A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
SlideShow.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef SLIDESHOW_H 00018 #define SLIDESHOW_H 00019 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 #include "Image.h" 00023 #include "Renderer.h" 00024 00025 /** 00026 * SlideShow engine. 00027 * 00028 * For information on how to use the SlideShow and some examples see 00029 * https://developer.mbed.org/teams/Embedded-Artists/wiki/LPC4088DM-Using-the-SlideShow-Engine 00030 * 00031 * @code 00032 * #include "mbed.h" 00033 * #include "SlideShow.h" 00034 * #include "Renderer.h" 00035 * 00036 * static void tRender(void const *args) 00037 * { 00038 * Renderer* s = (Renderer*)args; 00039 * s->render(); 00040 * } 00041 * 00042 * int main(void) { 00043 * // initialize the display 00044 * ... 00045 * 00046 * // create the renderer that will handle the display 00047 * Renderer r; 00048 * 00049 * // create the slideshow 00050 * SlideShow s(&r); 00051 * 00052 * // parse and validate the script 00053 * SlideShow::SlideShowError err = s.prepare("/mci/myscript.txt"); 00054 * if (err != SlideShow::Ok) { 00055 * // handle error here 00056 * } 00057 * 00058 * // Create the thread to handle the display 00059 * Thread tr(tRender, &r, osPriorityHigh); 00060 * 00061 * // Run the slideshow 00062 * s.run(); 00063 * } 00064 * @endcode 00065 */ 00066 class SlideShow { 00067 public: 00068 00069 enum SlideShowError { 00070 Ok, 00071 InvalidScript, 00072 RuntimeError, 00073 UserAbort, 00074 ScriptEnd, 00075 OutOfMemory, 00076 FileError, 00077 }; 00078 00079 /** A function that the script can call during execution 00080 * 00081 * @param calloutId identifier given in setCalloutHandler() 00082 * @param ss the slideshow instance 00083 * @param identifier parameter to the "callout" tag in the script 00084 * 00085 * @returns 00086 * Ok on success 00087 * One of the SlideShowError error codes on failure 00088 */ 00089 typedef SlideShowError (*calloutFunc)(int calloutId, SlideShow* ss, int identifier); 00090 00091 /** Create a new slideshow 00092 * 00093 * @param r the renderer 00094 * @param pathPrefix optional path to prepend to all paths in the script file 00095 * @param xoff optional x coordinate to draw the slideshow at, default is 0 00096 * @param yoff optional y coordinate to draw the slideshow at, default is 0 00097 * @param layer optional z-order, higher layers are drawn on top of lower layers 00098 * @param fileMutex optional mutex to prevent simultaneous access of the file system 00099 */ 00100 SlideShow(Renderer* r, const char* pathPrefix=NULL, int xoff=0, int yoff=0, int layer=0, Mutex* fileMutex=NULL); 00101 ~SlideShow(); 00102 00103 /** Parses the script file and prepares internal structures 00104 * 00105 * @param scriptFile the script with the slideshow 00106 * 00107 * @returns 00108 * Ok on success 00109 * One of the SlideShowError error codes on failure 00110 */ 00111 SlideShowError prepare(const char* scriptFile); 00112 00113 /** Run the slideshow 00114 * 00115 * This function will run until the script ends. If the script has 00116 * an eternal loop then this function will never return. 00117 * 00118 * @returns 00119 * Ok on success 00120 * One of the SlideShowError error codes on failure 00121 */ 00122 SlideShowError run(); 00123 00124 /** Register a function that the script can call. 00125 * 00126 * A callout function allows the use of the "callout" tag in a 00127 * slideshow script to e.g. notify the system or to trigger 00128 * something. 00129 * 00130 * There can only be one callout function. 00131 */ 00132 void setCalloutHandler(calloutFunc func, int calloutId); 00133 00134 /** Decouples this SlideShow from the Renderer 00135 */ 00136 void releaseScreen(void); 00137 00138 /** Stops the SlideShow. 00139 * 00140 * The SlideShow will be stopped at the next suitable time, 00141 * typically before processing the next step in the script. 00142 * 00143 * This function is typically called upon an external trigger 00144 * like the user pressing a button to end the slideshow. 