Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Dependents: testUniGraphic_150217 maze_TFT_MMA8451Q TFT_test_frdm-kl25z TFT_test_NUCLEO-F411RE ... more
ILI9486 Class Reference
Class for ILI9486 tft display controller to be copypasted and adapted for other controllers. More...
#include <ILI9486.h>
Inherits TFT.
Public Member Functions | |
ILI9486 (proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name, unsigned int LCDSIZE_X=320, unsigned int LCDSIZE_Y=480) | |
Create a PAR display interface. | |
ILI9486 (proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, PinName DC, const char *name, unsigned int LCDSIZE_X=320, unsigned int LCDSIZE_Y=480) | |
Create an SPI display interface. | |
virtual void | pixel (int x, int y, unsigned short color) |
Draw a pixel in the specified color. | |
virtual void | window (int x, int y, int w, int h) |
Set the window, which controls where items are written to the screen. | |
virtual unsigned short | pixelread (int x, int y) |
Read pixel color at location. | |
virtual void | window4read (int x, int y, int w, int h) |
Set the window from which gram is read from. | |
virtual void | window_pushpixel (unsigned short color) |
Push a single pixel into the window and increment position. | |
virtual void | window_pushpixel (unsigned short color, unsigned int count) |
Push some pixels of the same color into the window and increment position. | |
virtual void | window_pushpixelbuf (unsigned short *color, unsigned int lenght) |
Push array of pixel colors into the window and increment position. | |
virtual void | copy_to_lcd () |
Framebuffer is not used for TFT. | |
void | invert (unsigned char o) |
display inverted colors | |
virtual void | cls () |
clear the entire screen The inherited one sets windomax then fill with background color We override it to speedup | |
virtual void | set_orientation (int o) |
Set the orientation of the screen x,y: 0,0 is always top left. | |
virtual void | BusEnable (bool enable) |
Set ChipSelect high or low. | |
void | FastWindow (bool enable) |
Enable fast window (default disabled) used to speedup functions that plots single pixels, like circle, oblique lines or just sparse pixels. | |
void | setscrollarea (int startY, int areasize) |
Set scroll area boundaries scroll is done in hw but only on the native vertical axis TFTs are mainly native protrait view, so horizontal scroll if rotated in landscape view. | |
void | scroll (int lines) |
Scroll up(or left) the scrollarea. | |
void | scrollreset () |
Reset the scrollarea and display un-scrolled screen. | |
int | sizeX () |
get display X size in pixels (native, orientation independent) | |
int | sizeY () |
get display Y size in pixels (native, orientation independent) | |
virtual void | WindowMax (void) |
Set window to max possible size May be overridden in a derived class. | |
virtual void | circle (int x, int y, int r, unsigned short color) |
draw a circle | |
virtual void | fillcircle (int x, int y, int r, unsigned short color) |
draw a filled circle | |
virtual void | line (int x0, int y0, int x1, int y1, unsigned short color) |
draw a 1 pixel line | |
void | hline (int x0, int x1, int y, unsigned short color) |
draw a horizontal line | |
void | vline (int y0, int y1, int x, unsigned short color) |
draw a vertical line | |
virtual void | rect (int x0, int y0, int x1, int y1, unsigned short color) |
draw a rect | |
virtual void | fillrect (int x0, int y0, int x1, int y1, unsigned short color) |
draw a filled rect | |
virtual void | locate (int x, int y) |
setup cursor position for text | |
virtual int | _putc (int value) |
put a char on the screen | |
virtual void | character (int x, int y, int c) |
draw a character on given position out of the active font to the TFT | |
void | Bitmap (int x, int y, int w, int h, unsigned char *bitmap) |
paint a bitmap on the TFT | |
void | Bitmap_BW (Bitmap_s bm, int x, int y) |
paint monochrome bitmap to screen | |
int | BMP_16 (int x, int y, const char *Name_BMP) |
paint a 16 bit BMP from filesytem on the TFT (slow) | |
void | set_font (unsigned char *f, unsigned char firstascii=32, unsigned char lastascii=127, bool proportional=true) |
select the font to use | |
void | set_font_zoom (unsigned char x_mul, unsigned char y_mul) |
Zoom fount. | |
virtual int | columns () |
Get the number of columns based on the currently active font. | |
