i = no; 00253 } 00254 //surprise 00255 i = no2; 00256 for(int j = 0 ; j < 6 ; j++ ) 00257 { 00258 x = width-120; 00259 //pc.printf("2\n"); 00260 WD.makeMovieFrame(x,y,i); 00261 //backgrand display 00262 WD.writeDISP(y,y+MOVIE_VERT_SIZE,transfermode); 00263 WD.RestoreMovieFrame(x,y,i); 00264 if( ++i > no2+1 ) i = no2; 00265 } 00266 // swim reverse 00267 i = no; 00268 for(x = width-120 ; x >= -80 ; x-=Speed ) 00269 { 00270 //pc.printf("2\n"); 00271 WD.makeMovieFrame(x,y,i); 00272 //backgrand display 00273 WD.writeDISP(y,y+MOVIE_VERT_SIZE,transfermode); 00274 WD.RestoreMovieFrame(x,y,i); 00275 if( ++i > no+1 ) i = no; 00276 } 00277 } 00278 00279 /** 00280 * @brief Fish(bmp) swim from bottom to top 00281 */ 00282 void fish_swim_BottomToTop(int x,int Speed,int no,int transfermode) 00283 { 00284 int y; 00285 int i=no; 00286 for(y = height ; y >=-MOVIE_VERT_SIZE ; y-=Speed ) 00287 { 00288 //pc.printf("2\n"); 00289 WD.makeMovieFrame(x,y,i); 00290 //backgrand display 00291 WD.writeDISP(y,y+MOVIE_VERT_SIZE+Speed,transfermode); 00292 WD.RestoreMovieFrame(x,y,i); 00293 if( ++i > no+1 ) i = no; 00294 } 00295 } 00296 /** 00297 * @brief Fish(bmp) swim from top to bottom 00298 */ 00299 void fish_swim_TopToBottom(int x,int Speed,int no,int transfermode) 00300 { 00301 int y; 00302 int i=no; 00303 for(y = -MOVIE_VERT_SIZE ; y < height+Speed; y+=Speed ) 00304 { 00305 //pc.printf("2\n"); 00306 WD.makeMovieFrame_Updown(x,y,i); 00307 //backgrand display 00308 WD.writeDISP(y-Speed,y+MOVIE_VERT_SIZE,transfermode); 00309 WD.RestoreMovieFrame(x,y,i); 00310 if( ++i > no+1 ) i = no; 00311 } 00312 } 00313 /** 00314 * @brief read a bitmap file in SD. 8color mode 00315 */ 00316 void SD2BUF(char *filepath){ 00317 char R8[1],G8[1],B8[1] ,DUMMY[1],bc[2]; 00318 char RGB; 00319 FILE *fp ; 00320 00321 pc.printf("file=%s\n",filepath); 00322 fp = fopen(filepath, "rb"); 00323 if(fp != NULL) { 00324 //for(int i=0; i< 54 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 54bytes 00325 for(int i=0; i< 28 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 26bytes 00326 fscanf(fp,"%c",&(bc[0]));// color bit size 2bytes 00327 fscanf(fp,"%c",&(bc[1]));// color bit size 2bytes 00328 for(int i=0; i< 24 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 26bytes 00329 bmp_bitcount = bc[0]+bc[1]*256; 00330 if( bmp_bitcount == 1 ) 00331 { 00332 for(int i=0; i< 8 ; i++) fscanf(fp,"%c",DUMMY); // Pallet Data 00333 int x; 00334 for(int y=height-1; y>=0; y--) { 00335 for(x=0; x< width; x += 8) { //1bit monochrome 00336 fscanf(fp, "%c",G8); 00337 for(int i=0; i < 8 ; i++ ){ 00338 RGB = 0; 00339 if( (*G8 & 0x80) != 0) RGB = RGB|0x0e; 00340 WD.pixel(x+i,y,RGB); 00341 *G8 = *G8 << 1; 00342 } 00343 } 00344 if( width%8 != 0 ) 00345 { 00346 fscanf(fp, "%c",G8); 00347 int i; 00348 for(i=0; i < (width%8) ; i++ ){ 00349 RGB = 0; 00350 if( (*G8 & 0x40) != 0) RGB = RGB|0x0e; 00351 WD.pixel(x+i,y,RGB); 00352 *G8 = *G8 << 1; 00353 } 00354 } 00355 if( y!=0){ // The last data column doesn't need padding 00356 int wbyte = width/8 + ( width%8 == 0 ? 0:1 ); 00357 for(int x=(wbyte*3)%4; (x%4 !