An interrupt-driven interface to 4x4 keypad.
Dependents: FYPFinalProgram FYPFinalizeProgram KEYS Proyect_Patric_electronic_door_MSC_Ok_ESP ... more
Keypad Class Reference
An interrupt-based interface to 4x4 keypad. More...
#include <Keypad.h>
Public Member Functions | |
Keypad (PinName r0, PinName r1, PinName r2, PinName r3, PinName c0, PinName c1, PinName c2, PinName c3, int debounce_ms=20) | |
Create a 4x4 (row, col) or 4x3 keypad interface: | |
~Keypad () | |
Destructor. | |
void | start (void) |
Start the keypad interrupt routines. | |
void | stop (void) |
Stop the keypad interrupt routines. | |
void | attach (uint32_t(*fptr)(uint32_t)) |
User-defined function that to be called when a key is pressed. |
Detailed Description
An interrupt-based interface to 4x4 keypad.
On each key pressed on a keypad, the index of the key is passed to a user-defined function. User is free to define what to be done with the input.
Example:
#include "mbed.h" #include "Keypad.h" // Define your own keypad values char Keytable[] = { '1', '2', '3', 'A', // r0 '4', '5', '6', 'B', // r1 '7', '8', '9', 'C', // r2 '*', '0', '#', 'D' // r3 }; // c0 c1 c2 c3 uint32_t Index; uint32_t cbAfterInput(uint32_t index) { Index = index; return 0; } int main() { // r0 r1 r2 r3 c0 c1 c2 c3 Keypad keypad(p21, p22, p23, p24, p25, p26, p27, p28); keypad.attach(&cbAfterInput); keypad.start(); // energize the keypad via c0-c3 while (1) { __wfi(); printf("Interrupted\r\n"); printf("Index:%d => Key:%c\r\n", Index, Keytable[Index]); } }
Definition at line 72 of file Keypad.h.
Constructor & Destructor Documentation
Keypad | ( | PinName | r0, |
PinName | r1, | ||
PinName | r2, | ||
PinName | r3, | ||
PinName | c0, | ||
PinName | c1, | ||
PinName | c2, | ||
PinName | c3, | ||
int | debounce_ms = 20 |
||
) |
Create a 4x4 (row, col) or 4x3 keypad interface:
| Col0 | Col1 | Col2 | Col3 -------+------+------+------+----- Row 0 | x | x | x | x Row 1 | x | x | x | x Row 2 | x | x | x | x Row 3 | x | x | x | x
- Parameters:
-
row<0..3> Row data lines col<0..3> Column data lines debounce_ms Debounce in ms (Default to 20ms)
Definition at line 49 of file Keypad.cpp.
~Keypad | ( | ) |
Destructor.
Definition at line 91 of file Keypad.cpp.
Member Function Documentation
void attach | ( | uint32_t(*)(uint32_t) | fptr ) |
User-defined function that to be called when a key is pressed.
- Parameters:
-
fptr A function pointer takes a uint32_t and returns uint32_t
void start | ( | void | ) |
Start the keypad interrupt routines.
Definition at line 106 of file Keypad.cpp.
void stop | ( | void | ) |
Stop the keypad interrupt routines.
Definition at line 115 of file Keypad.cpp.
Generated on Tue Jul 12 2022 19:57:39 by
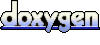