LinkSprite LS-Y201 JPEG Color Camera.
Dependents: Camera_LS_Y201_TestProgram Camera_LS_Y201_TestProgram ServoCamV1 RFIDdoor ... more
Camera_LS_Y201.h
00001 /** 00002 * ============================================================================= 00003 * LS-Y201 device driver class (Version 0.0.1) 00004 * Reference documents: LinkSprite JPEG Color Camera Serial UART Interface 00005 * January 2010 00006 * ============================================================================= 00007 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 * ============================================================================= 00027 */ 00028 00029 #ifndef LS_Y201_H 00030 #define LS_Y201_H 00031 00032 #include "mbed.h" 00033 #include "SerialBuffered.h" 00034 00035 /** 00036 * Camera 00037 */ 00038 class Camera_LS_Y201 { 00039 public: 00040 00041 /** 00042 * Create. 00043 * 00044 * @param tx Transmitter. 00045 * @param rx Receiver. 00046 */ 00047 Camera_LS_Y201(PinName tx, PinName rx); 00048 00049 /** 00050 * Dispose. 00051 */ 00052 ~Camera_LS_Y201(); 00053 00054 /** 00055 * Error code. 00056 */ 00057 enum ErrorCode { 00058 NoError = 0, 00059 UnexpectedReply, 00060 Timeout, 00061 SendError, 00062 RecvError, 00063 InvalidArguments 00064 }; 00065 00066 /** 00067 * Image size. 00068 */ 00069 enum ImageSize { 00070 ImageSize160x120, /**< 160x120. */ 00071 ImageSize320x280, /**< 320x280. */ 00072 ImageSize640x480 /**< 640x480. */ 00073 }; 00074 00075 /** 00076 * Reset module. 00077 * 00078 * @return Error code. 00079 */ 00080 ErrorCode reset(); 00081 00082 /** 00083 * Set image size. 00084 * 00085 * @param is Image size. 00086 * @return Error code. 00087 */ 00088 ErrorCode setImageSize(ImageSize is); 00089 00090 /** 00091 * Take picture. 00092 * 00093 * @return Error code. 00094 */ 00095 ErrorCode takePicture(); 00096 00097 /** 00098 * Read jpeg file size. 00099 * 00100 * @param fileSize File size. 00101 * @return Error code. 00102 */ 00103 ErrorCode readJpegFileSize(int *fileSize); 00104 00105 /** 00106 * Read jpeg file content. 00107 * 00108 * @param func A pointer to a call back function. 00109 * @return Error code. 00110 */ 00111 ErrorCode readJpegFileContent(void (*func)(int done, int total, uint8_t *buf, size_t siz)); 00112 00113 /** 00114 * Stop taking pictures. 00115 * 00116 * @return Error code. 00117 */ 00118 ErrorCode stopTakingPictures(); 00119 00120 private: 00121 SerialBuffered serial; 00122 00123 /** 00124 * Wait init end codes. 00125 * 00126 * @return Error code. 00127 */ 00128 ErrorCode waitInitEnd(); 00129 00130 /** 00131 * Send bytes to camera module. 00132 * 00133 * @param buf Pointer to the data buffer. 00134 * @param len Length of the data buffer. 00135 * 00136 * @return True if the data sended. 00137 */ 00138 bool sendBytes(uint8_t *buf, size_t len, int timeout_us); 00139 00140 /** 00141 * Receive bytes from camera module. 00142 * 00143 * @param buf Pointer to the data buffer. 00144 * @param len Length of the data buffer. 00145 * 00146 * @return True if the data received. 00147 */ 00148 bool recvBytes(uint8_t *buf, size_t len, int timeout_us); 00149 00150 /** 00151 * Wait received. 00152 * 00153 * @return True if the data received. 00154 */ 00155 bool waitRecv(); 00156 00157 /** 00158 * Wait idle state. 00159 * 00160 * @return True if it succeed. 00161 */ 00162 bool waitIdle(); 00163 00164 }; 00165 00166 #endif
Generated on Tue Jul 12 2022 20:55:29 by
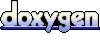