
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
Menu.h
00001 #define _ITEM_MAX 10 00002 #define NAMESIZ 20 00003 00004 typedef int (*FunctionPtr)(); 00005 00006 /** Simple menu interface model 00007 */ 00008 class Menu { 00009 public: 00010 00011 /** Create a new menu model 00012 */ 00013 Menu(); 00014 00015 /** add a new menu item 00016 */ 00017 void add(char *name, FunctionPtr f); 00018 00019 /** select the next menu item as the current item 00020 */ 00021 void next(void); 00022 00023 /** select the previous menu item as the current item 00024 */ 00025 void prev(void); 00026 00027 /** run the function associated with the current item 00028 */ 00029 void select(void); 00030 00031 /** return the text for the current item 00032 */ 00033 char *getItemName(void); 00034 00035 /** return text for a specified item 00036 */ 00037 char *getItemName(int i); 00038 00039 /** print all the menu items 00040 */ 00041 void printAll(void); 00042 00043 private: 00044 short _item; 00045 short _itemCount; 00046 char _name[_ITEM_MAX][NAMESIZ]; 00047 FunctionPtr _exec[_ITEM_MAX]; 00048 }; 00049
Generated on Tue Jul 12 2022 14:09:27 by
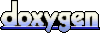