Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
MatrixCwiseUnaryOps.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2008-2009 Gael Guennebaud <gael.guennebaud@inria.fr> 00005 // Copyright (C) 2006-2008 Benoit Jacob <jacob.benoit.1@gmail.com> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 // This file is a base class plugin containing matrix specifics coefficient wise functions. 00012 00013 /** \returns an expression of the coefficient-wise absolute value of \c *this 00014 * 00015 * Example: \include MatrixBase_cwiseAbs.cpp 00016 * Output: \verbinclude MatrixBase_cwiseAbs.out 00017 * 00018 * \sa cwiseAbs2() 00019 */ 00020 EIGEN_STRONG_INLINE const CwiseUnaryOp<internal::scalar_abs_op<Scalar>, const Derived> 00021 cwiseAbs() const { return derived(); } 00022 00023 /** \returns an expression of the coefficient-wise squared absolute value of \c *this 00024 * 00025 * Example: \include MatrixBase_cwiseAbs2.cpp 00026 * Output: \verbinclude MatrixBase_cwiseAbs2.out 00027 * 00028 * \sa cwiseAbs() 00029 */ 00030 EIGEN_STRONG_INLINE const CwiseUnaryOp<internal::scalar_abs2_op<Scalar>, const Derived> 00031 cwiseAbs2() const { return derived(); } 00032 00033 /** \returns an expression of the coefficient-wise square root of *this. 00034 * 00035 * Example: \include MatrixBase_cwiseSqrt.cpp 00036 * Output: \verbinclude MatrixBase_cwiseSqrt.out 00037 * 00038 * \sa cwisePow(), cwiseSquare() 00039 */ 00040 inline const CwiseUnaryOp<internal::scalar_sqrt_op<Scalar>, const Derived> 00041 cwiseSqrt() const { return derived(); } 00042 00043 /** \returns an expression of the coefficient-wise inverse of *this. 00044 * 00045 * Example: \include MatrixBase_cwiseInverse.cpp 00046 * Output: \verbinclude MatrixBase_cwiseInverse.out 00047 * 00048 * \sa cwiseProduct() 00049 */ 00050 inline const CwiseUnaryOp<internal::scalar_inverse_op<Scalar>, const Derived> 00051 cwiseInverse() const { return derived(); }
Generated on Tue Jul 12 2022 17:46:58 by
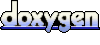