
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
IncrementalEncoder.cpp
00001 #include "IncrementalEncoder.h" 00002 00003 IncrementalEncoder::IncrementalEncoder(PinName pin): _lastTicks(0), _ticks(0), _new(false), _interrupt(pin) { 00004 _interrupt.mode(PullNone); // default is pulldown but my encoder board uses a pull-up and that just don't work 00005 _interrupt.rise(this, &IncrementalEncoder::_incRise); 00006 _interrupt.fall(this, &IncrementalEncoder::_incFall); 00007 _t.start(); 00008 _t.reset(); 00009 _lastTime = _t.read_us(); 00010 } 00011 00012 unsigned int IncrementalEncoder::read() { 00013 // disable interrupts? 00014 unsigned int ticks = _ticks - _lastTicks; 00015 _lastTicks = _ticks; 00016 _new=false; 00017 return ticks; 00018 } 00019 00020 unsigned int IncrementalEncoder::readTotal() { 00021 _new=false; 00022 return _ticks; 00023 } 00024 00025 unsigned int IncrementalEncoder::readRise() { 00026 _new=false; 00027 return _rise; 00028 } 00029 00030 unsigned int IncrementalEncoder::readFall() { 00031 _new=false; 00032 return _fall; 00033 } 00034 00035 unsigned int IncrementalEncoder::readTime() { 00036 return _time; 00037 } 00038 00039 void IncrementalEncoder::reset() { 00040 _ticks = _lastTicks = 0; 00041 } 00042 00043 void IncrementalEncoder::_increment() { 00044 _ticks++; 00045 } 00046 00047 #define A 0.3 // mix in how much from previous readings? 00048 void IncrementalEncoder::_incRise() { 00049 _rise++; 00050 _ticks++; 00051 _new=true; 00052 // compute time between ticks; only do this for rise 00053 // to eliminate jitter 00054 int now = _t.read_us(); 00055 _time = A*_time + (1-A)*(now - _lastTime); 00056 // Dead band: if _time > xxxx then turn off and wait until next tick 00057 // to start up again 00058 _lastTime = now; 00059 } 00060 00061 void IncrementalEncoder::_incFall() { 00062 _fall++; 00063 _ticks++; 00064 _new=true; 00065 }
Generated on Tue Jul 12 2022 14:09:25 by
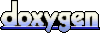