Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
WNCUDPSocket Class Reference
#include <WNCUDPSocket.h>
Inherits WNCSocket, and WNCInterface.
Public Member Functions | |
int | sendTo (WNCEndpoint &remote, char *packet, int length) |
sendTo - send data to the remote host. | |
int | receiveFrom (WNCEndpoint &remote, char *buffer, int length) |
receiveFrom - receive data from the remote host. | |
void | set_blocking (bool blocking, unsigned int timeout=1500) |
Set blocking or non-blocking mode of the socket and a timeout. | |
int | close (void) |
Close the socket. | |
int | connect (void) |
Open an LTE internet data connection. | |
void | doDebug (int val) |
Manipulate the debug output of the WncController, for debug purposes. | |
void | getICCID (string *str) |
Returns full ICCD on the SIM that is being used. | |
Static Public Member Functions | |
static int | init (const char *apn=NULL, MODSERIAL *debug=NULL) |
Initialize the interface (no connection at this point). | |
static int | disconnect () |
Disconnect Bring the interface down. | |
static char * | getMACAddress () |
Because the WNCInterface is cellular based there is no MAC Ethernet address to return, so this function returns a bogus MAC address created from the ICCD on the SIM that is being used. | |
static char * | getIPAddress () |
Get the IP address of your Ethernet interface. | |
static char * | getGateway () |
Get the Gateway address of your Ethernet interface. | |
static char * | getNetworkMask () |
Get the Network mask of your Ethernet interface. |
Detailed Description
UDP WNCSocket.
Definition at line 35 of file WNCUDPSocket.h.
Member Function Documentation
int close | ( | void | ) |
Close the socket.
- Parameters:
-
none
- Returns:
- 0 if closed successfully, -1 on failure
Definition at line 44 of file WNCUDPSocket.cpp.
int connect | ( | void | ) | [inherited] |
Open an LTE internet data connection.
- Returns:
- 0 on success, error code on failure
Definition at line 120 of file WNCInterface.cpp.
int disconnect | ( | ) | [static, inherited] |
Disconnect Bring the interface down.
- Returns:
- 0 on success, a negative number on failure
Definition at line 128 of file WNCInterface.cpp.
void doDebug | ( | int | val ) | [inherited] |
Manipulate the debug output of the WncController, for debug purposes.
- Returns:
- nothing.
Definition at line 70 of file WNCInterface.cpp.
char * getGateway | ( | ) | [static, inherited] |
Get the Gateway address of your Ethernet interface.
- Returns:
- a pointer to a string containing the Gateway address
Definition at line 149 of file WNCInterface.cpp.
void getICCID | ( | string * | str ) | [inherited] |
Returns full ICCD on the SIM that is being used.
Definition at line 195 of file WNCInterface.cpp.
char * getIPAddress | ( | ) | [static, inherited] |
Get the IP address of your Ethernet interface.
- Returns:
- a pointer to a string containing the IP address
Definition at line 135 of file WNCInterface.cpp.
char * getMACAddress | ( | void | ) | [static, inherited] |
Because the WNCInterface is cellular based there is no MAC Ethernet address to return, so this function returns a bogus MAC address created from the ICCD on the SIM that is being used.
- Returns:
- a pointer to a pesudo-MAC string containing "NN:NN:NN:NN:NN:NN"
Definition at line 177 of file WNCInterface.cpp.
char * getNetworkMask | ( | ) | [static, inherited] |
Get the Network mask of your Ethernet interface.
- Returns:
- a pointer to a string containing the Network mask
Definition at line 163 of file WNCInterface.cpp.
int init | ( | const char * | apn = NULL , |
MODSERIAL * | debug = NULL |
||
) | [static, inherited] |
Initialize the interface (no connection at this point).
- Returns:
- 0 on success, a negative number on failure
Definition at line 90 of file WNCInterface.cpp.
int receiveFrom | ( | WNCEndpoint & | remote, |
char * | buffer, | ||
int | length | ||
) |
receiveFrom - receive data from the remote host.
- Parameters:
-
remote,a pointer to the endpoint (class) packet,The buffer in which to store the data received from the host. length The maximum length of the buffer.
- Returns:
- the number of received bytes on success (>=0) or -1 on failure
Definition at line 78 of file WNCUDPSocket.cpp.
int sendTo | ( | WNCEndpoint & | remote, |
char * | packet, | ||
int | length | ||
) |
sendTo - send data to the remote host.
- Parameters:
-
remote,a pointer to the endpoint (class) packet,pointer to the buffer to send to the host. length The length of the buffer to send.
- Returns:
- the number of written bytes on success (>=0) or -1 on failure
Definition at line 50 of file WNCUDPSocket.cpp.
void set_blocking | ( | bool | blocking, |
unsigned int | timeout = 1500 |
||
) |
Set blocking or non-blocking mode of the socket and a timeout.
- Parameters:
-
blocking true for blocking mode, false for non-blocking mode.
- Returns:
- none.
Reimplemented from WNCSocket.
Definition at line 68 of file WNCUDPSocket.cpp.
Generated on Tue Jul 12 2022 22:13:21 by
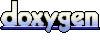