NeoPixel NeoMatrix 8x8 RGB LED library. Product found at https://www.adafruit.com/products/1487. NeoCore.s from Allen Wild's NeoStrip library: http://developer.mbed.org/users/aswild/code/NeoStrip/
Fork of NeoStrip by
NeoMatrix.h
00001 /** 00002 * NeoMatrix.h 00003 * 00004 * Taylor Powell 00005 * March 2015 00006 * 00007 * Library for the 8x8 grid array of Neopixel LEDs 00008 * 00009 */ 00010 00011 00012 #ifndef NEOARRAY_H 00013 #define NEOARRAY_H 00014 00015 #ifndef TARGET_LPC1768 00016 #error NeoArray only supports the NXP LPC1768 00017 #endif 00018 00019 // NeoColor struct definition to hold 24 bit 00020 // color data for each pixel, in GRB order 00021 typedef struct _NeoColor 00022 { 00023 uint8_t green; 00024 uint8_t red; 00025 uint8_t blue; 00026 } NeoColor; 00027 00028 /** 00029 * NeoArr objects manage the buffering and assigning of 00030 * addressable NeoPixels 00031 */ 00032 class NeoArr 00033 { 00034 public: 00035 00036 /** 00037 * Create a NeoArr object 00038 * 00039 * @param pin The mbed data pin name 00040 * @param N The number of arrays chained together. 00041 */ 00042 NeoArr(PinName pin, int N); 00043 00044 /** 00045 * Sets the brightness of the entire array. All functions using set pixel are affected by this value. 00046 * If a higher brightness is set, an external power supply may be necessary 00047 * 00048 * The default brightness is 0.5 00049 * 00050 * @param bright The brightness scaled between 0 and 1.0 00051 */ 00052 void setBrightness(float bright); 00053 00054 /** 00055 * Set a single pixel in the array to a specific color. 00056 * 00057 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00058 * @param x The x co-ordinate of the pixel 00059 * @param y The y co-ordinate of the pixel 00060 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00061 */ 00062 void setPixel(int idx, int x, int y, int color); 00063 00064 /** 00065 * Set a single pixel in the array to a specific color with reg, blue, and blue values in seperate arguments 00066 */ 00067 void setPixel(int idx, int x, int y, uint8_t red, uint8_t green, uint8_t blue); 00068 00069 /** 00070 * Draws a line of a specific color between any two points 00071 * 00072 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00073 * @param x1 The first x co-ordinate of the line 00074 * @param y1 The first y co-ordinate of the line 00075 * @param x2 The second x co-ordinate of the line 00076 * @param y2 The second y co-ordinate of the line 00077 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00078 */ 00079 00080 void drawLine(int idx, int x1, int y1, int x2, int y2, int color); 00081 00082 /** 00083 * Draws a line of a specific color between any two points with reg, blue, and blue values in seperate arguments 00084 */ 00085 void drawLine(int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue); 00086 /** 00087 * Draws a rectangle outline of a specific color given two opposite corner points 00088 * 00089 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00090 * @param x1 The first x co-ordinate of a corner 00091 * @param y1 The first y co-ordinate of a corner 00092 * @param x2 The second x co-ordinate of a corner 00093 * @param y2 The second y co-ordinate of a corner 00094 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00095 */ 00096 00097 void drawRect(int idx, int x1, int y1, int x2, int y2, int color); 00098 00099 /** 00100 * Draws a rectangle outline of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments 00101 */ 00102 void drawRect(int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue); 00103 00104 /** 00105 * Draws a filled rectangle of a specific color given two opposite corner points 00106 * 00107 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00108 * @param x1 The first x co-ordinate of a corner 00109 * @param y1 The first y co-ordinate of a corner 00110 * @param x2 The second x co-ordinate of a corner 00111 * @param y2 The second y co-ordinate of a corner 00112 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00113 */ 00114 00115 void drawFilledRect(int idx, int x1, int y1, int x2, int y2, int color); 00116 00117 /** 00118 * Draws a filled rectangle of a specific color given two opposite corner points with reg, blue, and blue values in seperate arguments 00119 */ 00120 void drawFilledRect(int idx, int x1, int y1, int x2, int y2, uint8_t red, uint8_t green, uint8_t blue); 00121 