Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Macros.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2008-2010 Gael Guennebaud <gael.guennebaud@inria.fr> 00005 // Copyright (C) 2006-2008 Benoit Jacob <jacob.benoit.1@gmail.com> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 #ifndef EIGEN_MACROS_H 00012 #define EIGEN_MACROS_H 00013 00014 #define EIGEN_WORLD_VERSION 3 00015 #define EIGEN_MAJOR_VERSION 2 00016 #define EIGEN_MINOR_VERSION 8 00017 00018 #define EIGEN_VERSION_AT_LEAST(x,y,z) (EIGEN_WORLD_VERSION>x || (EIGEN_WORLD_VERSION>=x && \ 00019 (EIGEN_MAJOR_VERSION>y || (EIGEN_MAJOR_VERSION>=y && \ 00020 EIGEN_MINOR_VERSION>=z)))) 00021 00022 00023 // Compiler identification, EIGEN_COMP_* 00024 00025 /// \internal EIGEN_COMP_GNUC set to 1 for all compilers compatible with GCC 00026 #ifdef __GNUC__ 00027 #define EIGEN_COMP_GNUC 1 00028 #else 00029 #define EIGEN_COMP_GNUC 0 00030 #endif 00031 00032 /// \internal EIGEN_COMP_CLANG set to 1 if the compiler is clang (alias for __clang__) 00033 #if defined(__clang__) 00034 #define EIGEN_COMP_CLANG 1 00035 #else 00036 #define EIGEN_COMP_CLANG 0 00037 #endif 00038 00039 00040 /// \internal EIGEN_COMP_LLVM set to 1 if the compiler backend is llvm 00041 #if defined(__llvm__) 00042 #define EIGEN_COMP_LLVM 1 00043 #else 00044 #define EIGEN_COMP_LLVM 0 00045 #endif 00046 00047 /// \internal EIGEN_COMP_ICC set to __INTEL_COMPILER if the compiler is Intel compiler, 0 otherwise 00048 #if defined(__INTEL_COMPILER) 00049 #define EIGEN_COMP_ICC __INTEL_COMPILER 00050 #else 00051 #define EIGEN_COMP_ICC 0 00052 #endif 00053 00054 /// \internal EIGEN_COMP_MINGW set to 1 if the compiler is mingw 00055 #if defined(__MINGW32__) 00056 #define EIGEN_COMP_MINGW 1 00057 #else 00058 #define EIGEN_COMP_MINGW 0 00059 #endif 00060 00061 /// \internal EIGEN_COMP_SUNCC set to 1 if the compiler is Solaris Studio 00062 #if defined(__SUNPRO_CC) 00063 #define EIGEN_COMP_SUNCC 1 00064 #else 00065 #define EIGEN_COMP_SUNCC 0 00066 #endif 00067 00068 /// \internal EIGEN_COMP_MSVC set to _MSC_VER if the compiler is Microsoft Visual C++, 0 otherwise. 00069 #if defined(_MSC_VER) 00070 #define EIGEN_COMP_MSVC _MSC_VER 00071 #else 00072 #define EIGEN_COMP_MSVC 0 00073 #endif 00074 00075 /// \internal EIGEN_COMP_MSVC_STRICT set to 1 if the compiler is really Microsoft Visual C++ and not ,e.g., ICC 00076 #if EIGEN_COMP_MSVC && !(EIGEN_COMP_ICC) 00077 #define EIGEN_COMP_MSVC_STRICT _MSC_VER 00078 #else 00079 #define EIGEN_COMP_MSVC_STRICT 0 00080 #endif 00081 00082 /// \internal EIGEN_COMP_IBM set to 1 if the compiler is IBM XL C++ 00083 #if defined(__IBMCPP__) || defined(__xlc__) 00084 #define EIGEN_COMP_IBM 1 00085 #else 00086 #define EIGEN_COMP_IBM 0 00087 #endif 00088 00089 /// \internal EIGEN_COMP_PGI set to 1 if the compiler is Portland Group Compiler 00090 #if defined(__PGI) 00091 #define EIGEN_COMP_PGI 1 00092 #else 00093 #define EIGEN_COMP_PGI 0 00094 #endif 00095 00096 /// \internal EIGEN_COMP_ARM set to 1 if the compiler is ARM Compiler 00097 #if defined(__CC_ARM) || defined(__ARMCC_VERSION) 00098 #define EIGEN_COMP_ARM 1 00099 #else 00100 #define EIGEN_COMP_ARM 0 00101 #endif 00102 00103 00104 /// \internal EIGEN_GNUC_STRICT set to 1 if the compiler is really GCC and not a compatible compiler (e.g., ICC, clang, mingw, etc.) 00105 #if EIGEN_COMP_GNUC && !