virtual int | rows () |
Get the number of rows based on the currently active font. | |
int | width () |
get the current oriented screen width in pixels | |
int | height () |
get the current oriented screen height in pixels | |
void | set_width (int width) |
set the current oriented screen width in pixels | |
void | set_height (int height) |
set the current oriented screen height in pixels | |
void | set_auto_up (bool up) |
setup auto update of screen | |
bool | get_auto_up (void) |
get status of the auto update function | |
virtual bool | claim (FILE *stream) |
redirect output from a stream (stoud, sterr) to display | |
virtual void | foreground (uint16_t colour) |
set the foreground color | |
virtual void | background (uint16_t colour) |
set the background color | |
Protected Member Functions | |
void | init () |
Init command sequence. | |
void | wr_cmd8 (unsigned char cmd) |
Send 8bit command to display controller. | |
void | wr_data8 (unsigned char data) |
Send 8bit data to display controller. | |
void | wr_data16 (unsigned short data) |
Send 2x8bit data to display controller. | |
virtual void | wr_gram (unsigned short data) |
Send 16bit pixeldata to display controller. | |
virtual void | wr_gram (unsigned short data, unsigned int count) |
Send same 16bit pixeldata to display controller multiple times. | |
virtual void | wr_grambuf (unsigned short *data, unsigned int lenght) |
Send array of pixeldata shorts to display controller. | |
virtual unsigned short | rd_gram () |
Read 16bit pixeldata from display controller (with dummy cycle) | |
virtual unsigned int | rd_reg_data32 (unsigned char reg) |
Read 4x8bit register data (with dummy cycle) | |
virtual unsigned int | rd_extcreg_data32 (unsigned char reg, unsigned char SPIreadenablecmd) |
Read 3x8bit ExtendedCommands register data. | |
void | hw_reset () |
HW reset sequence (without display init commands) | |
virtual void | auto_gram_read_format () |
Try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR autoset internal flags so pixelread() will always return correct value. | |
virtual void | identify () |
Try to identify display ID. |
Detailed Description
Class for ILI9486 tft display controller to be copypasted and adapted for other controllers.
Definition at line 12 of file ILI9486.h.
Constructor & Destructor Documentation
ILI9486 | ( | proto_t | displayproto, |
PortName | port, | ||
PinName | CS, | ||
PinName | reset, | ||
PinName | DC, | ||
PinName | WR, | ||
PinName | RD, | ||
const char * | name, | ||
unsigned int | LCDSIZE_X = 320 , |
||
unsigned int | LCDSIZE_Y = 480 |
||
) |
Create a PAR display interface.
- Parameters:
-
displayproto PAR_8 or PAR_16 port GPIO port name to use CS pin connected to CS of display reset pin connected to RESET of display DC pin connected to data/command of display WR pin connected to SDI of display RD pin connected to RS of display name The name used by the parent class to access the interface LCDSIZE_X x size in pixel - optional LCDSIZE_Y y size in pixel - optional
Definition at line 18 of file ILI9486.cpp.
ILI9486 | ( | proto_t | displayproto, |
int | Hz, | ||
PinName | mosi, | ||
PinName | miso, | ||
PinName | sclk, | ||
PinName | CS, | ||
PinName | reset, | ||
PinName | DC, | ||
const char * | name, | ||
unsigned int | LCDSIZE_X = 320 , |
||
unsigned int | LCDSIZE_Y = 480 |
||
) |
Create an SPI display interface.
- Parameters:
-
displayproto SPI_8 or SPI_16 Hz SPI speed in Hz mosi SPI pin miso SPI pin sclk SPI pin CS pin connected to CS of display reset pin connected to RESET of display DC pin connected to data/command of display name The name used by the parent class to access the interface LCDSIZE_X x size in pixel - optional LCDSIZE_Y y size in pixel - optional
Definition at line 31 of file ILI9486.cpp.
Member Function Documentation
int _putc | ( | int | value ) | [virtual, inherited] |
put a char on the screen
- Parameters:
-
value char to print
- Returns:
- printed char
Reimplemented from TextDisplay.
Definition at line 256 of file GraphicsDisplay.cpp.
void auto_gram_read_format | ( | ) | [protected, virtual, inherited] |
Try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR autoset internal flags so pixelread() will always return correct value.
void background | ( | uint16_t | colour ) | [virtual, inherited] |
set the background color
- Note:
- this method may be overridden in a derived class.
