Library for big numbers from http://www.ttmath.org/
Dependents: PIDHeater82 Conceptcontroller_v_1_0 AlarmClockApp COG4050_adxl355_tilt ... more
UInt< value_size > Class Template Reference
UInt implements a big integer value without a sign. More...
#include <ttmathuint.h>
Inherited by Int< exp >, and Int< value_size >.
Public Member Functions | |
template<class ostream_type > | |
void | PrintTable (ostream_type &output) const |
template<class char_type , class ostream_type > | |
void | PrintLog (const char_type *msg, ostream_type &output) const |
template<class char_type , class ostream_type > | |
void | PrintLog (const char_type *msg, uint carry, ostream_type &output) const |
uint | Size () const |
void | SetZero () |
void | SetOne () |
void | SetMax () |
void | SetMin () |
void | Swap (UInt< value_size > &ss2) |
void | SetFromTable (const uint *temp_table, uint temp_table_len) |
void | SetFromTable (const unsigned int *temp_table, uint temp_table_len) |
uint | AddOne () |
uint | SubOne () |
uint | Rcl (uint bits, uint c=0) |
uint | Rcr (uint bits, uint c=0) |
uint | CompensationToLeft () |
bool | FindLeadingBit (uint &table_id, uint &index) const |
bool | FindLowestBit (uint &table_id, uint &index) const |
uint | GetBit (uint bit_index) const |
uint | SetBit (uint bit_index) |
void | BitAnd (const UInt< value_size > &ss2) |
void | BitOr (const UInt< value_size > &ss2) |
void | BitXor (const UInt< value_size > &ss2) |
void | BitNot () |
void | BitNot2 () |
uint | MulInt (uint ss2) |
template<uint result_size> | |
void | MulInt (uint ss2, UInt< result_size > &result) const |
uint | Mul (const UInt< value_size > &ss2, uint algorithm=100) |
void | MulBig (const UInt< value_size > &ss2, UInt< value_size *2 > &result, uint algorithm=100) |
uint | Mul1 (const UInt< value_size > &ss2) |
void | Mul1Big (const UInt< value_size > &ss2_, UInt< value_size *2 > &result) |
uint | Mul2 (const UInt< value_size > &ss2) |
void | Mul2Big (const UInt< value_size > &ss2, UInt< value_size *2 > &result) |
uint | Mul3 (const UInt< value_size > &ss2) |
void | Mul3Big (const UInt< value_size > &ss2, UInt< value_size *2 > &result) |
uint | MulFastest (const UInt< value_size > &ss2) |
void | MulFastestBig (const UInt< value_size > &ss2, UInt< value_size *2 > &result) |
uint | DivInt (uint divisor, uint *remainder=0) |
uint | Div (const UInt< value_size > &divisor, UInt< value_size > *remainder=0, uint algorithm=3) |
uint | Div1 (const UInt< value_size > &divisor, UInt< value_size > *remainder=0) |
uint | Div1 (const UInt< value_size > &divisor, UInt< value_size > &remainder) |
uint | Div2 (const UInt< value_size > &divisor, UInt< value_size > *remainder=0) |
uint | Div2 (const UInt< value_size > &divisor, UInt< value_size > &remainder) |
uint | Div3 (const UInt< value_size > &ss2, UInt< value_size > *remainder=0) |
uint | Div3 (const UInt< value_size > &ss2, UInt< value_size > &remainder) |
uint | Pow (UInt< value_size > pow) |
void | Sqrt () |
void | ClearFirstBits (uint n) |
bool | IsTheHighestBitSet () const |
bool | IsTheLowestBitSet () const |
bool | IsOnlyTheHighestBitSet () const |
bool | IsOnlyTheLowestBitSet () const |
bool | IsZero () const |
bool | AreFirstBitsZero (uint bits) const |
template<uint argument_size> | |
uint | FromUInt (const UInt< argument_size > &p) |
template<uint argument_size> | |
uint | FromInt (const UInt< argument_size > &p) |
uint | FromUInt (uint value) |
uint | FromInt (uint value) |
uint | FromInt (sint value) |
template<uint argument_size> | |
UInt< value_size > & | operator= (const UInt< argument_size > &p) |
UInt< value_size > & | operator= (const UInt< value_size > &p) |
UInt< value_size > & | operator= (uint i) |
UInt (uint i) | |
UInt< value_size > & | operator= (sint i) |
UInt (sint i) | |
uint | FromUInt (ulint n) |
uint | FromInt (ulint n) |
uint | FromInt (slint n) |
UInt< value_size > & | operator= (ulint n) |
UInt (ulint n) | |
UInt< value_size > & | operator= (slint n) |
UInt (slint n) | |
uint | FromUInt (unsigned int i) |
uint | FromInt (unsigned int i) |
uint | FromInt (signed int i) |
UInt< value_size > & | operator= (unsigned int i) |
UInt (unsigned int i) | |
UInt< value_size > & | operator= (signed int i) |
UInt (signed int i) | |
UInt (const char *s) | |
UInt (const std::string &s) | |
UInt (const wchar_t *s) | |
UInt (const std::wstring &s) | |
UInt () | |
UInt (const UInt< value_size > &u) | |
template<uint argument_size> | |
UInt (const UInt< argument_size > &u) | |
~UInt () | |
uint | ToUInt () const |
uint | ToUInt (uint &result) const |
uint | ToInt (uint &result) const |
uint | ToInt (sint &result) const |
uint | ToUInt (ulint &result) const |
uint | ToInt (ulint &result) const |
uint | ToInt (slint &result) const |
uint | ToUInt (unsigned int &result) const |
uint | ToInt (unsigned int &result) const |
