adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
GapAdvertisingParams.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GAP_ADVERTISING_PARAMS_H__ 00018 #define __GAP_ADVERTISING_PARAMS_H__ 00019 00020 /** 00021 * This class provides a wrapper for the core advertising parameters, 00022 * including the advertising type (Connectable Undirected, 00023 * Non Connectable Undirected and so on), as well as the advertising and 00024 * timeout intervals. 00025 */ 00026 class GapAdvertisingParams { 00027 public: 00028 /** 00029 * Minimum Advertising interval for connectable undirected and connectable 00030 * directed events in 625us units - 20ms. 00031 */ 00032 static const unsigned GAP_ADV_PARAMS_INTERVAL_MIN = 0x0020; 00033 /** 00034 * Minimum Advertising interval for scannable and non-connectable 00035 * undirected events in 625us units - 100ms. 00036 */ 00037 static const unsigned GAP_ADV_PARAMS_INTERVAL_MIN_NONCON = 0x00A0; 00038 /** 00039 * Maximum Advertising interval in 625us units - 10.24s. 00040 */ 00041 static const unsigned GAP_ADV_PARAMS_INTERVAL_MAX = 0x4000; 00042 /** 00043 * Maximum advertising timeout seconds. 00044 */ 00045 static const unsigned GAP_ADV_PARAMS_TIMEOUT_MAX = 0x3FFF; 00046 00047 /** 00048 * Encapsulates the peripheral advertising modes, which determine how 00049 * the device appears to other central devices in hearing range. 00050 */ 00051 enum AdvertisingType_t { 00052 ADV_CONNECTABLE_UNDIRECTED, /**< Vol 3, Part C, Section 9.3.4 and Vol 6, Part B, Section 2.3.1.1. */ 00053 ADV_CONNECTABLE_DIRECTED, /**< Vol 3, Part C, Section 9.3.3 and Vol 6, Part B, Section 2.3.1.2. */ 00054 ADV_SCANNABLE_UNDIRECTED, /**< Include support for Scan Response payloads, see Vol 6, Part B, Section 2.3.1.4. */ 00055 ADV_NON_CONNECTABLE_UNDIRECTED /**< Vol 3, Part C, Section 9.3.2 and Vol 6, Part B, Section 2.3.1.3. */ 00056 }; 00057 /** 00058 * Type alias for GapAdvertisingParams::AdvertisingType_t. 00059 * 00060 * @deprecated This type alias will be dropped in future releases. 00061 */ 00062 typedef enum AdvertisingType_t AdvertisingType; 00063 00064 public: 00065 /** 00066 * Construct an instance of GapAdvertisingParams. 00067 * 00068 * @param[in] advType 00069 * Type of advertising. Default is 00070 * GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED. 00071 * @param[in] interval 00072 * Advertising interval in units of 0.625ms. Default is 00073 * GapAdvertisingParams::GAP_ADV_PARAMS_INTERVAL_MIN_NONCON. 00074 * @param[in] timeout 00075 * Advertising timeout. Default is 0. 00076 */ 00077 GapAdvertisingParams(AdvertisingType_t advType = ADV_CONNECTABLE_UNDIRECTED, 00078 uint16_t interval = GAP_ADV_PARAMS_INTERVAL_MIN_NONCON, 00079 uint16_t timeout = 0) : _advType(advType), _interval(interval), _timeout(timeout) { 00080 /* Interval checks. */ 00081 if (_advType == ADV_CONNECTABLE_DIRECTED) { 00082 /* Interval must be 0 in directed connectable mode. */ 00083 _interval = 0; 00084 } else if (_advType == ADV_NON_CONNECTABLE_UNDIRECTED) { 00085 /* Min interval is slightly larger than in other modes. */ 00086 if (_interval < GAP_ADV_PARAMS_INTERVAL_MIN_NONCON) { 00087 _interval = GAP_ADV_PARAMS_INTERVAL_MIN_NONCON; 00088 } 00089 if (_interval > GAP_ADV_PARAMS_INTERVAL_MAX) { 00090 _interval = GAP_ADV_PARAMS_INTERVAL_MAX; 00091 } 00092 } else { 00093 /* Stay within interval limits. */ 00094 if (_interval < GAP_ADV_PARAMS_INTERVAL_MIN) { 00095 _interval = GAP_ADV_PARAMS_INTERVAL_MIN; 00096 } 00097 if (_interval > GAP_ADV_PARAMS_INTERVAL_MAX) { 00098 _interval = GAP_ADV_PARAMS_INTERVAL_MAX; 00099 } 00100 } 00101 00102 /* Timeout checks. */ 00103 if (timeout) { 00104 /* Stay within timeout limits. */ 00105 if (_timeout > GAP_ADV_PARAMS_TIMEOUT_MAX) { 00106 _timeout = GAP_ADV_PARAMS_TIMEOUT_MAX; 00107 } 00108 } 00109 } 00110 00111 static const uint16_t UNIT_0_625_MS = 625; /**< Number of microseconds in 0.625 milliseconds. */ 00112 /** 00113 * Convert milliseconds to units of 0.625ms. 00114 * 00115 * @param[in] durationInMillis 00116 * The number of milliseconds to convert. 00117 * 00118 * @return The value of @p durationInMillis in units of 0.625ms. 00119 */ 00120 static uint16_t MSEC_TO_ADVERTISEMENT_DURATION_UNITS(uint32_t durationInMillis) { 00121 return (durationInMillis * 1000) / UNIT_0_625_MS; 00122 } 00123 /** 00124 * Convert units of 0.625ms to milliseconds. 00125 * 00126 * @param[in] gapUnits 00127 * The number of units of 0.625ms to convert. 00128 * 00129 * @return The value of @p gapUnits in milliseconds. 00130 */ 00131 static uint16_t ADVERTISEMENT_DURATION_UNITS_TO_MS(uint16_t gapUnits) { 00132 return (gapUnits * UNIT_0_625_MS) / 1000; 00133 } 00134 00135 /** 00136 * Get the advertising type. 00137 * 00138 * @return The advertising type. 00139 */ 00140 AdvertisingType_t getAdvertisingType(void) const { 00141 return _advType; 00142 } 00143 00144 /** 00145 * Get the advertising interval in milliseconds. 00146 * 00147 * @return The advertisement interval (in milliseconds). 00148 */ 00149 uint16_t getInterval(void) const { 00150 return ADVERTISEMENT_DURATION_UNITS_TO_MS(_interval); 00151 } 00152 00153 /** 00154 * Get the advertisement interval in units of 0.625ms. 00155 * 00156 * @return The advertisement interval in advertisement duration units (0.625ms units). 00157 */ 00158 uint16_t getIntervalInADVUnits(void) const { 00159 return _interval; 00160 } 00161 00162 /** 00163 * Get The advertising timeout. 00164 * 00165 * @return The advertising timeout (in seconds). 00166 */ 00167 uint16_t getTimeout(void) const { 00168 return _timeout; 00169 } 00170 00171 /** 00172 * Set the advertising type. 00173 * 00174 * @param[in] newAdvType 00175 * The new advertising type. 00176 */ 00177 void setAdvertisingType(AdvertisingType_t newAdvType) { 00178 _advType = newAdvType; 00179 } 00180 00181 /** 00182 * Set the advertising interval in milliseconds. 00183 * 00184 * @param[in] newInterval 00185 * The new advertising interval in milliseconds. 00186 */ 00187 void setInterval(uint16_t newInterval) { 00188 _interval = MSEC_TO_ADVERTISEMENT_DURATION_UNITS(newInterval); 00189 } 00190 00191 /** 00192 * Set the advertising timeout. 00193 * 00194 * @param[in] newTimeout 00195 * The new advertising timeout (in seconds). 00196 */ 00197 void setTimeout(uint16_t newTimeout) { 00198 _timeout = newTimeout; 00199 } 00200 00201 private: 00202 AdvertisingType_t _advType; /**< The advertising type. */ 00203 uint16_t _interval; /**< The advertising interval in ADV duration units (i.e. 0.625ms). */ 00204 uint16_t _timeout; /**< The advertising timeout in seconds. */ 00205 }; 00206 00207 #endif /* ifndef __GAP_ADVERTISING_PARAMS_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
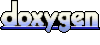