- Parameters:
-
color is color to use for background drawing.
Definition at line 61 of file TextDisplay.cpp.
void Bitmap | ( | int | x, |
int | y, | ||
int | w, | ||
int | h, | ||
unsigned char * | bitmap | ||
) | [inherited] |
paint a bitmap on the TFT
- Parameters:
-
x,y : upper left corner w width of bitmap h high of bitmap *bitmap pointer to the bitmap data
bitmap format: 16 bit R5 G6 B5
use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 use winhex to load this file and mark data stating at offset 0x46 to end use edit -> copy block -> C Source to export C array paste this array into your program
define the array as static const unsigned char to put it into flash memory cast the pointer to (unsigned char *) : tft.Bitmap(10,40,309,50,(unsigned char *)scala);
Definition at line 349 of file GraphicsDisplay.cpp.
void Bitmap_BW | ( | Bitmap_s | bm, |
int | x, | ||
int | y | ||
) | [inherited] |
paint monochrome bitmap to screen
- Parameters:
-
bm Bitmap in flash x x start y y start
Definition at line 324 of file GraphicsDisplay.cpp.
int BMP_16 | ( | int | x, |
int | y, | ||
const char * | Name_BMP | ||
) | [inherited] |
paint a 16 bit BMP from filesytem on the TFT (slow)
- Parameters:
-
x,y : position of upper left corner *Name_BMP name of the BMP file with drive: "/local/test.bmp"
- Returns:
- 1 if bmp file was found and painted
- 0 if bmp file was found not found
- -1 if file is no bmp
- -2 if bmp file is no 16 bit bmp
- -3 if bmp file is to big for screen
- -4 if buffer malloc go wrong
bitmap format: 16 bit R5 G6 B5
use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 copy to internal file system or SD card
Definition at line 375 of file GraphicsDisplay.cpp.
void BusEnable | ( | bool | enable ) | [virtual, inherited] |
void character | ( | int | x, |
int | y, | ||
int | c | ||
) | [virtual, inherited] |
draw a character on given position out of the active font to the TFT
- Parameters:
-
x x-position of char (top left) y y-position c char to print
Implements TextDisplay.
Definition at line 270 of file GraphicsDisplay.cpp.
void circle | ( | int | x, |
int | y, | ||
int | r, | ||
unsigned short | color | ||
) | [virtual, inherited] |
draw a circle
- Parameters:
-
x0,y0 center r radius color 16 bit color *
Definition at line 60 of file GraphicsDisplay.cpp.
bool claim | ( | FILE * | stream ) | [virtual, inherited] |
redirect output from a stream (stoud, sterr) to display
- Parameters:
-
stream stream that shall be redirected to the TextDisplay
- Note:
- this method may be overridden in a derived class.
- Returns:
- true if the claim succeeded.
Definition at line 65 of file TextDisplay.cpp.
void cls | ( | void | ) | [virtual, inherited] |
clear the entire screen The inherited one sets windomax then fill with background color We override it to speedup
Reimplemented from GraphicsDisplay.
Reimplemented in SEPS225.
int columns | ( | ) | [virtual, inherited] |
Get the number of columns based on the currently active font.
- Returns:
- number of columns.
- Note:
- this method may be overridden in a derived class.
Implements TextDisplay.
Definition at line 228 of file GraphicsDisplay.cpp.
virtual void copy_to_lcd | ( | void | ) | [virtual, inherited] |
void FastWindow | ( | bool | enable ) | [inherited] |
void fillcircle | ( | int | x, |
int | y, | ||
int | r, | ||
unsigned short | color | ||
) | [virtual, inherited] |
draw a filled circle
- Parameters:
-
x0,y0 center r radius color 16 bit color *
Definition at line 77 of file GraphicsDisplay.cpp.
void fillrect | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
unsigned short | color | ||
) | [virtual, inherited] |
draw a filled rect
- Parameters:
-
x0,y0 top left corner x1,y1 down right corner color 16 bit color
Definition at line 209 of file GraphicsDisplay.cpp.
void foreground | ( | uint16_t | colour ) | [virtual, inherited] |
set the foreground color
- Note:
- this method may be overridden in a derived class.
