
BLE
Dependencies: ADT7410 BLE_API mbed nRF51822
main.cpp
00001 //********************** 00002 // BLE demo for mbed_HRM1017 00003 // 00004 // successful combination 00005 //#define REV1 // mbed=rev.97/BLE_API=rev.341/nRF51822=rev.111 00006 //#define REV2 // mbed=rev.101/BLE_API=rev.738/nRF51822=rev.385 00007 #define REV3 // mbed=rev.106/BLE_API=rev.798/nRF51822=rev.428 00008 // 00009 // (C)Copyright 2015 All rights reserved by Y.Onodera 00010 // http://einstlab.web.fc2.com 00011 //********************** 00012 #if defined(TARGET_HRM1017) 00013 #include "mbed.h" 00014 #include "ADT7410.h" 00015 #ifdef REV1 00016 #include "BLEDevice.h" // BLEライブラリのヘッダ BLE_API=rev.341 00017 #include "BatteryService.h" // BatteryServiceのヘッダ 00018 #include "HeartRateService.h" // HeartRateServiceのヘッダ 00019 #include "HealthThermometerService.h" // HealthThermometerServiceのヘッダ 00020 #endif 00021 #ifdef REV2 00022 #include "BLE.h" // BLEライブラリのヘッダ BLE_API=rev.738 00023 #include "BatteryService.h" // BatteryServiceのヘッダ 00024 #include "HeartRateService.h" // HeartRateServiceのヘッダ 00025 #include "HealthThermometerService.h" // HealthThermometerServiceのヘッダ 00026 #endif 00027 #ifdef REV3 00028 #include "ble/BLE.h" // BLEライブラリのヘッダ BLE_API=rev.798 00029 #include "ble/services/BatteryService.h" // BatteryServiceのヘッダ 00030 #include "ble/services/HeartRateService.h" // HeartRateServiceのヘッダ 00031 #include "ble/services/HealthThermometerService.h" // HealthThermometerServiceのヘッダ 00032 #endif 00033 00034 #define THERMO 00035 //#define HEART 00036 //#define BATTERY 00037 00038 BLEDevice ble; // BLEのインスタンス生成 00039 DigitalOut led1(LED1); 00040 DigitalOut led2(LED2); 00041 DigitalOut sw(P0_0); 00042 Ticker tick; 00043 #ifdef THERMO 00044 I2C i2c(P0_22,P0_20); 00045 ADT7410 temperature(i2c); 00046 #endif 00047 00048 #define DEVICE_NAME "mbed_BLE" 00049 // UUIDリスト 00050 #ifdef THERMO 00051 uint16_t uuid16_list[] = {GattService::UUID_HEALTH_THERMOMETER_SERVICE, GattService::UUID_BATTERY_SERVICE}; 00052 #endif 00053 #ifdef HEART 00054 uint16_t uuid16_list[] = {GattService::UUID_HEART_RATE_SERVICE, GattService::UUID_BATTERY_SERVICE}; 00055 #endif 00056 #ifdef BATTERY 00057 uint16_t uuid16_list[] = {GattService::UUID_BATTERY_SERVICE}; 00058 #endif 00059 bool tickFlag = false; 00060 00061 00062 // インターバルタイマー 00063 void tickerCallback(void) 00064 { 00065 led1 = !led1; 00066 tickFlag = true; 00067 } 00068 00069 00070 // BLE接続処理 00071 #ifdef REV1 00072 // BLE_API=rev.341 00073 void connectionCallback( 00074 Gap::Handle_t handle, 00075 Gap::addr_type_t peerAddrType, const Gap::address_t peerAddr, 00076 const Gap::ConnectionParams_t *params) 00077 #else 00078 // BLE_API=rev.738 00079 void connectionCallback( 00080 const Gap::ConnectionCallbackParams_t *params) 00081 #endif 00082 { 00083 led2 = 1; 00084 #ifdef REV3 00085 ble.gap().stopAdvertising(); 00086 #else 00087 ble.stopAdvertising(); 00088 #endif 00089 } 00090 00091 00092 // BLE切断処理 00093 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00094 { 00095 led2 = 0; 00096 ble.gap().startAdvertising(); 00097 } 00098 00099 00100 // BLE接続タイムアウト処理 00101 #ifdef REV1 00102 // BLE_API=rev.341 00103 void connectTimeoutCallback(void) 00104 { 00105 // to do 00106 // Sleep 00107 // __WFE(); 00108 // __SEV(); 00109 // __WFE(); 00110 // System-off 00111 // NRF_POWER->SYSTEMOFF = 1; 00112 } 00113 #else 00114 // BLE_API rev.738 00115 void connectTimeoutCallback(Gap::TimeoutSource_t source) 00116 { 00117 } 00118 #endif 00119 00120 00121 void initAdvertising(void) 00122 { 00123 00124 // フラグを設定 00125 // LE_LIMITED_DISCOVERABLE = 期間限定で検出可能デバイス 00126 // LE_GENERAL_DISCOVERABLE = 検出可能デバイス 00127 // BREDR_NOT_SUPPORTED = Bluetooth Classicは未サポート, BLEオンリー 00128 #ifdef REV3 00129 ble.gap().accumulateAdvertisingPayload( 00130 GapAdvertisingData::LE_GENERAL_DISCOVERABLE | GapAdvertisingData::BREDR_NOT_SUPPORTED); 00131 #else 00132 ble.accumulateAdvertisingPayload( 00133 GapAdvertisingData::LE_GENERAL_DISCOVERABLE | GapAdvertisingData::BREDR_NOT_SUPPORTED); 00134 #endif 00135 // UUIDリストを設定 00136 #ifdef REV3 00137 ble.gap().accumulateAdvertisingPayload( 00138 GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, 