
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
HMC5843.h
00001 /** 00002 * @author Uwe Gartmann 00003 * @author Used HMC5843 library developed by Jose R. Padron and Aaron Berk as template 00004 * 00005 * @section LICENSE 00006 * 00007 * Copyright (c) 2010 ARM Limited 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 * 00027 * @section DESCRIPTION 00028 * 00029 * Honeywell HMC5843 digital compass. 00030 * 00031 * Datasheet: 00032 * 00033 * http://www.ssec.honeywell.com/magnetic/datasheets/HMC5843.pdf 00034 */ 00035 00036 #ifndef HMC5843_H 00037 #define HMC5843_H 00038 00039 #include "mbed.h" 00040 00041 #define HMC5843_I2C_ADDRESS 0x1E //7-bit address. 0x3C write, 0x3D read. 00042 #define HMC5843_I2C_WRITE 0x3C 00043 #define HMC5843_I2C_READ 0x3D 00044 00045 //Values Config A 00046 #define HMC5843_0_5HZ_NORMAL 0x00 00047 #define HMC5843_0_5HZ_POSITIVE 0x01 00048 #define HMC5843_0_5HZ_NEGATIVE 0x02 00049 00050 #define HMC5843_1HZ_NORMAL 0x04 00051 #define HMC5843_1HZ_POSITIVE 0x05 00052 #define HMC5843_1HZ_NEGATIVE 0x06 00053 00054 #define HMC5843_2HZ_NORMAL 0x08 00055 #define HMC5843_2HZ_POSITIVE 0x09 00056 #define HMC5843_2HZ_NEGATIVE 0x0A 00057 00058 #define HMC5843_5HZ_NORMAL 0x0C 00059 #define HMC5843_5HZ_POSITIVE 0x0D 00060 #define HMC5843_5HZ_NEGATIVE 0x0E 00061 00062 #define HMC5843_10HZ_NORMAL 0x10 00063 #define HMC5843_10HZ_POSITIVE 0x11 00064 #define HMC5843_10HZ_NEGATIVE 0x12 00065 00066 #define HMC5843_20HZ_NORMAL 0x14 00067 #define HMC5843_20HZ_POSITIVE 0x15 00068 #define HMC5843_20HZ_NEGATIVE 0x16 00069 00070 #define HMC5843_50HZ_NORMAL 0x18 00071 #define HMC5843_50HZ_POSITIVE 0x19 00072 #define HMC5843_50HZ_NEGATIVE 0x1A 00073 00074 //Values Config B 00075 #define HMC5843_0_7GA 0x00 00076 #define HMC5843_1_0GA 0x20 00077 #define HMC5843_1_5GA 0x40 00078 #define HMC5843_2_0GA 0x60 00079 #define HMC5843_3_2GA 0x80 00080 #define HMC5843_3_8GA 0xA0 00081 #define HMC5843_4_5GA 0xC0 00082 #define HMC5843_6_5GA 0xE0 00083 00084 //Values MODE 00085 #define HMC5843_CONTINUOUS 0x00 00086 #define HMC5843_SINGLE 0x01 00087 #define HMC5843_IDLE 0x02 00088 #define HMC5843_SLEEP 0x03 00089 00090 00091 00092 #define HMC5843_CONFIG_A 0x00 00093 #define HMC5843_CONFIG_B 0x01 00094 #define HMC5843_MODE 0x02 00095 #define HMC5843_X_MSB 0x03 00096 #define HMC5843_X_LSB 0x04 00097 #define HMC5843_Y_MSB 0x05 00098 #define HMC5843_Y_LSB 0x06 00099 #define HMC5843_Z_MSB 0x07 00100 #define HMC5843_Z_LSB 0x08 00101 #define HMC5843_STATUS 0x09 00102 #define HMC5843_IDENT_A 0x0A 00103 #define HMC5843_IDENT_B 0x0B 00104 #define HMC5843_IDENT_C 0x0C 00105 00106 00107 00108 /** 00109 * Interface library for the Honeywell HMC5843 digital compass. 00110 * @author Michael Shimniok http://www.bot-thoughts.com/ 00111 * @author Based on library by Uwe Gartmann 00112 * @author In turn based on HMC5843 library developed by Jose R. Padron and Aaron Berk as template 00113 * 00114 * This version is modified to follow the same member functions of my LSM303DLH library. The intent 00115 * is to make it drop in compatible with my other magnetometer libraries. For now, setScale() and 00116 * setOffset() have no effect at this time. 00117 * 00118 * @code 00119 * #include "mbed.h" 00120 * #include "HMC5843.h" 00121 * 00122 * HMC5843 compass(p28, p27); 00123 * ... 00124 * int m[3]; 00125 * ... 00126 * compass.readMag(m); 00127 * 00128 * @endcode 00129 */ 00130 class HMC5843 { 00131 00132 public: 00133 00134 /** 00135 * Create a new interface for an HMC5843. 00136 * 00137 * @param sda mbed pin to use for SDA line of I2C interface. 00138 * @param scl mbed pin to use for SCL line of I2C interface. 