Motor driver library for the AP1017.
AP1017 Class Reference
This is a device driver for the AP1017 with pulse width modulation. More...
#include <AP1017.h>
Public Types | |
enum | Status { SUCCESS = 0x00, ERROR_FREQUENCY = 0x01, ERROR_DUTY_CYCLE = 0x02, ERROR_DIRECTION = 0x03, ERROR_PERIOD = 0x04, ERROR_PULSEWIDTH = 0x05, ERROR_MOTORON = 0x06 } |
Return status enumeration for debugging. More... | |
enum | Rotation { DIRECTION_CW = 0x00, DIRECTION_CCW = 0x01, DIRECTION_COAST = 0x02, DIRECTION_BRAKE = 0x03 } |
Motor directions. More... | |
Public Member Functions | |
AP1017 (DigitalOut *A, DigitalOut *B, I2C *M) | |
Default constructor creates motors with PWM initial duty cycle of 0%. | |
~AP1017 (void) | |
Disables PWM for the motors. | |
Status | setDirection (Rotation dir) |
Sets the direction to clockwise, counterclockwise, brake or coast. | |
Rotation | getDirection (void) |
Returns the currently set direction. | |
Status | setSpeed (double dc) |
Sets the speed via setting the duty cycle. | |
double | getSpeed (void) |
Returns the currently set speed as a percentage. | |
Status | start (void) |
Engages the motor. | |
Status | stop (void) |
Stops forced rotation of the motor. | |
Status | brake (void) |
Applies forced braking of motor. | |
Status | coast (void) |
Removes force from the motor and allows it to spin freely. | |
bool | isMotorOn (void) |
Checks if the motor is currently running. |
Detailed Description
This is a device driver for the AP1017 with pulse width modulation.
Definition at line 12 of file AP1017.h.
Member Enumeration Documentation
enum Rotation |
enum Status |
Return status enumeration for debugging.
Constructor & Destructor Documentation
AP1017 | ( | DigitalOut * | A, |
DigitalOut * | B, | ||
I2C * | M | ||
) |
Default constructor creates motors with PWM initial duty cycle of 0%.
Motor EN pin connected to D2, INA connected to D0, INB connected to D1.
Definition at line 6 of file AP1017.cpp.
~AP1017 | ( | void | ) |
Disables PWM for the motors.
Definition at line 23 of file AP1017.cpp.
Member Function Documentation
AP1017::Status brake | ( | void | ) |
Applies forced braking of motor.
- Returns:
- Returns successful termination or pulse width error.
Definition at line 147 of file AP1017.cpp.
AP1017::Status coast | ( | void | ) |
Removes force from the motor and allows it to spin freely.
- Returns:
- Returns successful termination or pulse width error.
Definition at line 153 of file AP1017.cpp.
AP1017::Rotation getDirection | ( | void | ) |
Returns the currently set direction.
Definition at line 79 of file AP1017.cpp.
double getSpeed | ( | void | ) |
Returns the currently set speed as a percentage.
Definition at line 123 of file AP1017.cpp.
bool isMotorOn | ( | void | ) |
Checks if the motor is currently running.
- Returns:
- TRUE if motor is on, FALSE if not.
Definition at line 159 of file AP1017.cpp.
AP1017::Status setDirection | ( | AP1017::Rotation | dir ) |
Sets the direction to clockwise, counterclockwise, brake or coast.
Changing between clockwise and counterclockwise may only be performed when motor is off.
- Parameters:
-
dir Rotation type: DIRECTION_CW, DIRECTION_CCW, DIRECTION_COAST, or DIRECTION_BRAKE
- Returns:
- Returns successful termination, ERROR_MOTORON for invalid direction switching, or ERROR_DIRECTION for invalid direction.
Definition at line 31 of file AP1017.cpp.
AP1017::Status setSpeed | ( | double | dc ) |
Sets the speed via setting the duty cycle.
Duty cycle given as a percentage.
- Parameters:
-
dc Duty cycle as a proportion (0.0 to 1.0).
- Returns:
- Returns successful termination or dutyc cyle error.
Definition at line 102 of file AP1017.cpp.
AP1017::Status start | ( | void | ) |
Engages the motor.
- Returns:
- Returns successful termination or pulse width error.
Definition at line 130 of file AP1017.cpp.
AP1017::Status stop | ( | void | ) |
Stops forced rotation of the motor.
- Returns:
- Returns successful termination or pulse width error.
Definition at line 139 of file AP1017.cpp.
Generated on Wed Jul 13 2022 20:24:02 by
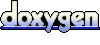