00139 (uint8_t *)uuid16_list, 00140 sizeof(uuid16_list)); 00141 #else 00142 ble.accumulateAdvertisingPayload( 00143 GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, 00144 (uint8_t *)uuid16_list, 00145 sizeof(uuid16_list)); 00146 #endif 00147 // デバイス名を設定(for GAP), default Device Name="nRF5x" 00148 // ble.setDeviceName(DEVICE_NAME); 00149 // デバイス名を設定(for Advertising) 00150 #ifdef REV3 00151 ble.gap().accumulateAdvertisingPayload( 00152 GapAdvertisingData::COMPLETE_LOCAL_NAME, 00153 (const uint8_t *)DEVICE_NAME, 00154 // strlen(DEVICE_NAME)); 00155 sizeof(DEVICE_NAME)); 00156 #else 00157 ble.accumulateAdvertisingPayload( 00158 GapAdvertisingData::COMPLETE_LOCAL_NAME, 00159 (const uint8_t *)DEVICE_NAME, 00160 // strlen(DEVICE_NAME)); 00161 sizeof(DEVICE_NAME)); 00162 #endif 00163 00164 // ADV_CONNECTABLE_UNDIRECTED = 接続可能デバイスに設定 00165 #ifdef REV3 00166 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00167 #else 00168 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00169 #endif 00170 00171 // アドバタイジング間隔の設定=100ms, 0.625ms単位 00172 #ifdef REV3 00173 ble.gap().setAdvertisingInterval(160); // 0.625ms * 160 = 100ms 00174 // ble.gap().setAdvertisingInterval(1600); // 0.625ms * 1600 = 1000ms 00175 #else 00176 ble.setAdvertisingInterval(160); // 0.625ms * 160 = 100ms 00177 // ble.setAdvertisingInterval(1600); // 0.625ms * 1600 = 1000ms 00178 #endif 00179 00180 // Appearance 00181 #ifdef THERMO 00182 #ifdef REV3 00183 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::THERMOMETER_EAR); 00184 #else 00185 ble.accumulateAdvertisingPayload(GapAdvertisingData::THERMOMETER_EAR); 00186 #endif 00187 #endif 00188 #ifdef HEART 00189 #ifdef REV3 00190 // ble.gap().setAppearance(ENERIC_HEART_RATE_SENSOR); 00191 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_HEART_RATE_SENSOR); 00192 #else 00193 // ble.setAppearance(ENERIC_HEART_RATE_SENSOR); 00194 ble.accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_HEART_RATE_SENSOR); 00195 #endif 00196 #endif 00197 // アドバタイジングタイムアウト=180sec(デフォルトは0でタイムアウトなし) 00198 // ble.setAdvertisingTimeout(180); 00199 00200 // アドバタイジング開始 00201 #ifdef REV3 00202 ble.gap().startAdvertising(); 00203 #else 00204 ble.startAdvertising(); 00205 #endif 00206 } 00207 00208 00209 int main(void) 00210 { 00211 led1 = 0; 00212 led2 = 0; 00213 sw = 1; // off 00214 00215 ble.init(); // BLE(nRF51822)の初期化 00216 // Valid values are -40, -20, -16, -12, -8, -4, 0, 4) 00217 if(ble.setTxPower(-40)!=BLE_ERROR_NONE) 00218 led2 = 1; 00219 00220 // バッテリ残量のイベント関数を設定 00221 tick.attach(&tickerCallback, 1); 00222 00223 // BLEのイベント関数を設定 00224 #ifdef REV3 00225 ble.gap().onConnection(connectionCallback); 00226 ble.gap().onDisconnection(disconnectionCallback); 00227 ble.gap().onTimeout(connectTimeoutCallback); 00228 #else 00229 ble.onConnection(connectionCallback); 00230 ble.onDisconnection(disconnectionCallback); 00231 ble.onTimeout(connectTimeoutCallback); 00232 #endif 00233 00234 // BatteryServiceのインスタンス生成=サービスを登録 00235 uint8_t batt=0; 00236 BatteryService bs(ble); 00237 00238 #ifdef THERMO 00239 // HeathTermometerServiceのインスタンス生成=サービスを登録 00240 float currentTemperature = 0.0; 00241 HealthThermometerService ts(ble, currentTemperature, HealthThermometerService::LOCATION_EAR); 00242 #endif 00243 #ifdef HEART 00244 // HeartRateServiceのインスタンス生成=サービスを登録 00245 uint8_t hrmCounter = 100; 00246 HeartRateService hr(ble, hrmCounter, HeartRateService::LOCATION_FINGER); 00247 #endif 00248 00249 // アドバタイジングの設定 00250 initAdvertising(); 00251 00252 // イベント待ちループ 00253 while(true) { 00254 ble.waitForEvent(); 00255 if(tickFlag){ 00256 // バッテリ残量の取り込み 00257 if(++batt>100)batt=0; 00258 // バッテリ残量のデータ更新 00259 bs.updateBatteryLevel(batt); 00260 #ifdef THERMO 00261 sw = 0; // on 00262 // currentTemperature-=0.1; 00263 wait_ms(100); 00264 currentTemperature=temperature.value()/128.0; 00265 ts.updateTemperature(currentTemperature); 00266 sw = 1; // off 00267 #endif 00268 #ifdef HEART 00269 hr.updateHeartRate(hrmCounter); 00270 #endif 00271 tickFlag = false; 00272 } 00273 } 00274 } 00275 00276 #endif 00277
Generated on Thu Jul 21 2022 15:00:32 by
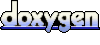