Library for big numbers from http://www.ttmath.org/
Dependents: PIDHeater82 Conceptcontroller_v_1_0 AlarmClockApp COG4050_adxl355_tilt ... more
Big< exp, man > Class Template Reference
Big implements the floating point numbers. More...
#include <ttmathbig.h>
Public Member Functions | |
uint | CheckCarry (uint c) |
uint | Standardizing () |
void | ClearInfoBit (unsigned char bit) |
void | SetInfoBit (unsigned char bit) |
bool | IsInfoBit (unsigned char bit) const |
void | SetZero () |
void | SetOne () |
void | Set05 () |
void | SetNan () |
void | SetZeroNan () |
void | Swap (Big< exp, man > &ss2) |
void | SetPi () |
void | Set05Pi () |
void | Set2Pi () |
void | SetE () |
void | SetLn2 () |
void | SetLn10 () |
void | SetMax () |
void | SetMin () |
bool | IsZero () const |
bool | IsSign () const |
bool | IsNan () const |
void | Abs () |
void | Sgn () |
void | SetSign () |
void | ChangeSign () |
uint | Add (Big< exp, man > ss2, bool round=true, bool adding=true) |
uint | Sub (const Big< exp, man > &ss2, bool round=true) |
uint | BitAnd (Big< exp, man > ss2) |
uint | BitOr (Big< exp, man > ss2) |
uint | BitXor (Big< exp, man > ss2) |
uint | MulUInt (uint ss2) |
uint | MulInt (sint ss2) |
uint | Mul (const Big< exp, man > &ss2, bool round=true) |
uint | Div (const Big< exp, man > &ss2, bool round=true) |
uint | Mod (const Big< exp, man > &ss2) |
uint | Mod2 () const |
template<uint pow_size> | |
uint | Pow (UInt< pow_size > pow) |
template<uint pow_size> | |
uint | Pow (Int< pow_size > pow) |
uint | PowUInt (Big< exp, man > pow) |
uint | PowInt (const Big< exp, man > &pow) |
uint | PowFrac (const Big< exp, man > &pow) |
uint | Pow (const Big< exp, man > &pow) |
uint | Sqrt () |
void | ExpSurrounding0 (const Big< exp, man > &x, uint *steps=0) |
uint | Exp (const Big< exp, man > &x) |
void | LnSurrounding1 (const Big< exp, man > &x, uint *steps=0) |
uint | Ln (const Big< exp, man > &x) |
uint | Log (const Big< exp, man > &x, const Big< exp, man > &base) |
template<uint another_exp, uint another_man> | |
uint | FromBig (const Big< another_exp, another_man > &another) |
uint | ToUInt () const |
uint | ToUInt (uint &result) const |
sint | ToInt () const |
uint | ToInt (uint &result) const |
uint | ToInt (sint &result) const |
template<uint int_size> | |
uint | ToUInt (UInt< int_size > &result) const |
template<uint int_size> | |
uint | ToInt (UInt< int_size > &result) const |
template<uint int_size> | |
uint | ToInt (Int< int_size > &result) const |
uint | FromUInt (uint value) |
uint | FromInt (uint value) |
uint | FromInt (sint value) |
uint | FromDouble (double value) |
uint | FromFloat (float value) |
double | ToDouble () const |
float | ToFloat () const |
uint | ToFloat (float &result) const |
uint | ToDouble (double &result) const |
Big< exp, man > & | operator= (sint value) |
Big< exp, man > & | operator= (uint value) |
Big< exp, man > & | operator= (float value) |
Big< exp, man > & | operator= (double value) |
Big (sint value) | |
Big (uint value) | |
Big (double value) | |
Big (float value) | |
uint | ToUInt (ulint &result) const |
uint | ToInt (ulint &result) const |
uint | ToInt (slint &result) const |
uint | FromUInt (ulint value) |
uint | FromInt (ulint value) |
uint | FromInt (slint value) |
Big (ulint value) | |
Big< exp, man > & | operator= (ulint value) |
Big (slint value) | |
Big< exp, man > & | operator= (slint value) |
uint | ToUInt (unsigned int &result) const |
uint | ToInt (unsigned int &result) const |
uint | ToInt (signed int &result) const |
Big< exp, man > & | operator= (unsigned int value) |
Big (unsigned int value) | |
Big< exp, man > & | operator= (signed int value) |
Big (signed int value) | |
template<uint int_size> | |
uint | FromUInt (UInt< int_size > value) |
template<uint int_size> | |
uint | FromInt (const UInt< int_size > &value) |
template<uint int_size> | |
uint | FromInt (Int< int_size > value) |
template<uint int_size> | |
Big< exp, man > & | operator= (const Int< int_size > &value) |
template<uint int_size> | |
Big (const Int< int_size > &value) | |
template<uint int_size> | |
