adaptation for book and plug demo
Fork of BLE_API by
GapAdvertisingParams Class Reference
This class provides a wrapper for the core advertising parameters, including the advertising type (Connectable Undirected, Non Connectable Undirected and so on), as well as the advertising and timeout intervals. More...
#include <GapAdvertisingParams.h>
Public Types | |
enum | AdvertisingType_t { ADV_CONNECTABLE_UNDIRECTED, ADV_CONNECTABLE_DIRECTED, ADV_SCANNABLE_UNDIRECTED, ADV_NON_CONNECTABLE_UNDIRECTED } |
Encapsulates the peripheral advertising modes, which determine how the device appears to other central devices in hearing range. More... | |
typedef enum AdvertisingType_t | AdvertisingType |
Type alias for GapAdvertisingParams::AdvertisingType_t. | |
Public Member Functions | |
GapAdvertisingParams (AdvertisingType_t advType=ADV_CONNECTABLE_UNDIRECTED, uint16_t interval=GAP_ADV_PARAMS_INTERVAL_MIN_NONCON, uint16_t timeout=0) | |
Construct an instance of GapAdvertisingParams. | |
AdvertisingType_t | getAdvertisingType (void) const |
Get the advertising type. | |
uint16_t | getInterval (void) const |
Get the advertising interval in milliseconds. | |
uint16_t | getIntervalInADVUnits (void) const |
Get the advertisement interval in units of 0.625ms. | |
uint16_t | getTimeout (void) const |
Get The advertising timeout. | |
void | setAdvertisingType (AdvertisingType_t newAdvType) |
Set the advertising type. | |
void | setInterval (uint16_t newInterval) |
Set the advertising interval in milliseconds. | |
void | setTimeout (uint16_t newTimeout) |
Set the advertising timeout. | |
Static Public Member Functions | |
static uint16_t | MSEC_TO_ADVERTISEMENT_DURATION_UNITS (uint32_t durationInMillis) |
Convert milliseconds to units of 0.625ms. | |
static uint16_t | ADVERTISEMENT_DURATION_UNITS_TO_MS (uint16_t gapUnits) |
Convert units of 0.625ms to milliseconds. | |
Static Public Attributes | |
static const unsigned | GAP_ADV_PARAMS_INTERVAL_MIN = 0x0020 |
Minimum Advertising interval for connectable undirected and connectable directed events in 625us units - 20ms. | |
static const unsigned | GAP_ADV_PARAMS_INTERVAL_MIN_NONCON = 0x00A0 |
Minimum Advertising interval for scannable and non-connectable undirected events in 625us units - 100ms. | |
static const unsigned | GAP_ADV_PARAMS_INTERVAL_MAX = 0x4000 |
Maximum Advertising interval in 625us units - 10.24s. | |
static const unsigned | GAP_ADV_PARAMS_TIMEOUT_MAX = 0x3FFF |
Maximum advertising timeout seconds. | |
static const uint16_t | UNIT_0_625_MS = 625 |
Number of microseconds in 0.625 milliseconds. |
Detailed Description
This class provides a wrapper for the core advertising parameters, including the advertising type (Connectable Undirected, Non Connectable Undirected and so on), as well as the advertising and timeout intervals.
Definition at line 26 of file GapAdvertisingParams.h.
Member Typedef Documentation
typedef enum AdvertisingType_t AdvertisingType |
Type alias for GapAdvertisingParams::AdvertisingType_t.
Definition at line 62 of file GapAdvertisingParams.h.
Member Enumeration Documentation
enum AdvertisingType_t |
Encapsulates the peripheral advertising modes, which determine how the device appears to other central devices in hearing range.
- Enumerator:
Definition at line 51 of file GapAdvertisingParams.h.
Constructor & Destructor Documentation
GapAdvertisingParams | ( | AdvertisingType_t | advType = ADV_CONNECTABLE_UNDIRECTED , |
uint16_t | interval = GAP_ADV_PARAMS_INTERVAL_MIN_NONCON , |
||
uint16_t | timeout = 0 |
||
) |
Construct an instance of GapAdvertisingParams.