- Parameters:
-
color is color to use for foreground drawing.
Definition at line 57 of file TextDisplay.cpp.
bool get_auto_up | ( | void | ) | [inherited] |
get status of the auto update function
- Returns:
- if auto update is on
Definition at line 471 of file GraphicsDisplay.cpp.
int height | ( | ) | [inherited] |
get the current oriented screen height in pixels
- Returns:
- screen height in pixels.
Definition at line 56 of file GraphicsDisplay.cpp.
void hline | ( | int | x0, |
int | x1, | ||
int | y, | ||
unsigned short | color | ||
) | [inherited] |
draw a horizontal line
- Parameters:
-
x0 horizontal start x1 horizontal stop y vertical position color 16 bit color
Definition at line 98 of file GraphicsDisplay.cpp.
void hw_reset | ( | ) | [protected, inherited] |
void identify | ( | ) | [protected, virtual, inherited] |
void init | ( | ) | [protected] |
Init command sequence.
Definition at line 45 of file ILI9486.cpp.
void invert | ( | unsigned char | o ) | [inherited] |
void line | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
unsigned short | color | ||
) | [virtual, inherited] |
draw a 1 pixel line
- Parameters:
-
x0,y0 start point x1,y1 stop point color 16 bit color
Definition at line 116 of file GraphicsDisplay.cpp.
void locate | ( | int | x, |
int | y | ||
) | [virtual, inherited] |
setup cursor position for text
- Parameters:
-
x x-position (top left) y y-position
Reimplemented from TextDisplay.
Definition at line 223 of file GraphicsDisplay.cpp.
void pixel | ( | int | x, |
int | y, | ||
unsigned short | color | ||
) | [virtual, inherited] |
Draw a pixel in the specified color.
- Parameters:
-
x is the horizontal offset to this pixel. y is the vertical offset to this pixel. color defines the color for the pixel.
Implements GraphicsDisplay.
Reimplemented in SEPS225.
unsigned short pixelread | ( | int | x, |
int | y | ||
) | [virtual, inherited] |
unsigned int rd_extcreg_data32 | ( | unsigned char | reg, |
unsigned char | SPIreadenablecmd | ||
) | [protected, virtual, inherited] |
Read 3x8bit ExtendedCommands register data.
- Parameters:
-
reg the register to read SPIreadenablecmd vendor/device specific cmd to read EXTC registers
- Returns:
- data as uint
- Note:
- EXTC regs (0xB0 to 0xFF) are read/write registers but needs special cmd to be read in SPI mode
unsigned short rd_gram | ( | ) | [protected, virtual, inherited] |
unsigned int rd_reg_data32 | ( | unsigned char | reg ) | [protected, virtual, inherited] |
void rect | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
unsigned short | color | ||
) | [virtual, inherited] |
draw a rect
- Parameters:
-
x0,y0 top left corner x1,y1 down right corner color 16 bit color *
Reimplemented in SEPS225.
Definition at line 187 of file GraphicsDisplay.cpp.
int rows | ( | ) | [virtual, inherited] |
Get the number of rows based on the currently active font.
- Returns:
- number of rows.
- Note:
- this method may be overridden in a derived class.
Implements TextDisplay.
Definition at line 232 of file GraphicsDisplay.cpp.
void scroll | ( | int | lines ) | [inherited] |
void scrollreset | ( | ) | [inherited] |
void set_auto_up | ( | bool | up ) | [inherited] |
setup auto update of screen
- Parameters:
-
up 1 = on , 0 = off if switched off the program has to call copy_to_lcd() to update screen from framebuffer
Definition at line 466 of file GraphicsDisplay.cpp.
void set_font | ( | unsigned char * | f, |
unsigned char | firstascii = 32 , |
||
unsigned char | lastascii = 127 , |
||
bool | proportional = true |
||
) | [inherited] |
select the font to use
- Parameters:
-
f pointer to font array firstascii first ascii code present in font array, default 32 (space) lastascii last ascii code present in font array, default 127 (DEL) proportional enable/disable variable font width (default enabled)
font array can created with GLCD Font Creator from http://www.mikroe.com you have to add 4 parameter at the beginning of the font array to use:
- the number of byte / char (not used in this revision, set to whatever)
- the vertial size in pixel
- the horizontal size in pixel
- the number of byte per vertical line (not used in this revision, set to whatever) you also have to change the array to cont unsigned char[] and __align(2)
Definition at line 236 of file GraphicsDisplay.cpp.
void set_font_zoom | ( | unsigned char | x_mul, |
unsigned char | y_mul | ||
) | [inherited] |
Zoom fount.