=0); x++) fscanf(fp, "%c",DUMMY);// 4byte boundery for every column(only windows bitmap format) 00358 } 00359 } 00360 }else 00361 if( bmp_bitcount == 24 ) 00362 { 00363 for(int y=height-1; y>=0; y--) { 00364 for(int x=0; x< width; x++) { //24bit color B 8bit -> G 8bit -> R 8bit 00365 fscanf(fp, "%c",B8); 00366 fscanf(fp, "%c",G8); 00367 fscanf(fp, "%c",R8); 00368 00369 RGB = RGB8(*R8,*G8,*B8); //6bit(8bit) MIP MASK 0000 1110 00370 WD.pixel(x,y,RGB); 00371 } 00372 if( y!=0) // The last data column doesn't need padding 00373 for(int x=(width*3)%4; (x%4 !=0); x++) fscanf(fp, "%c",DUMMY); // 4byte boundery for every column(only windows bitmap format) 00374 } 00375 } 00376 } 00377 fclose(fp); 00378 } 00379 00380 /** 00381 * @brief read a text file in SD. 00382 */ 00383 void SDtex2BUF(char *filepath){ 00384 int x,y,font,color; 00385 char text[40]; 00386 int ifEOF; 00387 FILE *fp ; 00388 00389 fp = fopen(filepath, "r"); 00390 if(fp != NULL) { 00391 while(ifEOF != -1){ 00392 ifEOF = fscanf(fp,"%d,%d,%d,%d,%[^,],",&x,&y,&font,&color,text); 00393 WD.locate(x,y); 00394 WD.foreground(color); 00395 if (font ==1) WD.set_font((unsigned char*) Arial12x12); 00396 else if (font ==2) WD.set_font((unsigned char*) Arial24x23); 00397 else if (font ==3) WD.set_font((unsigned char*) Arial28x28); 00398 else WD.set_font((unsigned char*) Neu42x35); 00399 00400 WD.printf("%s",text); 00401 } 00402 } 00403 fclose(fp); 00404 } 00405 00406 /* 00407 void DispAllDir(const char *fsrc) 00408 { 00409 DIR *d = opendir(fsrc); 00410 struct dirent *p; 00411 char filepath[40]; 00412 00413 while ((p = readdir(d)) != NULL) { 00414 sprintf(filepath, "%s/%s",fsrc,p->d_name); 00415 SD2BUF(filepath); 00416 WD.writeDISP(); 00417 } 00418 closedir(d); 00419 } 00420 */ 00421 00422 /** 00423 * @brief read a bitmap file in SD by file number. 00424 */ 00425 void ReadBmp(const char *fsrc, uint32_t FileNum) 00426 { 00427 DIR *d = opendir(fsrc); 00428 struct dirent *p; 00429 char filepath[60]; 00430 00431 for(uint32_t i=0; i< FileNum; i++) readdir(d); 00432 if ((p = readdir(d)) != NULL) { 00433 sprintf(filepath, "%s/%s",fsrc,p->d_name); 00434 SD2BUF(filepath); 00435 if(ifMargeTXT){ 00436 sprintf(filepath, "%s_txt/%s.txt",fsrc,p->d_name); 00437 SDtex2BUF(filepath); 00438 } 00439 } 00440 closedir(d); 00441 } 00442 00443 uint32_t CntFile(const char *fsrc) 00444 { 00445 DIR *d = opendir(fsrc); 00446 uint32_t counter = 0; 00447 while (readdir(d)!= NULL) counter++; 00448 closedir(d); 00449 return counter; 00450 } 00451 00452 void OffSequence(void){ 00453 BK.write(0); //add 20160712 17:00 00454 WD.background(Black); 00455 WD.clsBUF(); 00456 WD.SwDisp(0); 00457 WD.writeDISP(); 00458 WD.writeDISP(); 00459 bloff = 1; 00460 } 00461 00462 void ifswPWM(void){ 00463 if(bloff == 0){ 00464 if(blduty >= 10){ //0 00465 blduty = 0; //10 00466 COMSEL.write(1); // 00467 }else{ 00468 blduty += 1; //-= 00469 COMSEL.write(1); // 00470 } 00471 }else{ 00472 blduty = 0; 00473 COMSEL.write(1); // 00474 } 00475 // BKduty = BKduty - 0.125; 00476 // BKduty -= 0.125; 00477 // if(BKduty <= 0) BKduty = 1; 00478 // BK.write(BKduty); 00479 BK.write(blduty*0.1); // 00480 if(blduty == 0){ 00481 bk_timer = 1.0; 00482 }else{ 00483 bk_timer = 0.008; //0.016 20160712 00484 } 00485 } 00486 00487 void