- Parameters:
-
[in] advType Type of advertising. Default is GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED. [in] interval Advertising interval in units of 0.625ms. Default is GapAdvertisingParams::GAP_ADV_PARAMS_INTERVAL_MIN_NONCON. [in] timeout Advertising timeout. Default is 0.
Definition at line 77 of file GapAdvertisingParams.h.
Member Function Documentation
static uint16_t ADVERTISEMENT_DURATION_UNITS_TO_MS | ( | uint16_t | gapUnits ) | [static] |
Convert units of 0.625ms to milliseconds.
- Parameters:
-
[in] gapUnits The number of units of 0.625ms to convert.
- Returns:
- The value of
gapUnits
in milliseconds.
Definition at line 131 of file GapAdvertisingParams.h.
AdvertisingType_t getAdvertisingType | ( | void | ) | const |
Get the advertising type.
- Returns:
- The advertising type.
Definition at line 140 of file GapAdvertisingParams.h.
uint16_t getInterval | ( | void | ) | const |
Get the advertising interval in milliseconds.
- Returns:
- The advertisement interval (in milliseconds).
Definition at line 149 of file GapAdvertisingParams.h.
uint16_t getIntervalInADVUnits | ( | void | ) | const |
Get the advertisement interval in units of 0.625ms.
- Returns:
- The advertisement interval in advertisement duration units (0.625ms units).
Definition at line 158 of file GapAdvertisingParams.h.
uint16_t getTimeout | ( | void | ) | const |
Get The advertising timeout.
- Returns:
- The advertising timeout (in seconds).
Definition at line 167 of file GapAdvertisingParams.h.
static uint16_t MSEC_TO_ADVERTISEMENT_DURATION_UNITS | ( | uint32_t | durationInMillis ) | [static] |
Convert milliseconds to units of 0.625ms.
- Parameters:
-
[in] durationInMillis The number of milliseconds to convert.
- Returns:
- The value of
durationInMillis
in units of 0.625ms.
Definition at line 120 of file GapAdvertisingParams.h.
void setAdvertisingType | ( | AdvertisingType_t | newAdvType ) |
Set the advertising type.
- Parameters:
-
[in] newAdvType The new advertising type.
Definition at line 177 of file GapAdvertisingParams.h.
void setInterval | ( | uint16_t | newInterval ) |
Set the advertising interval in milliseconds.
- Parameters:
-
[in] newInterval The new advertising interval in milliseconds.
Definition at line 187 of file GapAdvertisingParams.h.
void setTimeout | ( | uint16_t | newTimeout ) |
Set the advertising timeout.
- Parameters:
-
[in] newTimeout The new advertising timeout (in seconds).
Definition at line 197 of file GapAdvertisingParams.h.
Field Documentation
const unsigned GAP_ADV_PARAMS_INTERVAL_MAX = 0x4000 [static] |
Maximum Advertising interval in 625us units - 10.24s.
Definition at line 41 of file GapAdvertisingParams.h.
const unsigned GAP_ADV_PARAMS_INTERVAL_MIN = 0x0020 [static] |
Minimum Advertising interval for connectable undirected and connectable directed events in 625us units - 20ms.
Definition at line 32 of file GapAdvertisingParams.h.
const unsigned GAP_ADV_PARAMS_INTERVAL_MIN_NONCON = 0x00A0 [static] |
Minimum Advertising interval for scannable and non-connectable undirected events in 625us units - 100ms.
Definition at line 37 of file GapAdvertisingParams.h.
const unsigned GAP_ADV_PARAMS_TIMEOUT_MAX = 0x3FFF [static] |
Maximum advertising timeout seconds.
Definition at line 45 of file GapAdvertisingParams.h.
const uint16_t UNIT_0_625_MS = 625 [static] |
Number of microseconds in 0.625 milliseconds.
Definition at line 111 of file GapAdvertisingParams.h.
Generated on Wed Jul 13 2022 09:31:10 by
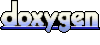