- Parameters:
-
x_mul horizontal size multiplier y_mul vertical size multiplier
Definition at line 251 of file GraphicsDisplay.cpp.
void set_height | ( | int | height ) | [inherited] |
set the current oriented screen height in pixels
- Parameters:
-
height screen height in pixels.
Definition at line 48 of file GraphicsDisplay.cpp.
void set_orientation | ( | int | o ) | [virtual, inherited] |
void set_width | ( | int | width ) | [inherited] |
set the current oriented screen width in pixels
- Parameters:
-
width screen width in pixels.
Definition at line 44 of file GraphicsDisplay.cpp.
void setscrollarea | ( | int | startY, |
int | areasize | ||
) | [inherited] |
Set scroll area boundaries scroll is done in hw but only on the native vertical axis TFTs are mainly native protrait view, so horizontal scroll if rotated in landscape view.
- Parameters:
-
startY boundary offset from top (or left if rotated), 0 for fullscreen scroll areasize size of the scroll area, 480 for fullscreen scroll of a 320x480 display
int sizeX | ( | ) | [inherited] |
int sizeY | ( | ) | [inherited] |
void vline | ( | int | y0, |
int | y1, | ||
int | x, | ||
unsigned short | color | ||
) | [inherited] |
draw a vertical line
- Parameters:
-
x horizontal position y0 vertical start y1 vertical stop color 16 bit color
Definition at line 107 of file GraphicsDisplay.cpp.
int width | ( | ) | [inherited] |
get the current oriented screen width in pixels
- Returns:
- screen width in pixels.
Definition at line 52 of file GraphicsDisplay.cpp.
void window | ( | int | x, |
int | y, | ||
int | w, | ||
int | h | ||
) | [virtual, inherited] |
Set the window, which controls where items are written to the screen.
When something hits the window width, it wraps back to the left side and down a row. If the initial write is outside the window, it will be captured into the window when it crosses a boundary.
- Parameters:
-
x is the left edge in pixels. y is the top edge in pixels. w is the window width in pixels. h is the window height in pixels.
Implements GraphicsDisplay.
Reimplemented in SEPS225.
void window4read | ( | int | x, |
int | y, | ||
int | w, | ||
int | h | ||
) | [virtual, inherited] |
void window_pushpixel | ( | unsigned short | color, |
unsigned int | count | ||
) | [virtual, inherited] |
Push some pixels of the same color into the window and increment position.
You must first call window() then push pixels.
- Parameters:
-
color is the pixel color. count,: how many
Implements GraphicsDisplay.
Reimplemented in SEPS225.
void window_pushpixel | ( | unsigned short | color ) | [virtual, inherited] |
Push a single pixel into the window and increment position.
You must first call window() then push pixels.
- Parameters:
-
color is the pixel color.
Implements GraphicsDisplay.
Reimplemented in SEPS225.
void window_pushpixelbuf | ( | unsigned short * | color, |
unsigned int | lenght | ||
) | [virtual, inherited] |
Push array of pixel colors into the window and increment position.
You must first call window() then push pixels.
- Parameters:
-
color is the pixel color.
Implements GraphicsDisplay.
Reimplemented in SEPS225.
void WindowMax | ( | void | ) | [virtual, inherited] |
Set window to max possible size May be overridden in a derived class.
Definition at line 40 of file GraphicsDisplay.cpp.
void wr_cmd8 | ( | unsigned char | cmd ) | [protected, inherited] |
void wr_data16 | ( | unsigned short | data ) | [protected, inherited] |
void wr_data8 | ( | unsigned char | data ) | [protected, inherited] |
void wr_gram | ( | unsigned short | data, |
unsigned int | count | ||
) | [protected, virtual, inherited] |
void wr_gram | ( | unsigned short | data ) | [protected, virtual, inherited] |
Generated on Tue Jul 12 2022 13:49:08 by
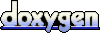