Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
ArrayCwiseUnaryOps.h
00001 00002 00003 /** \returns an expression of the coefficient-wise absolute value of \c *this 00004 * 00005 * Example: \include Cwise_abs.cpp 00006 * Output: \verbinclude Cwise_abs.out 00007 * 00008 * \sa abs2() 00009 */ 00010 EIGEN_STRONG_INLINE const CwiseUnaryOp<internal::scalar_abs_op<Scalar>, const Derived> 00011 abs() const 00012 { 00013 return derived(); 00014 } 00015 00016 /** \returns an expression of the coefficient-wise squared absolute value of \c *this 00017 * 00018 * Example: \include Cwise_abs2.cpp 00019 * Output: \verbinclude Cwise_abs2.out 00020 * 00021 * \sa abs(), square() 00022 */ 00023 EIGEN_STRONG_INLINE const CwiseUnaryOp<internal::scalar_abs2_op<Scalar>, const Derived> 00024 abs2() const 00025 { 00026 return derived(); 00027 } 00028 00029 /** \returns an expression of the coefficient-wise exponential of *this. 00030 * 00031 * Example: \include Cwise_exp.cpp 00032 * Output: \verbinclude Cwise_exp.out 00033 * 00034 * \sa pow(), log(), sin(), cos() 00035 */ 00036 inline const CwiseUnaryOp<internal::scalar_exp_op<Scalar>, const Derived> 00037 exp() const 00038 { 00039 return derived(); 00040 } 00041 00042 /** \returns an expression of the coefficient-wise logarithm of *this. 00043 * 00044 * Example: \include Cwise_log.cpp 00045 * Output: \verbinclude Cwise_log.out 00046 * 00047 * \sa exp() 00048 */ 00049 inline const CwiseUnaryOp<internal::scalar_log_op<Scalar>, const Derived> 00050 log() const 00051 { 00052 return derived(); 00053 } 00054 00055 /** \returns an expression of the coefficient-wise square root of *this. 00056 * 00057 * Example: \include Cwise_sqrt.cpp 00058 * Output: \verbinclude Cwise_sqrt.out 00059 * 00060 * \sa pow(), square() 00061 */ 00062 inline const CwiseUnaryOp<internal::scalar_sqrt_op<Scalar>, const Derived> 00063 sqrt() const 00064 { 00065 return derived(); 00066 } 00067 00068 /** \returns an expression of the coefficient-wise cosine of *this. 00069 * 00070 * Example: \include Cwise_cos.cpp 00071 * Output: \verbinclude Cwise_cos.out 00072 * 00073 * \sa sin(), acos() 00074 */ 00075 inline const CwiseUnaryOp<internal::scalar_cos_op<Scalar>, const Derived> 00076 cos() const 00077 { 00078 return derived(); 00079 } 00080 00081 00082 /** \returns an expression of the coefficient-wise sine of *this. 00083 * 00084 * Example: \include Cwise_sin.cpp 00085 * Output: \verbinclude Cwise_sin.out 00086 * 00087 * \sa cos(), asin() 00088 */ 00089 inline const CwiseUnaryOp<internal::scalar_sin_op<Scalar>, const Derived> 00090 sin() const 00091 { 00092 return derived(); 00093 } 00094 00095 /** \returns an expression of the coefficient-wise arc cosine of *this. 00096 * 00097 * Example: \include Cwise_acos.cpp 00098 * Output: \verbinclude Cwise_acos.out 00099 * 00100 * \sa cos(), asin() 00101 */ 00102 inline const CwiseUnaryOp<internal::scalar_acos_op<Scalar>, const Derived> 00103 acos() const 00104 { 00105 return derived(); 00106 } 00107 00108 /** \returns an expression of the coefficient-wise arc sine of *this. 00109 * 00110 * Example: \include Cwise_asin.cpp 00111 * Output: \verbinclude Cwise_asin.out 00112 * 00113 * \sa sin(), acos() 00114 */ 00115 inline const CwiseUnaryOp<internal::scalar_asin_op<Scalar>, const Derived> 00116 asin() const 00117 { 00118 return derived(); 00119 } 00120 00121 /** \returns an expression of the coefficient-wise tan of *this. 00122 * 00123 * Example: \include Cwise_tan.cpp 00124 * Output: \verbinclude Cwise_tan.out 00125 * 00126 * \sa cos(), sin() 00127 */ 00128 inline const CwiseUnaryOp<internal::scalar_tan_op<Scalar>, Derived> 00129 tan() const 00130 { 00131 return derived(); 00132 } 00133 00134 00135 /** \returns an expression of the coefficient-wise power of *this to the given exponent. 00136 * 00137 * Example: \include Cwise_pow.cpp 00138 * Output: \verbinclude Cwise_pow.out 00139 * 00140 * \sa exp(), log() 00141 */ 00142 inline const CwiseUnaryOp<internal::scalar_pow_op<Scalar>, const Derived> 00143 pow(const Scalar& exponent) const 00144 { 00145 return CwiseUnaryOp<internal::scalar_pow_op<Scalar>, const Derived> 00146 (derived(), internal::scalar_pow_op<Scalar>(exponent)); 00147 } 00148 00149 00150 /** \returns an expression of the coefficient-wise inverse of *this. 00151 * 00152 * Example: \include Cwise_inverse.cpp 00153 * Output: \verbinclude Cwise_inverse.out 00154 * 00155 * \sa operator/(), operator*() 00156 */ 00157 inline const CwiseUnaryOp<internal::scalar_inverse_op<Scalar>, const Derived> 00158 inverse() const 00159 { 00160 return derived(); 00161 } 00162 00163 /** \returns an expression of the coefficient-wise square of *this. 00164 * 00165 * Example: \include Cwise_square.cpp 00166 * Output: \verbinclude Cwise_square.out 00167 * 00168 * \sa operator/(), operator*(), abs2() 00169 */ 00170 inline const CwiseUnaryOp<internal::scalar_square_op<Scalar>, const Derived> 00171 square() const 00172 { 00173 return derived(); 00174 } 00175 00176 /** \returns an expression of the coefficient-wise cube of *this. 00177 * 00178 * Example: \include Cwise_cube.cpp 00179 * Output: \verbinclude Cwise_cube.out 00180 * 00181 * \sa square(), pow() 00182 */ 00183 inline const CwiseUnaryOp<internal::scalar_cube_op<Scalar>, const Derived> 00184 cube() const 00185 { 00186 return derived(); 00187 }
Generated on Tue Jul 12 2022 17:46:49 by
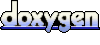