
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
speex.h
Go to the documentation of this file.
00001 /* Copyright (C) 2002-2006 Jean-Marc Valin*/ 00002 /** 00003 @file speex.h 00004 @brief Describes the different modes of the codec 00005 */ 00006 /* 00007 Redistribution and use in source and binary forms, with or without 00008 modification, are permitted provided that the following conditions 00009 are met: 00010 00011 - Redistributions of source code must retain the above copyright 00012 notice, this list of conditions and the following disclaimer. 00013 00014 - Redistributions in binary form must reproduce the above copyright 00015 notice, this list of conditions and the following disclaimer in the 00016 documentation and/or other materials provided with the distribution. 00017 00018 - Neither the name of the Xiph.org Foundation nor the names of its 00019 contributors may be used to endorse or promote products derived from 00020 this software without specific prior written permission. 00021 00022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE FOUNDATION OR 00026 CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00027 EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00028 PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00030 LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00031 NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00032 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 00034 */ 00035 00036 #ifndef SPEEX_H 00037 #define SPEEX_H 00038 /** @defgroup Codec Speex encoder and decoder 00039 * This is the Speex codec itself. 00040 * @{ 00041 */ 00042 00043 #include "speex/speex_bits.h" 00044 #include "speex/speex_types.h" 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /* Values allowed for *ctl() requests */ 00051 00052 /** Set enhancement on/off (decoder only) */ 00053 #define SPEEX_SET_ENH 0 00054 /** Get enhancement state (decoder only) */ 00055 #define SPEEX_GET_ENH 1 00056 00057 /*Would be SPEEX_SET_FRAME_SIZE, but it's (currently) invalid*/ 00058 /** Obtain frame size used by encoder/decoder */ 00059 #define SPEEX_GET_FRAME_SIZE 3 00060 00061 /** Set quality value */ 00062 #define SPEEX_SET_QUALITY 4 00063 /** Get current quality setting */ 00064 /* #define SPEEX_GET_QUALITY 5 -- Doesn't make much sense, does it? */ 00065 00066 /** Set sub-mode to use */ 00067 #define SPEEX_SET_MODE 6 00068 /** Get current sub-mode in use */ 00069 #define SPEEX_GET_MODE 7 00070 00071 /** Set low-band sub-mode to use (wideband only)*/ 00072 #define SPEEX_SET_LOW_MODE 8 00073 /** Get current low-band mode in use (wideband only)*/ 00074 #define SPEEX_GET_LOW_MODE 9 00075 00076 /** Set high-band sub-mode to use (wideband only)*/ 00077 #define SPEEX_SET_HIGH_MODE 10 00078 /** Get current high-band mode in use (wideband only)*/ 00079 #define SPEEX_GET_HIGH_MODE 11 00080 00081 /** Set VBR on (1) or off (0) */ 00082 #define SPEEX_SET_VBR 12 00083 /** Get VBR status (1 for on, 0 for off) */ 00084 #define SPEEX_GET_VBR 13 00085 00086 /** Set quality value for VBR encoding (0-10) */ 00087 #define SPEEX_SET_VBR_QUALITY 14 00088 /** Get current quality value for VBR encoding (0-10) */ 00089 #define SPEEX_GET_VBR_QUALITY 15 00090 00091 /** Set complexity of the encoder (0-10) */ 00092 #define SPEEX_SET_COMPLEXITY 16 00093 /** Get current complexity of the encoder (0-10) */ 00094 #define SPEEX_GET_COMPLEXITY 17 00095 00096 /** Set bit-rate used by the encoder (or lower) */ 00097 #define SPEEX_SET_BITRATE 18 00098 /** Get current bit-rate used by the encoder or decoder */ 00099 #define SPEEX_GET_BITRATE 19 00100 00101 /** Define a handler function for in-band Speex request*/ 00102 #define SPEEX_SET_HANDLER 20 00103 00104 /** Define a handler function for in-band user-defined request*/ 00105 #define SPEEX_SET_USER_HANDLER 22 00106 00107 /** Set sampling rate used in bit-rate computation */ 00108 #define SPEEX_SET_SAMPLING_RATE 24 00109 /** Get sampling rate used in