Big< exp, man > & | operator= (const UInt< int_size > &value) |
template<uint int_size> | |
Big (const UInt< int_size > &value) | |
template<uint another_exp, uint another_man> | |
Big< exp, man > & | operator= (const Big< another_exp, another_man > &value) |
template<uint another_exp, uint another_man> | |
Big (const Big< another_exp, another_man > &value) | |
Big () | |
~Big () | |
Big< exp, man > & | operator= (const Big< exp, man > &value) |
Big (const Big< exp, man > &value) | |
uint | ToString (std::string &result, uint base=10, bool scient=false, sint scient_from=15, sint round=-1, bool trim_zeroes=true, char comma= '.') const |
uint | ToString (std::string &result, const Conv &conv) const |
std::string | ToString (const Conv &conv) const |
std::string | ToString (uint base=10) const |
uint | ToString (std::wstring &result, uint base=10, bool scient=false, sint scient_from=15, sint round=-1, bool trim_zeroes=true, wchar_t comma= '.') const |
uint | ToString (std::wstring &result, const Conv &conv) const |
std::wstring | ToWString (const Conv &conv) const |
std::wstring | ToWString (uint base=10) const |
uint | FromString (const char *source, uint base=10, const char **after_source=0, bool *value_read=0) |
uint | FromString (const char *source, const Conv &conv, const char **after_source=0, bool *value_read=0) |
uint | FromString (const std::string &string, uint base=10, const char **after_source=0, bool *value_read=0) |
uint | FromString (const std::string &string, const Conv &conv, const char **after_source=0, bool *value_read=0) |
uint | FromString (const wchar_t *source, uint base=10, const wchar_t **after_source=0, bool *value_read=0) |
uint | FromString (const wchar_t *source, const Conv &conv, const wchar_t **after_source=0, bool *value_read=0) |
uint | FromString (const std::wstring &string, uint base=10, const wchar_t **after_source=0, bool *value_read=0) |
uint | FromString (const std::wstring &string, const Conv &conv, const wchar_t **after_source=0, bool *value_read=0) |
Big (const char *string) | |
Big (const std::string &string) | |
Big< exp, man > & | operator= (const char *string) |
Big< exp, man > & | operator= (const std::string &string) |
Big (const wchar_t *string) | |
Big (const std::wstring &string) | |
Big< exp, man > & | operator= (const wchar_t *string) |
Big< exp, man > & | operator= (const std::wstring &string) |
bool | SmallerWithoutSignThan (const Big< exp, man > &ss2) const |
bool | GreaterWithoutSignThan (const Big< exp, man > &ss2) const |
bool | EqualWithoutSign (const Big< exp, man > &ss2) const |
Big< exp, man > | operator- () const |
Big< exp, man > & | operator++ () |
Big< exp, man > | operator++ (int) |
Big< exp, man > | operator& (const Big< exp, man > &p2) const |
void | SkipFraction () |
void | RemainFraction () |
bool | IsInteger () const |
uint | Round () |
Static Public Member Functions | |
static const char * | LibTypeStr () |
static LibTypeCode | LibType () |
Friends | |
class | Big< exp-1, man > |
std::ostream & | operator<< (std::ostream &s, const Big< exp, man > &l) |
std::wostream & | operator<< (std::wostream &s, const Big< exp, man > &l) |
std::istream & | operator>> (std::istream &s, Big< exp, man > &l) |
std::wistream & | operator>> (std::wistream &s, Big< exp, man > &l) |
Detailed Description
template<uint exp, uint man>
class ttmath::Big< exp, man >
Big implements the floating point numbers.
Definition at line 63 of file ttmathbig.h.
Constructor & Destructor Documentation
Big | ( | sint | value ) |
a constructor for converting 'sint' to this class
Definition at line 3122 of file ttmathbig.h.
a constructor for converting 'uint' to this class
Definition at line 3130 of file ttmathbig.h.
Big | ( | double | value ) |
a constructor for converting 'double' to this class
Definition at line 3139 of file ttmathbig.h.
Big | ( | float | value ) |
a constructor for converting 'float' to this class
Definition at line 3148 of file ttmathbig.h.
a constructor for converting 'ulint' (64bit unsigned integer) to this class
Definition at line 3291 of file ttmathbig.h.