00145 */ 00146 void stop() { abortBeforeNextStep = true; } 00147 00148 private: 00149 00150 typedef uint16_t* image_t; 00151 00152 class Transition { 00153 public: 00154 typedef enum 00155 { 00156 None, 00157 LeftRight, 00158 DownUp, 00159 TopDown, 00160 Blinds, 00161 Fade, 00162 Unknown, 00163 } Type; 00164 00165 Transition(const char* typeStr) { 00166 if (typeStr == NULL) { 00167 t = Unknown; 00168 } else if (strcmp("none", typeStr) == 0) { 00169 t = None; 00170 } else if (strcmp("left-right", typeStr) == 0) { 00171 t = LeftRight; 00172 } else if (strcmp("down-up", typeStr) == 0) { 00173 t = DownUp; 00174 } else if (strcmp("top-down", typeStr) == 0) { 00175 t = TopDown; 00176 } else if (strcmp("blinds", typeStr) == 0) { 00177 t = Blinds; 00178 } else if (strcmp("fade", typeStr) == 0) { 00179 t = Fade; 00180 } else { 00181 t = Unknown; 00182 } 00183 }; 00184 ~Transition(); 00185 SlideShowError execute(SlideShow* ss, Image::ImageData_t* CurrentImage, Image::ImageData_t* NewImage); 00186 Type type() { return this->t; } 00187 const char* typeString() { 00188 switch(t) { 00189 case None: return "No"; 00190 case LeftRight: return "Left to Right"; 00191 case TopDown: return "Top to Bottom"; 00192 case Blinds: return "Blinds"; 00193 case Unknown: 00194 default: 00195 return "Unknown"; 00196 } 00197 }; 00198 00199 private: 00200 Type t; 00201 00202 enum Constants { 00203 LeftRight_DelayMs = 100, 00204 LeftRight_PixelsToSkip = 20, 00205 DownUp_DelayMs = 100, 00206 DownUp_LineSkip = 20, 00207 TopDown_DelayMs = 100, 00208 TopDown_LineSkip = 20, 00209 Blinds_LinesPerBlock = 8, 00210 Blinds_DelayMs = 20, 00211 Blinds_BeamColor = 0xffff, 00212 Blinds_BackColor = 0x0000, 00213 Fade_DelayMs = 80, 00214 }; 00215 }; 00216 00217 class Command { 00218 public: 00219 typedef enum 00220 { 00221 Clear, 00222 Goto, 00223 LoadImage, 00224 Show, 00225 Wait, 00226 Callout, 00227 } Type; 00228 00229 Command(Type cmd, int info=0, Transition* t=NULL, const char* filename=NULL, const char* prefix=NULL) { 00230 type = cmd; 00231 information = info; 00232 transition = t; 00233 if (filename == NULL) { 00234 fname = NULL; 00235 } else { 00236 fname = (char*)malloc(strlen(filename) + strlen(prefix) + 1); //+1 for null termination 00237 if (fname != NULL) { 00238 fname[0] = '\0'; 00239 strcat(fname, prefix); 00240 strcat(fname, filename); 00241 } 00242 } 00243 }; 00244 ~Command() { 00245 if (fname != NULL) { 00246 free(fname); 00247 fname = NULL; 00248 } 00249 }; 00250 void updateInfo(int newInfo) { information = newInfo; } 00251 int info() { return information; } 00252 void print(); 00253 SlideShowError handle(SlideShow* ss, int* seqIdx, int* lastTime); 00254 private: 00255 Type type; 00256 Transition* transition; 00257 int information; 00258 char* fname; 00259 }; 00260 00261 00262 typedef enum 00263 { 00264 Bmp, 00265 Raw 00266 } FileFormat; 00267 00268 typedef struct 00269 { 00270 const char* pLabel; 00271 int index; 00272 } LabelInfo; 00273 00274 enum { 00275 MaxNumPreparedImages = 100, 00276 } Constants; 00277 00278 int screenWidth; 00279 int screenHeight; 00280 int screenPixels; 00281 int screenBytes; 00282 int drawXoff; 00283 int drawYoff; 00284 00285 image_t ImageBackBuffer; 00286 Command** Sequence; 00287 int allocatedSequenceItems; 00288 int usedSequenceItems; 00289 00290 const char* pathPrefix; 00291 00292 int CurrentSlot; 00293 Image::ImageData_t PreparedImages[MaxNumPreparedImages]; 00294 00295 Mutex* fileMutex; 00296 00297 Renderer* rend; 00298 uint32_t rendHnd; 00299 int layer; 00300 00301 calloutFunc callout; 00302 int calloutId; 00303 00304 bool abortBeforeNextStep; 00305 00306 // Utilities 00307 SlideShowError loadFile(const char* path, uint8_t** pData, uint32_t* pSize); 00308 00309 // Parsing functions 00310 int getNextLine(char* pBuf, int* pOffset, char** ppLine); 00311 int splitLine(char* pLine, char** ppPart1, char** ppPart2, char** ppPart3); 00312 int findLabel(LabelInfo* pLabels, int numLabels, const char* pLabel); 00313 void freeSequence(void); 00314 SlideShowError expandSequence(); 00315 SlideShowError parseScript(char* pBuf); 00316 00317 // Command related functions 00318 SlideShowError loadImage(const char* pFileName, int slot); 00319 void delay(int lastTime, int millis); 00320 SlideShowError runScript(); 00321 00322 }; 00323 00324 #endif
Generated on Tue Jul 12 2022 21:27:04 by
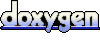