Motor driver library for the AP1017.
Embed:
(wiki syntax)
Show/hide line numbers
AP1017.cpp
00001 #include "AP1017.h" 00002 00003 /******************** Constructors & Destructors ****************************/ 00004 00005 // Default constructor 00006 AP1017::AP1017(DigitalOut* A, DigitalOut* B, I2C* M) : motorOn(false), dutyCycle(0.0) 00007 { 00008 i2cMotor = M; 00009 inA = A; 00010 inB = B; 00011 00012 // Instantiate TCA9554A 00013 motor = new TCA9554A(i2cMotor, TCA9554A::SLAVE_ADDRESS_38H); // 38H->Port 0->RSV 00014 00015 // Initialize RSV as output 00016 if (motor->configurePort(TCA9554A::PORT_0, TCA9554A::DIR_OUTPUT)!= TCA9554A::SUCCESS) { 00017 MSG("#AP1017: Error configuring TCA9554A port.\r\n"); 00018 } 00019 motor->setPortLevel(TCA9554A::PORT_0, TCA9554A::LOW); // Turn motor off 00020 } 00021 00022 // Default destructor 00023 AP1017::~AP1017(void) 00024 { 00025 stop(); 00026 delete inA, inB, motor, i2cMotor; 00027 } 00028 00029 /*********************** Member Functions ***********************************/ 00030 00031 AP1017::Status AP1017::setDirection(AP1017::Rotation dir) 00032 { 00033 direction = dir; 00034 00035 switch(direction){ 00036 case DIRECTION_CW: // direction = 0x00 00037 if(isMotorOn()) 00038 { 00039 MSG("#Error: Cannot change direction while motor is running.\r\n"); 00040 return ERROR_MOTORON; 00041 }else 00042 { 00043 inA->write(1); 00044 inB->write(0); 00045 MSG("#Direction: CCW\r\n"); 00046 } 00047 break; 00048 case DIRECTION_CCW: // direction = 0x01 00049 if(isMotorOn()) 00050 { 00051 MSG("#Error: Cannot change direction while motor is running.\r\n"); 00052 return ERROR_MOTORON; 00053 }else 00054 { 00055 inA->write(0); 00056 inB->write(1); 00057 MSG("#Direction: CW\r\n"); 00058 } 00059 break; 00060 case DIRECTION_BRAKE: // direction = 0x03 00061 inA->write(1); 00062 inB->write(1); 00063 MSG("#Direction: Brake\r\n"); 00064 break; 00065 case DIRECTION_COAST: // direction = 0x04 00066 inA->write(0); 00067 inB->write(0); 00068 motorOn = false; 00069 MSG("#Direction: Coast\r\n"); 00070 break; 00071 default: 00072 return ERROR_DIRECTION; 00073 } 00074 00075 return SUCCESS; 00076 } 00077 00078 00079 AP1017::Rotation AP1017::getDirection(void) 00080 { 00081 MSG("#Direction: "); 00082 switch(direction){ 00083 case DIRECTION_CW: 00084 MSG("CW\r\n"); 00085 break; 00086 case DIRECTION_CCW: 00087 MSG("CCW\r\n"); 00088 break; 00089 case DIRECTION_COAST: 00090 MSG("Coast\r\n"); 00091 break; 00092 case DIRECTION_BRAKE: 00093 MSG("Brake\r\n"); 00094 break; 00095 default: 00096 MSG("Error: Invalid direction\r\n"); 00097 } 00098 return direction; 00099 } 00100 00101 00102 AP1017::Status AP1017::setSpeed(double dc) 00103 { 00104 if((dc <= 100.0) && (dc >= 0.0)) 00105 { 00106 dutyCycle = dc/100.0; 00107 00108 if(motorOn == true){ 00109 MSG("Speed setting not yet implemented.\r\n"); 00110 //MSG("#Changed running motor speed: %.1f%%.\r\n", dc); 00111 } 00112 } 00113 else 00114 { 00115 dutyCycle = 0.0; 00116 return ERROR_DUTY_CYCLE; 00117 } 00118 00119 return SUCCESS; 00120 } 00121 00122 00123 double AP1017::getSpeed(void) 00124 { 00125 MSG("Speed: %.1f%%\r\n.", dutyCycle*100.0); 00126 return dutyCycle*100.0; 00127 } 00128 00129 00130 AP1017::Status AP1017::start(void) 00131 { 00132 motor->setPortLevel(TCA9554A::PORT_0, TCA9554A::HIGH); // set RSV high 00133 motorOn = true; // Set ON flag 00134 00135 return SUCCESS; 00136 } 00137 00138 00139 AP1017::Status AP1017::stop(void) 00140 { 00141 motor->setPortLevel(TCA9554A::PORT_0, TCA9554A::LOW); // set RSV low 00142 motorOn = false; // Set OFF flag 00143 00144 return SUCCESS; 00145 } 00146 00147 AP1017::Status AP1017::brake(void) 00148 { 00149 setDirection(DIRECTION_BRAKE); 00150 return SUCCESS; 00151 } 00152 00153 AP1017::Status AP1017::coast(void) 00154 { 00155 setDirection(DIRECTION_COAST); 00156 return SUCCESS; 00157 } 00158 00159 bool AP1017::isMotorOn(void) 00160 { 00161 return motorOn; 00162 }
Generated on Wed Jul 13 2022 20:24:02 by
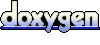