
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskAcceleration.h
00001 /** 00002 * @file TaskAcceleration.h 00003 * 00004 * @author Adrian 00005 * @date 30.05.2016 00006 * 00007 */ 00008 #include "MPU9250.h " 00009 #include "MPU9250AccelerationMessage.h " 00010 #include "main.h" 00011 00012 #ifndef TASKACCELERATION_H_ 00013 #define TASKACCELERATION_H_ 00014 00015 /** 00016 * @class TaskAcceleration 00017 * @brief This TaskAcceleration Class handles the acceleration measurement using the MPU9250. 00018 * Starting the task using the start() starts the measurement. 00019 * It can be used alongside with other measurement Tasks inside the mbed::rtos 00020 * environment. The Task Class basically wraps mbeds Thread functionality. 00021 */ 00022 class TaskAcceleration { 00023 public: 00024 TaskAcceleration(MPU9250*,Mutex*, Queue<MPU9250AccelerationMessage,ACCELERATION_QUEUE_LENGHT>*); 00025 TaskAcceleration(MPU9250*,Mutex*,Queue<MPU9250AccelerationMessage,ACCELERATION_QUEUE_LENGHT>*, 00026 osPriority, uint32_t, unsigned char*); 00027 virtual ~TaskAcceleration(); 00028 00029 00030 /** 00031 * Starts the task by building and its measurement 00032 * @return 00033 */ 00034 osStatus start(); 00035 00036 /** 00037 * Stops the task. Should only be used after start() was used 00038 * @return 00039 */ 00040 osStatus stop(); 00041 00042 00043 /** 00044 * Gets the actual state of the Task either RUNNING or SLEEPING 00045 * @return 00046 */ 00047 TASK_STATE getState(); 00048 00049 private: 00050 rtos::Thread* thread; 00051 rtos::Queue<MPU9250AccelerationMessage,ACCELERATION_QUEUE_LENGHT>* queue; 00052 rtos::Mutex* mutexI2C; 00053 osPriority priority; 00054 uint32_t stack_size; 00055 unsigned char *stack_pointer; 00056 00057 TASK_STATE state; 00058 00059 MPU9250* mpu9250; 00060 00061 00062 /** 00063 * @brief A Callback function thats called by the mbed::Thread of this TaskClass 00064 * @param 00065 */ 00066 static void callBack(void const *); 00067 00068 /** 00069 * @brief A thread safe method that measures the acceleration. After measuring the acceleration 00070 * of each axis it stores the value inside a MPU9250AccelerationMessage 00071 */ 00072 void measureAcceleration(); 00073 00074 00075 /** 00076 * @brief Sets the message Queue of the Task where the measured values will be stored 00077 * after the measurement 00078 * @param queueAcceleration the queue where the MPU9250AccelerationMessage will be stored 00079 */ 00080 void setQueue(Queue<MPU9250AccelerationMessage,ACCELERATION_QUEUE_LENGHT>* queueAcceleration); 00081 00082 /** 00083 * @brief Sets the mutex thats used for a thread safe measurement 00084 * @param mutexI2C the I2C mutex 00085 */ 00086 void setMutex(Mutex* mutexI2C); 00087 00088 /** 00089 * @brief Sets the priority of the Task 00090 * @param priority priority of the Task 00091 */ 00092 void setPriority(osPriority priority); 00093 00094 /** 00095 * @brief Sets the size of the Task 00096 * @param stackSize the stack size in Bytes 00097 */ 00098 void setStackSize(uint32_t stackSize); 00099 00100 /** 00101 * @brief Sets the stack pointer of for the task stack 00102 * @param stackPointer 00103 */ 00104 void setStackPointer(unsigned char* stackPointer); 00105 00106 00107 /** 00108 * @brief Sets the actual state of the Task 00109 * @param taskState either RUNNING or SLEEPING 00110 */ 00111 void setState(TASK_STATE taskState); 00112 00113 }; 00114 00115 #endif /* TASKACCELERATION_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
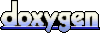