EaPaper Library for EM027BS013
Dependencies: EM027BS013 TFT_fonts
Fork of EaEpaper by
EaEpaper Class Reference
Display control class, based on GraphicsDisplay and TextDisplay. More...
#include <EaEpaper.h>
Inherits GraphicsDisplay.
Public Member Functions | |
EaEpaper (PinName sec03_SpiSCK, PinName sec04_SpiMOSI, PinName sec05_SpiMISO, PinName sec06_EpdCS, PinName sec07_EpdBusy, PinName sec08_EpdBorder, PinName sec09_I2cSCL, PinName sec10_I2cSDA, PinName sec11_FlashCS, PinName sec12_EpdReset, PinName sec13_EpdPanelOn, PinName sec14_EpdDischarge, const char *name="E_Paper") | |
Constructor. | |
virtual int | width () |
Get the width of the screen in pixel. | |
virtual int | height () |
Get the height of the screen in pixel. | |
void | clear () |
Clear the display. | |
void | write_disp (void) |
Write image buffer to display. | |
virtual void | pixel (int x, int y, int color) |
set or reset a single pixel | |
virtual void | cls (void) |
Fill the screen with white. | |
void | line (int x0, int y0, int x1, int y1, int color) |
draw a 1 pixel line | |
void | rect (int x0, int y0, int x1, int y1, int color) |
draw a rect | |
void | fillrect (int x0, int y0, int x1, int y1, int color) |
draw a filled rect | |
void | circle (int x0, int y0, int r, int color) |
draw a circle | |
void | fillcircle (int x0, int y0, int r, int color) |
draw a filled circle | |
void | setmode (int mode) |
set drawing mode | |
virtual void | locate (int x, int y) |
setup cursor position | |
virtual int | columns () |
calculate the max number of char in a line | |
virtual int | rows () |
calculate the max number of columns | |
virtual int | _putc (int value) |
put a char on the screen | |
virtual void | character (int x, int y, int c) |
paint a character on given position out of the active font to the screen buffer | |
void | set_font (unsigned char *f) |
select the font to use | |
void | print_bm (Bitmap bm, int x, int y) |
print bitmap to buffer | |
virtual bool | claim (FILE *stream) |
redirect output from a stream (stoud, sterr) to display |
Detailed Description
Display control class, based on GraphicsDisplay and TextDisplay.
Example with pinning for KL25Z:
#include "mbed.h" #include "EaEpaper.h" #include "Arial28x28.h" #include "Arial12x12.h" #include "font_big.h" #include "graphics.h" // the E-Paper board from embedded artists has a LM75 temp sensor to compensate the temperature effect. If it is cold the display reacts slower. // The LM75 need a I2C -> 2 pins : sda and scl // The display data is written via SPI -> 3 pins : mosi,miso,sck // There are also some control signals // The pwm pin has to be connected to a PWM enabled pin : pwm // The other signals are connected to normal IO`s // EaEpaper epaper(PTD7, // PWR_CTRL PTD6, // BORDER PTE31, // DISCHARGE PTA17, // RESET_DISP PTA16, // BUSY PTC17, // SSEL PTD4, // PWM PTD2,PTD3,PTD1, // MOSI,MISO,SCLK PTE0,PTE1); // SDA,SCL int main() { epaper.cls(); // clear screen epaper.set_font((unsigned char*) Arial28x28); // select the font epaper.locate(5,20); // set cursor epaper.printf("Hello Mbed"); // print text epaper.rect(3,15,150,50,1); // draw frame epaper.write_disp(); // update screen
Definition at line 89 of file EaEpaper.h.
Constructor & Destructor Documentation
EaEpaper | ( | PinName | sec03_SpiSCK, |
PinName | sec04_SpiMOSI, | ||
PinName | sec05_SpiMISO, | ||
PinName | sec06_EpdCS, | ||
PinName | sec07_EpdBusy, | ||
PinName | sec08_EpdBorder, | ||
PinName | sec09_I2cSCL, | ||
PinName | sec10_I2cSDA, | ||
PinName | sec11_FlashCS, | ||
PinName | sec12_EpdReset, | ||
PinName | sec13_EpdPanelOn, | ||
PinName | sec14_EpdDischarge, | ||
const char * | name = "E_Paper" |
||
) |
Constructor.
Definition at line 11 of file EaEpaper.cpp.