(EIGEN_COMP_CLANG || EIGEN_COMP_ICC || EIGEN_COMP_MINGW || EIGEN_COMP_PGI || EIGEN_COMP_IBM || EIGEN_COMP_ARM ) 00106 #define EIGEN_COMP_GNUC_STRICT 1 00107 #else 00108 #define EIGEN_COMP_GNUC_STRICT 0 00109 #endif 00110 00111 00112 #if EIGEN_COMP_GNUC 00113 #define EIGEN_GNUC_AT_LEAST(x,y) ((__GNUC__==x && __GNUC_MINOR__>=y) || __GNUC__>x) 00114 #define EIGEN_GNUC_AT_MOST(x,y) ((__GNUC__==x && __GNUC_MINOR__<=y) || __GNUC__<x) 00115 #define EIGEN_GNUC_AT(x,y) ( __GNUC__==x && __GNUC_MINOR__==y ) 00116 #else 00117 #define EIGEN_GNUC_AT_LEAST(x,y) 0 00118 #define EIGEN_GNUC_AT_MOST(x,y) 0 00119 #define EIGEN_GNUC_AT(x,y) 0 00120 #endif 00121 00122 // FIXME: could probably be removed as we do not support gcc 3.x anymore 00123 #if EIGEN_COMP_GNUC && (__GNUC__ <= 3) 00124 #define EIGEN_GCC3_OR_OLDER 1 00125 #else 00126 #define EIGEN_GCC3_OR_OLDER 0 00127 #endif 00128 00129 00130 // Architecture identification, EIGEN_ARCH_* 00131 00132 #if defined(__x86_64__) || defined(_M_X64) || defined(__amd64) 00133 #define EIGEN_ARCH_x86_64 1 00134 #else 00135 #define EIGEN_ARCH_x86_64 0 00136 #endif 00137 00138 #if defined(__i386__) || defined(_M_IX86) || defined(_X86_) || defined(__i386) 00139 #define EIGEN_ARCH_i386 1 00140 #else 00141 #define EIGEN_ARCH_i386 0 00142 #endif 00143 00144 #if EIGEN_ARCH_x86_64 || EIGEN_ARCH_i386 00145 #define EIGEN_ARCH_i386_OR_x86_64 1 00146 #else 00147 #define EIGEN_ARCH_i386_OR_x86_64 0 00148 #endif 00149 00150 /// \internal EIGEN_ARCH_ARM set to 1 if the architecture is ARM 00151 #if defined(__arm__) 00152 #define EIGEN_ARCH_ARM 1 00153 #else 00154 #define EIGEN_ARCH_ARM 0 00155 #endif 00156 00157 /// \internal EIGEN_ARCH_ARM64 set to 1 if the architecture is ARM64 00158 #if defined(__aarch64__) 00159 #define EIGEN_ARCH_ARM64 1 00160 #else 00161 #define EIGEN_ARCH_ARM64 0 00162 #endif 00163 00164 #if EIGEN_ARCH_ARM || EIGEN_ARCH_ARM64 00165 #define EIGEN_ARCH_ARM_OR_ARM64 1 00166 #else 00167 #define EIGEN_ARCH_ARM_OR_ARM64 0 00168 #endif 00169 00170 /// \internal EIGEN_ARCH_MIPS set to 1 if the architecture is MIPS 00171 #if defined(__mips__) || defined(__mips) 00172 #define EIGEN_ARCH_MIPS 1 00173 #else 00174 #define EIGEN_ARCH_MIPS 0 00175 #endif 00176 00177 /// \internal EIGEN_ARCH_SPARC set to 1 if the architecture is SPARC 00178 #if defined(__sparc__) || defined(__sparc) 00179 #define EIGEN_ARCH_SPARC 1 00180 #else 00181 #define EIGEN_ARCH_SPARC 0 00182 #endif 00183 00184 /// \internal EIGEN_ARCH_IA64 set to 1 if the architecture is Intel Itanium 00185 #if defined(__ia64__) 00186 #define EIGEN_ARCH_IA64 1 00187 #else 00188 #define EIGEN_ARCH_IA64 0 00189 #endif 00190 00191 /// \internal EIGEN_ARCH_PPC set to 1 if the architecture is PowerPC 00192 #if defined(__powerpc__) || defined(__ppc__) || defined(_M_PPC) 00193 #define EIGEN_ARCH_PPC 1 00194 #else 00195 #define EIGEN_ARCH_PPC 0 00196 #endif 00197 00198 00199 00200 // Operating system identification, EIGEN_OS_* 00201 00202 /// \internal EIGEN_OS_UNIX set to 1 if the OS is a unix variant 00203 #if defined(__unix__) || defined(__unix) 00204 #define EIGEN_OS_UNIX 1 00205 #else 00206 #define EIGEN_OS_UNIX 0 00207 #endif 00208 00209 /// \internal EIGEN_OS_LINUX set to 1 if the OS is based on Linux kernel 00210 #if defined(__linux__) 00211 #define EIGEN_OS_LINUX 1 00212 #else 00213 #define EIGEN_OS_LINUX 0 00214 #endif 00215 00216 /// \internal EIGEN_OS_ANDROID set to 1 if the OS is Android 00217 // note: ANDROID is defined when using ndk_build, __ANDROID__ is defined when using a standalone toolchain. 00218 #if defined(__ANDROID__) || defined(ANDROID) 00219 #define EIGEN_OS_ANDROID 1 00220 #else 00221 #define EIGEN_OS_ANDROID 0 00222 #endif 00223 00224 /// \internal EIGEN_OS_GNULINUX set to 1 if the OS is GNU Linux and not Linux-based OS (e.g., not android) 00225 #if defined(__gnu_linux__) && !