Big | ( | slint | value ) |
a constructor for converting 'slint' (64bit signed integer) to this class
Definition at line 3311 of file ttmathbig.h.
Big | ( | unsigned int | value ) |
a constructor for converting 32 bit unsigned int to this class this constructor is created only on a 64bit platform***
Definition at line 3429 of file ttmathbig.h.
Big | ( | signed int | value ) |
a constructor for converting 32 bit signed int to this class this constructor is created only on a 64bit platform***
Definition at line 3451 of file ttmathbig.h.
a constructor for converting from 'Int<int_size>' to this class
Definition at line 3557 of file ttmathbig.h.
a constructor for converting from 'UInt<int_size>' to this class
Definition at line 3579 of file ttmathbig.h.
a constructor for converting from 'Big<another_exp, another_man>' to this class
Definition at line 3601 of file ttmathbig.h.
Big | ( | ) |
a default constructor
by default we don't set any of the members to zero only NaN flag is set
if you want the mantissa and exponent to be set to zero define TTMATH_BIG_DEFAULT_CLEAR macro (useful for debug purposes)
Definition at line 3617 of file ttmathbig.h.
~Big | ( | ) |
a destructor
Definition at line 3636 of file ttmathbig.h.
a constructor for copying from another object of this class
Definition at line 3658 of file ttmathbig.h.
Big | ( | const char * | string ) |
a constructor for converting a string into this class
Definition at line 5276 of file ttmathbig.h.
Big | ( | const std::string & | string ) |
a constructor for converting a string into this class
Definition at line 5285 of file ttmathbig.h.
Big | ( | const wchar_t * | string ) |
a constructor for converting a string into this class
Definition at line 5319 of file ttmathbig.h.
Big | ( | const std::wstring & | string ) |
a constructor for converting a string into this class
Definition at line 5328 of file ttmathbig.h.
Member Function Documentation
void Abs | ( | ) |
this method clears the sign (there'll be an absolute value)
e.g. -1 -> 1 2 -> 2
Definition at line 676 of file ttmathbig.h.
Addition this = this + ss2
it returns carry if the sum is too big
Definition at line 922 of file ttmathbig.h.
bitwise AND
this and ss2 must be >= 0 return values: 0 - ok 1 - carry 2 - this or ss2 was negative
Definition at line 985 of file ttmathbig.h.
bitwise OR
this and ss2 must be >= 0 return values: 0 - ok 1 - carry 2 - this or ss2 was negative
Definition at line 1043 of file ttmathbig.h.
bitwise XOR
this and ss2 must be >= 0 return values: 0 - ok 1 - carry 2 - this or ss2 was negative
Definition at line 1098 of file ttmathbig.h.
void ChangeSign | ( | ) |
this method changes the sign when there is a value of zero then the sign is not changed
e.g. -1 -> 1 2 -> -2
Definition at line 732 of file ttmathbig.h.
this method sets NaN if there was a carry (and returns 1 in such a case)
c can be 0, 1 or other value different from zero
Definition at line 121 of file ttmathbig.h.
void ClearInfoBit | ( | unsigned char | bit ) |
this method clears a specific bit in the 'info' variable
bit is one of: TTMATH_BIG_SIGN, TTMATH_BIG_NAN etc.
Definition at line 224 of file ttmathbig.h.
division this = this / ss2
return value: 0 - ok 1 - carry (in a division carry can be as well) 2 - improper argument (ss2 is zero)
Definition at line 1441 of file ttmathbig.h.
bool EqualWithoutSign | ( | const Big< exp, man > & | ss2 ) | const |
this method performs the formula 'abs(this) == abs(ss2)' and returns the result
(in other words it treats 'this' and 'ss2' as values without a sign) we don't check the NaN flag
Definition at line 5438 of file ttmathbig.h.
Exponent this = exp(x) = e^x
we're using the fact that our value is stored in form of: x = mantissa * 2^exponent then e^x = e^(mantissa* 2^exponent) or e^x = (e^mantissa)^(2^exponent)
'Exp' returns a carry if we can't count the result ('x' is too big)
Definition at line 1934 of file ttmathbig.h.
Exponent this = exp(x) = e^x where x is in (-1,1)
we're using the formula exp(x) = 1 + (x)/(1!) + (x^2)/(2!) + (x^3)/(3!) + ...
