EaPaper Library for EM027BS013
Dependencies: EM027BS013 TFT_fonts
Fork of EaEpaper by
EaEpaper.cpp
00001 00002 #include "EaEpaper.h" 00003 00004 #define EA_IMG_BUF_SZ (5808) // 264 x 176 / 8 = 5808 00005 00006 #define LM75A_ADDRESS (0x92) // 0x49 << 1 00007 #define LM75A_CMD_TEMP 0x00 00008 00009 static uint8_t _newImage[EA_IMG_BUF_SZ]; 00010 00011 EaEpaper::EaEpaper(PinName sec03_SpiSCK, 00012 PinName sec04_SpiMOSI, 00013 PinName sec05_SpiMISO, 00014 PinName sec06_EpdCS, 00015 PinName sec07_EpdBusy, 00016 PinName sec08_EpdBorder, 00017 PinName sec09_I2cSCL, 00018 PinName sec10_I2cSDA, 00019 PinName sec11_FlashCS, 00020 PinName sec12_EpdReset, 00021 PinName sec13_EpdPanelOn, 00022 PinName sec14_EpdDischarge, 00023 const char* name): 00024 em_(sec03_SpiSCK, 00025 sec04_SpiMOSI, 00026 sec05_SpiMISO, 00027 sec06_EpdCS, 00028 sec07_EpdBusy, 00029 sec08_EpdBorder, 00030 sec09_I2cSCL, 00031 sec10_I2cSDA, 00032 sec11_FlashCS, 00033 sec12_EpdReset, 00034 sec13_EpdPanelOn, 00035 sec14_EpdDischarge), // sck 00036 GraphicsDisplay(name) 00037 { 00038 draw_mode = NORMAL; 00039 memset(_newImage, 0, EA_IMG_BUF_SZ); 00040 } 00041 00042 00043 int EaEpaper::width() 00044 { 00045 return 264; 00046 } 00047 00048 int EaEpaper::height() 00049 { 00050 return 176; 00051 } 00052 00053 00054 // erase pixel after power up 00055 void EaEpaper::clear() 00056 { 00057 memset(_newImage, 0xFF, EA_IMG_BUF_SZ); 00058 } 00059 00060 // update screen _newImage -> _oldImage 00061 void EaEpaper::write_disp(void) 00062 { 00063 em_.drawImage(_newImage); 00064 } 00065 00066 // read LM75A sensor on board to calculate display speed 00067 00068 // set one pixel in buffer _newImage 00069 00070 void EaEpaper::pixel(int x, int y, int color) 00071 { 00072 // first check parameter 00073 if(x > 263 || y > 175 || x < 0 || y < 0) return; 00074 00075 if(draw_mode == NORMAL) { 00076 if(color == 0) 00077 _newImage[(x / 8) + ((y-1) * 33)] &= ~(1 << (7-(x%8))); // erase pixel 00078 else 00079 _newImage[(x / 8) + ((y-1) * 33)] |= (1 << (7-(x%8))); // set pixel 00080 } else { // XOR mode 00081 if(color == 1) 00082 _newImage[(x / 8) + ((y-1) * 33)] ^= (1 << (7-(x%8))); // xor pixel 00083 } 00084 } 00085 00086 // clear screen 00087 void EaEpaper::cls(void) 00088 { 00089 memset(_newImage, 0xFF, EA_IMG_BUF_SZ); // clear display buffer 00090 } 00091 00092 // print line 00093 void EaEpaper::line(int x0, int y0, int x1, int y1, int color) 00094 { 00095 int dx = 0, dy = 0; 00096 int dx_sym = 0, dy_sym = 0; 00097 int dx_x2 = 0, dy_x2 = 0; 00098 int di = 0; 00099 00100 dx = x1-x0; 00101 dy = y1-y0; 00102 00103 if (dx > 0) { 00104 dx_sym = 1; 00105 } else { 00106 dx_sym = -1; 00107 } 00108 00109 if (dy > 0) { 00110 dy_sym = 1; 00111 } else { 00112 dy_sym = -1; 00113 } 00114 00115 dx = dx_sym*dx; 00116 dy = dy_sym*dy; 00117 00118 dx_x2 = dx*2; 00119 dy_x2 = dy*2; 00120 00121 if (dx >= dy) { 00122 di = dy_x2 - dx; 00123 while (x0 != x1) { 00124 00125 pixel(x0, y0, color); 00126 x0 += dx_sym; 00127 if (di<0) { 00128 di += dy_x2; 00129 } else { 00130 di += dy_x2 - dx_x2; 00131 y0 += dy_sym; 00132 } 00133 } 00134 pixel(x0, y0, color); 00135 } else { 00136 di = dx_x2 - dy; 00137 while (y0 != y1) { 00138 pixel(x0, y0, color); 00139 y0 += dy_sym; 00140 if (di < 0) { 00141 di += dx_x2; 00142 } else { 00143 di += dx_x2 - dy_x2; 00144 x0 += dx_sym; 00145 } 00146 } 00147 pixel(x0, y0, color); 00148 } 00149 } 00150 00151 // print rect 00152 void EaEpaper::rect(int x0, int y0, int x1, int y1, int color) 00153 { 00154 00155 if (x1 > x0) line(x0,y0,x1,y0,color); 00156 else line(x1,y0,x0,y0,color); 00157 00158 if (y1 > y0) line(x0,y0,x0,y1,color); 00159 else line(x0,y1,x0,y0,color); 00160 00161 if (x1 > x0) line(x0,y1,x1,y1,color); 00162 else line(x1,y1,x0,y1,color); 00163 00164 if (y1 > y0) line(x1,y0,x1,y1,color); 00165 else line(x1,y1,x1,y0,color); 00166 } 00167 00168 // print filled rect 00169 void EaEpaper::fillrect(int x0, int y0, int x1, int y1, int color) 00170 { 00171 int l,c,i; 00172 if(x0 > x1) { 00173 i = x0; 00174 x0 = x1; 00175 x1 = i; 00176 } 00177 00178 if(y0 > y1) { 00179 i = y0; 00180 y0 = y1; 00181 y1 = i; 00182 } 00183 00184 for(l = x0; l<= x1; l ++) { 00185 for(c = y0; c<= y1; c++) { 00186 pixel(l,c,color); 00187 } 00188 } 00189 } 00190 00191 // print circle 00192 void EaEpaper::circle(int x0, int y0, int r, int color) 00193 { 00194 int x = -r, y = 0, err = 2-2*r, e2; 00195 do { 00196 pixel(x0-x, y0+y,color); 00197 pixel(x0+x, y0+y,color); 00198 pixel(x0+x, y0-y,color); 00199 pixel(x0-x, y0-y,color); 00200 e2 = err; 00201 if (e2 <= y) { 00202 err += ++y*2+1; 00203 if (-x == y && e2 <= x) e2 = 0; 00204 } 00205 if (e2 > x) err += ++x*2+1; 00206 } while (x <= 0); 00207 00208 } 00209 00210 // print filled circle 00211 void EaEpaper::fillcircle(int x0, int y0, int r, int color) 00212 { 00213 int x = -r, y = 0, err = 2-2*r, e2; 00214 do { 00215 line(x0-x, y0-y, x0-x, y0+y, color); 00216 line(x0+x, y0-y, x0+x, y0+y, color); 00217 e2 = err; 00218 if (e2 <= y) { 00219 err += ++y*2+1; 00220 if (-x == y && e2 <= x) e2 = 0; 00221 } 00222 if (e2 > x) err += ++x*2+1; 00223 } while (x <= 0); 00224 } 00225 00226 // set drawing mode 00227 void EaEpaper::setmode(int mode) 00228 { 00229 draw_mode = mode; 00230 } 00231 00232 // set cursor position 00233 void EaEpaper::locate(int x, int y) 00234 { 00235 char_x = x; 00236 char_y = y; 00237 } 00238 00239 // calc char columns 00240 int EaEpaper::columns() 00241 { 00242 return width() / font[1]; 00243 } 00244 00245 // calc char rows 00246 int EaEpaper::rows() 00247 { 00248 return height() / font[2]; 00249 } 00250 00251 // print char 00252 int EaEpaper::_putc(int value) 00253 { 00254 if (value == '\n') { // new line 00255 char_x = 0; 00256 char_y = char_y + font[2]; 00257 if (char_y >= height() - font[2]) { 00258 char_y = 0; 00259 } 00260 } else { 00261 character(char_x, char_y, value); 00262 } 00263 return value; 00264 } 00265 00266 // paint char out of font 00267 void EaEpaper::character(int x, int y, int c) 00268 { 00269 unsigned int hor,vert,offset,bpl,j,i,b; 00270 unsigned char* zeichen; 00271 unsigned char z,w; 00272 00273 if ((c < 31) || (c > 127)) return; // test char range 00274 00275 // read font parameter from start of array 00276 offset = font[0]; // bytes / char 00277 hor = font[1]; // get hor size of font 00278 vert = font[2]; // get vert size of font 00279 bpl = font[3]; // bytes per line 00280 00281 if (char_x + hor > width()) { 00282 char_x = 0; 00283 char_y = char_y + vert; 00284 if (char_y >= height() - font[2]) { 00285 char_y = 0; 00286 } 00287 } 00288 00289 zeichen = &font[((c -32) * offset) + 4]; // start of char bitmap 00290 w = zeichen[0]; // width of actual char 00291 // construct the char into the buffer 00292 for (j=0; j<vert; j++) { // vert line 00293 for (i=0; i<hor; i++) { // horz line 00294 z = zeichen[bpl * i + ((j & 0xF8) >> 3)+1]; 00295 b = 1 << (j & 0x07); 00296 if (( z & b ) == 0x00) { 00297 pixel(x+i,y+j,0); 00298 } else { 00299 pixel(x+i,y+j,1); 00300 } 00301 00302 } 00303 } 00304 00305 char_x += w; 00306 } 00307 00308 // set actual font 00309 void EaEpaper::set_font(unsigned char* f) 00310 { 00311 font = f; 00312 } 00313 00314 void EaEpaper::print_bm(Bitmap bm, int x, int y) 00315 { 00316 int h,v,b; 00317 char d; 00318 00319 for(v=0; v < bm.ySize; v++) { // lines 00320 for(h=0; h < bm.xSize; h++) { // pixel 00321 if(h + x > width()) break; 00322 if(v + y > height()) break; 00323 d = bm.data[bm.Byte_in_Line * v + ((h & 0xF8) >> 3)]; 00324 b = 0x80 >> (h & 0x07); 00325 if((d & b) == 0) { 00326 pixel(x+h,y+v,0); 00327 } else { 00328 pixel(x+h,y+v,1); 00329 } 00330 } 00331 } 00332 00333 } 00334 00335
Generated on Thu Jul 14 2022 12:47:28 by
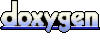