(EIGEN_OS_ANDROID) 00226 #define EIGEN_OS_GNULINUX 1 00227 #else 00228 #define EIGEN_OS_GNULINUX 0 00229 #endif 00230 00231 /// \internal EIGEN_OS_BSD set to 1 if the OS is a BSD variant 00232 #if defined(__FreeBSD__) || defined(__NetBSD__) || defined(__OpenBSD__) || defined(__bsdi__) || defined(__DragonFly__) 00233 #define EIGEN_OS_BSD 1 00234 #else 00235 #define EIGEN_OS_BSD 0 00236 #endif 00237 00238 /// \internal EIGEN_OS_MAC set to 1 if the OS is MacOS 00239 #if defined(__APPLE__) 00240 #define EIGEN_OS_MAC 1 00241 #else 00242 #define EIGEN_OS_MAC 0 00243 #endif 00244 00245 /// \internal EIGEN_OS_QNX set to 1 if the OS is QNX 00246 #if defined(__QNX__) 00247 #define EIGEN_OS_QNX 1 00248 #else 00249 #define EIGEN_OS_QNX 0 00250 #endif 00251 00252 /// \internal EIGEN_OS_WIN set to 1 if the OS is Windows based 00253 #if defined(_WIN32) 00254 #define EIGEN_OS_WIN 1 00255 #else 00256 #define EIGEN_OS_WIN 0 00257 #endif 00258 00259 /// \internal EIGEN_OS_WIN64 set to 1 if the OS is Windows 64bits 00260 #if defined(_WIN64) 00261 #define EIGEN_OS_WIN64 1 00262 #else 00263 #define EIGEN_OS_WIN64 0 00264 #endif 00265 00266 /// \internal EIGEN_OS_WINCE set to 1 if the OS is Windows CE 00267 #if defined(_WIN32_WCE) 00268 #define EIGEN_OS_WINCE 1 00269 #else 00270 #define EIGEN_OS_WINCE 0 00271 #endif 00272 00273 /// \internal EIGEN_OS_CYGWIN set to 1 if the OS is Windows/Cygwin 00274 #if defined(__CYGWIN__) 00275 #define EIGEN_OS_CYGWIN 1 00276 #else 00277 #define EIGEN_OS_CYGWIN 0 00278 #endif 00279 00280 /// \internal EIGEN_OS_WIN_STRICT set to 1 if the OS is really Windows and not some variants 00281 #if EIGEN_OS_WIN && !( EIGEN_OS_WINCE || EIGEN_OS_CYGWIN ) 00282 #define EIGEN_OS_WIN_STRICT 1 00283 #else 00284 #define EIGEN_OS_WIN_STRICT 0 00285 #endif 00286 00287 /// \internal EIGEN_OS_SUN set to 1 if the OS is SUN 00288 #if (defined(sun) || defined(__sun)) && !(defined(__SVR4) || defined(__svr4__)) 00289 #define EIGEN_OS_SUN 1 00290 #else 00291 #define EIGEN_OS_SUN 0 00292 #endif 00293 00294 /// \internal EIGEN_OS_SOLARIS set to 1 if the OS is Solaris 00295 #if (defined(sun) || defined(__sun)) && (defined(__SVR4) || defined(__svr4__)) 00296 #define EIGEN_OS_SOLARIS 1 00297 #else 00298 #define EIGEN_OS_SOLARIS 0 00299 #endif 00300 00301 00302 #if EIGEN_GNUC_AT_MOST(4,3) && !defined(__clang__) 00303 // see bug 89 00304 #define EIGEN_SAFE_TO_USE_STANDARD_ASSERT_MACRO 0 00305 #else 00306 #define EIGEN_SAFE_TO_USE_STANDARD_ASSERT_MACRO 1 00307 #endif 00308 00309 // 16 byte alignment is only useful for vectorization. Since it affects the ABI, we need to enable 00310 // 16 byte alignment on all platforms where vectorization might be enabled. In theory we could always 00311 // enable alignment, but it can be a cause of problems on some platforms, so we just disable it in 00312 // certain common platform (compiler+architecture combinations) to avoid these problems. 00313 // Only static alignment is really problematic (relies on nonstandard compiler extensions that don't 00314 // work everywhere, for example don't work on GCC/ARM), try to keep heap alignment even 00315 // when we have to disable static alignment. 00316 #if defined(__GNUC__) && !