AM2321 Temperature and Humidity Sensor mbed library
Dependents: AM2321_Example mbed_vfd_thermometer
AM2321.h
00001 /** 00002 * AM2321 (Aosong Guangzhou Electronics) 00003 * Temperature and Humidity Sensor mbed library 00004 * Last update : 2014/05/06 00005 */ 00006 00007 #ifndef __AM2321_H__ 00008 #define __AM2321_H__ 00009 00010 /** AM2321 (Aosong Guangzhou Electronics) 00011 * Temperature and Humidity Sensor mbed library 00012 * 00013 * Example: 00014 * @code 00015 * #include "mbed.h" 00016 * #include "AM2321.h" 00017 * 00018 * Serial pc(USBTX, USBRX); // Tx, Rx 00019 * AM2321 am2321(p28, p27); // SDA, SCL 00020 * 00021 * int main() 00022 * { 00023 * while(1) 00024 * { 00025 * if(am2321.poll()) 00026 * { 00027 * pc.printf(":%05u,%.1f,%.1f\n" 00028 * , count++ 00029 * , am2321.getTemperature() 00030 * , am2321.getHumidity() 00031 * ); 00032 * } 00033 * 00034 * wait(0.5); 00035 * } 00036 * } 00037 * @endcode 00038 */ 00039 class AM2321 00040 { 00041 private: 00042 typedef struct tagRESULT 00043 { 00044 float temperature; 00045 float humidity; 00046 }RESULT; 00047 00048 public: 00049 /** Constructor 00050 * @param sda [in] I2C Pin name (SDA) 00051 * @param scl [in] I2C Pin name (SCL) 00052 */ 00053 AM2321(PinName sda, PinName scl); 00054 00055 /** Read current temperature and humidity from AM2321 00056 * @return result (true=success) 00057 */ 00058 bool poll(); 00059 00060 /** Get last read temperature value 00061 * @return temperature value (degress) 00062 */ 00063 float getTemperature(void) const; 00064 00065 /** Get last read humidity value 00066 * @return humidity value (%RH) 00067 */ 00068 float getHumidity(void) const; 00069 00070 private: 00071 float getLogicalValue(uint16_t regVal) const; 00072 uint16_t calcCRC16(const uint8_t* src, int len) const; 00073 00074 I2C _i2c; 00075 RESULT _result; 00076 }; 00077 00078 00079 #endif // __AM2321_H__
Generated on Tue Jul 12 2022 19:43:48 by
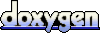