DC Motor
How to drive a standard DC motor using PWM and an H-Bridge
Hello World
Import programMotor_HelloWorld
Hello World for a simple motor driver class, used to control an H-Bridge with PwmOut and 2 DigitalOuts
Library
Import libraryMotordriver
motor drive libary to use speed control, coasting and dynamic braking. NOTE, dynamic braking my result in large currents. this may or may not set the motor driver on fire/break it/other undesired effects. so read the data sheet folks.
Pinout
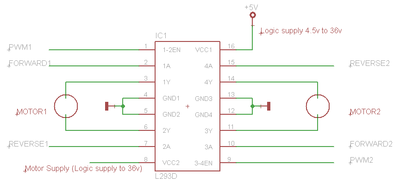
Notes
The speed function takes a value from 1 to -1. full forward to full reverse. however it will not switch from foward to reverse or reverse to forward without going through speed = 0, coast or stop. this stops the motor from going from full one direction to the other, and the large current draw associated with this. it returns the duty on the PWM line.
The coast function means the motor driver switches off, and does nothing.
The stop function dynamically brakes, which is supported on L298's but if dynamic braking develops more current than the motor drivers are rated for, things start to fail. read data sheet, and if in doubt, don't. it returns the duty on the PWM line, or -1 if you didn't set brakeable to 1.
main.cpp
// test code, this demonstrates working motor drivers. // full reverse to full stop, dynamicaly brake and switch off. #include motordriver.h Motor A(p22, p6, p5, 1); // pwm, fwd, rev, can brake Motor B(p21, p7, p8, 1); // pwm, fwd, rev, can brake int main() { for (float s= -1.0; s < 1.0 ; s += 0.01) { A.speed(s); B.speed(s); wait(0.02); } A.stop(); B.stop(); wait(1); A.coast(); B.coast(); }
Connection diagram, note the current sense pins(1,15) should be connected to ground through a 0.5 Ohm resistor. attaching to analog inputs is not required.