00122 /** 00123 * Fills the entire array screen with one color 00124 * 00125 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00126 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00127 */ 00128 void fillScreen(int idx, int color); 00129 00130 /** 00131 * Fills the entire array screen with one color with reg, blue, and blue values in seperate arguments 00132 * 00133 */ 00134 void fillScreen(int idx, uint8_t red,uint8_t green, uint8_t blue); 00135 00136 00137 /** 00138 * Draws a triangle outline of a specific color given three corner points 00139 * 00140 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00141 * @param x1 The first x co-ordinate of a corner 00142 * @param y1 The first y co-ordinate of a corner 00143 * @param x2 The second x co-ordinate of a corner 00144 * @param y2 The second y co-ordinate of a corner 00145 * @param x3 The third x co-ordinate of a corner 00146 * @param y3 The third y co-ordinate of a corner 00147 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00148 */ 00149 void drawTriangle(int idx, int x1, int y1, int x2, int y2, int x3, int y3, int color); 00150 00151 /** 00152 * Draws a triangle outline of a specific color given three corner points with reg, blue, and blue values in seperate arguments 00153 */ 00154 void drawTriangle(int idx, int x1, int y1, int x2, int y2, int x3, int y3, uint8_t red, uint8_t green, uint8_t blue); 00155 /** 00156 * Draws a filled triangle of a specific color given three corner points 00157 * 00158 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00159 * @param x1 The first x co-ordinate of a corner 00160 * @param y1 The first y co-ordinate of a corner 00161 * @param x2 The second x co-ordinate of a corner 00162 * @param y2 The second y co-ordinate of a corner 00163 * @param x3 The third x co-ordinate of a corner 00164 * @param y3 The third y co-ordinate of a corner 00165 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00166 */ 00167 00168 void drawFilledTriangle(int idx, int x1, int y1, int x2, int y2, int x3, int y3, int color); 00169 00170 /** 00171 * Draws a filled triangle of a specific color given three corner points with reg, blue, and blue values in seperate arguments 00172 */ 00173 void drawFilledTriangle(int idx, int x1, int y1, int x2, int y2, int x3, int y3, uint8_t red, uint8_t green, uint8_t blue); 00174 00175 /** 00176 * Draws a single 6x8 character on the array. The character will be pulled from font.h 00177 * 00178 * @param idx The index of the array to write on. Indexing starts at 0 (use 0 if there is only one array) 00179 * @param x The x co-ordinate of the lower left point of the character 00180 * @param y The y co-ordinate of the lower left point of the character 00181 * @para c The character to be drawn 00182 * @param color Integer golding a 24 bit color using RGB hex indexing (e.g. 0xff0000 is red) 00183 */ 00184 void drawChar(int idx, int x, int y, char c, int color); 00185 00186 /** 00187 * Draws a single 6x8 character on the array. The character will be pulled from font.h with reg, blue, and blue values in seperate arguments 00188 */ 00189 void drawChar(int idx, int x, int y, char c, uint8_t red, uint8_t green, uint8_t blue); 00190 00191 00192 /** 00193 * Displays a 64 bit image on board idx 00194 * 00195 * @param idx The index of the board 00196 * @param colors An array of length 64 containing the image to be displayed 00197 */ 00198 void showImage(int idx, const int *colors); 00199 00200 /** 00201 * Clears all pixels on all boards (sets them all to 0) 00202 */ 00203 void clear(); 00204 00205 /** 00206 * Write the colors out to the strip; this method must be called 00207 * to see any hardware effect. 00208 * 00209 * This function disables interrupts while the strip data is being sent, 00210 * each pixel takes approximately 30us to send, plus a 50us reset pulse 00211 * at the end. 00212 */ 00213 void write(); 00214 00215 00216 protected: 00217 NeoColor *arr; // pixel data buffer modified by setPixel() and used by neo_out() 00218 int N; // the number of pixels in the strip 00219 int Nbytes; // the number of bytes of pixel data (always N*3) 00220 float bright; // the master strip brightness 00221 gpio_t gpio; // gpio struct for initialization and getting register addresses 00222 }; 00223 00224 #endif 00225 00226
Generated on Tue Jul 12 2022 15:51:38 by
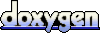