Definition at line 1858 of file ttmathbig.h.
converting methods
converting from another type of a Big object
Definition at line 2204 of file ttmathbig.h.
uint FromDouble | ( | double | value ) |
this method converts from standard double into this class
standard double means IEEE-754 floating point value with 64 bits it is as follows (from http://www.psc.edu/general/software/packages/ieee/ieee.html):
The IEEE double precision floating point standard representation requires a 64 bit word, which may be represented as numbered from 0 to 63, left to right. The first bit is the sign bit, S, the next eleven bits are the exponent bits, 'E', and the final 52 bits are the fraction 'F':
S EEEEEEEEEEE FFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFF 0 1 11 12 63
The value V represented by the word may be determined as follows:
If E=2047 and F is nonzero, then V=NaN ("Not a number") If E=2047 and F is zero and S is 1, then V=-Infinity If E=2047 and F is zero and S is 0, then V=Infinity If 0<E<2047 then V=(-1)**S * 2 ** (E-1023) * (1.F) where "1.F" is intended to represent the binary number created by prefixing F with an implicit leading 1 and a binary point. If E=0 and F is nonzero, then V=(-1)**S * 2 ** (-1022) * (0.F) These are "unnormalized" values. If E=0 and F is zero and S is 1, then V=-0 If E=0 and F is zero and S is 0, then V=0
Definition at line 2587 of file ttmathbig.h.
uint FromFloat | ( | float | value ) |
this method converts from float to this class
Definition at line 2797 of file ttmathbig.h.
a method for converting 'ulint' (64bit unsigned integer) to this class
Definition at line 3260 of file ttmathbig.h.
uint FromInt | ( | slint | value ) |
a method for converting 'slint' (64bit signed integer) to this class
Definition at line 3269 of file ttmathbig.h.
a method for converting from 'UInt<int_size>' to this class
Definition at line 3510 of file ttmathbig.h.
a method for converting from 'Int<int_size>' to this class
Definition at line 3520 of file ttmathbig.h.
a method for converting 'uint' to this class
Definition at line 2528 of file ttmathbig.h.
uint FromInt | ( | sint | value ) |
a method for converting 'sint' to this class
Definition at line 2537 of file ttmathbig.h.
uint FromString | ( | const char * | source, |
uint | base = 10 , |
||
const char ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
it returns 1 if the value is too big -- we cannot pass it into the range of our class Big<exp,man> (or if the base is incorrect)
that means only digits before the comma operator can make this value too big, all digits after the comma we can ignore
'source' - pointer to the string for parsing
if 'after_source' is set that when this method finishes it sets the pointer to the new first character after parsed value
'value_read' - if the pointer is provided that means the value_read will be true only when a value has been actually read, there can be situation where only such a string '-' or '+' will be parsed -- 'after_source' will be different from 'source' but no value has been read (there are no digits) on other words if 'value_read' is true -- there is at least one digit in the string
Definition at line 4907 of file ttmathbig.h.
uint FromString | ( | const char * | source, |
const Conv & | conv, | ||
const char ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4919 of file ttmathbig.h.
uint FromString | ( | const std::string & | string, |
uint | base = 10 , |
||
const char ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4928 of file ttmathbig.h.
uint FromString | ( | const std::string & | string, |
const Conv & | conv, | ||
const char ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4937 of file ttmathbig.h.
uint FromString | ( | const wchar_t * | source, |
uint | base = 10 , |
||
const wchar_t ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4948 of file ttmathbig.h.
uint FromString | ( | const wchar_t * | source, |
const Conv & | conv, | ||
const wchar_t ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4960 of file ttmathbig.h.
uint FromString | ( | const std::wstring & | string, |
uint | base = 10 , |
||
const wchar_t ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4969 of file ttmathbig.h.
uint FromString | ( | const std::wstring & | string, |
const Conv & | conv, | ||
const wchar_t ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
a method for converting a string into its value
Definition at line 4978 of file ttmathbig.h.
a method for converting 'ulint' (64bit unsigned integer) to this class
Definition at line 3199 of file ttmathbig.h.
a method for converting from 'UInt<int_size>' to this class
Definition at line 3497 of file ttmathbig.h.
a method for converting 'uint' to this class
Definition at line 2502 of file ttmathbig.h.
bool GreaterWithoutSignThan | ( | const Big< exp, man > & | ss2 ) | const |
this method performs the formula 'abs(this) > abs(ss2)' and returns the result
(in other words it treats 'this' and 'ss2' as values without a sign) we don't check the NaN flag
Definition at line 5406 of file ttmathbig.h.
bool IsInfoBit | ( | unsigned char | bit ) | const |
this method returns true if a specific bit in the 'info' variable is set
bit is one of: TTMATH_BIG_SIGN, TTMATH_BIG_NAN etc.
