A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
lpc_swim_image.c
00001 /* 00002 * @brief SWIM image management 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #include "lpc_types.h" 00033 #include "lpc_swim_image.h" 00034 00035 /***************************************************************************** 00036 * Private types/enumerations/variables 00037 ****************************************************************************/ 00038 00039 /***************************************************************************** 00040 * Public types/enumerations/variables 00041 ****************************************************************************/ 00042 00043 /***************************************************************************** 00044 * Private functions 00045 ****************************************************************************/ 00046 00047 /***************************************************************************** 00048 * Public functions 00049 ****************************************************************************/ 00050 00051 /* Puts a raw image into a window */ 00052 void swim_put_image(SWIM_WINDOW_T *win, 00053 const COLOR_T *image, 00054 int32_t xsize, 00055 int32_t ysize) 00056 { 00057 int32_t x, y; 00058 00059 /* Unknown values of rtype will do no rotation */ 00060 y = win->ypvmin; 00061 00062 xsize = xsize + win->xpvmin; 00063 ysize = ysize + win->ypvmin; 00064 00065 /* Move image to window pixel by pixel */ 00066 while ((y <= win->ypvmax) && (y < ysize)) { 00067 /* Set physical frame buffer address */ 00068 x = win->xpvmin; 00069 00070 /* Render a single line */ 00071 while ((x <= win->xpvmax) && (x < xsize)) { 00072 swim_put_pixel_physical(win, x, y, *image); 00073 image++; 00074 x++; 00075 } 00076 00077 /* Adjust to end of line if the image was clipped */ 00078 image = image + (xsize - x); 00079 00080 y++; 00081 } 00082 } 00083 00084 /* Puts a raw image into a window at a specific position*/ 00085 void swim_put_image_xy(SWIM_WINDOW_T *win, 00086 const COLOR_T *image, 00087 int32_t xsize, 00088 int32_t ysize, 00089 int32_t x1, 00090 int32_t y1) 00091 { 00092 int32_t x, y; 00093 00094 /* Unknown values of rtype will do no rotation */ 00095 y = win->ypvmin + y1; 00096 00097 xsize = xsize + win->xpvmin + x1; 00098 ysize = ysize + win->ypvmin + y1; 00099 00100 /* Move image to window pixel by pixel */ 00101 while ((y <= win->ypvmax) && (y < ysize)) { 00102 /* Set physical frame buffer address */ 00103 x = win->xpvmin + x1; 00104 00105 /* Render a single line */ 00106 while ((x <= win->xpvmax) && (x < xsize)) { 00107 swim_put_pixel_physical(win, x, y, *image); 00108 image++; 00109 x++; 00110 } 00111 00112 /* Adjust to end of line if the image was clipped */ 00113 image = image + (xsize - x); 00114 00115 y++; 00116 } 00117 } 00118 00119 /* Puts a raw image into a window at a specific position, skipping all transparent pixels */ 00120 void swim_put_transparent_image_xy(SWIM_WINDOW_T *win, 00121 const COLOR_T *image, 00122 int32_t xsize, 00123 int32_t ysize, 00124 int32_t x1, 00125 int32_t y1, 00126 COLOR_T tColor) 00127 { 00128 int32_t x, y; 00129 00130 /* Unknown values of rtype will do no rotation */ 00131 y = win->ypvmin + y1; 00132 00133 xsize = xsize + win->xpvmin + x1; 00134 ysize = ysize + win->ypvmin + y1; 00135 00136 /* Move image to window pixel by pixel */ 00137 while ((y <= win->ypvmax) && (y < ysize)) { 00138 /* Set physical frame buffer address */ 00139 x = win->xpvmin + x1; 00140 00141 /* Render a single line */ 00142 while ((x <= win->xpvmax) && (x < xsize)) { 00143 if (*image != tColor) { 00144 swim_put_pixel_physical(win, x, y, *image); 00145 } 00146 image++; 00147 x++; 00148 } 00149 00150 /* Adjust to end of line if the image was clipped */ 00151 image = image + (xsize - x); 00152 00153 y++; 00154 } 00155 } 00156 00157 /* Puts a raw image into a window inverted */ 00158 void swim_put_invert_image(SWIM_WINDOW_T *win, 00159 const COLOR_T *image, 00160 int32_t xsize, 00161 int32_t ysize) 00162 { 00163 int32_t x, y, xr, yr; 00164 00165 y = win->ypvmin; 00166 yr = ysize - 1; 00167 00168 /* Move image to window pixel by pixel */ 00169 while ((y <= win->ypvmax) && (yr >= 0)) { 00170 /* Set physical frame buffer address */ 00171 x = win->xpvmin; 00172 xr = xsize - 1; 00173 00174 /* Render a single line */ 00175 while ((x <= win->xpvmax) && (xr >= 0)) { 00176 swim_put_pixel_physical(win, x, y, image[xr + yr * xsize] ); 00177 x++; 00178 xr--; 00179 } 00180 00181 y++; 00182 yr--; 00183 } 00184 } 00185 00186 /* Puts a raw image into a window rotated left */ 00187 void swim_put_left_image(SWIM_WINDOW_T *win, 00188 const COLOR_T *image, 00189 int32_t xsize, 00190 int32_t ysize) 00191 { 00192 int32_t x, y, xr, yr; 00193 00194 x = win->xpvmin; 00195 yr = ysize - 1; 00196 00197 /* Move image to window pixel by pixel */ 00198 while ((x <= win->xpvmax) && (yr >= 0)) { 00199 /* Set physical frame buffer address to start drawing at 00200 bottom */ 00201 y = win->ypvmin; 00202 xr = 0; 00203 00204 /* Render a single line */ 00205 while ((y <= win->ypvmax) && (xr < xsize)) { 00206 /* Go to next line (y) */ 00207 swim_put_pixel_physical(win, x, y, 00208 image[(xsize - xr - 1) + (ysize - yr - 1) * xsize]); 00209 00210 /* Update picture to next x coordinate */ 00211 y++; 00212 xr++; 00213 } 00214 00215 x++; 00216 yr--; 00217 } 00218 } 00219 00220 /* Puts a raw image into a window rotated right */ 00221 void swim_put_right_image(SWIM_WINDOW_T *win, 00222 const COLOR_T *image, 00223 int32_t xsize, 00224 int32_t ysize) 00225 { 00226 int32_t x, y, xr, yr; 00227 00228 x = win->xpvmin; 00229 yr = ysize - 1; 00230 00231 /* Move image to window pixel by pixel */ 00232 while ((x <= win->xpvmax) && (yr >= 0)) { 00233 /* Set physical frame buffer address to start drawing at bottom */ 00234 y = win->ypvmin; 00235 xr = 0; 00236 00237 /* Render a single line */ 00238 while ((y <= win->ypvmax) && (xr < xsize)) { 00239 /* Go to next line (y) */ 00240 swim_put_pixel_physical(win, x, y, image[xr + yr * xsize]); 00241 00242 /* Update picture to next x coordinate */ 00243 y++; 00244 xr++; 00245 } 00246 00247 x++; 00248 yr--; 00249 } 00250 } 00251 00252 /* Puts and scales a raw image into a window */ 00253 void swim_put_scale_image(SWIM_WINDOW_T *win, 00254 const COLOR_T *image, 00255 int32_t xsize, 00256 int32_t ysize) 00257 { 00258 int32_t xsc, ysc; 00259 int32_t x, y; 00260 00261 /* Top of window */ 00262 y = win->ypvmin; 00263 00264 /* Rescale image into window */ 00265 while (y <= win->ypvmax) { 00266 x = win->xpvmin; 00267 00268 /* Scale he display size to the image size */ 00269 ysc = ((ysize - 1) * (y - win->ypvmin)) / win->yvsize; 00270 00271 /* Render a single line */ 00272 while (x <= win->xpvmax) { 00273 /* Get x pixel in image */ 00274 xsc = ((xsize - 1) * (x - win->xpvmin)) / win->xvsize; 00275 swim_put_pixel_physical(win, x, y, image[xsc + ysc * xsize] ); 00276 x++; 00277 } 00278 00279 y++; 00280 } 00281 } 00282 00283 /* Puts and scales a raw image into a window inverted */ 00284 void swim_put_scale_invert_image(SWIM_WINDOW_T *win, 00285 const