Opencv 3.1 project on GR-PEACH board
Fork of gr-peach-opencv-project by
Basic structures
[Core functionality]
Data Structures | |
struct | Ptr< T > |
Template class for smart pointers with shared ownership. More... | |
class | _InputArray |
This is the proxy class for passing read-only input arrays into OpenCV functions. More... | |
class | _OutputArray |
This type is very similar to InputArray except that it is used for input/output and output function parameters. More... | |
class | MatAllocator |
Custom array allocator. More... | |
class | MatCommaInitializer_< _Tp > |
Comma-separated Matrix Initializer. More... | |
class | Mat |
n-dimensional dense array class More... | |
class | Mat_< _Tp > |
Template matrix class derived from Mat. More... | |
class | UMat |
class | SparseMat |
The class SparseMat represents multi-dimensional sparse numerical arrays. More... | |
class | SparseMat_< _Tp > |
Template sparse n-dimensional array class derived from SparseMat. More... | |
class | MatConstIterator_< _Tp > |
Matrix read-only iterator. More... | |
class | MatIterator_< _Tp > |
Matrix read-write iterator. More... | |
class | SparseMatConstIterator |
Read-Only Sparse Matrix Iterator. More... | |
class | SparseMatIterator |
Read-write Sparse Matrix Iterator. More... | |
class | SparseMatConstIterator_< _Tp > |
Template Read-Only Sparse Matrix Iterator Class. More... | |
class | SparseMatIterator_< _Tp > |
Template Read-Write Sparse Matrix Iterator Class. More... | |
class | NAryMatIterator |
n-ary multi-dimensional array iterator. More... | |
class | MatExpr |
Matrix expression representation. More... | |
class | Matx< _Tp, m, n > |
Template class for small matrices whose type and size are known at compilation time. More... | |
class | DataType< Matx< _Tp, m, n > > |
class | MatxCommaInitializer< _Tp, m, n > |
Comma-separated Matrix Initializer. More... | |
class | Vec< _Tp, cn > |
Template class for short numerical vectors, a partial case of Matx. More... | |
class | DataType< Vec< _Tp, cn > > |
class | VecCommaInitializer< _Tp, m > |
Comma-separated Vec Initializer. More... | |
class | DataType< _Tp > |
Template "trait" class for OpenCV primitive data types. More... | |
class | DataDepth< _Tp > |
A helper class for cv::DataType. More... | |
class | Complex< _Tp > |
A complex number class. More... | |
class | Point_< _Tp > |
Template class for 2D points specified by its coordinates `x` and `y`. More... | |
class | Point3_< _Tp > |
Template class for 3D points specified by its coordinates `x`, `y` and `z`. More... | |
class | Size_< _Tp > |
Template class for specifying the size of an image or rectangle. More... | |
class | Rect_< _Tp > |
Template class for 2D rectangles. More... | |
class | RotatedRect |
The class represents rotated (i.e. More... | |
class | Range |
Template class specifying a continuous subsequence (slice) of a sequence. More... | |
class | Scalar_< _Tp > |
Template class for a 4-element vector derived from Vec. More... | |
class | KeyPoint |
Data structure for salient point detectors. More... | |
class | DMatch |
Class for matching keypoint descriptors. More... | |
class | TermCriteria |
The class defining termination criteria for iterative algorithms. More... | |
class | Formatted |
class | Formatter |
class | Algorithm |
This is a base class for all more or less complex algorithms in OpenCV. More... | |
Enumerations | |
enum | UMatUsageFlags |
Usage flags for allocator. More... | |
Functions | |
template<typename T > | |
void | swap (Ptr< T > &ptr1, Ptr< T > &ptr2) |
Equivalent to ptr1.swap(ptr2). | |
template<typename T > | |
bool | operator== (const Ptr< T > &ptr1, const Ptr< T > &ptr2) |
Return whether ptr1.get() and ptr2.get() are equal and not equal, respectively. | |
template<typename T > | |
Ptr< T > | makePtr () |
`makePtr<T>(...)` is equivalent to `Ptr<T>(new T(...))`. | |
template<typename T , typename A1 > | |
Ptr< T > | makePtr (const A1 &a1) |
template<typename T , typename A1 , typename A2 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2) |
template<typename T , typename A1 , typename A2 , typename A3 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9) |
template<typename T , typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 , typename A10 > | |
Ptr< T > | makePtr (const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9, const A10 &a10) |
Shorter aliases for the most popular specializations of Vec<T,n> | |
typedef Vec< uchar, 2 > | Vec2b |
typedef Vec< uchar, 3 > | Vec3b |
typedef Vec< uchar, 4 > | Vec4b |
typedef Vec< short, 2 > | Vec2s |
typedef Vec< short, 3 > | Vec3s |
typedef Vec< short, 4 > | Vec4s |
typedef Vec< ushort, 2 > | Vec2w |
typedef Vec< ushort, 3 > | Vec3w |
typedef Vec< ushort, 4 > | Vec4w |
typedef Vec< int, 2 > | Vec2i |
typedef Vec< int, 3 > | Vec3i |
typedef Vec< int, 4 > | Vec4i |
typedef Vec< int, 6 > | Vec6i |
typedef Vec< int, 8 > | Vec8i |
typedef Vec< float, 2 > | Vec2f |
typedef Vec< float, 3 > | Vec3f |
typedef Vec< float, 4 > | Vec4f |
typedef Vec< float, 6 > | Vec6f |
typedef Vec< double, 2 > | Vec2d |
typedef Vec< double, 3 > | Vec3d |
typedef Vec< double, 4 > | Vec4d |
typedef Vec< double, 6 > | Vec6d |
Enumeration Type Documentation
Function Documentation
Ptr<T> cv::makePtr | ( | ) |
`makePtr<T>(...)` is equivalent to `Ptr<T>(new T(...))`.
It is shorter than the latter, and it's marginally safer than using a constructor or Ptr::reset, since it ensures that the owned pointer is new and thus not owned by any other Ptr instance. Unfortunately, perfect forwarding is impossible to implement in C++03, and so makePtr is limited to constructors of T that have up to 10 arguments, none of which are non-const references.
Ptr<T> cv::makePtr | ( | const A1 & | a1 ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5, | ||
const A6 & | a6 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5, | ||
const A6 & | a6, | ||
const A7 & | a7 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5, | ||
const A6 & | a6, | ||
const A7 & | a7, | ||
const A8 & | a8 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5, | ||
const A6 & | a6, | ||
const A7 & | a7, | ||
const A8 & | a8, | ||
const A9 & | a9, | ||
const A10 & | a10 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3, | ||
const A4 & | a4, | ||
const A5 & | a5, | ||
const A6 & | a6, | ||
const A7 & | a7, | ||
const A8 & | a8, | ||
const A9 & | a9 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Ptr<T> cv::makePtr | ( | const A1 & | a1, |
const A2 & | a2, | ||
const A3 & | a3 | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
bool cv::operator== | ( | const Ptr< T > & | ptr1, |
const Ptr< T > & | ptr2 | ||
) |
Return whether ptr1.get() and ptr2.get() are equal and not equal, respectively.
void cv::swap | ( | Ptr< T > & | ptr1, |
Ptr< T > & | ptr2 | ||
) |
Equivalent to ptr1.swap(ptr2).
Provided to help write generic algorithms.
Generated on Tue Jul 12 2022 15:17:33 by
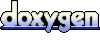