Serial Comm using the USBTX/USBRX seems to work fine. Using another set of pins (e.g., p9/p10), the serial comm is garbled.
I have two Putty sessions, one connected to USBTX/RX (com 11 on my pc) and the other to P9/P10 (com 12 on the pc). The code attempts to get a char from either one, echo it, and transmit to the other.
Anything I type into the com11 Putty is fine, but the echo'd response to com12 Putty is garbled. Anything typed into the com12 Putty is garbled, as well as what is echo'd to the com11 Putty.
Baud rates, etc. are supposedly set correctly.
#include "mbed.h"
// term is attached to a serial port on the PC running Putty. 9600,8,n,1
// mbedUSB is the native mbed USB. A separate instance of Putty
// runs on the PC attached to this com port - set to 19200,8,n,1
Serial term(p9, p10);
Serial mbedUSB(USBTX, USBRX); // tx, rx
BusOut BLUEleds(LED1, LED2, LED3, LED4);
int main() {
int cBytes;
short icnt=0;
// setup stuff
mbedUSB.baud(19200);
term.baud(9600);
wait(.1);
mbedUSB.format(8,Serial::None,1);
term.format(8,Serial::None,1);
wait(.1);
// announce you're ready
mbedUSB.printf("\fThis is a test\n");
term.printf("\fThis is a test, too\n");
// process keys
BLUEleds = 0;
while (1) {
if (icnt++ == 0) BLUEleds = BLUEleds ^ 0x0A; // i'm alive leds
// get char from USB term, echo locally and output to P9/p10 Term
if (mbedUSB.readable()) {
cBytes = mbedUSB.getc();
mbedUSB.putc(cBytes);
term.putc(cBytes);
}
// get char from p9/p10 Term, echo locally and output to USB term
if (term.readable()) {
cBytes = term.getc();
term.putc(cBytes);
mbedUSB.putc(cBytes);
}
}
}
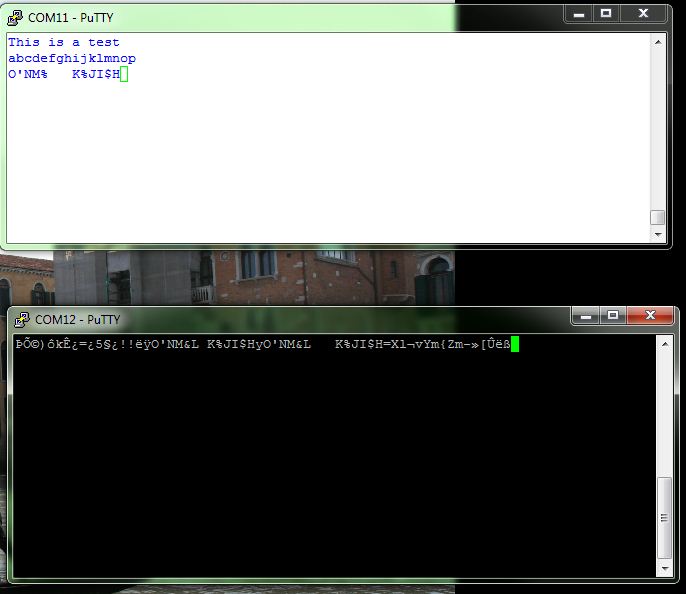
Serial Comm using the USBTX/USBRX seems to work fine. Using another set of pins (e.g., p9/p10), the serial comm is garbled.
I have two Putty sessions, one connected to USBTX/RX (com 11 on my pc) and the other to P9/P10 (com 12 on the pc). The code attempts to get a char from either one, echo it, and transmit to the other.
Anything I type into the com11 Putty is fine, but the echo'd response to com12 Putty is garbled. Anything typed into the com12 Putty is garbled, as well as what is echo'd to the com11 Putty.
Baud rates, etc. are supposedly set correctly.