(defined(__i386__) || defined(__x86_64__) || defined(__powerpc__) || defined(__ppc__) || defined(__ia64__)) 00317 #define EIGEN_GCC_AND_ARCH_DOESNT_WANT_STACK_ALIGNMENT 1 00318 #else 00319 #define EIGEN_GCC_AND_ARCH_DOESNT_WANT_STACK_ALIGNMENT 0 00320 #endif 00321 00322 // static alignment is completely disabled with GCC 3, Sun Studio, and QCC/QNX 00323 #if !EIGEN_GCC_AND_ARCH_DOESNT_WANT_STACK_ALIGNMENT \ 00324 && !EIGEN_GCC3_OR_OLDER \ 00325 && !defined(__SUNPRO_CC) \ 00326 && !defined(__QNXNTO__) 00327 #define EIGEN_ARCH_WANTS_STACK_ALIGNMENT 1 00328 #else 00329 #define EIGEN_ARCH_WANTS_STACK_ALIGNMENT 0 00330 #endif 00331 00332 #ifdef EIGEN_DONT_ALIGN 00333 #ifndef EIGEN_DONT_ALIGN_STATICALLY 00334 #define EIGEN_DONT_ALIGN_STATICALLY 00335 #endif 00336 #define EIGEN_ALIGN 0 00337 #else 00338 #define EIGEN_ALIGN 1 00339 #endif 00340 00341 // EIGEN_ALIGN_STATICALLY is the true test whether we want to align arrays on the stack or not. It takes into account both the user choice to explicitly disable 00342 // alignment (EIGEN_DONT_ALIGN_STATICALLY) and the architecture config (EIGEN_ARCH_WANTS_STACK_ALIGNMENT). Henceforth, only EIGEN_ALIGN_STATICALLY should be used. 00343 #if EIGEN_ARCH_WANTS_STACK_ALIGNMENT && !defined(EIGEN_DONT_ALIGN_STATICALLY) 00344 #define EIGEN_ALIGN_STATICALLY 1 00345 #else 00346 #define EIGEN_ALIGN_STATICALLY 0 00347 #ifndef EIGEN_DISABLE_UNALIGNED_ARRAY_ASSERT 00348 #define EIGEN_DISABLE_UNALIGNED_ARRAY_ASSERT 00349 #endif 00350 #endif 00351 00352 #ifdef EIGEN_DEFAULT_TO_ROW_MAJOR 00353 #define EIGEN_DEFAULT_MATRIX_STORAGE_ORDER_OPTION RowMajor 00354 #else 00355 #define EIGEN_DEFAULT_MATRIX_STORAGE_ORDER_OPTION ColMajor 00356 #endif 00357 00358 #ifndef EIGEN_DEFAULT_DENSE_INDEX_TYPE 00359 #define EIGEN_DEFAULT_DENSE_INDEX_TYPE std::ptrdiff_t 00360 #endif 00361 00362 // A Clang feature extension to determine compiler features. 00363 // We use it to determine 'cxx_rvalue_references' 00364 #ifndef __has_feature 00365 # define __has_feature(x) 0 00366 #endif 00367 00368 // Do we support r-value references? 00369 #if (__has_feature(cxx_rvalue_references) || \ 00370 (defined(__cplusplus) && __cplusplus >= 201103L) || \ 00371 (defined(_MSC_VER) && _MSC_VER >= 1600)) 00372 #define EIGEN_HAVE_RVALUE_REFERENCES 00373 #endif 00374 00375 00376 // Cross compiler wrapper around LLVM's __has_builtin 00377 #ifdef __has_builtin 00378 # define EIGEN_HAS_BUILTIN(x) __has_builtin(x) 00379 #else 00380 # define EIGEN_HAS_BUILTIN(x) 0 00381 #endif 00382 00383 /** Allows to disable some optimizations which might affect the accuracy of the result. 00384 * Such optimization are enabled by default, and set EIGEN_FAST_MATH to 0 to disable them. 00385 * They currently include: 00386 * - single precision Cwise::sin() and Cwise::cos() when SSE vectorization is enabled. 00387 */ 00388 #ifndef EIGEN_FAST_MATH 00389 #define EIGEN_FAST_MATH 1 00390 #endif 00391 00392 #define EIGEN_DEBUG_VAR(x) std::cerr << #x << " = " << x << std::endl; 00393 00394 // concatenate two tokens 00395 #define EIGEN_CAT2(a,b) a ## b 00396 #define EIGEN_CAT(a,b) EIGEN_CAT2(a,b) 00397 00398 // convert a token to a string 00399 #define EIGEN_MAKESTRING2(a) #a 00400 #define EIGEN_MAKESTRING(a) EIGEN_MAKESTRING2(a) 00401 00402 // EIGEN_STRONG_INLINE is a stronger version of the inline, using __forceinline on MSVC, 00403 // but it still doesn't use GCC's always_inline. This is useful in (common) situations where MSVC needs forceinline 00404 // but GCC is still doing fine with just inline. 00405 #if (defined _MSC_VER) || (defined __INTEL_COMPILER) 00406 #define EIGEN_STRONG_INLINE __forceinline 00407 #else 00408 #define EIGEN_STRONG_INLINE inline 00409 #endif 00410 00411 // EIGEN_ALWAYS_INLINE is the stronget, it has the effect of making the function inline and adding every possible 00412 // attribute to maximize inlining. This should only be used when really necessary: in particular, 00413 // it uses __attribute__((always_inline)) on GCC, which most of the time is useless and can severely harm compile times. 