bit-rate computation */ 00110 #define SPEEX_GET_SAMPLING_RATE 25 00111 00112 /** Reset the encoder/decoder memories to zero*/ 00113 #define SPEEX_RESET_STATE 26 00114 00115 /** Get VBR info (mostly used internally) */ 00116 #define SPEEX_GET_RELATIVE_QUALITY 29 00117 00118 /** Set VAD status (1 for on, 0 for off) */ 00119 #define SPEEX_SET_VAD 30 00120 00121 /** Get VAD status (1 for on, 0 for off) */ 00122 #define SPEEX_GET_VAD 31 00123 00124 /** Set Average Bit-Rate (ABR) to n bits per seconds */ 00125 #define SPEEX_SET_ABR 32 00126 /** Get Average Bit-Rate (ABR) setting (in bps) */ 00127 #define SPEEX_GET_ABR 33 00128 00129 /** Set DTX status (1 for on, 0 for off) */ 00130 #define SPEEX_SET_DTX 34 00131 /** Get DTX status (1 for on, 0 for off) */ 00132 #define SPEEX_GET_DTX 35 00133 00134 /** Set submode encoding in each frame (1 for yes, 0 for no, setting to no breaks the standard) */ 00135 #define SPEEX_SET_SUBMODE_ENCODING 36 00136 /** Get submode encoding in each frame */ 00137 #define SPEEX_GET_SUBMODE_ENCODING 37 00138 00139 /*#define SPEEX_SET_LOOKAHEAD 38*/ 00140 /** Returns the lookahead used by Speex */ 00141 #define SPEEX_GET_LOOKAHEAD 39 00142 00143 /** Sets tuning for packet-loss concealment (expected loss rate) */ 00144 #define SPEEX_SET_PLC_TUNING 40 00145 /** Gets tuning for PLC */ 00146 #define SPEEX_GET_PLC_TUNING 41 00147 00148 /** Sets the max bit-rate allowed in VBR mode */ 00149 #define SPEEX_SET_VBR_MAX_BITRATE 42 00150 /** Gets the max bit-rate allowed in VBR mode */ 00151 #define SPEEX_GET_VBR_MAX_BITRATE 43 00152 00153 /** Turn on/off input/output high-pass filtering */ 00154 #define SPEEX_SET_HIGHPASS 44 00155 /** Get status of input/output high-pass filtering */ 00156 #define SPEEX_GET_HIGHPASS 45 00157 00158 /** Get "activity level" of the last decoded frame, i.e. 00159 now much damage we cause if we remove the frame */ 00160 #define SPEEX_GET_ACTIVITY 47 00161 00162 00163 /* Preserving compatibility:*/ 00164 /** Equivalent to SPEEX_SET_ENH */ 00165 #define SPEEX_SET_PF 0 00166 /** Equivalent to SPEEX_GET_ENH */ 00167 #define SPEEX_GET_PF 1 00168 00169 00170 00171 00172 /* Values allowed for mode queries */ 00173 /** Query the frame size of a mode */ 00174 #define SPEEX_MODE_FRAME_SIZE 0 00175 00176 /** Query the size of an encoded frame for a particular sub-mode */ 00177 #define SPEEX_SUBMODE_BITS_PER_FRAME 1 00178 00179 00180 00181 /** Get major Speex version */ 00182 #define SPEEX_LIB_GET_MAJOR_VERSION 1 00183 /** Get minor Speex version */ 00184 #define SPEEX_LIB_GET_MINOR_VERSION 3 00185 /** Get micro Speex version */ 00186 #define SPEEX_LIB_GET_MICRO_VERSION 5 00187 /** Get extra Speex version */ 00188 #define SPEEX_LIB_GET_EXTRA_VERSION 7 00189 /** Get Speex version string */ 00190 #define SPEEX_LIB_GET_VERSION_STRING 9 00191 00192 /*#define SPEEX_LIB_SET_ALLOC_FUNC 10 00193 #define SPEEX_LIB_GET_ALLOC_FUNC 11 00194 #define SPEEX_LIB_SET_FREE_FUNC 12 00195 #define SPEEX_LIB_GET_FREE_FUNC 13 00196 00197 #define SPEEX_LIB_SET_WARNING_FUNC 14 00198 #define SPEEX_LIB_GET_WARNING_FUNC 15 00199 #define SPEEX_LIB_SET_ERROR_FUNC 16 00200 #define SPEEX_LIB_GET_ERROR_FUNC 17 00201 */ 00202 00203 /** Number of defined modes in Speex */ 00204 #define SPEEX_NB_MODES 3 00205 00206 /** modeID for the defined narrowband mode */ 00207 #define SPEEX_MODEID_NB 0 00208 00209 /** modeID for the defined wideband mode */ 00210 #define SPEEX_MODEID_WB 1 00211 00212 /** modeID for the defined ultra-wideband mode */ 00213 #define SPEEX_MODEID_UWB 2 00214 00215 #ifdef EPIC_48K 00216 /** modeID for the Epic 48K mode */ 00217 #define SPEEX_MODEID_NB_48K 1000 00218 #endif 00219 00220 struct SpeexMode; 00221 00222 00223 /* Prototypes for mode function pointers */ 00224 00225 /** Encoder state initialization function */ 00226 typedef void *(*encoder_init_func)(const struct SpeexMode *mode); 00227 00228 /** Encoder state destruction function */ 00229 typedef void (*encoder_destroy_func)(void *st); 00230 00231 /** Main encoding function */ 00232 typedef int (*encode_func)(void *state, void *in, SpeexBits *bits); 00233 00234 /** Function for controlling the encoder options */ 00235 typedef int (*encoder_ctl_func)(void *state, int request, void *ptr); 00236 00237 /** Decoder state initialization function */ 00238 typedef void *(*decoder_init_func)(const struct SpeexMode *mode); 00239 00240 /** Decoder state destruction function */ 00241 typedef void (*decoder_destroy_func)(void *st); 00242 00243 /** Main decoding function */ 00244 typedef int (*decode_func)(void *state, SpeexBits *bits, void *out); 00245 00246 /** Function for controlling the decoder options */ 00247 typedef int (*decoder_ctl_func)(void *state, int request, void *ptr); 00248 00249 00250 /** Query function for a mode */ 00251 typedef int (*mode_query_func)(const void *mode, int request, void *ptr); 00252 00253 /** Struct defining a Speex mode */ 00254 typedef struct SpeexMode { 00255 /** Pointer to the low-level mode data */ 00256 const void *mode; 00257 00258 /** Pointer to the mode query function */ 00259 mode_query_func query; 00260 00261 /** The name of the mode (you should not rely on this to identify the mode)*/ 00262 const char *modeName; 00263 00264 /**ID of the mode*/ 00265 int modeID; 00266 00267 /**Version number of the bitstream (incremented every time we break 00268 bitstream compatibility*/ 00269 int bitstream_version; 00270 00271 /** Pointer to encoder initialization function */ 00272 encoder_init_func enc_init; 00273 00274 /** Pointer to encoder destruction function */ 00275 encoder_destroy_func enc_destroy; 00276 00277 /** Pointer to frame encoding function */ 00278 encode_func enc; 00279 00280 /** Pointer to decoder initialization function */ 00281 decoder_init_func dec_init; 00282 00283 /** Pointer to decoder destruction function */ 00284 decoder_destroy_func dec_destroy; 00285 00286 /** Pointer to frame decoding function */ 00287 decode_func dec; 00288 00289 /** ioctl-like requests for encoder */ 00290 encoder_ctl_func enc_ctl; 00291 00292 /** ioctl-like requests for decoder */ 00293 decoder_ctl_func dec_ctl; 00294 00295 } SpeexMode; 00296 00297 /** 00298 * Returns a handle to a newly created Speex encoder state structure. For now, 00299 * the "mode" argument can be &nb_mode or &wb_mode . In the future, more modes 00300 * may be added. Note that for now if you have more than one channels to 00301 * encode, you need one state per channel. 00302 * 00303 * @param mode The mode to use (either speex_nb_mode or speex_wb.mode) 00304 * @return A newly created encoder state or NULL if state allocation fails 00305 */ 00306 void *speex_encoder_init(const SpeexMode *mode); 00307 00308 /** Frees all resources associated to an existing Speex encoder state. 00309 * @param state Encoder state to be destroyed */ 00310 void speex_encoder_destroy(void *state); 00311 00312 /** Uses an existing encoder state to encode one frame of speech pointed to by 00313 "in". The encoded bit-stream is saved in "bits". 00314 @param state Encoder state 00315 @param in Frame that will be encoded with a +-2^15 range. This data MAY be 00316 overwritten by the encoder and should be considered uninitialised 00317 after the call. 00318 @param bits Bit-stream where the data will be written 00319 @return 0 if frame needs not be transmitted (DTX only), 1 otherwise 00320 */ 00321 int speex_encode(void *state, float *in, SpeexBits *bits); 00322 00323 /** Uses an existing encoder state to encode one frame of speech pointed to by 00324 "in". The encoded bit-stream is saved in "bits". 