uint | ToInt (int &result) const |
template<class string_type > | |
void | ToStringBase (string_type &result, uint b=10, bool negative=false) const |
void | ToString (std::string &result, uint b=10) const |
uint | FromString (const char *s, uint b=10, const char **after_source=0, bool *value_read=0) |
uint | FromString (const std::string &s, uint b=10) |
UInt< value_size > & | operator= (const char *s) |
UInt< value_size > & | operator= (const std::string &s) |
uint | FromString (const wchar_t *s, uint b=10, const wchar_t **after_source=0, bool *value_read=0) |
uint | FromString (const std::wstring &s, uint b=10) |
UInt< value_size > & | operator= (const wchar_t *s) |
UInt< value_size > & | operator= (const std::wstring &s) |
bool | CmpSmaller (const UInt< value_size > &l, sint index=-1) const |
bool | CmpBigger (const UInt< value_size > &l, sint index=-1) const |
bool | CmpEqual (const UInt< value_size > &l, sint index=-1) const |
bool | CmpSmallerEqual (const UInt< value_size > &l, sint index=-1) const |
bool | CmpBiggerEqual (const UInt< value_size > &l, sint index=-1) const |
UInt< value_size > | operator- (const UInt< value_size > &p2) const |
UInt< value_size > & | operator++ () |
UInt< value_size > | operator++ (int) |
UInt< value_size > | operator~ () const |
uint | Add (const UInt< value_size > &ss2, uint c=0) |
uint | AddInt (uint value, uint index=0) |
uint | AddTwoInts (uint x2, uint x1, uint index) |
uint | Sub (const UInt< value_size > &ss2, uint c=0) |
uint | SubInt (uint value, uint index=0) |
Static Public Member Functions | |
template<class char_type , class ostream_type > | |
static void | PrintVectorLog (const char_type *msg, ostream_type &output, const uint *vector, uint vector_len) |
template<class char_type , class ostream_type > | |
static void | PrintVectorLog (const char_type *msg, uint carry, ostream_type &output, const uint *vector, uint vector_len) |
static uint | AddTwoWords (uint a, uint b, uint carry, uint *result) |
static uint | SubTwoWords (uint a, uint b, uint carry, uint *result) |
static void | DivTwoWords2 (uint a, uint b, uint c, uint *r, uint *rest) |
static const char * | LibTypeStr () |
static LibTypeCode | LibType () |
static uint | AddVector (const uint *ss1, const uint *ss2, uint ss1_size, uint ss2_size, uint *result) |
static uint | SubVector (const uint *ss1, const uint *ss2, uint ss1_size, uint ss2_size, uint *result) |
static sint | FindLeadingBitInWord (uint x) |
static sint | FindLowestBitInWord (uint x) |
static uint | SetBitInWord (uint &value, uint bit) |
static void | MulTwoWords (uint a, uint b, uint *result_high, uint *result_low) |
static void | DivTwoWords (uint a, uint b, uint c, uint *r, uint *rest) |
Data Fields | |
uint | table [value_size] |
Protected Member Functions | |
double | ToStringLog2 (uint x) const |
Friends | |
std::ostream & | operator<< (std::ostream &s, const UInt< value_size > &l) |
std::wostream & | operator<< (std::wostream &s, const UInt< value_size > &l) |
std::istream & | operator>> (std::istream &s, UInt< value_size > &l) |
std::wistream & | operator>> (std::wistream &s, UInt< value_size > &l) |
Detailed Description
template<uint value_size>
class ttmath::UInt< value_size >
UInt implements a big integer value without a sign.
value_size - how many bytes specify our value on 32bit platforms: value_size=1 -> 4 bytes -> 32 bits on 64bit platforms: value_size=1 -> 8 bytes -> 64 bits value_size = 1,2,3,4,5,6....
Definition at line 73 of file ttmathuint.h.
Constructor & Destructor Documentation
a constructor for converting the uint to this class
Definition at line 2762 of file ttmathuint.h.
UInt | ( | sint | i ) |
a constructor for converting the sint to this class
look at the description of UInt::operator=(sint)
Definition at line 2784 of file ttmathuint.h.
a constructor for converting unsigned 64 bit int to this class this constructor is created only on a 32bit platform***
Definition at line 2861 of file ttmathuint.h.
UInt | ( | slint | n ) |
a constructor for converting signed 64 bit int to this class this constructor is created only on a 32bit platform***
Definition at line 2883 of file ttmathuint.h.
UInt | ( | unsigned int | i ) |
a constructor for converting 32 bit unsigned int to this class this constructor is created only on a 64bit platform***
Definition at line 2940 of file ttmathuint.h.
UInt | ( | signed int | i ) |
a constructor for converting 32 bit signed int to this class this constructor is created only on a 64bit platform***
Definition at line 2962 of file ttmathuint.h.
UInt | ( | const char * | s ) |
a constructor for converting a string to this class (with the base=10)
Definition at line 2977 of file ttmathuint.h.
UInt | ( | const std::string & | s ) |
a constructor for converting a string to this class (with the base=10)
Definition at line 2986 of file ttmathuint.h.