00414 // FIXME with the always_inline attribute, 00415 // gcc 3.4.x reports the following compilation error: 00416 // Eval.h:91: sorry, unimplemented: inlining failed in call to 'const Eigen::Eval<Derived> Eigen::MatrixBase<Scalar, Derived>::eval() const' 00417 // : function body not available 00418 #if EIGEN_GNUC_AT_LEAST(4,0) 00419 #define EIGEN_ALWAYS_INLINE __attribute__((always_inline)) inline 00420 #else 00421 #define EIGEN_ALWAYS_INLINE EIGEN_STRONG_INLINE 00422 #endif 00423 00424 #if (defined __GNUC__) 00425 #define EIGEN_DONT_INLINE __attribute__((noinline)) 00426 #elif (defined _MSC_VER) 00427 #define EIGEN_DONT_INLINE __declspec(noinline) 00428 #else 00429 #define EIGEN_DONT_INLINE 00430 #endif 00431 00432 #if (defined __GNUC__) 00433 #define EIGEN_PERMISSIVE_EXPR __extension__ 00434 #else 00435 #define EIGEN_PERMISSIVE_EXPR 00436 #endif 00437 00438 // this macro allows to get rid of linking errors about multiply defined functions. 00439 // - static is not very good because it prevents definitions from different object files to be merged. 00440 // So static causes the resulting linked executable to be bloated with multiple copies of the same function. 00441 // - inline is not perfect either as it unwantedly hints the compiler toward inlining the function. 00442 #define EIGEN_DECLARE_FUNCTION_ALLOWING_MULTIPLE_DEFINITIONS 00443 #define EIGEN_DEFINE_FUNCTION_ALLOWING_MULTIPLE_DEFINITIONS inline 00444 00445 #ifdef NDEBUG 00446 # ifndef EIGEN_NO_DEBUG 00447 # define EIGEN_NO_DEBUG 00448 # endif 00449 #endif 00450 00451 // eigen_plain_assert is where we implement the workaround for the assert() bug in GCC <= 4.3, see bug 89 00452 #ifdef EIGEN_NO_DEBUG 00453 #define eigen_plain_assert(x) 00454 #else 00455 #if EIGEN_SAFE_TO_USE_STANDARD_ASSERT_MACRO 00456 namespace Eigen { 00457 namespace internal { 00458 inline bool copy_bool(bool b) { return b; } 00459 } 00460 } 00461 #define eigen_plain_assert(x) assert(x) 00462 #else 00463 // work around bug 89 00464 #include <cstdlib> // for abort 00465 #include <iostream> // for std::cerr 00466 00467 namespace Eigen { 00468 namespace internal { 00469 // trivial function copying a bool. Must be EIGEN_DONT_INLINE, so we implement it after including Eigen headers. 00470 // see bug 89. 00471 namespace { 00472 EIGEN_DONT_INLINE bool copy_bool(bool b) { return b; } 00473 } 00474 inline void assert_fail(const char *condition, const char *function, const char *file, int line) 00475 { 00476 std::cerr << "assertion failed: " << condition << " in function " << function << " at " << file << ":" << line << std::endl; 00477 abort(); 00478 } 00479 } 00480 } 00481 #define eigen_plain_assert(x) \ 00482 do { \ 00483 if(!Eigen::internal::copy_bool(x)) \ 00484 Eigen::internal::assert_fail(EIGEN_MAKESTRING(x), __PRETTY_FUNCTION__, __FILE__, __LINE__); \ 00485 } while(false) 00486 #endif 00487 #endif 00488 00489 // eigen_assert can be overridden 00490 #ifndef eigen_assert 00491 #define eigen_assert(x) eigen_plain_assert(x) 00492 #endif 00493 00494 #ifdef EIGEN_INTERNAL_DEBUGGING 00495 #define eigen_internal_assert(x) eigen_assert(x) 00496 #else 00497 #define eigen_internal_assert(x) 00498 #endif 00499 00500 #ifdef EIGEN_NO_DEBUG 00501 #define EIGEN_ONLY_USED_FOR_DEBUG(x) (void)x 00502 #else 00503 #define EIGEN_ONLY_USED_FOR_DEBUG(x) 00504 #endif 00505 00506 #ifndef EIGEN_NO_DEPRECATED_WARNING 00507 #if (defined __GNUC__) 00508 #define EIGEN_DEPRECATED __attribute__((deprecated)) 00509 #elif (defined _MSC_VER) 00510 #define EIGEN_DEPRECATED __declspec(deprecated) 00511 #else 00512 #define EIGEN_DEPRECATED 00513 #endif 00514 #else 00515 #define EIGEN_DEPRECATED 00516 #endif 00517 00518 #if (defined __GNUC__) 00519 #define EIGEN_UNUSED __attribute__((unused)) 00520 #else 00521 #define EIGEN_UNUSED 00522 #endif 00523 00524 // Suppresses 'unused variable' warnings. 