COLOR_T *image, 00286 int32_t xsize, 00287 int32_t ysize) 00288 { 00289 int32_t xsc, ysc; 00290 int32_t x, y; 00291 00292 /* Top of window */ 00293 y = win->ypvmin; 00294 00295 /* Rescale image into window */ 00296 while (y <= win->ypvmax) { 00297 x = win->xpvmin; 00298 00299 /* Scale he display size to the image size */ 00300 ysc = ((ysize - 1) * (y - win->ypvmin)) / win->yvsize; 00301 00302 /* Render a single line */ 00303 while (x <= win->xpvmax) { 00304 /* Get x pixel in image */ 00305 xsc = ((xsize - 1) * (x - win->xpvmin)) / win->xvsize; 00306 swim_put_pixel_physical(win, x, y, 00307 image[(xsize - 1 - xsc) + (ysize - 1 - ysc) * xsize]); 00308 x++; 00309 } 00310 00311 y++; 00312 } 00313 } 00314 00315 /* Puts and scales a raw image into a window rotated left */ 00316 void swim_put_scale_left_image(SWIM_WINDOW_T *win, 00317 const COLOR_T *image, 00318 int32_t xsize, 00319 int32_t ysize) 00320 { 00321 int32_t xsc, ysc; 00322 int32_t x, y; 00323 00324 /* Top of window */ 00325 y = win->ypvmin; 00326 00327 /* Rescale image into window */ 00328 while (y <= win->ypvmax) { 00329 x = win->xpvmin; 00330 00331 /* Scale y coords of picture into x axis */ 00332 ysc = ((xsize - 1) * (win->ypvmax - y)) / win->yvsize; 00333 00334 /* Render a single horizontal line with 'y' data */ 00335 while (x <= win->xpvmax) { 00336 /* Get x pixel in image */ 00337 xsc = ((ysize - 1) * (x - win->xpvmin)) / win->xvsize; 00338 swim_put_pixel_physical(win, x, y, image[ysc + xsc * xsize] ); 00339 x++; 00340 } 00341 00342 y++; 00343 } 00344 } 00345 00346 /* Puts and scales a raw image into a window rotated right */ 00347 void swim_put_scale_right_image(SWIM_WINDOW_T *win, 00348 const COLOR_T *image, 00349 int32_t xsize, 00350 int32_t ysize) 00351 { 00352 int32_t xsc, ysc; 00353 int32_t x, y; 00354 00355 /* Top of window */ 00356 y = win->ypvmin; 00357 00358 /* Rescale image into window */ 00359 while (y <= win->ypvmax) { 00360 x = win->xpvmin; 00361 00362 /* Scale y coords of picture into x axis */ 00363 ysc = ((xsize - 1) * (y - win->ypvmin)) / win->yvsize; 00364 00365 /* Render a single horizontal line with 'y' data */ 00366 while (x <= win->xpvmax) { 00367 /* Get x pixel in image */ 00368 xsc = ((ysize - 1) * (win->xpvmax - x)) / win->xvsize; 00369 swim_put_pixel_physical(win, x, y, image[ysc + xsc * xsize]); 00370 x++; 00371 } 00372 00373 y++; 00374 } 00375 } 00376 00377 /* SWIM image draw composite function */ 00378 void swim_put_win_image(SWIM_WINDOW_T *win, 00379 const COLOR_T *image, 00380 int32_t xsize, 00381 int32_t ysize, 00382 int32_t scale, 00383 SWIM_ROTATION_T rtype) 00384 { 00385 switch (rtype) { 00386 case INVERT: 00387 if (scale != 0) { 00388 swim_put_scale_invert_image(win, image, xsize, ysize); 00389 } 00390 else { 00391 swim_put_invert_image(win, image, xsize, ysize); 00392 } 00393 break; 00394 00395 case LEFT: 00396 if (scale != 0) { 00397 swim_put_scale_left_image(win, image, xsize, ysize); 00398 } 00399 else { 00400 swim_put_left_image(win, image, xsize, ysize); 00401 } 00402 break; 00403 00404 case RIGHT: 00405 if (scale != 0) { 00406 swim_put_scale_right_image(win, image, xsize, ysize); 00407 } 00408 else { 00409 swim_put_right_image(win, image, xsize, ysize); 00410 } 00411 break; 00412 00413 case NOROTATION: 00414 default: 00415 if (scale != 0) { 00416 swim_put_scale_image(win, image, xsize, ysize); 00417 } 00418 else { 00419 swim_put_image(win, image, xsize, ysize); 00420 } 00421 break; 00422 } 00423 } 00424
Generated on Tue Jul 12 2022 21:27:04 by
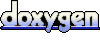