UInt | ( | const wchar_t * | s ) |
a constructor for converting a string to this class (with the base=10)
Definition at line 2997 of file ttmathuint.h.
UInt | ( | const std::wstring & | s ) |
a constructor for converting a string to this class (with the base=10)
Definition at line 3006 of file ttmathuint.h.
UInt | ( | ) |
a copy constructor
Definition at line 3042 of file ttmathuint.h.
a template for producting constructors for copying from another types
Definition at line 3056 of file ttmathuint.h.
~UInt | ( | ) |
a destructor
Definition at line 3068 of file ttmathuint.h.
Member Function Documentation
this method adding ss2 to the this and adding carry if it's defined (this = this + ss2 + c)
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it was)
basic mathematic functions
adding ss2 to the this and adding carry if it's defined (this = this + ss2 + c)
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it has been)
basic mathematic functions
this method adding ss2 to the this and adding carry if it's defined (this = this + ss2 + c)
this method is created only on a 64bit platform***
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it was)
Definition at line 141 of file ttmathuint_noasm.h.
this method adds one word (at a specific position) and returns a carry (if it was)
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: AddInt(2,1) then it'll be: table[0] = 10; table[1] = 30 + 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
adding one word (at a specific position) and returning a carry (if it has been)
e.g.
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: AddInt(2,1) then it'll be: table[0] = 10; table[1] = 30 + 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
this method adds one word (at a specific position) and returns a carry (if it was)
this method is created only on a 64bit platform***
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: AddInt(2,1) then it'll be: table[0] = 10; table[1] = 30 + 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 172 of file ttmathuint_noasm.h.
uint AddOne | ( | ) |
basic mathematic functions
this method adds one to the existing value
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 386 of file ttmathuint.h.
this method adds only two unsigned words to the existing value and these words begin on the 'index' position (it's used in the multiplication algorithm 2)
index should be equal or smaller than value_size-2 (index <= value_size-2) x1 - lower word, x2 - higher word
for example if we've got value_size equal 4 and: table[0] = 3 table[1] = 4 table[2] = 5 table[3] = 6 then let x1 = 10 x2 = 20 and index = 1
the result of this method will be: table[0] = 3 table[1] = 4 + x1 = 14 table[2] = 5 + x2 = 25 table[3] = 6
and no carry at the end of table[3]
(of course if there was a carry in table[2](5+20) then this carry would be passed to the table[3] etc.)
adding only two unsigned words to the existing value and these words begin on the 'index' position (it's used in the multiplication algorithm 2)
index should be equal or smaller than value_size-2 (index <= value_size-2) x1 - lower word, x2 - higher word
for example if we've got value_size equal 4 and: table[0] = 3 table[1] = 4 table[2] = 5 table[3] = 6 then let x1 = 10 x2 = 20 and index = 1
the result of this method will be: table[0] = 3 table[1] = 4 + x1 = 14 table[2] = 5 + x2 = 25 table[3] = 6
and no carry at the end of table[3]
(of course if there was a carry in table[2](5+20) then this carry would be passed to the table[3] etc.)
this method adds only two unsigned words to the existing value and these words begin on the 'index' position (it's used in the multiplication algorithm 2)
this method is created only on a 64bit platform***
index should be equal or smaller than value_size-2 (index <= value_size-2) x1 - lower word, x2 - higher word
for example if we've got value_size equal 4 and: table[0] = 3 table[1] = 4 table[2] = 5 table[3] = 6 then let x1 = 10 x2 = 20 and index = 1
the result of this method will be: table[0] = 3 table[1] = 4 + x1 = 14 table[2] = 5 + x2 = 25 table[3] = 6
and no carry at the end of table[3]
(of course if there was a carry in table[2](5+20) then this carry would be passed to the table[3] etc.)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 224 of file ttmathuint_noasm.h.
this method adds two words together returns carry
this method is created only when TTMATH_NOASM macro is defined
Definition at line 105 of file ttmathuint_noasm.h.
uint AddVector | ( | const uint * | ss1, |
const uint * | ss2, | ||
uint | ss1_size, | ||
uint | ss2_size, | ||
uint * | result | ||
) | [static] |
this static method addes one vector to the other 'ss1' is larger in size or equal to 'ss2'
ss1 points to the first (larger) vector ss2 points to the second vector ss1_size - size of the ss1 (and size of the result too) ss2_size - size of the ss2 result - is the result vector (which has size the same as ss1: ss1_size)
Example: ss1_size is 5, ss2_size is 3 ss1: ss2: result (output): 5 1 5+1 4 3 4+3 2 7 2+7 6 6 9 9 of course the carry is propagated and will be returned from the last item (this method is used by the Karatsuba multiplication algorithm)
Definition at line 265 of file ttmathuint_noasm.h.
bool AreFirstBitsZero | ( | uint | bits ) | const |
returning true if first 'bits' bits are equal zero
Definition at line 2594 of file ttmathuint.h.
void BitAnd | ( | const UInt< value_size > & | ss2 ) |
this method performs a bitwise operation AND
Definition at line 743 of file ttmathuint.h.
void BitNot | ( | ) |
this method performs a bitwise operation NOT
Definition at line 779 of file ttmathuint.h.
void BitNot2 | ( | ) |
this method performs a bitwise operation NOT but only on the range of <0, leading_bit>
for example: BitNot2(8) = BitNot2( 1000(bin) ) = 111(bin) = 7
Definition at line 795 of file ttmathuint.h.
