Motor driver library for the AP1017.
Embed:
(wiki syntax)
Show/hide line numbers
AP1017.h
00001 #ifndef __AP1017_H 00002 #define __AP1017_H 00003 00004 #include "mbed.h" 00005 #include "akdp_debug.h" 00006 #include "tca9554a.h" 00007 #include "I2C.h" 00008 00009 /** 00010 * This is a device driver for the AP1017 with pulse width modulation. 00011 */ 00012 class AP1017 00013 { 00014 public: 00015 00016 /** 00017 * Default constructor creates motors with PWM initial duty cycle of 0%. 00018 * Motor EN pin connected to D2, INA connected to D0, INB connected to D1. 00019 */ 00020 AP1017(DigitalOut* A, DigitalOut* B, I2C* M); 00021 00022 /** 00023 * Disables PWM for the motors. 00024 */ 00025 ~AP1017(void); 00026 00027 /** 00028 * Return status enumeration for debugging. 00029 */ 00030 typedef enum { 00031 SUCCESS = 0x00, /**< Successful termination */ 00032 ERROR_FREQUENCY = 0x01, /**< Frequency out of bounds */ 00033 ERROR_DUTY_CYCLE = 0x02, /**< Invalid duty cycle */ 00034 ERROR_DIRECTION = 0x03, /**< Invalid direction */ 00035 ERROR_PERIOD = 0x04, /**< Invalid period */ 00036 ERROR_PULSEWIDTH = 0x05, /**< Invalid pulse width */ 00037 ERROR_MOTORON = 0x06 /**< Direction switched while motor on */ 00038 } Status; 00039 00040 /** 00041 * Motor directions. 00042 */ 00043 typedef enum { 00044 DIRECTION_CW = 0x00, /**< Clockwise motor rotation */ 00045 DIRECTION_CCW = 0x01, /**< Counterclockwise motor rotation */ 00046 DIRECTION_COAST = 0x02, /**< Release motor to coast */ 00047 DIRECTION_BRAKE = 0x03 /**< Brake motor */ 00048 } Rotation; 00049 00050 00051 /** 00052 * Sets the direction to clockwise, counterclockwise, brake or coast. 00053 * Changing between clockwise and counterclockwise may only be performed 00054 * when motor is off. 00055 * 00056 * @param dir Rotation type: DIRECTION_CW, DIRECTION_CCW, DIRECTION_COAST, 00057 * or DIRECTION_BRAKE 00058 * @return Returns successful termination, ERROR_MOTORON for invalid 00059 * direction switching, or ERROR_DIRECTION for invalid direction. 00060 */ 00061 Status setDirection(Rotation dir); 00062 00063 /** 00064 * Returns the currently set direction. 00065 */ 00066 Rotation getDirection(void); 00067 00068 /** 00069 * Sets the speed via setting the duty cycle. Duty cycle given 00070 * as a percentage. 00071 * 00072 * @param dc Duty cycle as a proportion (0.0 to 1.0). 00073 * @return Returns successful termination or dutyc cyle error. 00074 */ 00075 Status setSpeed(double dc); 00076 00077 /** 00078 * Returns the currently set speed as a percentage. 00079 */ 00080 double getSpeed(void); 00081 00082 /** 00083 * Engages the motor. 00084 * 00085 * @return Returns successful termination or pulse width error. 00086 */ 00087 Status start(void); 00088 00089 /** 00090 * Stops forced rotation of the motor. 00091 * 00092 * @return Returns successful termination or pulse width error. 00093 */ 00094 Status stop(void); 00095 00096 /** 00097 * Applies forced braking of motor. 00098 * 00099 * @return Returns successful termination or pulse width error. 00100 */ 00101 Status brake(void); 00102 00103 /** 00104 * Removes force from the motor and allows it to spin freely. 00105 * 00106 * @return Returns successful termination or pulse width error. 00107 */ 00108 Status coast(void); 00109 00110 /** 00111 * Checks if the motor is currently running. 00112 * 00113 * @return TRUE if motor is on, FALSE if not. 00114 */ 00115 bool isMotorOn(void); 00116 00117 private: 00118 00119 bool motorOn; // Status flag for the motor 00120 double dutyCycle; // Given as proportion: 0.00 to 1.00 00121 Rotation direction; 00122 00123 TCA9554A *motor; // Motor object 00124 I2C* i2cMotor; 00125 00126 DigitalOut* inA; 00127 DigitalOut* inB; 00128 /* inA=L, inB=L -> Standby (Coast) 00129 * inA=H, inB=L -> Forward (CW) 00130 * inA=L, inB=H -> Reverse (CCW) 00131 * inA=H, inB=H -> Brake 00132 */ 00133 00134 }; 00135 00136 #endif
Generated on Wed Jul 13 2022 20:24:02 by
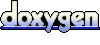