Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
WNCInterface.cpp
00001 /* ===================================================================== 00002 Copyright © 2016, Avnet (R) 00003 00004 Contributors: 00005 * James M Flynn, www.em.avnet.com 00006 00007 Licensed under the Apache License, Version 2.0 (the "License"); 00008 you may not use this file except in compliance with the License. 00009 You may obtain a copy of the License at 00010 00011 http://www.apache.org/licenses/LICENSE-2.0 00012 00013 Unless required by applicable law or agreed to in writing, 00014 software distributed under the License is distributed on an 00015 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00016 either express or implied. See the License for the specific 00017 language governing permissions and limitations under the License. 00018 00019 @file WNCInterface.cpp 00020 @version 1.0 00021 @date Sept 2016 00022 @author James Flynn 00023 00024 ======================================================================== */ 00025 00026 00027 #ifndef __MODULE__ 00028 #define __MODULE__ "WNCInterface.cpp" 00029 #endif 00030 00031 #include "WNCInterface.h" 00032 00033 ///////////////////////////////////////////////////// 00034 // NXP GPIO Pins that are used to initialize the WNC Shield 00035 ///////////////////////////////////////////////////// 00036 DigitalOut mdm_uart2_rx_boot_mode_sel(PTC17); // on powerup, 0 = boot mode, 1 = normal boot 00037 DigitalOut mdm_power_on(PTB9); // 0 = turn modem on, 1 = turn modem off (should be held high for >5 seconds to cycle modem) 00038 DigitalOut mdm_wakeup_in(PTC2); // 0 = let modem sleep, 1 = keep modem awake -- Note: pulled high on shield 00039 DigitalOut mdm_reset(PTC12); // active high 00040 DigitalOut shield_3v3_1v8_sig_trans_ena(PTC4); // 0 = disabled (all signals high impedence, 1 = translation active 00041 DigitalOut mdm_uart1_cts(PTD0); 00042 00043 char * _fatal_err_loc; //GLOBAL::holds any error location info 00044 MODSERIAL * _dbgout; 00045 Mutex _WNCLock; 00046 00047 using namespace WncControllerK64F_fk; // namespace for the controller class use 00048 00049 // Define pin associations for the controller class to use be careful to 00050 // keep the order of the pins in the initialization list. 00051 WncGpioPinListK64F wncPinList = { 00052 &mdm_uart2_rx_boot_mode_sel, 00053 &mdm_power_on, 00054 &mdm_wakeup_in, 00055 &mdm_reset, 00056 &shield_3v3_1v8_sig_trans_ena, 00057 &mdm_uart1_cts 00058 }; 00059 00060 static MODSERIAL mdmUart(PTD3,PTD2,256,4096); //UART for WNC Module 00061 00062 WncControllerK64F *WNCInterface::_pwnc; 00063 WncIpStats WNCInterface::myNetStats; 00064 string WNCInterface::mac; 00065 00066 WNCInterface::WNCInterface() { 00067 _dbgout = NULL; 00068 } 00069 00070 void WNCInterface::doDebug( int v ) { 00071 //basic debug = 0x01 00072 //more debug = 0x02 00073 //all debug = 0x03 00074 M_LOCK; 00075 _pwnc->enableDebug( (v&1), (v&2) ); 00076 M_ULOCK; 00077 } 00078 00079 // 00080 // Power-up the WNC module. The caller can optionally configure. 00081 // Inputs: 00082 // apn - Caller can specify an APN. If none is provided will use "m2m.com.attz" 00083 // debug- specify the amount of debug the WNC controller should output: 00084 // 1 - Basic Debug output 00085 // 2 - Verbose Debug output 00086 // 3 - Full Debug output 00087 // Returns: 0 if unable to initialize the WNC module 00088 // -1 if successfully initialized 00089 // 00090 int WNCInterface::init(const char* apn, MODSERIAL * debug) { 00091 int ret = 0; 00092 00093 M_LOCK; 00094 if( debug ) { 00095 _dbgout = debug; 00096 _pwnc = new WncControllerK64F_fk::WncControllerK64F::WncControllerK64F(&wncPinList, &mdmUart, debug); 00097 #if WNC_DEBUG == 1 00098 _pwnc->enableDebug(1,1); 00099 #endif 00100 } 00101 else 00102 _pwnc = new WncControllerK64F_fk::WncControllerK64F::WncControllerK64F(&wncPinList, &mdmUart, NULL); 00103 00104 if( apn==NULL ) 00105 apn = APN_DEFAULT; 00106 00107 ret = ( _pwnc->powerWncOn(apn,40) )? 2:0; 00108 ret |= ( _pwnc->setApnName(apn) )? 1:0; 00109 ret |= ( _pwnc->getWncNetworkingStats(&myNetStats) )? 4:0; 00110 M_ULOCK; 00111 00112 return ret; 00113 } 00114 00115 // 00116 // check to see if we are connected to the internet or not. The 00117 // connection is supposed to happen during init. If we are 00118 // connected to the internet return 0 otherwise return -1 00119 // 00120 int WNCInterface::connect(void) { 00121 return ( _pwnc->getWncStatus() == WNC_GOOD )? 0 : -1; 00122 } 00123 00124 // 00125 // ok, the user wants to disconnect. At present, this isn't possible 00126 // with the WNC, so just fake it and say we did... 00127 // 00128 int WNCInterface::disconnect() { 00129 return 0; 00130 } 00131 00132 // 00133 // update the networking stats and return the IP Address 00134 // 00135 char * WNCInterface::getIPAddress() { 00136 M_LOCK; 00137 if ( _pwnc->getWncNetworkingStats(&myNetStats) ) { 00138 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), null); 00139 M_ULOCK; 00140 return &myNetStats.ip[0]; 00141 } 00142 M_ULOCK; 00143 return NULL; 00144 } 00145 00146 // 00147 // update the networking stats and return the Gateway Address 00148 // 00149 char * WNCInterface::getGateway() { 00150 M_LOCK; 00151 if ( _pwnc->getWncNetworkingStats(&myNetStats) ) { 00152 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), null); 00153 M_ULOCK; 00154 return &WNCInterface::myNetStats.gateway[0]; 00155 } 00156 M_ULOCK; 00157 return NULL; 00158 } 00159 00160 // 00161 // update the networking stats and return the Network Mask 00162 // 00163 char * WNCInterface::getNetworkMask() { 00164 M_LOCK; 00165 if ( _pwnc->getWncNetworkingStats(&myNetStats) ) { 00166 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), null); 00167 M_ULOCK; 00168 return &WNCInterface::myNetStats.mask[0]; 00169 } 00170 M_ULOCK; 00171 return NULL; 00172 } 00173 00174 // 00175 // return a pesudo-MAC address created from the ICCID 00176 // 00177 char* WNCInterface::getMACAddress( void ) { 00178 string str; 00179 00180 M_LOCK; 00181 if( _pwnc->getICCID(&str) ) { 00182 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), null); 00183 mac = str.substr(3,20); 00184 mac[2]=mac[5]=mac[8]=mac[11]=mac[14]=':'; 00185 M_ULOCK; 00186 return (char*)mac.c_str(); 00187 } 00188 M_ULOCK; 00189 return NULL; 00190 } 00191 00192 // 00193 // return the ICCID 00194 // 00195 void WNCInterface::getICCID(string *str) { 00196 _pwnc->getICCID(str); 00197 } 00198 00199
Generated on Tue Jul 12 2022 22:13:21 by
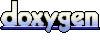