void BitOr | ( | const UInt< value_size > & | ss2 ) |
this method performs a bitwise operation OR
Definition at line 755 of file ttmathuint.h.
void BitXor | ( | const UInt< value_size > & | ss2 ) |
this method performs a bitwise operation XOR
Definition at line 767 of file ttmathuint.h.
void ClearFirstBits | ( | uint | n ) |
this method sets n first bits to value zero
For example: let n=2 then if there's a value 111 (bin) there'll be '100' (bin)
Definition at line 2484 of file ttmathuint.h.
bool CmpBigger | ( | const UInt< value_size > & | l, |
sint | index = -1 |
||
) | const |
this method returns true if 'this' is bigger than 'l'
'index' is an index of the first word from will be the comparison performed (note: we start the comparison from back - from the last word, when index is -1 /default/ it is automatically set into the last word)
I introduced it for some kind of optimization made in the second division algorithm (Div2)
Definition at line 3582 of file ttmathuint.h.
bool CmpBiggerEqual | ( | const UInt< value_size > & | l, |
sint | index = -1 |
||
) | const |
this method returns true if 'this' is bigger than or equal 'l'
'index' is an index of the first word from will be the comparison performed (note: we start the comparison from back - from the last word, when index is -1 /default/ it is automatically set into the last word)
Definition at line 3665 of file ttmathuint.h.
bool CmpEqual | ( | const UInt< value_size > & | l, |
sint | index = -1 |
||
) | const |
this method returns true if 'this' is equal 'l'
'index' is an index of the first word from will be the comparison performed (note: we start the comparison from back - from the last word, when index is -1 /default/ it is automatically set into the last word)
Definition at line 3610 of file ttmathuint.h.
bool CmpSmaller | ( | const UInt< value_size > & | l, |
sint | index = -1 |
||
) | const |
methods for comparing
this method returns true if 'this' is smaller than 'l'
'index' is an index of the first word from will be the comparison performed (note: we start the comparison from back - from the last word, when index is -1 /default/ it is automatically set into the last word) I introduced it for some kind of optimization made in the second division algorithm (Div2)
Definition at line 3551 of file ttmathuint.h.
bool CmpSmallerEqual | ( | const UInt< value_size > & | l, |
sint | index = -1 |
||
) | const |
this method returns true if 'this' is smaller than or equal 'l'
'index' is an index of the first word from will be the comparison performed (note: we start the comparison from back - from the last word, when index is -1 /default/ it is automatically set into the last word)
Definition at line 3636 of file ttmathuint.h.
uint CompensationToLeft | ( | ) |
this method moves all bits into the left side (it returns value how many bits have been moved)
Definition at line 598 of file ttmathuint.h.
uint Div | ( | const UInt< value_size > & | divisor, |
UInt< value_size > * | remainder = 0 , |
||
uint | algorithm = 3 |
||
) |
division this = this / ss2
return values: 0 - ok 1 - division by zero 'this' will be the quotient 'remainder' - remainder
Definition at line 1603 of file ttmathuint.h.
the first division algorithm radix 2
Definition at line 1729 of file ttmathuint.h.
the first division algorithm radix 2
Definition at line 1752 of file ttmathuint.h.
the second division algorithm
return values: 0 - ok 1 - division by zero
Definition at line 1839 of file ttmathuint.h.
the second division algorithm
return values: 0 - ok 1 - division by zero
Definition at line 1860 of file ttmathuint.h.
the third division algorithm
Definition at line 2102 of file ttmathuint.h.
the third division algorithm
Definition at line 2085 of file ttmathuint.h.
Division
division by one unsigned word
returns 1 when divisor is zero
Definition at line 1545 of file ttmathuint.h.
Division
this method calculates 64bits word a:b / 32bits c (a higher, b lower word) r = a:b / c and rest - remainder
WARNING: the c has to be suitably large for the result being keeped in one word, if c is equal zero there'll be a hardware interruption (0) and probably the end of your program
Division
this method calculates 64bits word a:b / 32bits c (a higher, b lower word) r = a:b / c and rest - remainder
WARNING: if r (one word) is too small for the result or c is equal zero there'll be a hardware interruption (0) and probably the end of your program
Division
this method calculates 64bits word a:b / 32bits c (a higher, b lower word) r = a:b / c and rest - remainder
this method is created only on a 64bit platform***
WARNING: if r (one word) is too small for the result or c is equal zero there'll be a hardware interruption (0) and probably the end of your program
Definition at line 776 of file ttmathuint_noasm.h.
this method is available only on 64bit platforms
the same algorithm like the third division algorithm in ttmathuint.h but now with the radix=2^32
Definition at line 852 of file ttmathuint_noasm.h.
this method looks for the highest set bit
result: if 'this' is not zero: return value - true 'table_id' - the index of a word <0..value_size-1> 'index' - the index of this set bit in the word <0..TTMATH_BITS_PER_UINT)
if 'this' is zero: return value - false both 'table_id' and 'index' are zero
Definition at line 649 of file ttmathuint.h.
sint FindLeadingBitInWord | ( | uint | x ) | [static] |
this method returns the number of the highest set bit in x if the 'x' is zero this method returns '-1'
Definition at line 577 of file ttmathuint_noasm.h.
