NeoPixel NeoMatrix 8x8 RGB LED library. Product found at https://www.adafruit.com/products/1487. NeoCore.s from Allen Wild's NeoStrip library: http://developer.mbed.org/users/aswild/code/NeoStrip/
Fork of NeoStrip by
NeoArr Class Reference
NeoArr objects manage the buffering and assigning of addressable NeoPixels. More...
#include <NeoMatrix.h>
Public Member Functions | |
NeoArr (PinName pin, int N) | |
Create a NeoArr object. | |
void | setBrightness (float bright) |
Sets the brightness of the entire array. | |
void | setPixel (int idx, int x, int y, int color) |
Set a single pixel in the array to a specific color. | |
void | setPixel (int idx, int x, int y, uint8_t red, uint8_t green, uint8_t blue) |
Set a single pixel in the array to a specific color with reg, blue, and blue values in seperate arguments. | |
void | drawLine (int idx, int x1, int y1, int x2, int y2, int color) |
Draws a line of a specific color between any two points. | |
void | drawLine (int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue) |
Draws a line of a specific color between any two points with reg, blue, and blue values in seperate arguments. | |
void | drawRect (int idx, int x1, int y1, int x2, int y2, int color) |
Draws a rectangle outline of a specific color given two opposite corner points. | |
void | drawRect (int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue) |
Draws a rectangle outline of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments. | |
void | drawFilledRect (int idx, int x1, int y1, int x2, int y2, int color) |
Draws a filled rectangle of a specific color given two opposite corner points. | |
void | drawFilledRect (int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue) |
Draws a filled rectangle of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments. | |
void | fillScreen (int idx, int color) |
Fills the entire array screen with one color. | |
void | fillScreen (int idx, uint8_t red, uint8_t green, uint8_t blue) |
Fills the entire array screen with one color with reg, blue, and blue values in seperate arguments. | |
void | drawTriangle (int idx, int x1, int y1, int x2, int y2, int x3, int y3, int color) |
Draws a triangle outline of a specific color given three corner points. | |
void | drawTriangle (int idx, int x1, int y1, int x2, int y2, int x3, int y3, uint8_t red, uint8_t green, uint8_t blue) |
Draws a triangle outline of a specific color given three corner points with reg, blue, and blue values in seperate arguments. | |
void | drawFilledTriangle (int idx, int x1, int y1, int x2, int y2, int x3, int y3, int color) |
Draws a filled triangle of a specific color given three corner points. | |
void | drawFilledTriangle (int idx, int x1, int y1, int x2, int y2, int x3, int y3, uint8_t red, uint8_t green, uint8_t blue) |
Draws a filled triangle of a specific color given three corner points with reg, blue, and blue values in seperate arguments. | |
void | drawChar (int idx, int x, int y, char c, int color) |
Draws a single 6x8 character on the array. | |
void | drawChar (int idx, int x, int y, char c, uint8_t red, uint8_t green, uint8_t blue) |
Draws a single 6x8 character on the array. | |
void | showImage (int idx, const int *colors) |
Displays a 64 bit image on board idx. | |
void | clear () |
Clears all pixels on all boards (sets them all to 0) | |
void | write () |
Write the colors out to the strip; this method must be called to see any hardware effect. |
Detailed Description
NeoArr objects manage the buffering and assigning of addressable NeoPixels.
Definition at line 32 of file NeoMatrix.h.
Constructor & Destructor Documentation
NeoArr | ( | PinName | pin, |
int | N | ||
) |
Create a NeoArr object.
- Parameters:
-
pin The mbed data pin name N The number of arrays chained together.
Definition at line 39 of file NeoMatrix.cpp.
Member Function Documentation
void clear | ( | ) |
Clears all pixels on all boards (sets them all to 0)
Definition at line 270 of file NeoMatrix.cpp.
void drawChar | ( | int | idx, |
int | x, | ||
int | y, | ||
char | c, | ||
int | color | ||
) |
Draws a single 6x8 character on the array.
The character will be pulled from font.h
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x The x co-ordinate of the lower left point of the character y The y co-ordinate of the lower left point of the character c The character to be drawn color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 224 of file NeoMatrix.cpp.
void drawChar | ( | int | idx, |
int | x, | ||
int | y, | ||
char | c, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a single 6x8 character on the array.
