An interrupt-driven interface to 4x4 keypad.
Dependents: FYPFinalProgram FYPFinalizeProgram KEYS Proyect_Patric_electronic_door_MSC_Ok_ESP ... more
Keypad.h
00001 /* mbed Keypad library, using user-defined interrupt callback 00002 * Copyright (c) 2012 Yoong Hor Meng 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining 00005 * a copy of this software and associated documentation files (the 00006 * "Software"), to deal in the Software without restriction, including 00007 * without limitation the rights to use, copy, modify, merge, publish, 00008 * distribute, sublicense, and/or sell copies of the Software, and to 00009 * permit persons to whom the Software is furnished to do so, subject to 00010 * the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included 00013 * in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00016 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00018 * IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 00019 * CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 00020 * TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE 00021 * SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE 00022 */ 00023 00024 #ifndef KEYPAD_H 00025 #define KEYPAD_H 00026 00027 #include "mbed.h" 00028 #include "FPointer.h" 00029 00030 /** 00031 * An interrupt-based interface to 4x4 keypad. 00032 * 00033 * On each key pressed on a keypad, the index of the key is passed to a 00034 * user-defined function. User is free to define what to be done with the 00035 * input. 00036 * 00037 * Example: 00038 * @code 00039 * #include "mbed.h" 00040 * #include "Keypad.h" 00041 * 00042 * // Define your own keypad values 00043 * char Keytable[] = { '1', '2', '3', 'A', // r0 00044 * '4', '5', '6', 'B', // r1 00045 * '7', '8', '9', 'C', // r2 00046 * '*', '0', '#', 'D' // r3 00047 * }; 00048 * // c0 c1 c2 c3 00049 * 00050 * uint32_t Index; 00051 * 00052 * uint32_t cbAfterInput(uint32_t index) { 00053 * Index = index; 00054 * return 0; 00055 * } 00056 * 00057 * int main() { 00058 * // r0 r1 r2 r3 c0 c1 c2 c3 00059 * Keypad keypad(p21, p22, p23, p24, p25, p26, p27, p28); 00060 * keypad.attach(&cbAfterInput); 00061 * keypad.start(); // energize the keypad via c0-c3 00062 * 00063 * while (1) { 00064 * __wfi(); 00065 * printf("Interrupted\r\n"); 00066 * printf("Index:%d => Key:%c\r\n", Index, Keytable[Index]); 00067 * } 00068 * } 00069 * @endcode 00070 */ 00071 00072 class Keypad { 00073 public: 00074 /** Create a 4x4 (row, col) or 4x3 keypad interface: 00075 * 00076 * | Col0 | Col1 | Col2 | Col3 00077 * -------+------+------+------+----- 00078 * Row 0 | x | x | x | x 00079 * Row 1 | x | x | x | x 00080 * Row 2 | x | x | x | x 00081 * Row 3 | x | x | x | x 00082 * 00083 * @param row<0..3> Row data lines 00084 * @param col<0..3> Column data lines 00085 * @param debounce_ms Debounce in ms (Default to 20ms) 00086 */ 00087 Keypad(PinName r0, PinName r1, PinName r2, PinName r3, 00088 PinName c0, PinName c1, PinName c2, PinName c3, 00089 int debounce_ms = 20); 00090 00091 /** Destructor 00092 */ 00093 ~Keypad(); 00094 00095 /** Start the keypad interrupt routines 00096 */ 00097 void start(void); 00098 00099 /** Stop the keypad interrupt routines 00100 */ 00101 void stop(void); 00102 00103 /** User-defined function that to be called when a key is pressed 00104 * @param fptr A function pointer takes a uint32_t and 00105 * returns uint32_t 00106 */ 00107 void attach(uint32_t (*fptr)(uint32_t)); 00108 00109 protected: 00110 InterruptIn *_rows[4]; 00111 DigitalOut *_cols[4]; 00112 int _debounce; // miliseconds 00113 int _nRow; 00114 int _nCol; 00115 FPointer _callback; // Called after each input 00116 00117 void _checkIndex(int row, InterruptIn *therow); 00118 void _cbRow0Rise(void); 00119 void _cbRow1Rise(void); 00120 void _cbRow2Rise(void); 00121 void _cbRow3Rise(void); 00122 void _setupRiseTrigger(void); 00123 }; 00124 00125 #endif // KEYPAD_H 00126
Generated on Tue Jul 12 2022 19:57:39 by
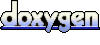