this method looks for the smallest set bit
result: if 'this' is not zero: return value - true 'table_id' - the index of a word <0..value_size-1> 'index' - the index of this set bit in the word <0..TTMATH_BITS_PER_UINT)
if 'this' is zero: return value - false both 'table_id' and 'index' are zero
Definition at line 681 of file ttmathuint.h.
sint FindLowestBitInWord | ( | uint | x ) | [static] |
this method returns the number of the highest set bit in x if the 'x' is zero this method returns '-1'
Definition at line 600 of file ttmathuint_noasm.h.
uint FromInt | ( | slint | n ) |
this method converts signed 64 bit int type to this class this method is created only on a 32bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2834 of file ttmathuint.h.
this method converts unsigned 64 bit int type to this class this method is created only on a 32bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2824 of file ttmathuint.h.
uint FromInt | ( | signed int | i ) |
this method converts 32 bit signed int type to this class this operator is created only on a 64bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2918 of file ttmathuint.h.
uint FromInt | ( | unsigned int | i ) |
this method converts 32 bit unsigned int type to this class this operator is created only on a 64bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2908 of file ttmathuint.h.
this method converts an UInt<another_size> type to this class
this operation has mainly sense if the value from p is equal or smaller than that one which is returned from UInt<value_size>::SetMax()
it returns a carry if the value 'p' is too big
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2674 of file ttmathuint.h.
this method converts the uint type to this class
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2700 of file ttmathuint.h.
uint FromInt | ( | sint | value ) |
this method converts the sint type to this class
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2709 of file ttmathuint.h.
uint FromString | ( | const char * | s, |
uint | b = 10 , |
||
const char ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
this method converts a string into its value it returns carry=1 if the value will be too big or an incorrect base 'b' is given
string is ended with a non-digit value, for example: "12" will be translated to 12 as well as: "12foo" will be translated to 12 too
existing first white characters will be ommited
if the value from s is too large the rest digits will be skipped
after_source (if exists) is pointing at the end of the parsed string
value_read (if exists) tells whether something has actually been read (at least one digit)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3450 of file ttmathuint.h.
this method converts a string into its value
(it returns carry=1 if the value will be too big or an incorrect base 'b' is given)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3461 of file ttmathuint.h.
uint FromString | ( | const wchar_t * | s, |
uint | b = 10 , |
||
const wchar_t ** | after_source = 0 , |
||
bool * | value_read = 0 |
||
) |
this method converts a string into its value
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3495 of file ttmathuint.h.
this method converts a string into its value
(it returns carry=1 if the value will be too big or an incorrect base 'b' is given)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3506 of file ttmathuint.h.
uint FromUInt | ( | unsigned int | i ) |
this method converts 32 bit unsigned int type to this class this operator is created only on a 64bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2899 of file ttmathuint.h.
this method converts unsigned 64 bit int type to this class this method is created only on a 32bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2797 of file ttmathuint.h.
conversion methods
this method converts an UInt<another_size> type to this class
this operation has mainly sense if the value from p is equal or smaller than that one which is returned from UInt<value_size>::SetMax()
it returns a carry if the value 'p' is too big
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2633 of file ttmathuint.h.
this method converts the uint type to this class
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2683 of file ttmathuint.h.
getting the 'bit_index' bit
bit_index bigger or equal zero
Definition at line 706 of file ttmathuint.h.
bool IsOnlyTheHighestBitSet | ( | ) | const |
returning true if only the highest bit is set
Definition at line 2541 of file ttmathuint.h.
bool IsOnlyTheLowestBitSet | ( | ) | const |
returning true if only the lowest bit is set
Definition at line 2565 of file ttmathuint.h.
bool IsTheHighestBitSet | ( | ) | const |
this method returns true if the highest bit of the value is set
Definition at line 2523 of file ttmathuint.h.
bool IsTheLowestBitSet | ( | ) | const |
this method returns true if the lowest bit of the value is set
Definition at line 2532 of file ttmathuint.h.
bool IsZero | ( | ) | const |
this method returns true if the value is equal zero
Definition at line 2581 of file ttmathuint.h.
LibTypeCode LibType | ( | ) | [static] |
returning the currect type of the library
Definition at line 84 of file ttmathuint_noasm.h.
const char * LibTypeStr | ( | ) | [static] |
returning the string represents the currect type of the library we have following types: asm_vc_32 - with asm code designed for Microsoft Visual C++ (32 bits) asm_gcc_32 - with asm code designed for GCC (32 bits) asm_vc_64 - with asm for VC (64 bit) asm_gcc_64 - with asm for GCC (64 bit) no_asm_32 - pure C++ version (32 bit) - without any asm code no_asm_64 - pure C++ version (64 bit) - without any asm code
Definition at line 66 of file ttmathuint_noasm.h.
the multiplication 'this' = 'this' * ss2
algorithm: 100 - means automatically choose the fastest algorithm
Definition at line 923 of file ttmathuint.h.
multiplication: this = this * ss2 can return carry
Definition at line 1020 of file ttmathuint.h.
multiplication: result = this * ss2
result is twice bigger than 'this' and 'ss2' this method never returns carry
Definition at line 1040 of file ttmathuint.h.
the second version of the multiplication algorithm
this algorithm is similar to the 'schoolbook method' which is done by hand
multiplication: this = this * ss2
it returns carry if it has been
Definition at line 1079 of file ttmathuint.h.
multiplication: result = this * ss2
result is twice bigger than this and ss2 this method never returns carry
Definition at line 1110 of file ttmathuint.h.
multiplication: this = this * ss2
This is Karatsuba Multiplication algorithm, we're using it when value_size is greater than or equal to TTMATH_USE_KARATSUBA_MULTIPLICATION_FROM_SIZE macro (defined in ttmathuint.h). If value_size is smaller then we're using Mul2Big() instead.