The character will be pulled from font.h with reg, blue, and blue values in seperate arguments
Definition at line 233 of file NeoMatrix.cpp.
void drawFilledRect | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a filled rectangle of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments.
Definition at line 147 of file NeoMatrix.cpp.
void drawFilledRect | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draws a filled rectangle of a specific color given two opposite corner points.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x1 The first x co-ordinate of a corner y1 The first y co-ordinate of a corner x2 The second x co-ordinate of a corner y2 The second y co-ordinate of a corner color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 138 of file NeoMatrix.cpp.
void drawFilledTriangle | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
int | color | ||
) |
Draws a filled triangle of a specific color given three corner points.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x1 The first x co-ordinate of a corner y1 The first y co-ordinate of a corner x2 The second x co-ordinate of a corner y2 The second y co-ordinate of a corner x3 The third x co-ordinate of a corner y3 The third y co-ordinate of a corner color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 196 of file NeoMatrix.cpp.
void drawFilledTriangle | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a filled triangle of a specific color given three corner points with reg, blue, and blue values in seperate arguments.
Definition at line 205 of file NeoMatrix.cpp.
void drawLine | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draws a line of a specific color between any two points.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x1 The first x co-ordinate of the line y1 The first y co-ordinate of the line x2 The second x co-ordinate of the line y2 The second y co-ordinate of the line color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 79 of file NeoMatrix.cpp.
void drawLine | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a line of a specific color between any two points with reg, blue, and blue values in seperate arguments.
Definition at line 88 of file NeoMatrix.cpp.
void drawRect | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draws a rectangle outline of a specific color given two opposite corner points.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x1 The first x co-ordinate of a corner y1 The first y co-ordinate of a corner x2 The second x co-ordinate of a corner y2 The second y co-ordinate of a corner color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 104 of file NeoMatrix.cpp.
void drawRect | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a rectangle outline of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments.
Definition at line 113 of file NeoMatrix.cpp.
void drawTriangle | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Draws a triangle outline of a specific color given three corner points with reg, blue, and blue values in seperate arguments.
Definition at line 187 of file NeoMatrix.cpp.
void drawTriangle | ( | int | idx, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
int | color | ||
) |
Draws a triangle outline of a specific color given three corner points.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x1 The first x co-ordinate of a corner y1 The first y co-ordinate of a corner x2 The second x co-ordinate of a corner y2 The second y co-ordinate of a corner x3 The third x co-ordinate of a corner y3 The third y co-ordinate of a corner color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 178 of file NeoMatrix.cpp.
void fillScreen | ( | int | idx, |
int | color | ||
) |
Fills the entire array screen with one color.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 161 of file NeoMatrix.cpp.
void fillScreen | ( | int | idx, |
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Fills the entire array screen with one color with reg, blue, and blue values in seperate arguments.
Definition at line 170 of file NeoMatrix.cpp.
void setBrightness | ( | float | bright ) |
Sets the brightness of the entire array.
All functions using set pixel are affected by this value. If a higher brightness is set, an external power supply may be necessary
The default brightness is 0.5
- Parameters:
-
bright The brightness scaled between 0 and 1.0
Definition at line 55 of file NeoMatrix.cpp.
void setPixel | ( | int | idx, |
int | x, | ||
int | y, | ||
int | color | ||
) |
Set a single pixel in the array to a specific color.
- Parameters:
-
idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) x The x co-ordinate of the pixel y The y co-ordinate of the pixel color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red)
Definition at line 61 of file NeoMatrix.cpp.
void setPixel | ( | int | idx, |
int | x, | ||
int | y, | ||
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Set a single pixel in the array to a specific color with reg, blue, and blue values in seperate arguments.
Definition at line 70 of file NeoMatrix.cpp.
void showImage | ( | int | idx, |
const int * | colors | ||
) |
Displays a 64 bit image on board idx.
- Parameters:
-
idx The index of the board colors An array of length 64 containing the image to be displayed
Definition at line 258 of file NeoMatrix.cpp.
void write | ( | ) |
Write the colors out to the strip; this method must be called to see any hardware effect.
This function disables interrupts while the strip data is being sent, each pixel takes approximately 30us to send, plus a 50us reset pulse at the end.
Definition at line 280 of file NeoMatrix.cpp.
Generated on Tue Jul 12 2022 15:51:38 by
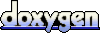