ifOFFseq(void){ 00488 if(swPWMorOFF.IfAtTime()){ 00489 swPWMorOFF.IfCntClose(OFF); 00490 swPWMorOFF.IfCntState(OFF); 00491 OffSequence(); 00492 } 00493 } 00494 /** 00495 * @brief read a bitmap,Animation page file in SD. 00496 */ 00497 void SD2MOVIEBUF(char *filepath,int memnum){ 00498 char R8[1],G8[1],B8[1] ,DUMMY[1],bc[2]; 00499 char RGB; 00500 FILE *fp ; 00501 00502 pc.printf("[%d]%s\n",memnum,filepath); 00503 fp = fopen(filepath, "rb"); 00504 if(fp != NULL) { 00505 //for(int i=0; i< 54 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 54bytes 00506 for(int i=0; i< 28 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 26bytes 00507 fscanf(fp,"%c",&(bc[0]));// color bit size 2bytes 00508 fscanf(fp,"%c",&(bc[1]));// color bit size 2bytes 00509 for(int i=0; i< 24 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 26bytes 00510 bmp_bitcount = bc[0]+bc[1]*256; 00511 pc.printf("bitcount=%d\n",bmp_bitcount); 00512 if( bmp_bitcount == 1 ) 00513 { 00514 for(int i=0; i< 8 ; i++) fscanf(fp,"%c",DUMMY); // Pallet Data 00515 int x; 00516 for(int y=MOVIE_VERT_SIZE-1; y>=0; y--) { 00517 for(x=0; x< MOVIE_HORI_SIZE; x += 8) { //1bit monochrome 00518 fscanf(fp, "%c",G8); 00519 for(int i=0; i < 8 ; i++ ){ 00520 RGB = 0; 00521 if( (*G8 & 0x80) != 0) RGB = RGB|0x0e; 00522 WD.movie_pixel(x+i,y,RGB,memnum); 00523 *G8 = *G8 << 1; 00524 } 00525 } 00526 if( MOVIE_HORI_SIZE%8 != 0 ) 00527 { 00528 fscanf(fp, "%c",G8); 00529 int i; 00530 for(i=0; i < (width%8) ; i++ ){ 00531 RGB = 0; 00532 if( (*G8 & 0x40) != 0) RGB = RGB|0x0e; 00533 WD.movie_pixel(x+i,y,RGB,memnum); 00534 *G8 = *G8 << 1; 00535 } 00536 } 00537 if( y!=0){ // The last data column doesn't need padding 00538 int wbyte = MOVIE_HORI_SIZE/8 + ( MOVIE_HORI_SIZE%8 == 0 ? 0:1 ); 00539 for(int x=(wbyte*3)%4; (x%4 !=0); x++) fscanf(fp, "%c",DUMMY);// 4byte boundery for every column(only windows bitmap format) 00540 } 00541 } 00542 }else 00543 if( bmp_bitcount == 24 ) 00544 { 00545 for(int y=MOVIE_VERT_SIZE-1; y>=0; y--) { 00546 for(int x=0; x< MOVIE_HORI_SIZE; x++) { //24bit color B 8bit -> G 8bit -> R 8bit 00547 fscanf(fp, "%c",B8); 00548 fscanf(fp, "%c",G8); 00549 fscanf(fp, "%c",R8); 00550 00551 RGB = RGB8(*R8,*G8,*B8); //6bit(8bit) MIP MASK 0000 1110 00552 WD.movie_pixel(x,y,RGB,memnum); 00553 } 00554 if( y!=0) // The last data column doesn't need padding 00555 for(int x=(MOVIE_HORI_SIZE*3)%4; (x%4 !=0); x++) fscanf(fp, "%c",DUMMY); // 4byte boundery for every column(only windows bitmap format) 00556 } 00557 } 00558 } 00559 fclose(fp); 00560 } 00561 /** 00562 * @brief read a bitmap,All Animation page file in SD. 00563 */ 00564 void ReadMovieBmp(const char *fsrc, uint32_t FileNum) 00565 { 00566 DIR *d = opendir(fsrc); 00567 struct dirent *p; 00568 char filepath[60]; 00569 00570 if( FileNum >= MOVIE_NUM ) return; 00571 00572 pc.printf("read bmp No%d\n",FileNum); 00573 for(uint32_t i=0; i< FileNum; i++) readdir(d); 00574 if ((p = readdir(d)) != NULL) { 00575 sprintf(filepath, "%s/%s",fsrc,p->d_name); 00576 SD2MOVIEBUF(filepath,FileNum); 00577 pc.printf("read finish\n"); 00578 } 00579 closedir(d); 00580 }
Generated on Wed Jul 13 2022 14:26:38 by
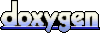