Karatsuba multiplication: Assume we have: this = x = x1*B^m + x0 ss2 = y = y1*B^m + y0 where x0 and y0 are less than B^m the product from multiplication we can show as: x*y = (x1*B^m + x0)(y1*B^m + y0) = z2*B^(2m) + z1*B^m + z0 where z2 = x1*y1 z1 = x1*y0 + x0*y1 z0 = x0*y0 this is standard schoolbook algorithm with O(n^2), Karatsuba observed that z1 can be given in other form: z1 = (x1 + x0)*(y1 + y0) - z2 - z0 / z1 = (x1*y1 + x1*y0 + x0*y1 + x0*y0) - x1*y1 - x0*y0 = x1*y0 + x0*y1 / and to calculate the multiplication we need only three multiplications (with some additions and subtractions)
Our objects 'this' and 'ss2' we divide into two parts and by using recurrence we calculate the multiplication. Karatsuba multiplication has O( n^(ln(3)/ln(2)) )
Definition at line 1203 of file ttmathuint.h.
multiplication: result = this * ss2
result is twice bigger than this and ss2, this method never returns carry, (Karatsuba multiplication)
Definition at line 1236 of file ttmathuint.h.
void MulBig | ( | const UInt< value_size > & | ss2, |
UInt< value_size *2 > & | result, | ||
uint | algorithm = 100 |
||
) |
the multiplication 'result' = 'this' * ss2
since the 'result' is twice bigger than 'this' and 'ss2' this method never returns a carry
algorithm: 100 - means automatically choose the fastest algorithm
Definition at line 951 of file ttmathuint.h.
multiplication this = this * ss2
Definition at line 1462 of file ttmathuint.h.
multiplication result = this * ss2
this method is trying to select the fastest algorithm (in the future this method can be improved)
Definition at line 1493 of file ttmathuint.h.
multiplication: result = this * ss2
we're using this method only when result_size is greater than value_size if so there will not be a carry
Definition at line 873 of file ttmathuint.h.
Multiplication
multiplication: this = this * ss2
it can return a carry
Definition at line 835 of file ttmathuint.h.
Multiplication
multiplication: result_high:result_low = a * b result_high - higher word of the result result_low - lower word of the result
this methos never returns a carry this method is used in the second version of the multiplication algorithms
multiplication: result_high:result_low = a * b result_high - higher word of the result result_low - lower word of the result
this methos never returns a carry this method is used in the second version of the multiplication algorithms
Multiplication
multiplication: result_high:result_low = a * b result_high - higher word of the result result_low - lower word of the result
this methos never returns a carry this method is used in the second version of the multiplication algorithms
this method is created only on a 64bit platform***
Definition at line 667 of file ttmathuint_noasm.h.
UInt<value_size>& operator++ | ( | ) |
Prefix operator e.g ++variable
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3825 of file ttmathuint.h.
UInt<value_size> operator++ | ( | int | ) |
Postfix operator e.g variable++
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3836 of file ttmathuint.h.
standard mathematical operators
Definition at line 3731 of file ttmathuint.h.
the assignment operator
Definition at line 2737 of file ttmathuint.h.
this method converts the uint type to this class
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2751 of file ttmathuint.h.
UInt<value_size>& operator= | ( | sint | i ) |
this method converts the sint type to this class
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2771 of file ttmathuint.h.
this operator converts an UInt<another_size> type to this class
it doesn't return a carry
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2726 of file ttmathuint.h.
UInt<value_size>& operator= | ( | signed int | i ) |
an operator for converting 32 bit signed int to this class this constructor is created only on a 64bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2950 of file ttmathuint.h.
UInt<value_size>& operator= | ( | unsigned int | i ) |
this operator converts 32 bit unsigned int type to this class this operator is created only on a 64bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2928 of file ttmathuint.h.
UInt<value_size>& operator= | ( | const char * | s ) |
this operator converts a string into its value (with base = 10)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3470 of file ttmathuint.h.
UInt<value_size>& operator= | ( | const std::string & | s ) |
this operator converts a string into its value (with base = 10)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3481 of file ttmathuint.h.
this operator converts unsigned 64 bit int type to this class this operator is created only on a 32bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2849 of file ttmathuint.h.
UInt<value_size>& operator= | ( | const std::wstring & | s ) |
this operator converts a string into its value (with base = 10)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3526 of file ttmathuint.h.
UInt<value_size>& operator= | ( | const wchar_t * | s ) |
this operator converts a string into its value (with base = 10)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3515 of file ttmathuint.h.
UInt<value_size>& operator= | ( | slint | n ) |
this operator converts signed 64 bit int type to this class this operator is created only on a 32bit platform***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 2871 of file ttmathuint.h.
