Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
SetPhysicsProperties.h
00001 #ifndef _ROS_SERVICE_SetPhysicsProperties_h 00002 #define _ROS_SERVICE_SetPhysicsProperties_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 #include "geometry_msgs/Vector3.h" 00008 #include "gazebo_msgs/ODEPhysics.h" 00009 00010 namespace gazebo_msgs 00011 { 00012 00013 static const char SETPHYSICSPROPERTIES[] = "gazebo_msgs/SetPhysicsProperties"; 00014 00015 class SetPhysicsPropertiesRequest : public ros::Msg 00016 { 00017 public: 00018 typedef double _time_step_type; 00019 _time_step_type time_step; 00020 typedef double _max_update_rate_type; 00021 _max_update_rate_type max_update_rate; 00022 typedef geometry_msgs::Vector3 _gravity_type; 00023 _gravity_type gravity; 00024 typedef gazebo_msgs::ODEPhysics _ode_config_type; 00025 _ode_config_type ode_config; 00026 00027 SetPhysicsPropertiesRequest(): 00028 time_step(0), 00029 max_update_rate(0), 00030 gravity(), 00031 ode_config() 00032 { 00033 } 00034 00035 virtual int serialize(unsigned char *outbuffer) const 00036 { 00037 int offset = 0; 00038 union { 00039 double real; 00040 uint64_t base; 00041 } u_time_step; 00042 u_time_step.real = this->time_step; 00043 *(outbuffer + offset + 0) = (u_time_step.base >> (8 * 0)) & 0xFF; 00044 *(outbuffer + offset + 1) = (u_time_step.base >> (8 * 1)) & 0xFF; 00045 *(outbuffer + offset + 2) = (u_time_step.base >> (8 * 2)) & 0xFF; 00046 *(outbuffer + offset + 3) = (u_time_step.base >> (8 * 3)) & 0xFF; 00047 *(outbuffer + offset + 4) = (u_time_step.base >> (8 * 4)) & 0xFF; 00048 *(outbuffer + offset + 5) = (u_time_step.base >> (8 * 5)) & 0xFF; 00049 *(outbuffer + offset + 6) = (u_time_step.base >> (8 * 6)) & 0xFF; 00050 *(outbuffer + offset + 7) = (u_time_step.base >> (8 * 7)) & 0xFF; 00051 offset += sizeof(this->time_step); 00052 union { 00053 double real; 00054 uint64_t base; 00055 } u_max_update_rate; 00056 u_max_update_rate.real = this->max_update_rate; 00057 *(outbuffer + offset + 0) = (u_max_update_rate.base >> (8 * 0)) & 0xFF; 00058 *(outbuffer + offset + 1) = (u_max_update_rate.base >> (8 * 1)) & 0xFF; 00059 *(outbuffer + offset + 2) = (u_max_update_rate.base >> (8 * 2)) & 0xFF; 00060 *(outbuffer + offset + 3) = (u_max_update_rate.base >> (8 * 3)) & 0xFF; 00061 *(outbuffer + offset + 4) = (u_max_update_rate.base >> (8 * 4)) & 0xFF; 00062 *(outbuffer + offset + 5) = (u_max_update_rate.base >> (8 * 5)) & 0xFF; 00063 *(outbuffer + offset + 6) = (u_max_update_rate.base >> (8 * 6)) & 0xFF; 00064 *(outbuffer + offset + 7) = (u_max_update_rate.base >> (8 * 7)) & 0xFF; 00065 offset += sizeof(this->max_update_rate); 00066 offset += this->gravity.serialize(outbuffer + offset); 00067 offset += this->ode_config.serialize(outbuffer + offset); 00068 return offset; 00069 } 00070 00071 virtual int deserialize(unsigned char *inbuffer) 00072 { 00073 int offset = 0; 00074 union { 00075 double real; 00076 uint64_t base; 00077 } u_time_step; 00078 u_time_step.base = 0; 00079 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00080 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00081 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00082 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00083 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00084 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00085 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00086 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00087 this->time_step = u_time_step.real; 00088 offset += sizeof(this->time_step); 00089 union { 00090 double real; 00091 uint64_t base; 00092 } u_max_update_rate; 00093 u_max_update_rate.base = 0; 00094 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00095 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00096 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00097 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00098 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00099 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00100 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00101 u_max_update_rate.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00102 this->max_update_rate = u_max_update_rate.real; 00103 offset += sizeof(this->max_update_rate); 00104 offset += this->gravity.deserialize(inbuffer + offset); 00105 offset += this->ode_config.deserialize(inbuffer + offset); 00106 return offset; 00107 } 00108 00109 const char * getType(){ return SETPHYSICSPROPERTIES; }; 00110 const char * getMD5(){ return "abd9f82732b52b92e9d6bb36e6a82452"; }; 00111 00112 }; 00113 00114 class SetPhysicsPropertiesResponse : public ros::Msg 00115 { 00116 public: 00117 typedef bool _success_type; 00118 _success_type success; 00119 typedef const char* _status_message_type; 00120 _status_message_type status_message; 00121 00122 SetPhysicsPropertiesResponse(): 00123 success(0), 00124 status_message("") 00125 { 00126 } 00127 00128 virtual int serialize(unsigned char *outbuffer) const 00129 { 00130 int offset = 0; 00131 union { 00132 bool real; 00133 uint8_t base; 00134 } u_success; 00135 u_success.real = this->success; 00136 *(outbuffer + offset + 0) = (u_success.base >> (8 * 0)) & 0xFF; 00137 offset += sizeof(this->success); 00138 uint32_t length_status_message = strlen(this->status_message); 00139 varToArr(outbuffer + offset, length_status_message); 00140 offset += 4; 00141 memcpy(outbuffer + offset, this->status_message, length_status_message); 00142 offset += length_status_message; 00143 return offset; 00144 } 00145 00146 virtual int deserialize(unsigned char *inbuffer) 00147 { 00148 int offset = 0; 00149 union { 00150 bool real; 00151 uint8_t base; 00152 } u_success; 00153 u_success.base = 0; 00154 u_success.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00155 this->success = u_success.real; 00156 offset += sizeof(this->success); 00157 uint32_t length_status_message; 00158 arrToVar(length_status_message, (inbuffer + offset)); 00159 offset += 4; 00160 for(unsigned int k= offset; k< offset+length_status_message; ++k){ 00161 inbuffer[k-1]=inbuffer[k]; 00162 } 00163 inbuffer[offset+length_status_message-1]=0; 00164 this->status_message = (char *)(inbuffer + offset-1); 00165 offset += length_status_message; 00166 return offset; 00167 } 00168 00169 const char * getType(){ return SETPHYSICSPROPERTIES; }; 00170 const char * getMD5(){ return "2ec6f3eff0161f4257b808b12bc830c2"; }; 00171 00172 }; 00173 00174 class SetPhysicsProperties { 00175 public: 00176 typedef SetPhysicsPropertiesRequest Request; 00177 typedef SetPhysicsPropertiesResponse Response; 00178 }; 00179 00180 } 00181 #endif
Generated on Tue Jul 12 2022 18:49:20 by
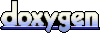