00525 namespace Eigen { 00526 namespace internal { 00527 template<typename T> void ignore_unused_variable(const T&) {} 00528 } 00529 } 00530 #define EIGEN_UNUSED_VARIABLE(var) Eigen::internal::ignore_unused_variable(var); 00531 00532 #if !defined(EIGEN_ASM_COMMENT) 00533 #if (defined __GNUC__) && ( defined(__i386__) || defined(__x86_64__) ) 00534 #define EIGEN_ASM_COMMENT(X) __asm__("#" X) 00535 #else 00536 #define EIGEN_ASM_COMMENT(X) 00537 #endif 00538 #endif 00539 00540 /* EIGEN_ALIGN_TO_BOUNDARY(n) forces data to be n-byte aligned. This is used to satisfy SIMD requirements. 00541 * However, we do that EVEN if vectorization (EIGEN_VECTORIZE) is disabled, 00542 * so that vectorization doesn't affect binary compatibility. 00543 * 00544 * If we made alignment depend on whether or not EIGEN_VECTORIZE is defined, it would be impossible to link 00545 * vectorized and non-vectorized code. 00546 */ 00547 #if (defined __GNUC__) || (defined __PGI) || (defined __IBMCPP__) || (defined __ARMCC_VERSION) 00548 #define EIGEN_ALIGN_TO_BOUNDARY(n) __attribute__((aligned(n))) 00549 #elif (defined _MSC_VER) 00550 #define EIGEN_ALIGN_TO_BOUNDARY(n) __declspec(align(n)) 00551 #elif (defined __SUNPRO_CC) 00552 // FIXME not sure about this one: 00553 #define EIGEN_ALIGN_TO_BOUNDARY(n) __attribute__((aligned(n))) 00554 #else 00555 #error Please tell me what is the equivalent of __attribute__((aligned(n))) for your compiler 00556 #endif 00557 00558 #define EIGEN_ALIGN8 EIGEN_ALIGN_TO_BOUNDARY(8) 00559 #define EIGEN_ALIGN16 EIGEN_ALIGN_TO_BOUNDARY(16) 00560 00561 #if EIGEN_ALIGN_STATICALLY 00562 #define EIGEN_USER_ALIGN_TO_BOUNDARY(n) EIGEN_ALIGN_TO_BOUNDARY(n) 00563 #define EIGEN_USER_ALIGN16 EIGEN_ALIGN16 00564 #else 00565 #define EIGEN_USER_ALIGN_TO_BOUNDARY(n) 00566 #define EIGEN_USER_ALIGN16 00567 #endif 00568 00569 #ifdef EIGEN_DONT_USE_RESTRICT_KEYWORD 00570 #define EIGEN_RESTRICT 00571 #endif 00572 #ifndef EIGEN_RESTRICT 00573 #define EIGEN_RESTRICT __restrict 00574 #endif 00575 00576 #ifndef EIGEN_STACK_ALLOCATION_LIMIT 00577 // 131072 == 128 KB 00578 #define EIGEN_STACK_ALLOCATION_LIMIT 131072 00579 #endif 00580 00581 #ifndef EIGEN_DEFAULT_IO_FORMAT 00582 #ifdef EIGEN_MAKING_DOCS 00583 // format used in Eigen's documentation 00584 // needed to define it here as escaping characters in CMake add_definition's argument seems very problematic. 00585 #define EIGEN_DEFAULT_IO_FORMAT Eigen::IOFormat(3, 0, " ", "\n", "", "") 00586 #else 00587 #define EIGEN_DEFAULT_IO_FORMAT Eigen::IOFormat() 00588 #endif 00589 #endif 00590 00591 // just an empty macro ! 