Definition at line 247 of file ttmathbig.h.
bool IsInteger | ( | ) | const |
this method returns true if the value is integer (there is no a fraction)
(we don't check nan)
Definition at line 5816 of file ttmathbig.h.
bool IsNan | ( | ) | const |
this method returns true when there is not a valid number
Definition at line 661 of file ttmathbig.h.
bool IsSign | ( | ) | const |
this method returns true when there's the sign set also we don't check the NaN flag
Definition at line 652 of file ttmathbig.h.
bool IsZero | ( | ) | const |
testing whether there is a value zero or not
Definition at line 642 of file ttmathbig.h.
static LibTypeCode LibType | ( | ) | [static] |
returning the currect type of the library
Definition at line 154 of file ttmathbig.h.
static const char* LibTypeStr | ( | ) | [static] |
returning the string represents the currect type of the library we have following types: asm_vc_32 - with asm code designed for Microsoft Visual C++ (32 bits) asm_gcc_32 - with asm code designed for GCC (32 bits) asm_vc_64 - with asm for VC (64 bit) asm_gcc_64 - with asm for GCC (64 bit) no_asm_32 - pure C++ version (32 bit) - without any asm code no_asm_64 - pure C++ version (64 bit) - without any asm code
Definition at line 145 of file ttmathbig.h.
Natural logarithm this = ln(x) (a logarithm with the base equal 'e')
we're using the fact that our value is stored in form of: x = mantissa * 2^exponent then ln(x) = ln (mantissa * 2^exponent) = ln (mantissa) + (exponent * ln (2))
the mantissa we'll show as a value from range <1,2) because the logarithm is decreasing too fast when 'x' is going to 0
return values: 0 - ok 1 - overflow (carry) 2 - incorrect argument (x<=0)
Definition at line 2109 of file ttmathbig.h.
Natural logarithm this = ln(x) where x in range <1,2)
we're using the formula: ln x = 2 * [ (x-1)/(x+1) + (1/3)((x-1)/(x+1))^3 + (1/5)((x-1)/(x+1))^5 + ... ]
Definition at line 2011 of file ttmathbig.h.
Logarithm from 'x' with a 'base'
we're using the formula: Log(x) with 'base' = ln(x) / ln(base)
return values: 0 - ok 1 - overflow 2 - incorrect argument (x<=0) 3 - incorrect base (a<=0 lub a=1)
Definition at line 2153 of file ttmathbig.h.
the remainder from a division
e.g. 12.6 mod 3 = 0.6 because 12.6 = 3*4 + 0.6 -12.6 mod 3 = -0.6 bacause -12.6 = 3*(-4) + (-0.6) 12.6 mod -3 = 0.6 -12.6 mod -3 = -0.6
it means: in other words: this(old) = ss2 * q + this(new)
return value: 0 - ok 1 - carry 2 - improper argument (ss2 is zero)
Definition at line 1511 of file ttmathbig.h.
uint Mod2 | ( | ) | const |
this method returns: 'this' mod 2 (either zero or one)
this method is much faster than using Mod( object_with_value_two )
Definition at line 1531 of file ttmathbig.h.
multiplication this = this * ss2 this method returns a carry
Definition at line 1344 of file ttmathbig.h.
uint MulInt | ( | sint | ss2 ) |
Multiplication this = this * ss2 (ss2 is sint)
ss2 with a sign
Definition at line 1204 of file ttmathbig.h.
Multiplication this = this * ss2 (ss2 is uint)
ss2 without a sign
Definition at line 1150 of file ttmathbig.h.
bitwise operators (we do not define bitwise not)
Definition at line 5672 of file ttmathbig.h.
Big<exp,man>& operator++ | ( | ) |
Prefix operator e.g ++variable
Definition at line 5625 of file ttmathbig.h.
Big<exp,man> operator++ | ( | int | ) |
Postfix operator e.g variable++
Definition at line 5636 of file ttmathbig.h.
Big<exp,man> operator- | ( | ) | const |
standard mathematical operators
an operator for changing the sign
this method is not changing 'this' but the changed value is returned
Definition at line 5541 of file ttmathbig.h.
Big<exp, man>& operator= | ( | sint | value ) |
an operator= for converting 'sint' to this class
Definition at line 3078 of file ttmathbig.h.
an operator= for converting 'uint' to this class
Definition at line 3089 of file ttmathbig.h.
Big<exp, man>& operator= | ( | float | value ) |
an operator= for converting 'float' to this class
Definition at line 3100 of file ttmathbig.h.
