
ex
Fork of mbed-os-example-mbed5-blinky by
Speex encoder and decoder
This is the Speex codec itself. More...
Data Structures | |
struct | SpeexMode |
Struct defining a Speex mode. More... | |
Typedefs | |
typedef void *(* | encoder_init_func )(const struct SpeexMode *mode) |
Encoder state initialization function. | |
typedef void(* | encoder_destroy_func )(void *st) |
Encoder state destruction function. | |
typedef int(* | encode_func )(void *state, void *in, SpeexBits *bits) |
Main encoding function. | |
typedef int(* | encoder_ctl_func )(void *state, int request, void *ptr) |
Function for controlling the encoder options. | |
typedef void *(* | decoder_init_func )(const struct SpeexMode *mode) |
Decoder state initialization function. | |
typedef void(* | decoder_destroy_func )(void *st) |
Decoder state destruction function. | |
typedef int(* | decode_func )(void *state, SpeexBits *bits, void *out) |
Main decoding function. | |
typedef int(* | decoder_ctl_func )(void *state, int request, void *ptr) |
Function for controlling the decoder options. | |
typedef int(* | mode_query_func )(const void *mode, int request, void *ptr) |
Query function for a mode. | |
typedef struct SpeexMode | SpeexMode |
Struct defining a Speex mode. | |
Functions | |
void * | speex_encoder_init (const SpeexMode *mode) |
Returns a handle to a newly created Speex encoder state structure. | |
void | speex_encoder_destroy (void *state) |
Frees all resources associated to an existing Speex encoder state. | |
int | speex_encode (void *state, float *in, SpeexBits *bits) |
Uses an existing encoder state to encode one frame of speech pointed to by "in". | |
int | speex_encode_int (void *state, spx_int16_t *in, SpeexBits *bits) |
Uses an existing encoder state to encode one frame of speech pointed to by "in". | |
int | speex_encoder_ctl (void *state, int request, void *ptr) |
Used like the ioctl function to control the encoder parameters. | |
void * | speex_decoder_init (const SpeexMode *mode) |
Returns a handle to a newly created decoder state structure. | |
void | speex_decoder_destroy (void *state) |
Frees all resources associated to an existing decoder state. | |
int | speex_decode (void *state, SpeexBits *bits, float *out) |
Uses an existing decoder state to decode one frame of speech from bit-stream bits. | |
int | speex_decode_int (void *state, SpeexBits *bits, spx_int16_t *out) |
Uses an existing decoder state to decode one frame of speech from bit-stream bits. | |
int | speex_decoder_ctl (void *state, int request, void *ptr) |
Used like the ioctl function to control the encoder parameters. | |
int | speex_mode_query (const SpeexMode *mode, int request, void *ptr) |
Query function for mode information. | |
int | speex_lib_ctl (int request, void *ptr) |
Functions for controlling the behavior of libspeex. | |
const SpeexMode * | speex_lib_get_mode (int mode) |
Obtain one of the modes available. | |
Variables | |
const SpeexMode | speex_nb_mode |
Default narrowband mode. | |
const SpeexMode | speex_wb_mode |
Default wideband mode. | |
const SpeexMode | speex_uwb_mode |
Default "ultra-wideband" mode. | |
const SpeexMode | speex_nb_48k_mode |
4.8 kbps narrowband mode | |
const SpeexMode *const | speex_mode_list [SPEEX_NB_MODES] |
List of all modes available. |
Detailed Description
This is the Speex codec itself.
Typedef Documentation
typedef int(* decode_func)(void *state, SpeexBits *bits, void *out) |
typedef int(* decoder_ctl_func)(void *state, int request, void *ptr) |
typedef void(* decoder_destroy_func)(void *st) |
typedef void*(* decoder_init_func)(const struct SpeexMode *mode) |
typedef int(* encode_func)(void *state, void *in, SpeexBits *bits) |
typedef int(* encoder_ctl_func)(void *state, int request, void *ptr) |
typedef void(* encoder_destroy_func)(void *st) |
typedef void*(* encoder_init_func)(const struct SpeexMode *mode) |
typedef int(* mode_query_func)(const void *mode, int request, void *ptr) |
Function Documentation
int speex_decode | ( | void * | state, |
SpeexBits * | bits, | ||
float * | out | ||
) |
Uses an existing decoder state to decode one frame of speech from bit-stream bits.
The output speech is saved written to out.
- Parameters:
-
state Decoder state bits Bit-stream from which to decode the frame (NULL if the packet was lost) out Where to write the decoded frame
- Returns:
- return status (0 for no error, -1 for end of stream, -2 corrupt stream)
int speex_decode_int | ( | void * | state, |
SpeexBits * | bits, | ||
spx_int16_t * | out | ||
) |
Uses an existing decoder state to decode one frame of speech from bit-stream bits.
