EaPaper Library for EM027BS013
Dependencies: EM027BS013 TFT_fonts
Fork of EaEpaper by
EaEpaper.h
00001 /* mbed library for 264*176 pixel 2.7 INCH E-PAPER DISPLAY from Pervasive Displays 00002 * Copyright (c) 2013 Peter Drescher - DC2PD 00003 * 00004 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00005 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00006 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00007 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00008 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00009 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00010 * THE SOFTWARE. 00011 */ 00012 00013 00014 // 09.11.2013 initial Version 00015 00016 #ifndef EAEPAPER_H 00017 #define EAEPAPER_H 00018 00019 /** 00020 * Includes 00021 */ 00022 #include "mbed.h" 00023 #include "EM027BS013.h" 00024 #include "GraphicsDisplay.h" 00025 00026 // we have to double buffer the display 00027 #define EA_IMG_BUF_SZ (5808) // 264 x 176 / 8 = 5808 00028 00029 /** Draw mode 00030 * NORMAl 00031 * XOR set pixel by xor of the screen 00032 */ 00033 enum {NORMAL,XOR}; 00034 00035 /** Bitmap 00036 */ 00037 struct Bitmap{ 00038 int xSize; 00039 int ySize; 00040 int Byte_in_Line; 00041 char* data; 00042 }; 00043 00044 /* color definitions */ 00045 #define Black 0x0 00046 #define White 0x1 00047 00048 /** Display control class, based on GraphicsDisplay and TextDisplay 00049 * 00050 * Example with pinning for KL25Z: 00051 * @code 00052 * #include "mbed.h" 00053 * #include "EaEpaper.h" 00054 * #include "Arial28x28.h" 00055 * #include "Arial12x12.h" 00056 * #include "font_big.h" 00057 * #include "graphics.h" 00058 00059 * // the E-Paper board from embedded artists has a LM75 temp sensor to compensate the temperature effect. If it is cold the display reacts slower. 00060 * // The LM75 need a I2C -> 2 pins : sda and scl 00061 * // The display data is written via SPI -> 3 pins : mosi,miso,sck 00062 * // There are also some control signals 00063 * // The pwm pin has to be connected to a PWM enabled pin : pwm 00064 * // The other signals are connected to normal IO`s 00065 * // 00066 * EaEpaper epaper(PTD7, // PWR_CTRL 00067 * PTD6, // BORDER 00068 * PTE31, // DISCHARGE 00069 * PTA17, // RESET_DISP 00070 * PTA16, // BUSY 00071 * PTC17, // SSEL 00072 * PTD4, // PWM 00073 * PTD2,PTD3,PTD1, // MOSI,MISO,SCLK 00074 * PTE0,PTE1); // SDA,SCL 00075 * 00076 * int main() { 00077 * 00078 * epaper.cls(); // clear screen 00079 * epaper.set_font((unsigned char*) Arial28x28); // select the font 00080 * epaper.locate(5,20); // set cursor 00081 * epaper.printf("Hello Mbed"); // print text 00082 * epaper.rect(3,15,150,50,1); // draw frame 00083 * epaper.write_disp(); // update screen 00084 * 00085 * @endcode 00086 */ 00087 00088 00089 class EaEpaper : public GraphicsDisplay { 00090 00091 public: 00092 00093 /** 00094 * Constructor. 00095 */ 00096 EaEpaper::EaEpaper(PinName sec03_SpiSCK, 00097 PinName sec04_SpiMOSI, 00098 PinName sec05_SpiMISO, 00099 PinName sec06_EpdCS, 00100 PinName sec07_EpdBusy, 00101 PinName sec08_EpdBorder, 00102 PinName sec09_I2cSCL, 00103 PinName sec10_I2cSDA, 00104 PinName sec11_FlashCS, 00105 PinName sec12_EpdReset, 00106 PinName sec13_EpdPanelOn, 00107 PinName sec14_EpdDischarge, // I2C 00108 const char* name ="E_Paper"); 00109 00110 /** Get the width of the screen in pixel 00111 * 00112 * @param 00113 * @returns width of screen in pixel 00114 * 00115 */ 00116 virtual int width(); 00117 00118 /** Get the height of the screen in pixel 00119 * 00120 * @returns height of screen in pixel 00121 * 00122 */ 00123 virtual int height(); 00124 00125 00126 /** 00127 * Clear the display 00128 */ 00129 void clear(); 00130 00131 /** 00132 * Write image buffer to display 00133 */ 00134 void write_disp(void); 00135 00136 /** 00137 * set or reset a single pixel 00138 * 00139 * @param x horizontal position 00140 * @param y vertical position 00141 * @param color : 0 white, 1 black 00142 */ 00143 virtual void pixel(int x, int y, int color); 00144 00145 /** Fill the screen with white 00146 * 00147 */ 00148 virtual void cls(void); 00149 00150 /** draw a 1 pixel line 00151 * 00152 * @param x0,y0 start point 00153 * @param x1,y1 stop point 00154 * @param color : 0 white, 1 black 00155 */ 00156 void line(int x0, int y0, int x1, int y1, int color); 00157 00158 /** draw a rect 00159 * 00160 * @param x0,y0 top left corner 00161 * @param x1,y1 down right corner 00162 * @param color : 0 white, 1 black 00163 */ 00164 void rect(int x0, int y0, int x1, int y1, int color); 00165 00166 /** draw a filled rect 00167 * 00168 * @param x0,y0 top left corner 00169 * @param x1,y1 down right corner 00170 * @param color : 0 white, 1 black 00171 */ 00172 void fillrect(int x0, int y0, int x1, int y1, int color); 00173 00174 /** draw a circle 00175 * 00176 * @param x0,y0 center 00177 * @param r radius 00178 * @param color : 0 white, 1 black * 00179 */ 00180 void circle(int x0, int y0, int r, int color); 00181 00182 /** draw a filled circle 00183 * 00184 * @param x0,y0 center 00185 * @param r radius 00186 * @param color : 0 white, 1 black * 00187 */ 00188 void fillcircle(int x0, int y0, int r, int color); 00189 00190 /** set drawing mode 00191 * @param NORMAL : paint normal color, XOR : paint XOR of current pixels 00192 */ 00193 void setmode(int mode); 00194 00195 /** setup cursor position 00196 * 00197 * @param x x-position (top left) 00198 * @param y y-position 00199 */ 00200 virtual void locate(int x, int y); 00201 00202 /** calculate the max number of char in a line 00203 * 00204 * @returns max columns 00205 * depends on actual font size 00206 */ 00207 virtual int columns(); 00208 00209 /** calculate the max number of columns 00210 * 00211 * @returns max column 00212 * depends on actual font size 00213 */ 00214 virtual int rows(); 00215 00216 /** put a char on the screen 00217 * 00218 * @param value char to print 00219 * @returns printed char 00220 */ 00221 virtual int _putc(int value); 00222 00223 /** paint a character on given position out of the active font to the screen buffer 00224 * 00225 * @param x x-position (top left) 00226 * @param y y-position 00227 * @param c char code 00228 */ 00229 virtual void character(int x, int y, int c); 00230 00231 /** select the font to use 00232 * 00233 * @param f pointer to font array 00234 * 00235 * font array can created with GLCD Font Creator from http://www.mikroe.com 00236 * you have to add 4 parameter at the beginning of the font array to use: 00237 * - the number of byte / char 00238 * - the vertial size in pixel 00239 * - the horizontal size in pixel 00240 * - the number of byte per vertical line 00241 * you also have to change the array to char[] 00242 */ 00243 void set_font(unsigned char* f); 00244 00245 /** print bitmap to buffer 00246 * 00247 * @param bm struct Bitmap in flash 00248 * @param x x start 00249 * @param y y start 00250 * 00251 */ 00252 void print_bm(Bitmap bm, int x, int y); 00253 00254 00255 unsigned char* font; 00256 unsigned int draw_mode; 00257 00258 private: 00259 00260 EM027BS013 em_; 00261 unsigned int char_x; 00262 unsigned int char_y; 00263 00264 }; 00265 00266 #endif /* EAEPAPER_H */
Generated on Thu Jul 14 2022 12:47:28 by
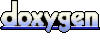