00592 #define EIGEN_EMPTY 00593 00594 #if defined(_MSC_VER) && (_MSC_VER < 1900) && (!defined(__INTEL_COMPILER)) 00595 #define EIGEN_INHERIT_ASSIGNMENT_EQUAL_OPERATOR(Derived) \ 00596 using Base::operator =; 00597 #elif defined(__clang__) // workaround clang bug (see http://forum.kde.org/viewtopic.php?f=74&t=102653) 00598 #define EIGEN_INHERIT_ASSIGNMENT_EQUAL_OPERATOR(Derived) \ 00599 using Base::operator =; \ 00600 EIGEN_STRONG_INLINE Derived& operator=(const Derived& other) { Base::operator=(other); return *this; } \ 00601 template <typename OtherDerived> \ 00602 EIGEN_STRONG_INLINE Derived& operator=(const DenseBase<OtherDerived>& other) { Base::operator=(other.derived()); return *this; } 00603 #else 00604 #define EIGEN_INHERIT_ASSIGNMENT_EQUAL_OPERATOR(Derived) \ 00605 using Base::operator =; \ 00606 EIGEN_STRONG_INLINE Derived& operator=(const Derived& other) \ 00607 { \ 00608 Base::operator=(other); \ 00609 return *this; \ 00610 } 00611 #endif 00612 00613 /** \internal 00614 * \brief Macro to manually inherit assignment operators. 00615 * This is necessary, because the implicitly defined assignment operator gets deleted when a custom operator= is defined. 00616 */ 00617 #define EIGEN_INHERIT_ASSIGNMENT_OPERATORS(Derived) EIGEN_INHERIT_ASSIGNMENT_EQUAL_OPERATOR(Derived) 00618 00619 /** 00620 * Just a side note. Commenting within defines works only by documenting 00621 * behind the object (via '!<'). Comments cannot be multi-line and thus 00622 * we have these extra long lines. What is confusing doxygen over here is 00623 * that we use '\' and basically have a bunch of typedefs with their 00624 * documentation in a single line. 00625 **/ 00626 00627 #define EIGEN_GENERIC_PUBLIC_INTERFACE(Derived) \ 00628 typedef typename Eigen::internal::traits<Derived>::Scalar Scalar; /*!< \brief Numeric type, e.g. float, double, int or std::complex<float>. */ \ 00629 typedef typename Eigen::NumTraits<Scalar>::Real RealScalar; /*!< \brief The underlying numeric type for composed scalar types. \details In cases where Scalar is e.g. std::complex<T>, T were corresponding to RealScalar. */ \ 00630 typedef typename Base::CoeffReturnType CoeffReturnType; /*!< \brief The return type for coefficient access. \details Depending on whether the object allows direct coefficient access (e.g. for a MatrixXd), this type is either 'const Scalar&' or simply 'Scalar' for objects that do not allow direct coefficient access. */ \ 00631 typedef typename Eigen::internal::nested<Derived>::type Nested; \ 00632 typedef typename Eigen::internal::traits<Derived>::StorageKind StorageKind; \ 00633 typedef typename Eigen::internal::traits<Derived>::Index Index; \ 00634 enum { RowsAtCompileTime = Eigen::internal::traits<Derived>::RowsAtCompileTime, \ 00635 ColsAtCompileTime = Eigen::internal::traits<Derived>::ColsAtCompileTime, \ 00636 Flags = Eigen::internal::traits<Derived>::Flags, \ 00637 CoeffReadCost = Eigen::internal::traits<Derived>::CoeffReadCost, \ 00638 SizeAtCompileTime = Base::SizeAtCompileTime, \ 00639 MaxSizeAtCompileTime = Base::MaxSizeAtCompileTime, \ 00640 IsVectorAtCompileTime = Base::IsVectorAtCompileTime }; 00641 00642 00643 #define EIGEN_DENSE_PUBLIC_INTERFACE(Derived) \ 00644 typedef typename Eigen::internal::traits<Derived>::Scalar Scalar; /*!< \brief Numeric type, e.g. float, double, int or std::complex<float>. */ \ 00645 typedef typename Eigen::NumTraits<Scalar>::Real RealScalar; /*!< \brief The underlying numeric type for composed scalar types. \details In cases where Scalar is e.g. std::complex<T>, T were corresponding to RealScalar. */ \ 00646 typedef typename Base::PacketScalar PacketScalar; \ 00647 typedef typename Base::CoeffReturnType CoeffReturnType; /*!