Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
WNCUDPSocket.cpp
00001 /* ===================================================================== 00002 Copyright © 2016, Avnet (R) 00003 00004 Contributors: 00005 * James M Flynn, www.em.avnet.com 00006 00007 Licensed under the Apache License, Version 2.0 (the "License"); 00008 you may not use this file except in compliance with the License. 00009 You may obtain a copy of the License at 00010 00011 http://www.apache.org/licenses/LICENSE-2.0 00012 00013 Unless required by applicable law or agreed to in writing, 00014 software distributed under the License is distributed on an 00015 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00016 either express or implied. See the License for the specific 00017 language governing permissions and limitations under the License. 00018 00019 @file WNCInterface.cpp 00020 @version 1.0 00021 @date Sept 2016 00022 00023 ======================================================================== */ 00024 00025 #include "../WNCInterface.h" 00026 00027 #include "WNCUDPSocket.h" 00028 #include <cstring> 00029 00030 WNCUDPSocket::WNCUDPSocket() : 00031 _is_blocking(0), 00032 _btimeout(0){ 00033 } 00034 00035 WNCUDPSocket::~WNCUDPSocket() { 00036 } 00037 00038 int WNCUDPSocket::init(void) { 00039 _is_blocking = false; // start out not blocking, user will set it if desired 00040 return ( WNCInterface::_pwnc->getWncStatus() == WNC_GOOD )? 0:-1; 00041 } 00042 00043 00044 int WNCUDPSocket::close(void) { 00045 WNCSocket::disconnect(); 00046 return ( WNCInterface::_pwnc->getWncStatus() == WNC_GOOD )? 0:-1; 00047 } 00048 00049 // -1 if unsuccessful, else number of bytes written 00050 int WNCUDPSocket::sendTo(WNCEndpoint &remote, char *packet, int length) { 00051 int ret = -1; 00052 00053 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), fail); 00054 if( remote._epAddr.port ) { //make sure the WNCEndpoint has an port assoicated with it 00055 if( WNCSocket::connect(remote._epAddr.IP,SOCK_DGRAM,remote._epAddr.port) ) { 00056 if( WNCInterface::_pwnc->write(0,packet,length) ) 00057 ret = length; 00058 close(); 00059 } 00060 } 00061 return ret; 00062 } 00063 00064 // 00065 // blocking is used to make the WNC keep checking for incoming data for a 00066 // period of time. 00067 00068 void WNCUDPSocket::set_blocking (bool blocking, unsigned int timeout) { 00069 _is_blocking = blocking; // true or false 00070 _btimeout = timeout; // user specifies in msec 00071 00072 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), void); 00073 WNCInterface::_pwnc->setReadRetryWait(0, 0); 00074 WNCInterface::_pwnc->setReadRetries(0, 0); 00075 } 00076 00077 // -1 if unsuccessful, else number of bytes received 00078 int WNCUDPSocket::receiveFrom(WNCEndpoint &remote, char *buffer, int length) { 00079 const uint8_t *ptr; 00080 Timer t; 00081 int done, ret = -1; 00082 00083 //make sure the WNCEndpoint has an port assoicated with it 00084 if( !remote._epAddr.port ) 00085 return -1; 00086 00087 CHK_WNCFE(( WNCInterface::_pwnc->getWncStatus() == FATAL_FLAG ), fail); 00088 ret = WNCSocket::connect(remote._epAddr.IP,SOCK_DGRAM,remote._epAddr.port); 00089 00090 t.start(); 00091 do { 00092 ret = WNCInterface::_pwnc->read(0, &ptr); 00093 done = ret | (t.read_ms() > _btimeout); 00094 } 00095 while( _is_blocking && !done ); 00096 t.stop(); 00097 00098 if( ret > length ) 00099 ret = length; 00100 memcpy( buffer, ptr, ret ); 00101 00102 return ret; 00103 } 00104 00105 00106
Generated on Tue Jul 12 2022 22:13:21 by
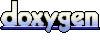