The output speech is saved written to out.
- Parameters:
-
state Decoder state bits Bit-stream from which to decode the frame (NULL if the packet was lost) out Where to write the decoded frame
- Returns:
- return status (0 for no error, -1 for end of stream, -2 corrupt stream)
int speex_decoder_ctl | ( | void * | state, |
int | request, | ||
void * | ptr | ||
) |
Used like the ioctl function to control the encoder parameters.
- Parameters:
-
state Decoder state request ioctl-type request (one of the SPEEX_* macros) ptr Data exchanged to-from function
- Returns:
- 0 if no error, -1 if request in unknown, -2 for invalid parameter
void speex_decoder_destroy | ( | void * | state ) |
Frees all resources associated to an existing decoder state.
- Parameters:
-
state State to be destroyed
void* speex_decoder_init | ( | const SpeexMode * | mode ) |
Returns a handle to a newly created decoder state structure.
For now, the mode argument can be &nb_mode or &wb_mode . In the future, more modes may be added. Note that for now if you have more than one channels to decode, you need one state per channel.
- Parameters:
-
mode Speex mode (one of speex_nb_mode or speex_wb_mode)
- Returns:
- A newly created decoder state or NULL if state allocation fails
int speex_encode | ( | void * | state, |
float * | in, | ||
SpeexBits * | bits | ||
) |
Uses an existing encoder state to encode one frame of speech pointed to by "in".
The encoded bit-stream is saved in "bits".
- Parameters:
-
state Encoder state in Frame that will be encoded with a +-2^15 range. This data MAY be overwritten by the encoder and should be considered uninitialised after the call. bits Bit-stream where the data will be written
- Returns:
- 0 if frame needs not be transmitted (DTX only), 1 otherwise
int speex_encode_int | ( | void * | state, |
spx_int16_t * | in, | ||
SpeexBits * | bits | ||
) |
Uses an existing encoder state to encode one frame of speech pointed to by "in".
The encoded bit-stream is saved in "bits".
- Parameters:
-
state Encoder state in Frame that will be encoded with a +-2^15 range bits Bit-stream where the data will be written
- Returns:
- 0 if frame needs not be transmitted (DTX only), 1 otherwise
int speex_encoder_ctl | ( | void * | state, |
int | request, | ||
void * | ptr | ||
) |
Used like the ioctl function to control the encoder parameters.
- Parameters:
-
state Encoder state request ioctl-type request (one of the SPEEX_* macros) ptr Data exchanged to-from function
- Returns:
- 0 if no error, -1 if request in unknown, -2 for invalid parameter
void speex_encoder_destroy | ( | void * | state ) |
Frees all resources associated to an existing Speex encoder state.
- Parameters:
-
state Encoder state to be destroyed
void* speex_encoder_init | ( | const SpeexMode * | mode ) |
Returns a handle to a newly created Speex encoder state structure.
For now, the "mode" argument can be &nb_mode or &wb_mode . In the future, more modes may be added. Note that for now if you have more than one channels to encode, you need one state per channel.
- Parameters:
-
mode The mode to use (either speex_nb_mode or speex_wb.mode)
- Returns:
- A newly created encoder state or NULL if state allocation fails
int speex_lib_ctl | ( | int | request, |
void * | ptr | ||
) |
Functions for controlling the behavior of libspeex.
- Parameters:
-
request ioctl-type request (one of the SPEEX_LIB_* macros) ptr Data exchanged to-from function
- Returns:
- 0 if no error, -1 if request in unknown, -2 for invalid parameter
const SpeexMode* speex_lib_get_mode | ( | int | mode ) |
Obtain one of the modes available.
int speex_mode_query | ( | const SpeexMode * | mode, |
int | request, | ||
void * | ptr | ||
) |
Query function for mode information.
- Parameters:
-
mode Speex mode request ioctl-type request (one of the SPEEX_* macros) ptr Data exchanged to-from function
- Returns:
- 0 if no error, -1 if request in unknown, -2 for invalid parameter
Variable Documentation
const SpeexMode* const speex_mode_list[SPEEX_NB_MODES] |
List of all modes available.
const SpeexMode speex_nb_48k_mode |
4.8 kbps narrowband mode
const SpeexMode speex_nb_mode |
Default narrowband mode.
const SpeexMode speex_uwb_mode |
Default "ultra-wideband" mode.
const SpeexMode speex_wb_mode |
Default wideband mode.
Generated on Tue Jul 12 2022 16:28:54 by
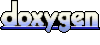