< \brief The return type for coefficient access. \details Depending on whether the object allows direct coefficient access (e.g. for a MatrixXd), this type is either 'const Scalar&' or simply 'Scalar' for objects that do not allow direct coefficient access. */ \ 00648 typedef typename Eigen::internal::nested<Derived>::type Nested; \ 00649 typedef typename Eigen::internal::traits<Derived>::StorageKind StorageKind; \ 00650 typedef typename Eigen::internal::traits<Derived>::Index Index; \ 00651 enum { RowsAtCompileTime = Eigen::internal::traits<Derived>::RowsAtCompileTime, \ 00652 ColsAtCompileTime = Eigen::internal::traits<Derived>::ColsAtCompileTime, \ 00653 MaxRowsAtCompileTime = Eigen::internal::traits<Derived>::MaxRowsAtCompileTime, \ 00654 MaxColsAtCompileTime = Eigen::internal::traits<Derived>::MaxColsAtCompileTime, \ 00655 Flags = Eigen::internal::traits<Derived>::Flags, \ 00656 CoeffReadCost = Eigen::internal::traits<Derived>::CoeffReadCost, \ 00657 SizeAtCompileTime = Base::SizeAtCompileTime, \ 00658 MaxSizeAtCompileTime = Base::MaxSizeAtCompileTime, \ 00659 IsVectorAtCompileTime = Base::IsVectorAtCompileTime }; \ 00660 using Base::derived; \ 00661 using Base::const_cast_derived; 00662 00663 00664 #define EIGEN_PLAIN_ENUM_MIN(a,b) (((int)a <= (int)b) ? (int)a : (int)b) 00665 #define EIGEN_PLAIN_ENUM_MAX(a,b) (((int)a >= (int)b) ? (int)a : (int)b) 00666 00667 // EIGEN_SIZE_MIN_PREFER_DYNAMIC gives the min between compile-time sizes. 0 has absolute priority, followed by 1, 00668 // followed by Dynamic, followed by other finite values. The reason for giving Dynamic the priority over 00669 // finite values is that min(3, Dynamic) should be Dynamic, since that could be anything between 0 and 3. 00670 #define EIGEN_SIZE_MIN_PREFER_DYNAMIC(a,b) (((int)a == 0 || (int)b == 0) ? 0 \ 00671 : ((int)a == 1 || (int)b == 1) ? 1 \ 00672 : ((int)a == Dynamic || (int)b == Dynamic) ? Dynamic \ 00673 : ((int)a <= (int)b) ? (int)a : (int)b) 00674 00675 // EIGEN_SIZE_MIN_PREFER_FIXED is a variant of EIGEN_SIZE_MIN_PREFER_DYNAMIC comparing MaxSizes. The difference is that finite values 00676 // now have priority over Dynamic, so that min(3, Dynamic) gives 3. Indeed, whatever the actual value is 00677 // (between 0 and 3), it is not more than 3. 00678 #define EIGEN_SIZE_MIN_PREFER_FIXED(a,b) (((int)a == 0 || (int)b == 0) ? 0 \ 00679 : ((int)a == 1 || (int)b == 1) ? 1 \ 00680 : ((int)a == Dynamic && (int)b == Dynamic) ? Dynamic \ 00681 : ((int)a == Dynamic) ? (int)b \ 00682 : ((int)b == Dynamic) ? (int)a \ 00683 : ((int)a <= (int)b) ? (int)a : (int)b) 00684 00685 // see EIGEN_SIZE_MIN_PREFER_DYNAMIC. No need for a separate variant for MaxSizes here. 00686 #define EIGEN_SIZE_MAX(a,b) (((int)a == Dynamic || (int)b == Dynamic) ? Dynamic \ 00687 : ((int)a >= (int)b) ? (int)a : (int)b) 00688 00689 #define EIGEN_ADD_COST(a,b) int(a)==Dynamic || int(b)==Dynamic ? Dynamic : int(a)+int(b) 00690 00691 #define EIGEN_LOGICAL_XOR(a,b) (((a) || (b)) && !((a) && (b))) 00692 00693 #define EIGEN_IMPLIES(a,b) (!(a) || (b)) 00694 00695 #define EIGEN_MAKE_CWISE_BINARY_OP(METHOD,FUNCTOR) \ 00696 template<typename OtherDerived> \ 00697 EIGEN_STRONG_INLINE const CwiseBinaryOp<FUNCTOR<Scalar>, const Derived, const OtherDerived> \ 00698 (METHOD)(const EIGEN_CURRENT_STORAGE_BASE_CLASS<OtherDerived> &other) const \ 00699 { \ 00700 return CwiseBinaryOp<FUNCTOR<Scalar>, const Derived, const OtherDerived>(derived(), other.derived()); \ 00701 } 00702 00703 // the expression type of a cwise product 00704 #define EIGEN_CWISE_PRODUCT_RETURN_TYPE(LHS,RHS) \ 00705 CwiseBinaryOp< \ 00706 internal::scalar_product_op< \ 00707 typename internal::traits<LHS>::Scalar, \ 00708 typename internal::traits<RHS>::Scalar \ 00709 >, \ 00710 const LHS, \ 00711 const RHS \ 00712 > 00713 00714 #endif // EIGEN_MACROS_H
Generated on Tue Jul 12 2022 17:46:56 by
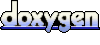