Interface library for ST LSM303DLM 3-axis magnetometer/accelerometer
Dependents: AVC_2012 m3pi_Kompass Fish_2014Fall Fish_2014Fall ... more
LSM303DLM.h
00001 #ifndef __LSM303DLM_H 00002 #define __LSM303DLM_H 00003 00004 #include "mbed.h" 00005 00006 // register addresses 00007 00008 #define CTRL_REG1_A 0x20 00009 #define CTRL_REG2_A 0x21 00010 #define CTRL_REG3_A 0x22 00011 #define CTRL_REG4_A 0x23 00012 #define CTRL_REG5_A 0x24 00013 #define CTRL_REG6_A 0x25 // DLHC only 00014 #define HP_FILTER_RESET_A 0x25 // DLH, DLM only 00015 #define REFERENCE_A 0x26 00016 #define STATUS_REG_A 0x27 00017 00018 #define OUT_X_L_A 0x28 00019 #define OUT_X_H_A 0x29 00020 #define OUT_Y_L_A 0x2A 00021 #define OUT_Y_H_A 0x2B 00022 #define OUT_Z_L_A 0x2C 00023 #define OUT_Z_H_A 0x2D 00024 00025 #define INT1_CFG_A 0x30 00026 #define INT1_SRC_A 0x31 00027 #define INT1_THS_A 0x32 00028 #define INT1_DURATION_A 0x33 00029 #define INT2_CFG_A 0x34 00030 #define INT2_SRC_A 0x35 00031 #define INT2_THS_A 0x36 00032 #define INT2_DURATION_A 0x37 00033 00034 #define CRA_REG_M 0x00 00035 #define CRB_REG_M 0x01 00036 #define MR_REG_M 0x02 00037 00038 #define OUT_X_H_M 0x03 00039 #define OUT_X_L_M 0x04 00040 #define OUT_Y_H_M 0x07 00041 #define OUT_Y_L_M 0x08 00042 #define OUT_Z_H_M 0x05 00043 #define OUT_Z_L_M 0x06 00044 00045 #define SR_REG_M 0x09 00046 #define IRA_REG_M 0x0A 00047 #define IRB_REG_M 0x0B 00048 #define IRC_REG_M 0x0C 00049 00050 #define WHO_AM_I_M 0x0F 00051 00052 #define OUT_Z_H_M 0x05 00053 #define OUT_Z_L_M 0x06 00054 #define OUT_Y_H_M 0x07 00055 #define OUT_Y_L_M 0x08 00056 00057 /** Tilt-compensated compass interface Library for the STMicro LSM303DLm 3-axis magnetometer, 3-axis acceleromter 00058 * @author Michael Shimniok http://www.bot-thoughts.com/ 00059 * 00060 * This is an early revision; I've not yet implemented heading calculation and the interface differs from my 00061 * earlier LSM303DLH; I hope to make this library drop in compatible at some point in the future. 00062 * setScale() and setOffset() have no effect at this time. 00063 * 00064 * @code 00065 * #include "mbed.h" 00066 * #include "LSM303DLM.h" 00067 * 00068 * LSM303DLM compass(p28, p27); 00069 * ... 00070 * int a[3], m[3]; 00071 * ... 00072 * compass.readAcc(a); 00073 * compass.readMag(m); 00074 * 00075 * @endcode 00076 */ 00077 class LSM303DLM { 00078 00079 public: 00080 /** Create a new interface for an LSM303DLM 00081 * 00082 * @param sda is the pin for the I2C SDA line 00083 * @param scl is the pin for the I2C SCL line 00084 */ 00085 LSM303DLM(PinName sda, PinName scl); 00086 00087 /** sets the x, y, and z offset corrections for hard iron calibration 00088 * 00089 * Calibration details here: 00090 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00091 * 00092 * If you gather raw magnetometer data and find, for example, x is offset 00093 * by hard iron by -20 then pass +20 to this member function to correct 00094 * for hard iron. 00095 * 00096 * @param x is the offset correction for the x axis 00097 * @param y is the offset correction for the y axis 00098 * @param z is the offset correction for the z axis 00099 */ 00100 void setOffset(float x, float y, float z); 00101 00102 /** sets the scale factor for the x, y, and z axes 00103 * 00104 * Calibratio details here: 00105 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00106 * 00107 * Sensitivity of the three axes is never perfectly identical and this 00108 * function can help to correct differences in sensitivity. You're 00109 * supplying a multipler such that x, y and z will be normalized to the 00110 * same max/min values 00111 */ 00112 void setScale(float x, float y, float z); 00113 00114 /** read the calibrated accelerometer and magnetometer values 00115 * 00116 * @param a is the accelerometer 3d vector, written by the function 00117 * @param m is the magnetometer 3d vector, written by the function 00118 */ 00119 void read(int a[3], int m[3]); 00120 00121 /** read the calibrated accelerometer values 00122 * 00123 * @param a is the accelerometer 3d vector, written by the function 00124 */ 00125 void readAcc(int a[3]); 00126 00127 /** read the calibrated magnetometer values 00128 * 00129 * @param m is the magnetometer 3d vector, written by the function 00130 */ 00131 void readMag(int m[3]); 00132 00133 /** sets the I2C bus frequency 00134 * 00135 * @param frequency is the I2C bus/clock frequency, either standard (100000) or fast (400000) 00136 */ 00137 void frequency(int hz); 00138 00139 private: 00140 I2C _device; 00141 char _data[6]; 00142 int offset[3]; 00143 int scale[3]; 00144 void init(); 00145 }; 00146 00147 #endif
Generated on Tue Jul 12 2022 18:32:34 by
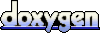