
Simply Clock
Dependencies: EthernetNetIf NTPClient_NetServices TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "time.h" 00003 #include "TextLCD.h" 00004 #include "EthernetNetIf.h" 00005 #include "NTPClient.h" 00006 00007 #define TIMEZONE (9 * 60 * 60) 00008 00009 TextLCD lcd(p21, p22, p23, p24, p25, p26); 00010 00011 EthernetNetIf eth; 00012 NTPClient ntp; 00013 00014 unsigned long convert_gregorian_to_julian(int year, int month, int day) 00015 { 00016 if (month < 3) { 00017 month += 9; 00018 year--; 00019 } else { 00020 month -= 3; 00021 } 00022 year += 4800; 00023 int c = year / 100; 00024 return c * 146097 / 4 + (year - c * 100) * 1461 / 4 + (153 * month + 2) / 5 + day - 32045; 00025 } 00026 00027 void convert_julian_to_gregorian(unsigned long j, int *year, int *month, int *day) 00028 { 00029 int y, m, d; 00030 y = (j * 4 + 128179) / 146097; 00031 d = (j * 4 - y * 146097 + 128179) / 4 * 4 + 3; 00032 j = d / 1461; 00033 d = (d - j * 1461) / 4 * 5 + 2; 00034 m = d / 153; 00035 d = (d - m * 153) / 5 + 1; 00036 y = (y - 48) * 100 + j; 00037 if (m < 10) { 00038 m += 3; 00039 } else { 00040 m -= 9; 00041 y++; 00042 } 00043 *year = y; 00044 *month = m; 00045 *day = d; 00046 } 00047 00048 void display(int year, int month, int day, int hour, int minute, int second) 00049 { 00050 lcd.cls(); 00051 lcd.printf("%04u-%02u-%02u\n", year, month, day); 00052 lcd.printf("%02u:%02u:%02u\n", hour, minute, second); 00053 } 00054 00055 int main() 00056 { 00057 eth.setup(); 00058 00059 Host server(IpAddr(), 123, "ntp.jst.mfeed.ad.jp"); 00060 00061 bool adjust = true; 00062 00063 double last = 0; 00064 00065 while (1) { 00066 if (adjust) { 00067 ntp.setTime(server); 00068 adjust = false; 00069 } else { 00070 wait(0.1); 00071 } 00072 00073 time_t t = time(0); 00074 double s = t + 2440588.0 * 24 * 60 * 60; // chronological julian second 00075 00076 if (s > last) { 00077 last = s; 00078 00079 s += TIMEZONE; 00080 00081 unsigned long cjd = (unsigned long)(s / (24 * 60 * 60)); // chronological julian day 00082 int year, month, day, hour, minute, second; 00083 convert_julian_to_gregorian(cjd, &year, &month, &day); 00084 second = (int)fmod(s, 24 * 60 * 60); 00085 hour = second / (60 * 60); 00086 minute = second / 60 % 60; 00087 second %= 60; 00088 00089 display(year, month, day, hour, minute, second); 00090 00091 if (minute == 59 && second == 30) { 00092 adjust = true; 00093 } 00094 } 00095 } 00096 00097 return 0; 00098 } 00099
Generated on Tue Jul 19 2022 02:33:30 by
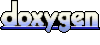