Member Function Documentation
int _putc | ( | int | value ) | [virtual] |
put a char on the screen
- Parameters:
-
value char to print
- Returns:
- printed char
Definition at line 252 of file EaEpaper.cpp.
void character | ( | int | x, |
int | y, | ||
int | c | ||
) | [virtual] |
paint a character on given position out of the active font to the screen buffer
- Parameters:
-
x x-position (top left) y y-position c char code
Definition at line 267 of file EaEpaper.cpp.
void circle | ( | int | x0, |
int | y0, | ||
int | r, | ||
int | color | ||
) |
draw a circle
- Parameters:
-
x0,y0 center r radius color : 0 white, 1 black *
Definition at line 192 of file EaEpaper.cpp.
bool claim | ( | FILE * | stream ) | [virtual, inherited] |
redirect output from a stream (stoud, sterr) to display
- Parameters:
-
stream stream that shall be redirected to the TextDisplay
Definition at line 65 of file TextDisplay.cpp.
void clear | ( | ) |
Clear the display.
Definition at line 55 of file EaEpaper.cpp.
void cls | ( | void | ) | [virtual] |
Fill the screen with white.
Definition at line 87 of file EaEpaper.cpp.
int columns | ( | ) | [virtual] |
calculate the max number of char in a line
- Returns:
- max columns depends on actual font size
Definition at line 240 of file EaEpaper.cpp.
void fillcircle | ( | int | x0, |
int | y0, | ||
int | r, | ||
int | color | ||
) |
draw a filled circle
- Parameters:
-
x0,y0 center r radius color : 0 white, 1 black *
Definition at line 211 of file EaEpaper.cpp.
void fillrect | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
int | color | ||
) |
draw a filled rect
- Parameters:
-
x0,y0 top left corner x1,y1 down right corner color : 0 white, 1 black
Definition at line 169 of file EaEpaper.cpp.
int height | ( | ) | [virtual] |
Get the height of the screen in pixel.
- Returns:
- height of screen in pixel
Definition at line 48 of file EaEpaper.cpp.
void line | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
int | color | ||
) |
draw a 1 pixel line
- Parameters:
-
x0,y0 start point x1,y1 stop point color : 0 white, 1 black
Definition at line 93 of file EaEpaper.cpp.
void locate | ( | int | x, |
int | y | ||
) | [virtual] |
setup cursor position
- Parameters:
-
x x-position (top left) y y-position
Definition at line 233 of file EaEpaper.cpp.
void pixel | ( | int | x, |
int | y, | ||
int | color | ||
) | [virtual] |
set or reset a single pixel
- Parameters:
-
x horizontal position y vertical position color : 0 white, 1 black
Definition at line 70 of file EaEpaper.cpp.
void print_bm | ( | Bitmap | bm, |
int | x, | ||
int | y | ||
) |
print bitmap to buffer
- Parameters:
-
bm struct Bitmap in flash x x start y y start
Definition at line 314 of file EaEpaper.cpp.
void rect | ( | int | x0, |
int | y0, | ||
int | x1, | ||
int | y1, | ||
int | color | ||
) |
draw a rect
- Parameters:
-
x0,y0 top left corner x1,y1 down right corner color : 0 white, 1 black
Definition at line 152 of file EaEpaper.cpp.
int rows | ( | ) | [virtual] |
calculate the max number of columns
- Returns:
- max column depends on actual font size
Definition at line 246 of file EaEpaper.cpp.
void set_font | ( | unsigned char * | f ) |
select the font to use
- Parameters:
-
f pointer to font array
font array can created with GLCD Font Creator from http://www.mikroe.com you have to add 4 parameter at the beginning of the font array to use:
- the number of byte / char
- the vertial size in pixel
- the horizontal size in pixel
- the number of byte per vertical line you also have to change the array to char[]
Definition at line 309 of file EaEpaper.cpp.
void setmode | ( | int | mode ) |
set drawing mode
- Parameters:
-
NORMAL : paint normal color, XOR : paint XOR of current pixels
Definition at line 227 of file EaEpaper.cpp.
int width | ( | ) | [virtual] |
Get the width of the screen in pixel.
- Parameters:
-
@returns width of screen in pixel
Definition at line 43 of file EaEpaper.cpp.
void write_disp | ( | void | ) |
Write image buffer to display.
Definition at line 61 of file EaEpaper.cpp.
Generated on Thu Jul 14 2022 12:47:28 by
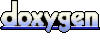