UInt<value_size> operator~ | ( | ) | const |
bitwise operators
Definition at line 3871 of file ttmathuint.h.
power this = this ^ pow binary algorithm (r-to-l)
return values: 0 - ok 1 - carry 2 - incorrect argument (0^0)
Definition at line 2402 of file ttmathuint.h.
void PrintLog | ( | const char_type * | msg, |
uint | carry, | ||
ostream_type & | output | ||
) | const |
this method is used when macro TTMATH_DEBUG_LOG is defined
Definition at line 169 of file ttmathuint.h.
void PrintLog | ( | const char_type * | msg, |
ostream_type & | output | ||
) | const |
this method is used when macro TTMATH_DEBUG_LOG is defined
Definition at line 159 of file ttmathuint.h.
void PrintTable | ( | ostream_type & | output ) | const |
some methods used for debugging purposes
this method is only for debugging purposes or when we want to make a table of a variable (constant) in ttmathbig.h
it prints the table in a nice form of several columns
Definition at line 97 of file ttmathuint.h.
static void PrintVectorLog | ( | const char_type * | msg, |
ostream_type & | output, | ||
const uint * | vector, | ||
uint | vector_len | ||
) | [static] |
this method is used when macro TTMATH_DEBUG_LOG is defined
Definition at line 135 of file ttmathuint.h.
static void PrintVectorLog | ( | const char_type * | msg, |
uint | carry, | ||
ostream_type & | output, | ||
const uint * | vector, | ||
uint | vector_len | ||
) | [static] |
this method is used when macro TTMATH_DEBUG_LOG is defined
Definition at line 148 of file ttmathuint.h.
moving all bits into the left side 'bits' times return value <- this <- C
bits is from a range of <0, man * TTMATH_BITS_PER_UINT> or it can be even bigger then all bits will be set to 'c'
the value c will be set into the lowest bits and the method returns state of the last moved bit
Definition at line 460 of file ttmathuint.h.
moving all bits into the right side 'bits' times c -> this -> return value
bits is from a range of <0, man * TTMATH_BITS_PER_UINT> or it can be even bigger then all bits will be set to 'c'
the value c will be set into the highest bits and the method returns state of the last moved bit
Definition at line 555 of file ttmathuint.h.
setting the 'bit_index' bit and returning the last state of the bit
bit_index bigger or equal zero
Definition at line 726 of file ttmathuint.h.
this method sets a special bit in the 'value' and returns the last state of the bit (zero or one)
bit is from <0,TTMATH_BITS_PER_UINT-1>
e.g. uint x = 100; uint bit = SetBitInWord(x, 3); now: x = 108 and bit = 0
this method sets a special bit in the 'value' and returns the last state of the bit (zero or one)
bit is from <0,31> e.g. uint x = 100; uint bit = SetBitInWord(x, 3); now: x = 108 and bit = 0
this method sets a special bit in the 'value' and returns the last state of the bit (zero or one)
this method is created only on a 64bit platform***
bit is from <0,63>
e.g. uint x = 100; uint bit = SetBitInWord(x, 3); now: x = 108 and bit = 0
Definition at line 630 of file ttmathuint_noasm.h.
this method copies the value stored in an another table (warning: first values in temp_table are the highest words -- it's different from our table)
we copy as many words as it is possible
if temp_table_len is bigger than value_size we'll try to round the lowest word from table depending on the last not used bit in temp_table (this rounding isn't a perfect rounding -- look at the description below)
and if temp_table_len is smaller than value_size we'll clear the rest words in the table
Definition at line 266 of file ttmathuint.h.
void SetFromTable | ( | const unsigned int * | temp_table, |
uint | temp_table_len | ||
) |
this method copies the value stored in an another table (warning: first values in temp_table are the highest words -- it's different from our table)
this method is created only on a 64bit platform***
we copy as many words as it is possible
if temp_table_len is bigger than value_size we'll try to round the lowest word from table depending on the last not used bit in temp_table (this rounding isn't a perfect rounding -- look at the description below)
and if temp_table_len is smaller than value_size we'll clear the rest words in the table
warning: we're using 'temp_table' as a pointer at 32bit words
Definition at line 325 of file ttmathuint.h.
void SetMax | ( | ) |
this method sets the max value which this class can hold (all bits will be one)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 215 of file ttmathuint.h.
void SetMin | ( | ) |
this method sets the min value which this class can hold (for an unsigned integer value the zero is the smallest value)
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 228 of file ttmathuint.h.
void SetOne | ( | ) |
this method sets one
Definition at line 202 of file ttmathuint.h.
void SetZero | ( | ) |
this method sets zero
Definition at line 188 of file ttmathuint.h.
uint Size | ( | ) | const |
this method returns the size of the table
Definition at line 179 of file ttmathuint.h.
void Sqrt | ( | ) |
square root e.g. Sqrt(9) = 3 ('digit-by-digit' algorithm)
Definition at line 2438 of file ttmathuint.h.
this method's subtracting ss2 from the 'this' and subtracting carry if it has been defined (this = this - ss2 - c)
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it was)
subtracting ss2 from the 'this' and subtracting carry if it has been defined (this = this - ss2 - c)
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it has been)
this method's subtracting ss2 from the 'this' and subtracting carry if it has been defined (this = this - ss2 - c)
this method is created only on a 64bit platform***
c must be zero or one (might be a bigger value than 1) function returns carry (1) (if it was)
Definition at line 325 of file ttmathuint_noasm.h.
this method subtracts one word (at a specific position) and returns a carry (if it was)
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: SubInt(2,1) then it'll be: table[0] = 10; table[1] = 30 - 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
this method subtracts one word (at a specific position) and returns a carry (if it was)
e.g.