00325 @param state Encoder state 00326 @param in Frame that will be encoded with a +-2^15 range 00327 @param bits Bit-stream where the data will be written 00328 @return 0 if frame needs not be transmitted (DTX only), 1 otherwise 00329 */ 00330 int speex_encode_int(void *state, spx_int16_t *in, SpeexBits *bits); 00331 00332 /** Used like the ioctl function to control the encoder parameters 00333 * 00334 * @param state Encoder state 00335 * @param request ioctl-type request (one of the SPEEX_* macros) 00336 * @param ptr Data exchanged to-from function 00337 * @return 0 if no error, -1 if request in unknown, -2 for invalid parameter 00338 */ 00339 int speex_encoder_ctl(void *state, int request, void *ptr); 00340 00341 00342 /** Returns a handle to a newly created decoder state structure. For now, 00343 * the mode argument can be &nb_mode or &wb_mode . In the future, more modes 00344 * may be added. Note that for now if you have more than one channels to 00345 * decode, you need one state per channel. 00346 * 00347 * @param mode Speex mode (one of speex_nb_mode or speex_wb_mode) 00348 * @return A newly created decoder state or NULL if state allocation fails 00349 */ 00350 void *speex_decoder_init(const SpeexMode *mode); 00351 00352 /** Frees all resources associated to an existing decoder state. 00353 * 00354 * @param state State to be destroyed 00355 */ 00356 void speex_decoder_destroy(void *state); 00357 00358 /** Uses an existing decoder state to decode one frame of speech from 00359 * bit-stream bits. The output speech is saved written to out. 00360 * 00361 * @param state Decoder state 00362 * @param bits Bit-stream from which to decode the frame (NULL if the packet was lost) 00363 * @param out Where to write the decoded frame 00364 * @return return status (0 for no error, -1 for end of stream, -2 corrupt stream) 00365 */ 00366 int speex_decode(void *state, SpeexBits *bits, float *out); 00367 00368 /** Uses an existing decoder state to decode one frame of speech from 00369 * bit-stream bits. The output speech is saved written to out. 00370 * 00371 * @param state Decoder state 00372 * @param bits Bit-stream from which to decode the frame (NULL if the packet was lost) 00373 * @param out Where to write the decoded frame 00374 * @return return status (0 for no error, -1 for end of stream, -2 corrupt stream) 00375 */ 00376 int speex_decode_int(void *state, SpeexBits *bits, spx_int16_t *out); 00377 00378 /** Used like the ioctl function to control the encoder parameters 00379 * 00380 * @param state Decoder state 00381 * @param request ioctl-type request (one of the SPEEX_* macros) 00382 * @param ptr Data exchanged to-from function 00383 * @return 0 if no error, -1 if request in unknown, -2 for invalid parameter 00384 */ 00385 int speex_decoder_ctl(void *state, int request, void *ptr); 00386 00387 00388 /** Query function for mode information 00389 * 00390 * @param mode Speex mode 00391 * @param request ioctl-type request (one of the SPEEX_* macros) 00392 * @param ptr Data exchanged to-from function 00393 * @return 0 if no error, -1 if request in unknown, -2 for invalid parameter 00394 */ 00395 int speex_mode_query(const SpeexMode *mode, int request, void *ptr); 00396 00397 /** Functions for controlling the behavior of libspeex 00398 * @param request ioctl-type request (one of the SPEEX_LIB_* macros) 00399 * @param ptr Data exchanged to-from function 00400 * @return 0 if no error, -1 if request in unknown, -2 for invalid parameter 00401 */ 00402 int speex_lib_ctl(int request, void *ptr); 00403 00404 /** Default narrowband mode */ 00405 extern const SpeexMode speex_nb_mode; 00406 00407 /** Default wideband mode */ 00408 extern const SpeexMode speex_wb_mode; 00409 00410 /** Default "ultra-wideband" mode */ 00411 extern const SpeexMode speex_uwb_mode; 00412 00413 #ifdef EPIC_48K 00414 /** 4.8 kbps narrowband mode */ 00415 extern const SpeexMode speex_nb_48k_mode; 00416 #endif 00417 00418 /** List of all modes available */ 00419 extern const SpeexMode * const speex_mode_list[SPEEX_NB_MODES]; 00420 00421 /** Obtain one of the modes available */ 00422 const SpeexMode * speex_lib_get_mode (int mode); 00423 00424 #ifdef __cplusplus 00425 } 00426 #endif 00427 00428 /** @}*/ 00429 #endif
Generated on Tue Jul 12 2022 16:28:54 by
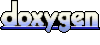