A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
App.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef APP_H 00018 #define APP_H 00019 00020 /** 00021 * Base class for all Apps. The idea is that you can have multiple 00022 * Apps in your program. Each App has three funtions: 00023 * 00024 * setup() is called to let the App load all needed resources 00025 * 00026 * runToCompletion() is called and will not return until the App exits 00027 * 00028 * teardown() is called to let the App free allocated resources 00029 * 00030 * For an example of Apps in use, see the AppLauncher class 00031 */ 00032 class App { 00033 public: 00034 virtual ~App() {}; 00035 00036 /** Prepare the App before it is started. 00037 * 00038 * This function can be implemented to allocate memory, load startup 00039 * images or other time consuming preparations. 00040 * 00041 * The return value is to let the caller now if the application can 00042 * be started or not. 00043 * 00044 * @param x the touched x coordinate 00045 * @param y the touched y coordinate 00046 * @param pressed true if the user pressed the display 00047 * 00048 * @returns 00049 * true if the app can be started 00050 * false if the setup failed 00051 */ 00052 virtual bool setup() { return true; } 00053 00054 /** Runs the App to completion 00055 * 00056 * This function should not return until the App has finished. 00057 */ 00058 virtual void runToCompletion() = 0; 00059 00060 /** Cleanup 00061 * 00062 * Implement to free up the memory allocated in setup(). 00063 * 00064 * @returns 00065 * true if the teardown finished successfully 00066 * false if the teardown failed 00067 */ 00068 virtual bool teardown() { return true; } 00069 }; 00070 00071 #endif
Generated on Tue Jul 12 2022 21:27:03 by
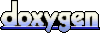