Big<exp, man>& operator= | ( | double | value ) |
an operator= for converting 'double' to this class
Definition at line 3111 of file ttmathbig.h.
an operator for converting 'ulint' (64bit unsigned integer) to this class
Definition at line 3300 of file ttmathbig.h.
Big<exp, man>& operator= | ( | slint | value ) |
an operator for converting 'slint' (64bit signed integer) to this class
Definition at line 3320 of file ttmathbig.h.
Big<exp, man>& operator= | ( | unsigned int | value ) |
an operator= for converting 32 bit unsigned int to this class this operator is created only on a 64bit platform***
Definition at line 3417 of file ttmathbig.h.
Big<exp, man>& operator= | ( | signed int | value ) |
an operator for converting 32 bit signed int to this class this operator is created only on a 64bit platform***
Definition at line 3439 of file ttmathbig.h.
an operator= for converting from 'Int<int_size>' to this class
Definition at line 3545 of file ttmathbig.h.
an operator= for converting from 'UInt<int_size>' to this class
Definition at line 3567 of file ttmathbig.h.
an operator= for converting from 'Big<another_exp, another_man>' to this class
Definition at line 3589 of file ttmathbig.h.
the default assignment operator
Definition at line 3644 of file ttmathbig.h.
Big<exp, man>& operator= | ( | const char * | string ) |
an operator= for converting a string into its value
Definition at line 5294 of file ttmathbig.h.
Big<exp, man>& operator= | ( | const std::string & | string ) |
an operator= for converting a string into its value
Definition at line 5305 of file ttmathbig.h.
Big<exp, man>& operator= | ( | const wchar_t * | string ) |
an operator= for converting a string into its value
Definition at line 5337 of file ttmathbig.h.
Big<exp, man>& operator= | ( | const std::wstring & | string ) |
an operator= for converting a string into its value
Definition at line 5348 of file ttmathbig.h.
power this = this ^ pow (pow without a sign)
binary algorithm (r-to-l)
return values: 0 - ok 1 - carry 2 - incorrect arguments (0^0)
Definition at line 1556 of file ttmathbig.h.
power this = this ^ pow p can be negative
return values: 0 - ok 1 - carry 2 - incorrect arguments 0^0 or 0^(-something)
Definition at line 1608 of file ttmathbig.h.
power this = this ^ pow pow can be negative and with fraction
return values: 0 - ok 1 - carry 2 - incorrect argument ('this' or 'pow')
Definition at line 1769 of file ttmathbig.h.
power this = this ^ pow this must be greater than zero (this > 0) pow can be negative and with fraction
return values: 0 - ok 1 - carry 2 - incorrect argument ('this' <= 0)
Definition at line 1739 of file ttmathbig.h.
power this = this ^ [pow] pow is treated as a value without a fraction pow can be negative
return values: 0 - ok 1 - carry 2 - incorrect arguments 0^0 or 0^(-something)
Definition at line 1703 of file ttmathbig.h.
power this = this ^ abs([pow]) pow is treated as a value without a sign and without a fraction if pow has a sign then the method pow.Abs() is used if pow has a fraction the fraction is skipped (not used in calculation)
return values: 0 - ok 1 - carry 2 - incorrect arguments (0^0)
Definition at line 1647 of file ttmathbig.h.
void RemainFraction | ( | ) |
this method remains only a fraction from the value
for example: 30.56 will be 0.56 -12.67 -- -0.67
Definition at line 5775 of file ttmathbig.h.
uint Round | ( | ) |
this method rounds to the nearest integer value (it returns a carry if it was)
for example: 2.3 = 2 2.8 = 3
-2.3 = -2 -2.8 = 3
Definition at line 5863 of file ttmathbig.h.
void Set05 | ( | ) |
this method sets value 0.5
Definition at line 285 of file ttmathbig.h.
void Set05Pi | ( | ) |
this method sets the value of 0.5 * pi
Definition at line 404 of file ttmathbig.h.
void Set2Pi | ( | ) |
this method sets the value of 2 * pi
Definition at line 415 of file ttmathbig.h.
void SetE | ( | ) |
this method sets the value of e (the base of the natural logarithm)
Definition at line 427 of file ttmathbig.h.
void SetInfoBit | ( | unsigned char | bit ) |
this method sets a specific bit in the 'info' variable
bit is one of: TTMATH_BIG_SIGN, TTMATH_BIG_NAN etc.