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: SubInt(2,1) then it'll be: table[0] = 10; table[1] = 30 - 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
this method subtracts one word (at a specific position) and returns a carry (if it was)
this method is created only on a 64bit platform***
if we've got (value_size=3): table[0] = 10; table[1] = 30; table[2] = 5; and we call: SubInt(2,1) then it'll be: table[0] = 10; table[1] = 30 - 2; table[2] = 5;
of course if there was a carry from table[2] it would be returned
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 358 of file ttmathuint_noasm.h.
uint SubOne | ( | ) |
this method subtracts one from the existing value
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 395 of file ttmathuint.h.
this method subtractes one word from the other returns carry
this method is created only when TTMATH_NOASM macro is defined
Definition at line 292 of file ttmathuint_noasm.h.
uint SubVector | ( | const uint * | ss1, |
const uint * | ss2, | ||
uint | ss1_size, | ||
uint | ss2_size, | ||
uint * | result | ||
) | [static] |
this static method subtractes one vector from the other 'ss1' is larger in size or equal to 'ss2'
ss1 points to the first (larger) vector ss2 points to the second vector ss1_size - size of the ss1 (and size of the result too) ss2_size - size of the ss2 result - is the result vector (which has size the same as ss1: ss1_size)
Example: ss1_size is 5, ss2_size is 3 ss1: ss2: result (output): 5 1 5-1 4 3 4-3 2 7 2-7 6 6-1 (the borrow from previous item) 9 9 return (carry): 0 of course the carry (borrow) is propagated and will be returned from the last item (this method is used by the Karatsuba multiplication algorithm)
Definition at line 398 of file ttmathuint_noasm.h.
void Swap | ( | UInt< value_size > & | ss2 ) |
this method swappes this for an argument
Definition at line 239 of file ttmathuint.h.
uint ToInt | ( | sint & | result ) | const |
this method converts the value to sint type (signed integer) can return a carry if the value is too long to store it in sint type
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3115 of file ttmathuint.h.
uint ToInt | ( | slint & | result ) | const |
this method converts the value to slint type (64 bit signed integer) can return a carry if the value is too long to store it in slint type this method is created only on a 32 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3176 of file ttmathuint.h.
this method converts the value to uint type can return a carry if the value is too long to store it in uint type
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3105 of file ttmathuint.h.
uint ToInt | ( | unsigned int & | result ) | const |
this method converts the value to a 32 unsigned integer can return a carry if the value is too long to store it in this type this method is created only on a 64 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3220 of file ttmathuint.h.
this method converts the value to ulint type (64 bit unsigned integer) can return a carry if the value is too long to store it in ulint type this method is created only on a 32 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3165 of file ttmathuint.h.
uint ToInt | ( | int & | result ) | const |
this method converts the value to a 32 signed integer can return a carry if the value is too long to store it in this type this method is created only on a 64 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3231 of file ttmathuint.h.
void ToString | ( | std::string & | result, |
uint | b = 10 |
||
) | const |
this method converts the value to a string with a base equal 'b'
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3346 of file ttmathuint.h.
void ToStringBase | ( | string_type & | result, |
uint | b = 10 , |
||
bool | negative = false |
||
) | const |
an auxiliary method for converting to a string it's used from Int::ToString() too (negative is set true then)
Definition at line 3292 of file ttmathuint.h.
double ToStringLog2 | ( | uint | x ) | const [protected] |
an auxiliary method for converting into the string it returns the log (with the base 2) from x where x is in <2;16>
Definition at line 3257 of file ttmathuint.h.
uint ToUInt | ( | unsigned int & | result ) | const |
this method converts the value to a 32 unsigned integer can return a carry if the value is too long to store it in this type this method is created only on a 64 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3200 of file ttmathuint.h.
uint ToUInt | ( | ) | const |
this method returns the lowest value from table
we must be sure when we using this method whether the value will hold in an uint type or not (the rest value from the table must be zero)
Definition at line 3079 of file ttmathuint.h.
this method converts the value to ulint type (64 bit unsigned integer) can return a carry if the value is too long to store it in ulint type this method is created only on a 32 bit platform ***
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3137 of file ttmathuint.h.
this method converts the value to uint type can return a carry if the value is too long to store it in uint type
Reimplemented in Int< value_size >, and Int< exp >.
Definition at line 3089 of file ttmathuint.h.
Friends And Related Function Documentation
std::ostream& operator<< | ( | std::ostream & | s, |
const UInt< value_size > & | l | ||
) | [friend] |
output to standard streams
Definition at line 4004 of file ttmathuint.h.
std::wostream& operator<< | ( | std::wostream & | s, |
const UInt< value_size > & | l | ||
) | [friend] |
output to standard streams
Definition at line 4015 of file ttmathuint.h.
std::istream& operator>> | ( | std::istream & | s, |
UInt< value_size > & | l | ||
) | [friend] |
input from standard streams
Definition at line 4062 of file ttmathuint.h.
std::wistream& operator>> | ( | std::wistream & | s, |
UInt< value_size > & | l | ||
) | [friend] |
input from standard streams
Definition at line 4073 of file ttmathuint.h.
Field Documentation
buffer for the integer value table[0] - the lowest word of the value
Definition at line 81 of file ttmathuint.h.
Generated on Tue Jul 12 2022 14:03:19 by
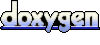