00139 */ 00140 HMC5843(PinName sda, PinName scl); 00141 00142 00143 /** sets the x, y, and z offset corrections for hard iron calibration 00144 * 00145 * Calibration details here: 00146 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00147 * 00148 * If you gather raw magnetometer data and find, for example, x is offset 00149 * by hard iron by -20 then pass +20 to this member function to correct 00150 * for hard iron. 00151 * 00152 * @param x is the offset correction for the x axis 00153 * @param y is the offset correction for the y axis 00154 * @param z is the offset correction for the z axis 00155 */ 00156 void setOffset(float x, float y, float z); 00157 00158 00159 /** sets the scale factor for the x, y, and z axes 00160 * 00161 * Calibratio details here: 00162 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00163 * 00164 * Sensitivity of the three axes is never perfectly identical and this 00165 * function can help to correct differences in sensitivity. You're 00166 * supplying a multipler such that x, y and z will be normalized to the 00167 * same max/min values 00168 */ 00169 void setScale(float x, float y, float z); 00170 00171 00172 /** 00173 * Enter into sleep mode. 00174 */ 00175 void setSleepMode(); 00176 00177 00178 /** 00179 * Set Device in Default Mode. 00180 * HMC5843_CONTINUOUS, HMC5843_10HZ_NORMAL HMC5843_1_0GA 00181 */ 00182 void setDefault(); 00183 00184 00185 /** 00186 * Set the operation mode. 00187 * 00188 * @param mode 0x00 -> Continuous 00189 * 0x01 -> Single 00190 * 0x02 -> Idle 00191 * @param ConfigA values 00192 * @param ConfigB values 00193 */ 00194 void setOpMode(int mode, int ConfigA, int ConfigB); 00195 00196 00197 /** 00198 * Write to a register on the device. 00199 * 00200 * @param address is the register address to be written. 00201 * @param data is the data to write. 00202 */ 00203 void write(int address, int data); 00204 00205 00206 /** read the calibrated accelerometer and magnetometer values 00207 * 00208 * @param m is the magnetometer 3d vector, written by the function 00209 */ 00210 void read(int m[3]); 00211 00212 00213 /** read the calibrated magnetometer values 00214 * 00215 * @param m is the magnetometer 3d vector, written by the function 00216 */ 00217 void readMag(int m[3]); 00218 00219 00220 /** 00221 * Get the output of all three axes. 00222 * 00223 * @param Pointer to a buffer to hold the magnetics value for the 00224 * x-axis, y-axis and z-axis [in that order]. 00225 */ 00226 void readData(int* readings); 00227 00228 00229 /** 00230 * Get the output of X axis. 00231 * 00232 * @return x-axis magnetic value 00233 */ 00234 int getMx(); 00235 00236 00237 /** 00238 * Get the output of Y axis. 00239 * 00240 * @return y-axis magnetic value 00241 */ 00242 int getMy(); 00243 00244 00245 /** 00246 * Get the output of Z axis. 00247 * 00248 * @return z-axis magnetic value 00249 */ 00250 int getMz(); 00251 00252 /** 00253 * Get the current operation mode. 00254 * 00255 * @return Status register values 00256 */ 00257 int getStatus(void); 00258 00259 00260 /** 00261 * Read the memory location on the device which contains the address. 00262 * 00263 * @param Pointer to a buffer to hold the address value 00264 * Expected H, 4 and 3. 00265 */ 00266 void getAddress(char * address); 00267 00268 00269 /** sets the I2C bus frequency 00270 * 00271 * @param frequency is the I2C bus/clock frequency, either standard (100000) or fast (400000) 00272 */ 00273 void frequency(int hz); 00274 00275 private: 00276 I2C* i2c_; 00277 00278 }; 00279 00280 #endif /* HMC5843_H */
Generated on Tue Jul 12 2022 14:09:25 by
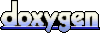