Definition at line 236 of file ttmathbig.h.
void SetLn10 | ( | ) |
this method sets the value of ln(10) the natural logarithm from 10
I introduced this constant especially to make the conversion ToString() being faster. In fact the method ToString() is keeping values of logarithms it has calculated but it must calculate the logarithm at least once. If a program, which uses this library, is running for a long time this would be ok, but for programs which are running shorter, for example for CGI applications which only once are printing values, this would be much inconvenience. Then if we're printing with base (radix) 10 and the mantissa of our value is smaller than or equal to TTMATH_BUILTIN_VARIABLES_SIZE we don't calculate the logarithm but take it from this constant.
Definition at line 552 of file ttmathbig.h.
void SetLn2 | ( | ) |
this method sets the value of ln(2) the natural logarithm from 2
Definition at line 484 of file ttmathbig.h.
void SetMax | ( | ) |
this method sets the maximum value which can be held in this type
Definition at line 612 of file ttmathbig.h.
void SetMin | ( | ) |
this method sets the minimum value which can be held in this type
Definition at line 626 of file ttmathbig.h.
void SetNan | ( | ) |
this method sets NaN flag (Not a Number) when this flag is set that means there is no a valid number
Definition at line 296 of file ttmathbig.h.
void SetOne | ( | ) |
this method sets one
Definition at line 271 of file ttmathbig.h.
void SetPi | ( | ) |
this method sets the value of pi
Definition at line 393 of file ttmathbig.h.
void SetSign | ( | ) |
this method sets the sign
e.g. -1 -> -1 2 -> -2
we do not check whether there is a zero or not, if you're using this method you must be sure that the value is (or will be afterwards) different from zero
Definition at line 718 of file ttmathbig.h.
void SetZero | ( | ) |
this method sets zero
Definition at line 256 of file ttmathbig.h.
void SetZeroNan | ( | ) |
this method sets NaN flag (Not a Number) also clears the mantissa and exponent (similarly as it would be a zero value)
Definition at line 306 of file ttmathbig.h.
void Sgn | ( | ) |
this method remains the 'sign' of the value e.g. -2 = -1 0 = 0 10 = 1
Definition at line 688 of file ttmathbig.h.
void SkipFraction | ( | ) |
this method makes an integer value by skipping any fractions
for example: 10.7 will be 10 12.1 -- 12 -20.2 -- 20 -20.9 -- 20 -0.7 -- 0 0.8 -- 0
Definition at line 5741 of file ttmathbig.h.
bool SmallerWithoutSignThan | ( | const Big< exp, man > & | ss2 ) | const |
methods for comparing
this method performs the formula 'abs(this) < abs(ss2)' and returns the result
(in other words it treats 'this' and 'ss2' as values without a sign) we don't check the NaN flag
Definition at line 5374 of file ttmathbig.h.
uint Sqrt | ( | ) |
this function calculates the square root e.g. let this=9 then this.Sqrt() gives 3
return: 0 - ok 1 - carry 2 - improper argument (this<0 or NaN)
Definition at line 1806 of file ttmathbig.h.
uint Standardizing | ( | ) |
this method moves all bits from mantissa into its left side (suitably changes the exponent) or if the mantissa is zero it sets the exponent to zero as well (and clears the sign bit and sets the zero bit)
it can return a carry the carry will be when we don't have enough space in the exponent
you don't have to use this method if you don't change the mantissa and exponent directly
Definition at line 173 of file ttmathbig.h.
Subtraction this = this - ss2
it returns carry if the result is too big
Definition at line 970 of file ttmathbig.h.
void Swap | ( | Big< exp, man > & | ss2 ) |
this method swappes this for an argument
Definition at line 316 of file ttmathbig.h.
double ToDouble | ( | ) | const |
this method converts from this class into the 'double'
if the value is too big: 'result' will be +/-infinity (depending on the sign) if the value is too small: 'result' will be 0
Definition at line 2811 of file ttmathbig.h.
uint ToDouble | ( | double & | result ) | const |
this method converts from this class into the 'double'
if the value is too big: 'result' will be +/-infinity (depending on the sign) and the method returns 1 if the value is too small: 'result' will be 0 and the method returns 1
Definition at line 2938 of file ttmathbig.h.
uint ToFloat | ( | float & | result ) | const |
this method converts from this class into the 'float'
if the value is too big: 'result' will be +/-infinity (depending on the sign) and the method returns 1 if the value is too small: 'result' will be 0 and the method returns 1
Definition at line 2901 of file ttmathbig.h.
float ToFloat | ( | ) | const |
this method converts from this class into the 'float'
if the value is too big: 'result' will be +/-infinity (depending on the sign) if the value is too small: 'result' will be 0
Definition at line 2881 of file ttmathbig.h.
uint ToInt | ( | slint & | result ) | const |
this method converts 'this' into 'result' (64 bit unsigned integer) if the value is too big this method returns a carry (1)
Definition at line 3185 of file ttmathbig.h.
uint ToInt | ( | unsigned int & | result ) | const |
this method converts 'this' into 'result' (32 bit unsigned integer) this method is created only on a 64bit platform*** if the value is too big this method returns a carry (1)
Definition at line 3358 of file ttmathbig.h.
uint ToInt | ( | sint & | result ) | const |
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2356 of file ttmathbig.h.
sint ToInt | ( | ) | const |
this method converts 'this' into sint
Definition at line 2330 of file ttmathbig.h.
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2345 of file ttmathbig.h.
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2468 of file ttmathbig.h.
this method converts 'this' into 'result' (64 bit unsigned integer) if the value is too big this method returns a carry (1)
Definition at line 3175 of file ttmathbig.h.
uint ToInt | ( | signed int & | result ) | const |
this method converts 'this' into 'result' (32 bit signed integer) this method is created only on a 64bit platform*** if the value is too big this method returns a carry (1)
Definition at line 3369 of file ttmathbig.h.
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2480 of file ttmathbig.h.
uint ToString | ( | std::string & | result, |
uint | base = 10 , |
||
bool | scient = false , |
||
sint | scient_from = 15 , |
||
sint | round = -1 , |
||
bool | trim_zeroes = true , |
||
char | comma = '.' |
||
) | const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
output: return value: 0 - ok and 'result' will be an object of type std::string (or std::wstring) which holds the value 1 - if there is a carry (it shoudn't be in a normal situation - if it is that means there is somewhere an error in the library)
Definition at line 3675 of file ttmathbig.h.
std::string ToString | ( | uint | base = 10 ) |
const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3723 of file ttmathbig.h.
std::string ToString | ( | const Conv & | conv ) | const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3710 of file ttmathbig.h.
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3700 of file ttmathbig.h.
uint ToString | ( | std::wstring & | result, |
uint | base = 10 , |
||
bool | scient = false , |
||
sint | scient_from = 15 , |
||
sint | round = -1 , |
||
bool | trim_zeroes = true , |
||
wchar_t | comma = '.' |
||
) | const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3740 of file ttmathbig.h.
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3765 of file ttmathbig.h.
this method converts 'this' into 'result' (64 bit unsigned integer) if the value is too big this method returns a carry (1)
Definition at line 3160 of file ttmathbig.h.
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2448 of file ttmathbig.h.
uint ToUInt | ( | unsigned int & | result ) | const |
this method converts 'this' into 'result' (32 bit unsigned integer) this method is created only on a 64bit platform*** if the value is too big this method returns a carry (1)
Definition at line 3339 of file ttmathbig.h.
this method converts 'this' into 'result'
if the value is too big this method returns a carry (1)
Definition at line 2315 of file ttmathbig.h.
uint ToUInt | ( | ) | const |
this method converts 'this' into uint
Definition at line 2300 of file ttmathbig.h.
std::wstring ToWString | ( | uint | base = 10 ) |
const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3788 of file ttmathbig.h.
std::wstring ToWString | ( | const Conv & | conv ) | const |
a method for converting into a string struct Conv is defined in ttmathtypes.h, look there for more information about parameters
Definition at line 3775 of file ttmathbig.h.
Friends And Related Function Documentation
friend class Big< exp-1, man > [friend] |
in the method 'ToString_CreateNewMantissaAndExponent()' we're using type 'Big<exp+1,man>' and we should have the ability to use some necessary methods from that class (methods which are private here)
Definition at line 3877 of file ttmathbig.h.
std::ostream& operator<< | ( | std::ostream & | s, |
const Big< exp, man > & | l | ||
) | [friend] |
output to standard streams
Definition at line 5923 of file ttmathbig.h.
std::wostream& operator<< | ( | std::wostream & | s, |
const Big< exp, man > & | l | ||
) | [friend] |
output to standard streams
Definition at line 5934 of file ttmathbig.h.
std::istream& operator>> | ( | std::istream & | s, |
Big< exp, man > & | l | ||
) | [friend] |
input from standard streams
Definition at line 6022 of file ttmathbig.h.
std::wistream& operator>> | ( | std::wistream & | s, |
Big< exp, man > & | l | ||
) | [friend] |
input from standard streams
Definition at line 6033 of file ttmathbig.h.
Generated on Tue Jul 12 2022 14:03:18 by
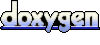